26 How To Check If Object Is Undefined In Javascript
In javascript, we can check if an object is empty or not by using. JSON.stringify. Object.keys (ECMA 5+) Object.entries (ECMA 7+) And if you are using any third party libraries like jquery, lodash, Underscore etc you can use their existing methods for checking javascript empty object. Checking for undefined-ness is not an accurate way of testing whether a key exists. ... javascript javascript check if key exists in map javascript check if value exists in array javascript object contains value javascript check if object exists in array if value in object javascript check if object has key javascript es6 how to check if a json ...
Uncaught Typeerror Cannot Read Property Of Undefined
To check null in JavaScript, use triple equals operator (===) or Object.is () method. To find the difference between null and undefined, use the triple equality operator or Object.is () method. To loosely check if the variable is null, use a double equality operator (==). The double equality operator can not tell the difference between null and ...
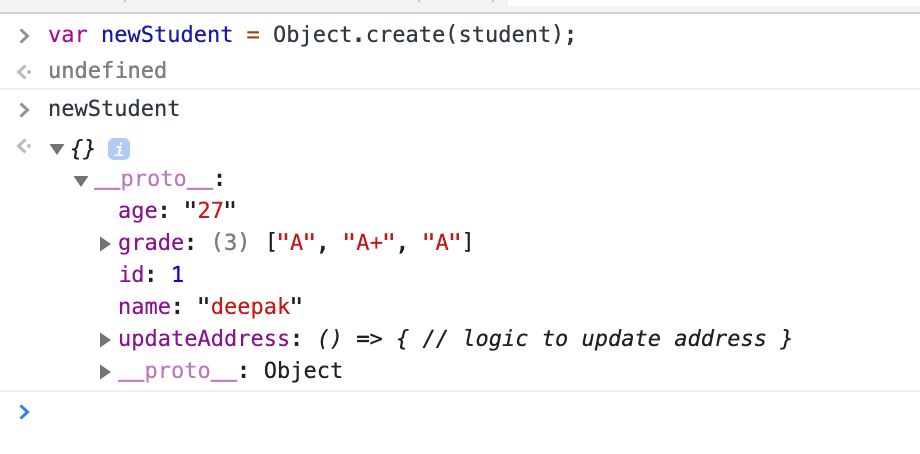
How to check if object is undefined in javascript. At the end we see that even though null and undefined are considered equal, they are not the same identity (equal without type conversion).As discussed, this is because they are of different types behind the scenes: null being an object and undefined being an undefined type. With that out of the way we can start to understand why trying to access a property of null or undefined may fail. The above expression produces undefined if obj, obj.level1, or obj.level1.method are null or undefined; otherwise, it will call the function. The optional chaining operator¶ In a JavaScript, the correct way to check if an object property is undefined is to use the typeof operator. The typeof operator returns a string indicating the type of the unevaluated operand. If the value is not defined, typeof returns the 'undefined' string.
How to check for null, undefined, empty variables in JavaScript where the variable could be anything like array or object, string, number, Boolean? Hi, coder. So, this is one of the most asked questions that how to check null, undefined, empty variables in JavaScript? Check by Value (Strict equality Operator): Here you will get that variable is assigned a value or not if the variable is not assigned a value it will display undefined. Fortunately, JavaScript offers a bunch of ways to determine if the object has a specific property: obj.prop !== undefined: compare against undefined directly. typeof obj.prop !== 'undefined': verify the property value type. obj.hasOwnProperty ('prop'): verify whether the object has an own property.
To check undefined in JavaScript, use (===) triple equals operator. In modern browsers, you can compare the variable directly to undefined using the triple equals operator. There are also two more ways to check if the variable is undefined or not in JavaScript, but those ways are not recommended. Those two are the following. The first way is to invoke object.hasOwnProperty (propName). The method returns true if the propName exists inside object, and false otherwise. hasOwnProperty () searches only within the own properties of the object. The second approach makes use of propName in object operator. If you are still concerned, there are two ways to check if a value is undefined even if the global undefined has been overwritten. You can use the void operator to obtain the value of undefined. This will work even if the global window.undefined value has been over-written: if (name === void (0)) {...}
The typeof operator for undefined value returns undefined. Hence, you can check the undefined value using typeof operator. Also, null values are checked using the === operator. Note: We cannot use the typeof operator for null as it returns object. Get code examples like"how to check if object is undefined in javascript". Write more code and save time using our ready-made code examples. Here's a Code Recipe to check if an object is empty or not. For newer browsers, you can use plain vanilla JS and use the new "Object.keys" 🍦 But for older browser support, you can install the Lodash library and use their "isEmpty" method 🤖 const empty = {}; Object.keys(empty).length === 0 && empty.constructor === Object _.isEmpty(empty)
If myObject is "falsey" (e.g. undefined) the && operator won't bother trying to evaluate the right-hand expression, thereby avoiding the attempt to reference a property of a non-existent object. The variable myObject must have course already have been declared, the test above is for whether it has been assigned a defined value. JavaScript variables start with the value of undefined if they are not given a value when they are declared (or initialized) using var, let, or const. Variables that have not been declared usually... C hecking for null is a common task that every JavaScript developer has to perform at some point or another. The typeof keyword returns "object" for null, so that means a little bit more effort is required. Comparisons can be made: null === null to check strictly for null or null == undefined to check loosely for either null or undefined.
JavaScript undefined and null: Let's talk about it one last time! JavaScript: Equality comparison with ==, === and Object.is; The JavaScript `this` Keyword + 5 Key Binding Rules Explained for JS Beginners; That's all for now. See you again with my next article soon. Until then, please take good care of yourself. In simple words you can say a null value means no value or absence of a value, and undefined means a variable that has been declared but no yet assigned a value. To check if a variable is undefined or null you can use the equality operator == or strict equality operator === (also called identity operator). The easiest way to check if an object property exists but is undefined in JavaScript is to make use of the Object.prototype.hasOwnProperty method which will let you find properties in an object.
In versions of JavaScript prior to ECMAScript 5, the property named "undefined" on the global object was writeable, and therefore a simple check foo === undefinedmight behave unexpectedly if it had accidentally been redefined. In modern JavaScript, the property is read-only. In a JavaScript program, the correct way to check if an object property is undefined is to use the typeof operator. typeof returns a string that tells the type of the operand. It is used without parentheses, passing it any value you want to check: check undefined object javascript one liner set to emtpy; check type javascript; how to check if array is empty or not in javascript; query selector has clas; classList has class; javascript hasclass; convert empty string to boolean javascript; javascript check if boolean is undefined; isset js;
The hasOwnProperty () method returns true if the specified property is a direct property of the object — even if the value is null or undefined. The method returns false if the property is inherited, or has not been declared at all. Unlike the in operator, this method does not check for the specified property in the object's prototype chain. The in operator is another way to check the presence of a property in an object in JavaScript. It returns true if the property exists in an object. Otherwise, it returns false. Let us use the in operator to look for the cake property in the food object: Using typeof operator We can use the typeof operator to check if an object property is undefined. The typeof operator returns the string representation of a given object property type. if (typeof obj.age === 'undefined'){ console.log('age property is undefined'); }
In JavaScript obj.propName === undefined is true if either obj has a property 'propName' and the property's value is trictly equal undefined, or if obj does not have a property 'propName'. If you want to check whether obj has a property and that property is strictly equal to undefined, you should use the in operator. You can use the typeof operator in combination with the strict equality operator (===) to check or detect if a JavaScript object property is undefined. Let's take a look at the following example to understand how it actually works:
Remove Unnecessary Check In Gettag Js Issue 4683 Lodash
3 Ways To Clone Objects In Javascript Samanthaming Com
Undefined Vs Null Find Out The Top 8 Most Awesome Differences
Everything About Javascript Objects By Deepak Gupta
Is There A Standard Function To Check For Null Undefined Or
Check If Folder Or File Exists Using Javascript Phpcoder Tech
Javascript Claims Object Is Undefined When It S Been Checked
Understanding Javascript Execution Context By Examples
How To Check Property Exists In Javascript Object In 2021
7 Tips To Handle Undefined In Javascript
Undefined Vs Null Find Out The Top 8 Most Awesome Differences
Set Default Values To Variables If Null Or Undefined Javascript
Null And Undefined In Javascript What S The Difference
Top 10 Javascript Errors From 1000 Projects And How To
How To Check For An Undefined Or Null Variable In Javascript
Javascript Error Handling Typeerror Null Or Undefined Has
How To Check For Undefined In Javascript By Dr Derek
Solved Q Which Of The Fol Answers Course
Here S Why Mapping A Constructed Array In Javascript Doesn T
Top 10 Javascript Errors From 1000 Projects And How To
How To Check If A Variable In Javascript Is Undefined Or Null
How To Replace A Value If Null Or Undefined In Javascript
The 10 Most Common Mistakes Javascript Developers Make Toptal
Determine If Variable Is Undefined Or Null
7 Tips To Handle Undefined In Javascript
0 Response to "26 How To Check If Object Is Undefined In Javascript"
Post a Comment