33 Integer Part Of A Number Javascript
Given a float number, The task is to separate the number into the integer and decimal part using JavaScript. For example, a value of 15.6 would be split into two numbers, i.e. 15 and 0.6 Here are few methods discussed. Method 1: Using split () method. This method is used to split a string into an array of substrings, and returns the new array. Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. Generate random whole numbers within a range javascript
Javascript Function To Add Two Integer Numbers
The number from a string in javascript can be extracted into an array of numbers by using the match method. This function takes a regular expression as an argument and extracts the number from the string. Regular expression for extracting a number is (/ (\d+)/). Example 1: This example uses match () function to extract number from string.
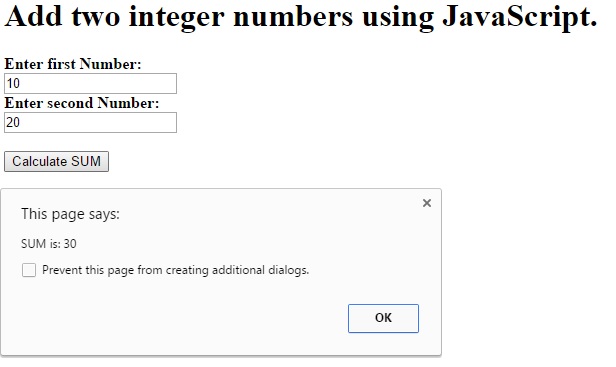
Integer part of a number javascript. Get decimal portion of a number with JavaScript (12) I have float numbers like 3.2 and 1.6. I need to separate the number into the integer and decimal part. For example, a value of 3.2 would be split into two numbers, i.e. 3 and 0.2. Getting the integer portion is easy: n = Math.floor(n); Getting the integer part from a number in Javascript. Published Jan 28, 2020, 12:15. Just a thing I learned today: using the bitwise not operator (~) on a number in Javascript ignores its fractional part (it converts it to integer first), therefore using it twice gives you the integer part of original number. Details. Mathematical function, suitable for both symbolic and numerical manipulation. IntegerPart [ x] in effect takes all digits to the left of the decimal point and drops the others. IntegerPart [ x] + FractionalPart [ x] is always exactly x. IntegerPart [ x] returns an integer when x is any numeric quantity, whether or not it is an explicit ...
Jul 10, 2020 - Return Value: The Math.trunc() method returns the integer part of the given number. More codes for the above method are as follows: Program 1: When a negative number of float type is passed as a parameter On This Page # Using Math.trunc () Introduced in ES6, Math.trunc () returns the truncated integer part of a number (which in case of a decimal number would remove the fractional digits, and return only the integer part). To illustrate this, let's look at the following examples: In JavaScript, a number can be a primitive value (typeof = number) or an object (typeof = object). The valueOf() method is used internally in JavaScript to convert Number objects to primitive values. There is no reason to use it in your code.
This method below allows you to get a specific number from a int value based on a specific index. public static int getSpecificNum(int number, int index) { int numOfDigits = 0; int pow = 1, test = 0, counter = 0; //gets the number of digits. 1 week ago - The Math.trunc() function returns the integer part of a number by removing any fractional digits. But you have to decide on a sign ... or to a JavaScript number, which has an explicit sign as opposed to an implicit one. Therefore, signed 32-bit integers are partitioned into two groups: Highest bit is 0: number is zero or positive. Highest bit is 1: number is negative. The highest bit is often called the ...
The remainder / modulus operator ( %) returns the remainder after (integer) division. This operator returns the remainder left over when one operand is divided by a second operand. When the first operand is a negative value, the return value will always be negative, and vice versa for positive values. In the example above, 10 can be subtracted ... There are of course other ways to cast to Number in Javascript, including but not limited to: Number() parseInt() parseFloat() ~~ >>>0; For a full chart of what works when, please refer here. I ... Nov 06, 2017 - In JavaScript, you can represent a number is an actual number (ex. 42), or as a string (ex. '42'). If you were to use a strict comparison to compare the two, it would fail because they’re two different types of objects.
2. How to Convert a String into an Integer¶. Use parseInt() function, which parses a string and returns an integer.. The first argument of parseInt must be a string.parseInt will return an integer found in the beginning of the string. Remember, that only the first number in the string will be returned. Here is the code, function getDecimal ( n) { return ( n - Math.floor( n)); } console.log(5.224); //Output: 0.2240000000000002. In this code, we are creating a function that will accept the floating number from the argument 'n' and use this value in the function. Now, to return just the decimal part we have to subtract the number counterpart ... In JavaScript, floor() is a function that is used to return the largest integer value that is less than or equal to a number. In other words, the floor() function rounds a number down and returns an integer value. Because the floor() function is a static function of the Math object, it must ...
For getting the integer part, you can't get much simpler than. TRUNC (x) as Mtefft said above. Another way of getting the fractional part is. MOD (x, 1) The result has the same sign as the argument, so MOD (-1.2, 1) is -.2. You can use ABS (MOD (x, 1)) if you never want negative results. Getting the Integer Portion from a Division. JavaScript. MeesterSluggo. August 30, 2014, 3:30am #1. Hello, Basically I'm seeking the opposite of the mod (%) operator. For example, if I divide 14 ... JavaScript Program to Find the Factors of a Number In this example, you will learn to write a JavaScript program that finds all the factors of an integer. To understand this example, you should have the knowledge of the following JavaScript programming topics:
The Math.trunc () method can be used to truncate the fractional portion of a number. The number can also be rounded to the next integer using Math.round (), Math.ceil () and Math.floor () methods. Getting the Number without Fractional Units The Math.trunc () method simply truncates the decimal part of the number and returns the integer part. The floor () method rounds a number DOWNWARDS to the nearest integer, and returns the result. If the passed argument is an integer, the value will not be rounded. parseInt () is widely used in JavaScript. With this function, we can convert the values of different data types to the integer type. In this section, we will see the conversion of a float number to an integer number. The syntax of parseInt () is parseInt (<value>).
Jul 17, 2014 - The parseInt() function parses a string and returns an integer. The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript ... Nov 13, 2011 - How can you find the whole number of a given decimal or other number in JavaScript? Given Result ----- ------ 1.2 1 1.5 1 1.9 1 What's the best way to perform this for both positive ... Javascript Web Development Front End Technology To remove the decimal part from a number, use the JavaScript Math.trunc () method. The method returns the integer part of a number by removing the decimal part.
The Number object contains only the default methods that are a part of every object's definition. Forces a number to display in exponential notation, even if the number is in the range in which JavaScript normally uses standard notation. Formats a number with a specific number of digits to the right of the decimal. Nov 12, 2020 - Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. ... Write a javascript program to find roots of quadratic equation. Jul 08, 2020 - How to ignore loop in else condition using JavaScript ? ... Below is the example of the Math floor() Method. ... The Math.floor method is used to round off the number passed as a parameter to its nearest integer in Downward direction of rounding i.e. towards the lesser value.
You can use parseInt() function to get the integer part than use that to extract the decimal part var myNumber = 3.2; var integerPart = parseInt(myNumber); var decimalPart = myNumber - integerPart; Or … Get only the integer part of a float number on javascript with ~~1.6. #javascript. #stuff. Dec 28, 2015 - I'd like to convert a float to a whole number in JavaScript. Actually, I'd like to know how to do BOTH of the standard conversions: by truncating and by rounding. And efficiently, not via convertin...
How do I separate the integer and decimal part of a number, ie. If I had 123456, and divided it by 1000 = 123.456, how do I obtain 123 and 456 as seperate variables? This is the sort of thing that is done using the "int" and "mod" function in Excel Just use Math.floor and % (modulus operator). var intPart = Math.floor(123456 / 1000); The base can vary from 2 to 36.By default it's 10.. Common use cases for this are: base=16 is used for hex colors, character encodings etc, digits can be 0..9 or A..F.. base=2 is mostly for debugging bitwise operations, digits can be 0 or 1.. base=36 is the maximum, digits can be 0..9 or A..Z.The whole latin alphabet is used to represent a number. A funny, but useful case for 36 is when we ... In JavaScript, trunc () is a function that is used to return the integer portion of a number. It truncates the number and removes all fractional digits. Because the trunc () function is a static function of the Math object, it must be invoked through the placeholder object called Math.
There are 8 basic data types in JavaScript. number for numbers of any kind: integer or floating-point, integers are limited by ± (2 53 -1). bigint is for integer numbers of arbitrary length. string for strings. A string may have zero or more characters, there's no separate single-character type. Mar 14, 2018 - Ways of iterating over a array in JavaScript. How to ignore loop in else condition using JavaScript ? ... The Math.round() function in JavaScript is used to round the number passed as parameter to its nearest integer. Syntax One way to square a number in JavaScript is to use the pow () method from the Math library. The function takes two arguments: the first one is our target variable or value, and the second is the number of times we want to multiply it by itself. In case we want to square that number, we will send 2 as the second argument.
In JavaScript, Number type can only represent numbers less than (253 - 1) and more than - (253 - 1). However, if you need to use a larger number than that, you can use the BigInt data type. A BigInt number is created by appending n to the end of an integer. Apr 15, 2019 - Math.round (floating argument): Round a number to its nearest integer. Syntax: ... Math.trunc (floating argument): Return the integer part of a floating-point number by removing the fractional digits. Syntax: Jan 28, 2020 - Just a thing I learned today: using the bitwise not operator (~) on a number in Javascript ignores its fractional part (it converts it to integer first), therefore using it twice gives you the integer part of original number. Thanks to fetishlace for clarifications.
The second bit of good news is that unlike some other programming languages, JavaScript only has one data type for numbers, both integers and decimals — you guessed it, Number. This means that whatever type of numbers you are dealing with in JavaScript, you handle them in exactly the same way.
How To Convert A String To A Number In Javascript
Javascript Check If A Value Is A Integer Number Code Example
Https Www Codecademy Com Paths Web Development Tracks
How To Get Decimal Portion Of A Number Using Javascript
Javascript Round Method Math Object W3resource
Pros And Cons Of Javascript Weigh Them And Choose Wisely
How To Convert A String To A Number In Javascript
Floor Function To Get Highest Lower Integer Of A Number In
Reverse An Integer With Javascript By Ngoc Vuong Medium
Javascript Parseint Convert A String To Integer Number In
Integer Part From Wolfram Mathworld
Javascript Math Check Whether A Value Is An Integer Or Not
How Do I Convert A Float Number To A Whole Number In
Check If Variable Is A Number In Javascript Mkyong Com
10 New Javascript Features In Es2020 That You Should Know
How To Convert A String To A Number In Javascript
How To Convert A String To A Number In Javascript
Animating Number Counters Css Tricks
Javascript Converts The Strings In The Array Into Integer
Javascript Math Check If A Number Is A Whole Number Or Has A
Javascript Divisor And Modulus How To Calculate Function On
How To Get Numbers From A String In Javascript
Javascript Essentials Numbers And Math By Codedraken
R Data Types Become An Expert In Its Implementation
Write A Javascript Function That Takes An Integer N Chegg Com
How To Convert A String To An Integer In Javascript Stack
Leetcode 1290 Convert Binary Number In A Linked List To
Github Bosnaufal Readable Number Simple Javascript
Javascript Numbers Get Skilled In The Implementation Of Its
How To Convert A String To An Integer In Javascript Stack
Javascript How Can I Split A Decimal Number Into 2 Part
0 Response to "33 Integer Part Of A Number Javascript"
Post a Comment