27 Javascript Function Return Array
Jul 26, 2021 - It checks all the elements of the array and whichever the first element satisfies the condition is going to print. ... thisValue: This parameter is optional, if a value to be passed to the function to be used as its “this” value else the value “undefined” will be passed as its “this” value. Return ... Feb 21, 2014 - Participate in discussions with other Treehouse members and learn.
The JavaScript filter function iterates over the existing values in an array and returns the values that pass. The search criteria in the JavaScript filter function are passed using a callbackfn. Arrow functions can also be used to make JavaScript filter array code more readable. Syntax of JavaScript filter(): array.filter(function(value, index ...
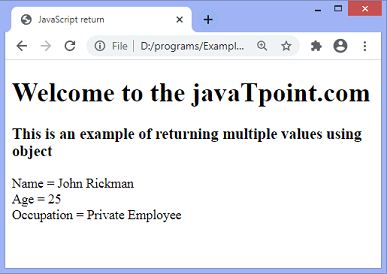
Javascript function return array. Write a function filterRange(arr, a, b) that gets an array arr, looks for elements with values higher or equal to a and lower or equal to b and return a result as an array. The function should not modify the array. It should return the new array. For instance: May 20, 2020 - Add the new candy object to the end of the array. Return the updated inventory array with the new candy object added to the end. javascript ... Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript Jul 20, 2021 - The values() method returns a new Array Iterator object that contains the values for each index in the array.
JavaScript filter syntax: let myArray = array.filter(callback(element[, index[, arr]])[, thisArg]) JavaScript. Copy. myArray - The new array which is going to be returned. array - You will be running the filter function on this array. callback - You will be employing this function to see if the elements in the array pass certain test or not. filter () - returns an array of all items for which the function returns true. forEach () - no return value (just run the function on every element in the list) map () - returns a new list with the result of each item in an array. some () - returns true if the function returns true for at least one of the items. PHP Array Functions PHP String Functions PHP File System Functions PHP Date/Time Functions PHP Calendar Functions PHP MySQLi Functions PHP Filters PHP Error Levels ... A function cannot return multiple values. However, you can get the similar results by returning an array containing multiple values.
The JavaScript find () method is used to returns a value of the first element in an array that satisfied the provided testing function, otherwise the return will be undefined. The map () method does not execute the function for array elements without values. Array.Prototype.filter (): It is used to get a new array that has only those array elements which pass the test implemented by the callback function. It accepts a callback function as an argument. This callback function has to return a true or false. Elements for which the callback function returned true are added to the newly returned array. 13/9/2016 · Second, you want to return an Object, not an Array. An object can be assigned property values akin to an associative array or hash -- an array cannot. So we change the declaration of var IDs = new Array();to var IDs = new Object();. After those changes your code will run fine, but it can be simplified further.
Function Definitions. In JavaScript, functions can be declared using the function keyword, followed by the function name, and, in parantheses, the list of parameters (without a var declaration). The actual function definition is enclosed in curly brackets. The return keyword is used to return a value or just terminate the function. Anonymous functions are often passed as arguments to higher-order functions or are used as the result of a higher-order function that requires to return a function. Here is a classic example of how to use an anonymous function: setTimeout (function {console. log ("This will be displayed in a second")}, 1000) Example 2: Sorting using Custom Function. When compareFunction is passed,. All non-undefined array elements are sorted according to the return value of compareFunction.All undefined elements are sorted to the end of the array and compareFunction is not called for them.; Suppose we want to sort the above names array such that the longest name comes last, rather than sorting it alphabetically.
Search the array for an element, starting at the end, and returns its position. map () Creates a new array with the result of calling a function for each array element. pop () Removes the last element of an array, and returns that element. push () Adds new elements to the end of an array, and returns the new length. 6/4/2018 · When you pass an array into a function in JavaScript, it is passed as a reference. Anything you do that alters the array inside the function will also alter the original array. for example this... Add the new candy object to the end of the array. Return the updated inventory array with the new candy object added to the end. javascript
Jul 07, 2020 - Functions return only one value. How can we simulate returning multiple values from a function? Array.from () lets you create Array s from: array-like objects (objects with a length property and indexed elements); or iterable objects (objects such as Map and Set). Array.from () has an optional parameter mapFn, which allows you to execute a map () function on each element of the array being created. Apr 09, 2020 - JavaScript doesn’t support functions that return multiple values. However, you can wrap multiple values into an array or an object and return the array or the object. Use destructuring assignment syntax to unpack values from the array, or properties from objects. ... Primitive vs. Reference Values ... The JavaScript ...
Return an array from a function : Introduction « Array « JavaScript Tutorial. Home; JavaScript Tutorial; Language Basics; Operators; Statement; Development; Number Data Type; String; Function; ... Return an array from a function : Introduction « Array « JavaScript Tutorial. JavaScript Tutorial; Step 1 — Calling a Function on Each Item in an Array.map () accepts a callback function as one of its arguments, and an important parameter of that function is the current value of the item being processed by the function. This is a required parameter. With this parameter, you can modify each item in an array and create a new function. If it's an array, use [] in place of "new Object ()" and then after those statements, return obj; will return an array. No I want to know how to return associative arrays ? May 29 '08 # 7
Jan 21, 2021 - Wurundjeri Country 313 Healesville-Yarra Glen Road Healesville VIC 3777 Australia · © Copyright 2020 TarraWarrra Museum of Art | Disclaimer and Copyright The find() method returns the value of the first array element that satisfies the provided test function. Example let numbers = [1, 3, 4, 9, 8]; // function to check even number function isEven(element) { return element % 2 == 0; } Write a JavaScript function to get the last element of an array. Passing a parameter 'n' will return the last 'n' elements of the array.
The array.values () function is an inbuilt function in JavaScript which is used to returns a new array Iterator object that contains the values for each index in the array i.e, it prints all the elements of the array. Syntax: arr.values () Return values: It returns a new array iterator object i.e, elements of the given array. The function isOdd() accepts an array and has a second optional parameter for an array. If not provided, the array has a default value of an empty array. The function checks each number in the array to see if it is an odd number. If the number is odd, it adds it to the array from the second parameter. After all numbers are checked, the array ... Arrays in JavaScript can work both as a queue and as a stack. They allow you to add/remove elements both to/from the beginning or the end. In computer science the data structure that allows this, is called deque. ... Write the function getMaxSubSum(arr) that will return that sum.
1 week ago - The properties and elements returned from this match are as follows: ... Creates a new Array object. ... The constructor function is used to create derived objects. Allows a function to return an array. Example function disp():string[] { return new Array("Mary","Tom","Jack","Jill") } var nums:string[] = disp() for(var i in nums) { console.log(nums[i]) } On compiling, it will generate following JavaScript … In this case, we return all the selected option s' values on the screen: let elems = document.querySelectorAll('select option:checked') let values = Array. prototype.map.call( elems, function(obj) { return obj. value }) Copy to Clipboard. An easier way would be the Array.from () method.
Write a JavaScript function which returns the n rows by n columns identity matrix. Go to the editor Click me to see the solution. 11. Write a JavaScript function which will take an array of numbers stored and find the second lowest and second greatest numbers, respectively. Go to the editor Sample array : [1,2,3,4,5] Expected Output : 2,4 Using the arrays in JavaScript, we can return multiple elements from a function. First we need to store all the elements that we want to return from the function in the array. We can use the array.push () function to push elements in the array and then at the end of function using the return statement, we return the array. 20/7/2020 · Accessing an array returned by a function in JavaScript. Javascript Web Development Object Oriented Programming. Following is the code for accessing an array returned by a function in JavaScript −.
In this example, person.firstName returns John: ... JavaScript variables can be objects. Arrays are special kinds of objects. Because of this, you can have variables of different types in the same Array. You can have objects in an Array. You can have functions in an Array. How to Reverse an Array in JavaScript with the Slice and Reverse Methods . The slice method is used to return the selected elements as a new array. When you call the method without any argument, it will return a new array that's identical to the original (from the first element to the last). Next, you call the reverse method on the newly ... function arrayFromArgs() { return Array .prototype.slice.call ( arguments ); } var fruits = arrayFromArgs ( 'Apple', 'Orange', 'Banana' ); console .log (fruits); Code language: JavaScript (javascript) ES6 introduces the Array.from () method that creates a new instance of the Array from an array-like or iterable object.
5 Ways To Convert Array Of Objects To Object In Javascript
How To Find Even Numbers In An Array Using Javascript
Learn Return Next Element With A Function Javascript The
Javascript Foreach 10 Javascript Array Methods You Should
Define A Javascript Function Named Smaller That Takes Chegg Com
Return Multiple Results From Your Custom Function Office
Should You Use Includes Or Filter To Check If An Array
Javascript Merge Array Of Objects By Key Es6 Reactgo
3 Pragmatic Uses Of Javascript Array Slice Method
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
Javascript Array Findindex Method
Top 11 And More Must Know Javascript Functions
Return An Array In C Javatpoint
Breaking The Loop How To Use Higher Order Functions To
Useful Javascript Array And Object Methods By Robert Cooper
Remove Duplicates From An Array Javascript Tuts Make
How To Access Object Array Values In Javascript Stack Overflow
Detailed Explanation Of Native Array Method In Javascript
Dynamic Array In Javascript Using An Array Literal And
How To Remove Array Duplicates In Es6 By Samantha Ming
Find The Min Max Element Of An Array In Javascript Stack
Javascript Array Push How To Add Element In Array
5 Easy Ways To Vlookup And Return Multiple Values
Javascript Reducer A Simple Yet Powerful Array Method
0 Response to "27 Javascript Function Return Array"
Post a Comment