25 Convert String To Json Array In Javascript
Convert string array to array in javascript . Use jQuery to convert JSON array to HTML bulleted list . How to generate a JSON object dynamically with duplicate keys? Serializing an interface/abstract object using NewtonSoft.JSON . Accessing properties with a dot in their name . JSON.stringify() returns the string JSON format of the Javascript object. Usage. Let us use the same object that we used in the previous section. But this time, we will use the JSON.stringify() to convert it into a JSON string. Refer to the following code.
Filtering Json Array In Javascript Code Example
json is nothing but javascript object notation. You just need to parse it as suggested by Sudhir. You can also use jQuery.parseJSON for it. And to do ajax, I strongly suggest you to use some library, preferably jQuery.
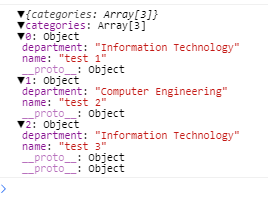
Convert string to json array in javascript. Use the JavaScript function JSON.parse() to convert text into a JavaScript ... on a JSON derived from an array, the method will return a JavaScript array, instead of a JavaScript ... Date objects are not allowed in JSON. If you need to include a date, write it as a string. You can convert it back into a date object later: Example. ... Use the JSON.parse () Expression to Convert a String Into an Array The JSON.parse () expression is used to parse the data received from the web server into the objects and arrays. If the received data is in the form of a JSON object, it will convert it into a JavaScript object. Convert Array of Objects to String. To convert an array of objects into a string in JavaScript, use the JSON.stringify() method. The JSON.stringify() is a built-in method that converts a JavaScript object or value to a JSON string.
10/10/2020 · Convert String to JSON Using eval () The eval () function in JavaScript is used to take an expression and return the string. As a result, it can be used to convert the string into JSON. The string or an expression can be the value of eval (), and even if you pass multiple statements as an expression, the result will still work. Append extra an [and ]to the beginning and end of the string. This will make it an array. Then use eval()or some safe JSON serializer to serialize the string and make it a real JavaScript datatype. You should use https://github /douglascrockford/JSON-jsinstead of eval(). eval is only if you're doing some quick debugging/testing. 18/2/2010 · Just double-checking: is the array you want to send to the page a JavaScript array or is it on the server? – Ian Oxley Feb 19 '10 at 10:22 it's a Javascript array, I will be sending it to a Python script and Python will use the JSON string and work with that. – dotty Feb 19 '10 at 10:24
If you have a well-formed JSON string, you should be able to do var as = JSON.parse (jstring); I do this all the time when transfering arrays through AJAX. Convert an Array to JSON Using the JSON.stringify () Function in JavaScript We use JSON to send and receive data from a server, and the data should be in a string format. We can convert a JavaScript array to JSON using the JSON.stringify () function. Javascript Object Notation. In layman's terms, we use the JSON.stringfy (ARRAY) function to turn an array or object into a JSON encoded string - Then store or transfer it somewhere. We can then retrieve this JSON encoded string, do the opposite of JSON.parse (STRING) to get the "original" array or object back. USEFUL BITS & LINKS
The String.split () method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as an argument. You can also optionally pass in an integer as a second parameter to specify the number of splits. Now that we have our JSON array ready, we can move forward to the next and final step, converting it into a String array. Conversion into a String array. The approach that we are using here, will first insert all the JSON array elements into a List since it is then easier to convert List into an array. 1. Creating a List. Let's start by ... Convert Array to JSON Object JavaScript You can use JSON.stringify to convert an array into a JSON formatted string in JavaScript. Suppose there is an array such as " [1, 2, 3, 4]". If you want to convert this array to JSON Object in javascript.
Convert JSON Array String to List. Many times the JSON string contains an array to store multiple data. This can be converted to an array or list of objects in C#. The following example shows how to parse JSON array to C# list collection. Definition and Usage. The toString () method returns a string with all array values separated by commas. toString () does not change the original array. forEach () method in the array is used to iterate the array of elements in the same order. This has a callback function which called for every element. Using lambda expression, Loop through each element and add the element to a temporary object. Finally, JSON.stringify () method is used to convert Object to JSON string
You can use the simple javascript JSON.stringify () method to simply convert a JavaScript object a JSON string. javascript string to json You can use the JSON.parse () method in JavaScript, to convert a JSON string into a JSON object. Convert String to JSON Using json.stringify () Converting your PHP array into a valid JSON string To convert a PHP array into a JSON string, you can make use of the built in function json_encode (). This function takes an array as an argument and outputs a valid JSON string that can then be used in Javascript. You convert the whole array to JSON as one object by calling JSON.stringify () on the array, which results in a single JSON string. To convert back to an array from JSON, you'd call JSON.parse () on the string, leaving you with the original array. To convert each item in an array into JSON format, then you'll want to loop over the array.
The JSON.stringify () method converts a JavaScript object, array, or value to a JSON string that can be sent over the wire using the Fetch API (or another communication library). Weird Answer - Array to JSON with indexes as keys Use the JavaScript function JSON.stringify () to convert it into a string. const myJSON = JSON.stringify(obj); The result will be a string following the JSON notation. myJSON is now a string, and ready to be sent to a server: Converting your PHP array into a valid JSON stringlink. To convert a PHP array into a JSON string, you can make use of the built in function json_encode (). This function takes an array as an argument and outputs a valid JSON string that can then be used in Javascript. Converting an.
Learn how to convert an array to string in javascript. An array in javascript can hold many any types of data that is available in the language. We will see different ways to form a string from different types of array. Using toString() method to create a string from array. Using toString() method on an array returns the string representation ... JSON is a common datatype to exchange the data from the client and server. When we want to send data from the client to a web server, the data must be a string. Javascript JSON to String. To convert a JavaScript object to string, use the JSON.stringify() function. The JSON.stringify() method converts JavaScript object into string. 26/4/2020 · This array contains the values of JavaScript object obtained from the JSON string with the help of JavaScript. There are two approaches to solve this problem which are discussed below: Approach 1: First convert the JSON string to the JavaScript object using JSON.Parse() method and then take out the values of the object and push them into the array using push() method .
In typescript/javascript, the response returned from REST API or back servers are in the form String JSON text, Developers need to know the ways to convert to JSON or class object. Typescript is a superset of javascript with type assertions feature. In javascript, we have used JSON.parse () method to convert to JSON, you can check here. The stringify() is used to convert JavaScript code into a string. We can use the stringify() function to convert object, date, and even array into a string. In this tutorial, we will discuss how to use the stringify() function to convert a JavaScript array into JSON. JSON.stringify() Observe the following JavaScript code. Convert String to Array JavaScript |split, Separator, Regex, JSON.parse Posted June 11, 2020 May 15, 2021 by Rohit How can I convert a string to a JavaScript array?
Modifying Json Data Using Json Modify In Sql Server
Convert String To Json Objects In Javascript With Eval
How Can I Convert String To Json Object In Java Edureka
4 Ways To Convert String To Character Array In Javascript
How To Convert Mongodb Response Array From Javascript Object
How To Convert The Php Array To Json String
In Java How To Convert Arraylist To Jsonobject Crunchify
Cannot Deserialize The Current Json Object E G Name
Convert String To Json Object Using Javascript
Java67 How To Convert Json String To Java Object Gson Json
Javascript Array Serialized In Json Enclosed In Quotes Like
Python How To Convert A List To A Json Array Examples
Getting The Json Array From Servlet To Javascript Variable
Converting Json Object Into Javascript Php Array Pakainfo
How To Convert Json Array To Arraylist In Java Javatpoint
How To Convert Json String To Json Array
Add Json Object To Json Array Javascript Code Example
Java67 3 Ways To Ignore Null Fields While Converting Java
Convert Json String To Data Array Using Javascript Or C
Javascript Convert An Array To Json Geeksforgeeks
C Convert Json String To List Object Index Number Of Json
Convert Array To Json Object Javascript Tuts Make
Convert Json String To Array Javascript Code Example
6 Ways To Convert String To Array In Javascript
0 Response to "25 Convert String To Json Array In Javascript"
Post a Comment