34 Javascript Sum Array Object Values
First iterate through the array and push the 'name' into another object's property. If the property exists add the 'value' to the value of the property otherwise initialize the property to the 'value'. Once you build this object, iterate through the properties and push them to another array. Here is some code: totalValue is the sum accumulator as before. currentValue is the current element of the array. Now, this element is an object. So the only thing we have to do different in this example is to add the totalValue with the x property of the current value.
Javascript Array Distinct Ever Wanted To Get Distinct
You can use the reduce () method to find the sum of an array of numbers. The reduce () method executes the specified reducer function on each member of the array resulting in a single output value as in the following example:
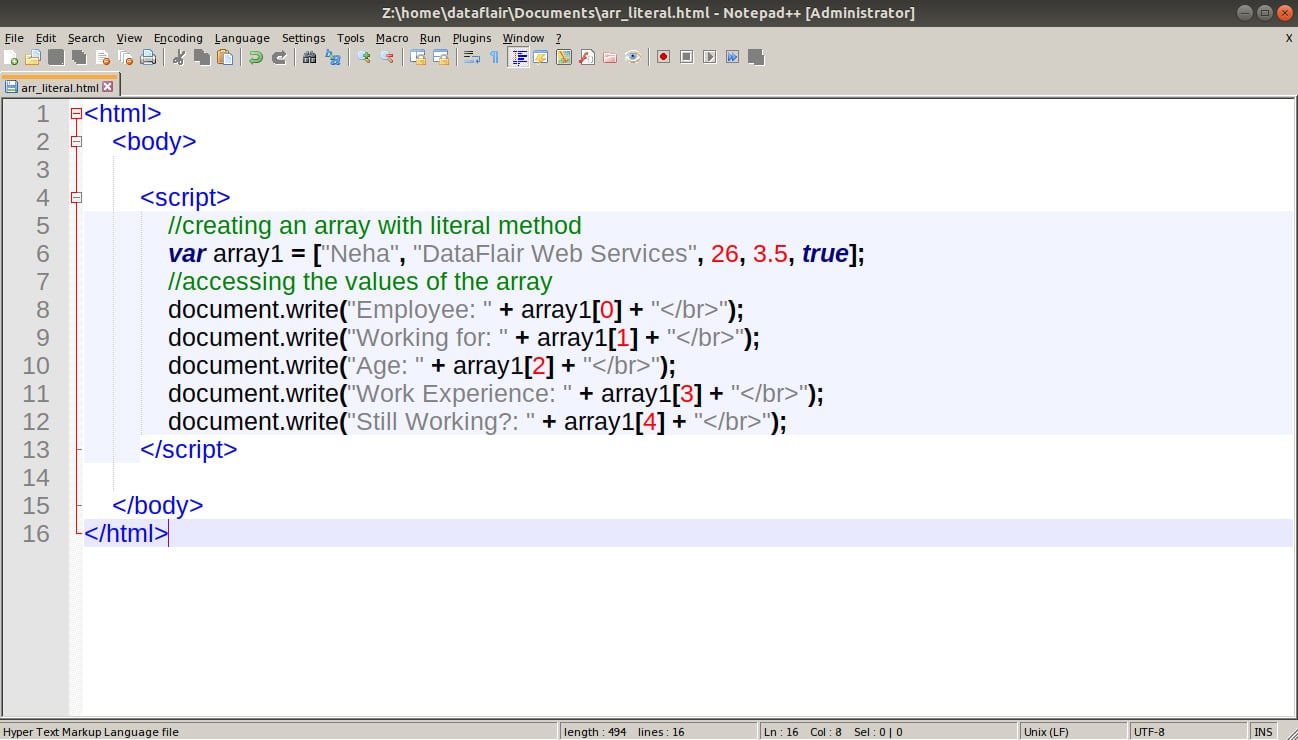
Javascript sum array object values. Morioh is the place to create a Great Personal Brand, connect with Developers around the World and Grow your Career! This post will discuss how to find the sum of all the values of an array in JavaScript. 1. Using Array.prototype.reduce() function. The reduce() method can be used to execute a reducer function on each array element. To calculate the sum of all the values of an array, the reducer function should add the current element value to the already computed sum of the previous values. JavaScript code recipe: sum an array of objects. Learn how to sum a property in an array of objects using JavaScript reduce with example code. Posted on January 18, 2021. Sometimes, you need to count the sum of property in an array of objects. For example, you may find an array of items, and you want to sum the total quantity a person must pay ...
arr = [{x:1}, {x:3}] arr.reduce((accumulator, current) => accumulator + current.x, 0); To sum up the values contained in an array of objects, you must supply an initialValue, so that each item passes through your function. let initialValue = 0 let sum = [ { x : 1 } , { x : 2 } , { x : 3 } ] . reduce ( function ( accumulator , currentValue ) { return accumulator + currentValue . x } , initialValue ) console . log ( sum ) // logs 6 Array.prototype.reduce() function can be used to iterate through an array, adding the current element value to the sum of the previous item values. Javascript sum array. To find the Javascript sum array of two numbers, use array.reduce() method. The reduce() method reduces an array to a single value.
21/11/2020 · Sum of array object property values in new array of objects in JavaScript Javascript Web Development Front End Technology Object Oriented Programming Suppose we have an array of objects that contains the data about some students and their marks like this − Jun 13, 2021 - In this article, we will learn better and efficient ways to sum the values of an array of objects in JavaScript. In our examples, we will sum the Amount for all objects in the following array. Definition and Usage. The reduce() method executes a reducer function for each value of an array.. reduce() returns a single value which is the function's accumulated result. reduce() does not execute the function for empty array elements. reduce() does not change the original array.
Here will take e.g. sum of array values using for loop, sum of array object values using javaScript reduce () method, javascript reduce array of objects by key, javascript reduce array values using javascript map () method. If you should also read this javascript array posts: Convert Array to Comma Separated String javaScript Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: Mar 25, 2021 - Use the reduce() Method to Sum an Array in a JavaScript Array · The reduce() method loops over the array and calls the reducer function to store the value of array computation by the function in an accumulator. An accumulator is a variable remembered throughout all iterations to store the ...
Metro Network Map, The Kiboomers The Circle Song, Gujarat Government Holiday List 2020, Pandas Series Replace Multiple Values, Stores In Pella, Iowa, Ariel Drawing With Colour, Richland County Wi, Antioch University New England Reviews, Sebastian Apocalypse Outfit, Soul Calibur 6 - Critical ... Sum from array values with similar key in JavaScript Javascript Web Development Object Oriented Programming Let's say, here is an array that contains some data about the stocks sold and purchased by some company over a period of time. Transforming objects. Objects lack many methods that exist for arrays, e.g. map, filter and others. If we'd like to apply them, then we can use Object.entries followed by Object.fromEntries:. Use Object.entries(obj) to get an array of key/value pairs from obj.; Use array methods on that array, e.g. map, to transform these key/value pairs. Use Object.fromEntries(array) on the resulting array ...
Sum of nested object values in Array using JavaScript; Sum from array values with similar key in JavaScript; Sum of all prime numbers in an array - JavaScript; Sum of all positives present in an array in JavaScript; Sum all perfect cube values upto n using JavaScript; Previous Page Print Page. Next Page . Advertisements JavaScript exercises, practice and solution: Write a JavaScript function to calculate the sum of values in an array. Array.from() Creates a new Array instance from an array-like or iterable object.. Array.isArray() Returns true if the argument is an array, or false otherwise.. Array.of() Creates a new Array instance with a variable number of arguments, regardless of number or type of the arguments.
javascript sum array of objects; javascript array unique values; duplicate an array in javascript n times; remove null from array javascript; javascript filter null values from array; js map value in range; array contains case insensitive javascript; merge sort javascript; javascript group by property array of objects; javascript group by on ... There are different methods to sum the array this is very basic questions which comes up when starting with JavaScript. Below are the methods which we can use to sum the numbers in the array. The methods include JavaScript sum array reduce method, JavaScript sum array foreach, JavaScript sum array of objects, JavaScript sum array for loop. Sep 18, 2020 - We are required to write a JavaScript function that takes in one such array and combines the value property of all those objects that have similar value for the firstName property.
Oct 09, 2020 - arr = [{x:1}, {x:3}] arr.reduce((accumulator, current) => accumulator + current.x, 0); Feb 11, 2021 - Hi everyone! I've been battling with this thing the whole day. Let's say that I have for example a .json which looks like this: I'm trying to add … Result object, empty. Loop through an array of objects using Array.prototype.forEach() method. Iterate over each object's (basket's) key-value pairs using Object.entries() method. Check if the result object has a property with the name of the key.
Creating a SUM of nested object values in JavaScript. Ask Question Asked 4 years, 3 months ago. Active 7 months ago. Viewed 4k times 1 0. I'm using the following code to query an API, which is working well to return nested values in JSON: ... You can do it using Array#map() to create a new array and Array#reduce() to sum the amountString. You can write a function for this task, which gets the array to iterate over an the property, which value should be added. The key feature of the function is the Array#reducemethode and a property which returns the actual count calue and the actual property value. function count(array, key) { return array.reduce(function (r, a) { If we need to sum a simple array, we can use the reduce method, that executes a reducer function (that you provide) on each member of the array resulting in a single output value. For example: let sum = [1, 2, 3].reduce ( (a, b) => a + b, 0); console.log (sum); // expected output: 6. But if we have an objects array like the following:
It could also be used to sum values in an object array as well as flattening an array. Sum all value of an array. Here is an example that calculates the sum of all elements in an array by using the reduce() method: const array = [1, 9, 7, 4, 3]; const sum = array. reduce ((accumulator, current) => {return accumulator + current;}); console. log ... 1 week ago - The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop. (The only difference is that a for...in loop enumerates properties in the prototype chain as well.) const sumValues = (obj) => Object.keys (obj).reduce ((acc, value) => acc + obj [value], 0); We're making it an immutable function while we're at it. What reduce is doing here is simply this: Start with a value of 0 for the accumulator, and add the value of the current looped item to it. Yay for functional programming and ES2015!
Oct 09, 2020 - arr = [{x:1}, {x:3}] arr.reduce((accumulator, current) => accumulator + current.x, 0); Feb 20, 2021 - To sum up the values contained in an array of objects, you must supply an initialValue, so that each item passes through your function. For each time, it takes the current value of the item in the array and sum with the accumulator. So to properly sum it you need to set the initial value of your accumulator as the second argument of the reduce method. ... Find object by id in an array of JavaScript objects. 6101. How to return the response from an asynchronous call.
Get code examples like"javascript object array sum of values in object". Write more code and save time using our ready-made code examples. 8/4/2020 · Object.values() returns an array of the objects property values. We can use the .reduce() array method on that array to sum up all the values into a single value. The .reduce() method takes a function as a parameter which accepts four arguments but only the first two are important for our purposes: the accumulator and the current value. Home » JavaScript » Sum array of objects values by multiple keys in array. Search for: Search for: JavaScript September 17, 2020. Sum array of objects values by multiple keys in array. Suppose I have:
How To Sum An Array Of Numbers In Power Automate
Associative Array In Javascript Examples Of Associative Array
Data Structures In Javascript Arrays Hashmaps And Lists
Javascript Sum All Numbers In A Range And Diff Two Arrays
Javascript Array Reduce How To Create Reducer Function
Sum And Product Of Array Elements Using Javascript
How To Access Object Array Values In Javascript Stack Overflow
Sum All The Values In An Object By Filtering Country
Javascript Group By Sum Array Reduce Code Example
Javascript Program To Find The Sum Of All Odd Numbers Below
Sum And Product Of Array Elements Using Javascript
Javascript Sum Array Values Code Example
Java Exercises Sum Values Of An Array W3resource
How To Sum Up Currency Values Inside An Array Of Objects In
Javascript Array Reduce Amp Reduceright Reducing An Array Into
Javascript Array A Complete Guide For Beginners Dataflair
C Program To Calculate Sum And Average Of N Numbers Without Using Arrays Using For Loop
How To Group An Array Of Objects Through A Key Using Array
Javascript Sum Array Object Values Examples Tuts Make
Data Structures Objects And Arrays Eloquent Javascript
Better Array Check With Array Isarray By Samantha Ming
Javascript Map How To Use The Js Map Function Array Method
How To Write A Program To Print The Sum Of N Numbers Using
Pivot A Javascript Array Convert A Column To A Row Techbrij
Sum Of Array Elements Using Recursion Geeksforgeeks
Javascript Array Reduce How To Create Reducer Function
How To Find The Sum Of An Array Of Numbers Javascript
Add Two Numbers Represented By Two Arrays Geeksforgeeks
How To Sum An Array Of Arrays In Javascript Stack Overflow
Javascript Array Compute The Sum And Product Of An Array Of
Javascript Array A Complete Guide For Beginners Dataflair
Javascript Basic Compute The Sum Of Two Parts And Store Into
0 Response to "34 Javascript Sum Array Object Values"
Post a Comment