33 How To Click A Button In Selenium Webdriver Using Javascript
Unable to click button with selenium webdriver using C#. 0. Unable to click on link - no element found exception using Java selenium webdriver. 0. Not able to click on menu using Selenium WebDriver Java. 0. In the screen how to navigate to the row which contains the text that needs to be Edited and then click on Edit button.Can some one pls ... 31/8/2020 · Here in Javascript the syntax is quite different than the Selenium webdriver, but the method name is the same as selenium webdriver i.e: click To perform click operation on buttons/radio buttons/checkboxes/links using javascript, we have two ways, FindElement (Javascript) + Click (Javascript) FindElement (WebDriver) + Click (Javascript)
Pro Tips For Selenium Learn How To Execute Javascript Use
Screenshots in Selenium should be the way forward to make the most out of Selenium automation testing. In this blog, we deep dive into how to take screenshots in Selenium WebDriver using JavaScript. Source. Selenium WebDriver has built-in functionality to capture screenshots of the page, and it's quite easy to use.
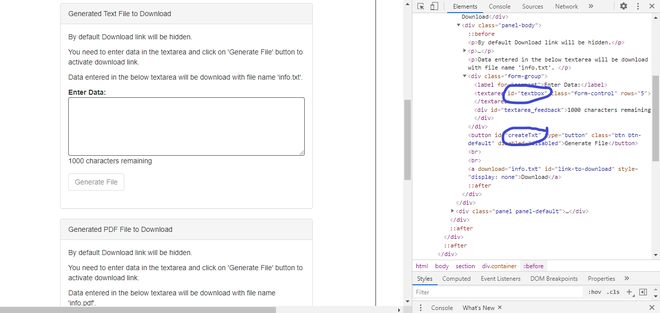
How to click a button in selenium webdriver using javascript. You should try to add some waiting time before clicking button as follow: from selenium.webdriver mon.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC button = WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.ID, "btnNew"))); button.click(); In Selenium Webdriver, we can just use element.click () method to click on any element. But sometimes, when there are any issues performing click on any element, we can use JavaScriptExecutor. Below is the example to perform click using JavaScriptExecutor. The code sets aside the instance of selenium-webdriver, and then builds the browser using WebDriver and the Firefox plugin. In the browser, the code opens Google and fetches its title using promise. This title is then sent as output to the console before quitting the browser. Best Practices for using JavaScript with Selenium WebDriver
26/11/2020 · Selenium can automatically click on buttons that appear on a webpage. This article revolves around how to click any button using Selenium in a webpage. In order to do this there are two major steps we have to take : Find the button. Click on the button. We can find the button on the web page by using methods like find_element_by_class_name (), ... Check Box. Toggling a check box on/off is also done using the click() method.. The code below will click on Facebook's "Keep me logged in" check box twice and then output the result as TRUE when it is toggled on, and FALSE if it is toggled off. We can either use the click method on the web element like a normal button as we have done above or use the submit method on any web element in the form or on the submit button itself. When submit() is used, WebDriver will look up the DOM to know which form the element belongs to, and then trigger its submit function.
How to Right Click in Selenium using Action Class? Before jumping to Right click action in Selenium Automation, let's first understand What is Right Click in general. What is Right click? As the name suggests, when a user tries to click the Right mouse button on a website or a web element to look at its context menu. E.g. Code Implementation with Javascript for clicking a button. from selenium import webdriver #browser exposes an executable file #Through Selenium test we will invoke the executable file which will then #invoke #actual browser driver = webdriver.Chrome(executable_path="C:\\chromedriver.exe") # to maximize the browser window driver.maximize_window ... Click on a button in selenium webdriver using JavaScript: Syntax: ... Example of selenium webdriver with javaScript to click on hidden element on page and get title of that page. steps: 1. Access the url "https://webnersolutions ". 2. Open the sub heading using the javascript 3. Get the title of sub heading pages.
Advanced Operations Using Selenium.Click() With basic operations covered, we now step into performing advanced operations using the Selenium click button method. Using Selenium Click Button with X,Y coordinate . We can use movebyOffset to perform a click anywhere on the page by feeding (x,y) coordinates to the WebDriver. You can even generate a ... Executing a click via JavaScript has some behaviors of which you should be aware. If, for example, the code bound to the onclick event of your element invokes window.alert (), you may find your Selenium code hanging, depending on the implementation of the browser driver. That said, you can use the JavascriptExecutor class to do this. If these locators do not work as expected, or you are handling a tricky XPath, you can use JavaScriptExecutor to perform the desired operation on the WebElement.. On similar lines, to click on a WebElement, we generally use the click () method provided by Selenium Webdriver. driver.findElement (By.id ("button")).click (); 1
How to perform Mouse Click action in Selenium WebDriver. Mouse click (single click) is one of the basic mouse actions in Selenium WebDriver that you would be required to perform during the Selenium test automation process. Mouse clicks are primarily used to perform click actions on buttons, checkboxes, radio buttons, etc. How to click Onclick Javascript form using Selenium? Ask Question Asked 7 years, 3 months ago. ... Get HTML source of WebElement in Selenium WebDriver using Python. 0. How to click on required button with same class using selenium. 1. How to use the Selenium Click command. To put it in simple words, the click command emulates a click operation for a link, button, checkbox or radio button. In Selenium Webdriver, execute click after finding an element. In SeleniumIDE, the recorder will do the identifying, and the command is simply click.
How to click a href link using Selenium? We can click a href link with Selenium webdriver. There are multiple ways to achieve this. First of all we need to identify the link with the help of the locators like link text and partial link text. The link text locator identifies the element whose text matches with text enclosed within the anchor tag. However, you can execute JavaScript from Java using WebDriver, and you could call some JavaScript code that clicks a particular button. WebDriver driver; // Assigned elsewhere JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript("window.document.getElementById('gbqfb').click()"); How to handle Alert in Selenium WebDriver. Alert interface provides the below few methods which are widely used in Selenium Webdriver. 1) void dismiss() // To click on the 'Cancel' button of the alert. driver.switchTo().alert().dismiss(); 2) void accept() // To click on the 'OK' button of the alert.
26/8/2021 · 1. Introduction. In this short tutorial, we're going to take a look at a simple example of how to click and element in Selenium WebDriver using JavaScript. For our demo, we'll use JUnit and Selenium to open https://baeldung and search for “Selenium” articles. 2. 4 days ago - JavaScriptExecutor is an Interface that helps to execute JavaScript through Selenium Webdriver. JavaScriptExecutor provides two methods ""executescript"" & ""executeAsyncScript"" to run javascript on the selected window or current page. Typing Enter/Return key in Selenium. We can type Enter/Return key in Selenium. We shall use the sendKeys method and pass Keys.ENTER as an argument to the method. Also, we can use pass Keys.RETURN as an argument to the sendKeys method for the same purpose. To use the Keys class, we have to incorporate import org.openqa.selenium.Keys to the code ...
Selenium can automatically click on buttons that appear on a webpage. In this example we will open a site and click on a radio button and submit button. Related course Browser Automation with Python Selenium. Selenium button click Start by importing the selenium module and creating a web driver object. We then use the method: Mar 03, 2016 - Originally reported on Google Code with ID 2556 Below is the html tag, WebDriver click doesn't work. This website is not a public url so I am putting the html tag for your reference. Step 3: Install the Selenium webdriver package. This can be done by opening terminal/command prompt in the same directory where the node is installed and running the below command. npm install selenium-webdriver. Step 4: Install drivers for browser that will be used for automating the browser. The browser used in this article is Chrome, hence ...
Do you know we can also Click in Selenium webdriver using JavaScript using different approaches. I have covered previously as well like How to perform click operation in Selenium Webdriver but now we are going to talk about something new I mean how to click in Selenium webdriver using JavaScript for an element which is disabled. We have already discussed the technique to handle checkbox and radio button in Selenium WebDriver.The technique which we have discussed earlier is simple and default one. As we have also discussed that sometimes click button command does not work in Selenium, hence, in such case, JavascriptExecutor technique to click on web element came as the savior. We can use the JavaScript Executor in Selenium to click an element. Selenium can execute JavaScript commands with the help of the method executeScript. Sometimes while clicking a link, we get the IllegalStateException, to avoid this exception, the JavaScript executor is used instead of the method click.
How We Use JavaScriptExecutor in Selenium. Scenario #1: To Type Text in a Text Box. Scenario #2: To Click on a Button. Scenario #3: To Handle Checkbox. Scenario #4: To generate Alert Pop window in selenium. Scenario #5: To refresh browser window using Javascript. Let us discuss some differences between them. The submit () function is applicable only for <form> and makes handling of form easier. It can be used with any element inside a form. The click () is only applicable to buttons with type submit in a form. The submit () function shall wait for the page to load however the click () waits only if any ...
How To Perform Click In Selenium Webdriver Using Javascript
Use Of Switch To Commands For Handling Javascript Pop Ups And
Selenium Webdriver Navigation Commands Javatpoint
How To Right Click And Select Option In Webdriverjs
How To Handle Alerts Popups In Selenium Webdriver Selenium
Selenium Javascript Automation Testing Tutorial For Beginners
How To Click A Web Element Which Is Invisible On Screen
How To Select Checkbox And Radio Button In Selenium Webdriver
How To Handle Checkbox In Selenium Webdriver Koi
Setting Up A Testops Environment Using Selenium Webdriver And
Browser Automation With Javascript Selenium Webdriver Dev
Double Click And Right Click In Selenium With Examples
Tools Qa How To Perform Right Click And Double Click In
Work With Select Element Tips And Tricks Documentation
Download File In Selenium Using Python Geeksforgeeks
Javascriptexecutor In Selenium Complete Guide 2021 Update
How To Perform Double Click In Selenium Browserstack
Double Click And Right Click In Selenium With Examples
Selenium Webdriver Click A Javascript Button Stack Overflow
Selenium Webdriver Running Test On Chrome Browser Javatpoint
Can T Click A Button With Javascript Using Execute Script
Javascriptexecutor In Selenium Webdriver With Example
How To Handle Scroll Bar In Selenium Webdriver
Javascriptexecutor In Selenium Webdriver Scientech Easy
9t Webdriver Handling Radio Buttons And Checkboxes Java
Webdriverio Tutorial Handling Alerts And Overlay In Selenium
5 Filling In Web Forms Web Scraping Using Selenium Python
How To Locate Elements Using Selenium Python With Examples
Handling Radio Buttons And Checkboxes Using Selenium
Python Selenium Click On Button Stack Overflow
How To Select And Click The First Enabled Button Software
Selenium Webdriver First Test Case Javatpoint
0 Response to "33 How To Click A Button In Selenium Webdriver Using Javascript"
Post a Comment