21 Event Driven Computation In Javascript
Nov 01, 2018 - A brief look at the various modules and methods for implementing an effective Event-Driven Programming architecture in a Node.js project. · Event can be something the browser does, or something a user does. · JavaScript's interaction with HTML is handled through events that occur when the user or the browser manipulates a page. · When the page loads, it is called an event. · When the user clicks a button, that click too is an event.
Client Side Programming With Javascript 2 Event Driven
The term "event-driven architecture" covers a wide range of distributed systems like MicroServices, Serverless etc. And this chapter from the book Software Architecture Patterns says event-driven architectures have many useful features, but they are hard to build and test. One reason for that is because designing a system that is robust, i.e. works in all situations, requires significant ...
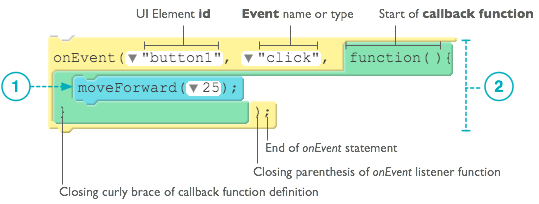
Event driven computation in javascript. Whenever an HTML element is clicked, the most simple case, an event is dispatched. Developers can intercept these events (JavaScript engines are event-driven) with an event listener. Event listeners in the DOM have access to this because the function runs in the context object who fired up the event (an HTML element most of the times). Consider ... Apr 09, 2018 - A discussion and examples of how to apply event driven architecture node.js. This is an initial article to be followed by a complete series of articles. Javascript sacrificed convenience for simplicity 83. ... and it was the right choice 84. • Event driven programming • Event driven javascript • History of javascript design 85. How can we tame complexity? 86. Add Wait() stupid! 87.
Fourth, the user clicks the equals key. Right after they click equals, but before the calculation, we set secondValue as displayedNum. Fifth, the calculator calculates the result of 5 - 1 and gives 4. The result gets updated to the display. firstValue and operator get carried forward to the next calculation since we did not update them. On the Text Field Properties dialog, select the Calculate tab. Select the desired calculation option: Predefined, Simplified field notation, or Custom calculation script. Enter the actual calculation data. This data will, of course, be different for each of the different types of calculations. Event-driven computing is hardly a new idea; people in the database world have used database triggers for years. The concept is simple: whenever you add, change, or delete data, an event is triggered to perform a variety of functions.
Event driven programming follows mainly a publish-subscribe pattern, ie a class (as an example) communicates with another class with events, not by calling methods directly. The main advantages are: Loose coupling, i.e. you can remove or add features without breaking your core. Sep 28, 2015 - Event driven architecture in JavaScript can easily become a pain. Introduction to a module that helps you manage triggers and subscriptions better. Understanding event-driven control flow • Often interested in sequences of events 4 mousedown start mouseup stop
Sep 06, 2020 - Update: This article is now part of my book “Node.js Beyond The Basics”.Read the updated version of this content and more about Node at jscomplete /node-beyond-basics.Most of Node’s objects — like HTTP requests, responses, and streams — implement the EventEmitter module so they ... Event-driven programming with JavaScript is a useful way to create interactive websites. Typically, after the webpage has loaded the JavaScript program continues to run waiting for an event. If you connect this event to a JavaScript function then the function will run when the event occurs. JavaScript lets you execute code when events are detected. HTML allows event handler attributes, with JavaScript code, to be added to HTML elements. With single quotes: <element event='some JavaScript'>. With double quotes: <element event="some JavaScript">. In the following example, an onclick attribute (with code), is added to a <button> element:
Jun 30, 2015 - When you start building dashboards for interacting with data, such as calculators, editors, or result browsers, understanding JavaScript and client-side MVC becomes important. Why do you need an event-driven application design and a separation of interface state and behavior? Creates date based on specified date and time. To demonstrate the different ways to refer to a specific date, we'll create new Date objects that will represent July 4th, 1776 at 12:30pm GMT in three different ways. usa.js. new Date(-6106015800000); new Date("July 4 1776 12:30"); new Date(1776, 6, 4, 12, 30, 0, 0); Copy. Mar 25, 2019 - Fundamental Operators To understand at the fundamental level how the event driven mechanism in node.js works, lets start with the fundamental design of the computer and understand how the computer works.
I am trying to perform some computations on a server. For this, the client initially inputs some data which I am capturing through Javascript. Now, I would perhaps make a XMLHttpRequest to a server to send this data. Let's say the computation takes an hour and the client leaves the system or switches off the system. Javascript has events to provide a dynamic interface to a webpage. These events are hooked to elements in the Document Object Model (DOM). These events by default use bubbling propagation i.e, upwards in the DOM from children to parent. We can bind events either as inline or in an external script. Sep 25, 2019 - If it is applied to the right place and for the right scenario, the event-driven programming paradigm can be a huge win for application architecture.
Event-driven programming is the dominant paradigm used in graphical user interfaces and other applications (e.g. Javascript web applications) that are centered around performing certain actions in response to user input. Event Driven Programming is a computer programming paradigm. computation. In Chapter 4 we propose automatic parallelisation of event-driven programs with resolution of con icts via transactional memory as a form of concurrent programming. Parallelisation maintains simple semantics of event-driven programs yet can take ad-vantage of modern multiprocessors. To our knowledge, this is the rst attempt to Event-driven programming is the dominant paradigm used in graphical user interfaces and other applications (e.g., JavaScript web applications) that are centered on performing certain actions in response to user input. This is also true of programming for device drivers (e.g., P in USB device driver stacks).
When making event-driven apps in App Lab you usually go through the following steps: Step 1 - Design Mode. Add a UI element (or a few) in Design Mode. Step 2 - Add onEvent to Code. Set the id and event type to listen for some event on that element. Step 3 - Write the code for the event handling function. Event-driven programming has been around for a while, but it's now becoming more and more prevalent. The traditional model of an application splits the logic into two parts: the event loop and the message queue. We'll use JavaScript as an example to explore how this technique is employed in modern… //In the javascript code var theForm = document.forms["cakeform"]; How to use radio buttons in a calculation. In order to use radio buttons in a calculation, we need to give the radio buttons a name. All the radio buttons that belong to the same group should have the same name. This way, if you had more than one group of radio buttons, the code ...
When making event-driven apps in App Lab you usually go through the following steps: Step 1 - Design Mode. Add a UI element (or a few) in Design Mode. Step 2 - Add onEvent to Code. Set the id and event type to listen for some event on that element. Step 3 - Write the code for the event handling ... Associate the web resource containing your JavaScript code to model-driven apps forms to be able to associate functions in your code with events. As the JavaScript code in this walk through is targeted at the account record, we will associate the web resource with the account form. JavaScript is an integral part of practically every webpage, mobile app and web-based software. While JavaScript's client side scripting capabilities can make applications more dynamic and engaging, it also introduces the possibility of inefficiencies by relying on the user's own browser and device. Consequently, poorly written JavaScript can make it difficult to ensure a consistent experience ...
JavaScript in the browser uses an event-driven programming model. Everything starts by following an event. The event could be the DOM is loaded, or an asynchronous request that finishes fetching, or a user clicking an element or scrolling the page, or the user types on the keyboard. There are a lot of different kind of events. Now create the lesson12_tryout2.js file. This file will contain 3 functions: init() initializes the script by binding the displaySquare() function to its appropriate event, that is, the btnSubmit onclick event; displaySquare() checks the answer submitted by the user, calls the function that performs the calculation, and displays the result; calculateSquare(input) gets passed the number value ... JavaScript applications is event-based programming. In this programming model, a callback function ... specific to the event-driven programming model, such aslost events (situations where an event is ... A promise represents the value of an asynchronous computation, and is in one of three states ...
Event-driven computation underlies many applications, ranging from graphical user interfaces to systems for discrete event simulation and business process modeling. An important characteristic of event-driven computation is that control is relinquished to an environment that waits for events ... For events you need event loop, which detects that the actual event (say, data from network) happens, and then generates the the software event structure and calls appropriate event handler, or in more complex systems, chain of event handlers, until a handler marks the event accepted. Nov 14, 2017 - Event driven programming is the programming paradigm in the field of computer science. In this type of programming paradigm, flow of execution is determined by the events like user clicks or other…
Jan 11, 2018 - A brief look at the various modules and methods for implementing an effective Event-Driven Programming architecture in a Node.js project. The main idea of Node.js: use non-blocking, event-driven I/O to remain lightweight and efficient in the face of data-intensive real-time applications that run across distributed devices. That's ... Event-driven programming is a programming paradigm in which the flow of the program is determined by events. An event-driven program performs actions in response to events. When an event occurs it triggers a callback function. Now, let's try to understand Node and see how all these relate to it.
This changes EVERYTHING! The fact that Javascript is event-driven is the reason for closures, weird scoping/context, and the myriad of callbacks you see eve... Hello friends,In this video lesson, we will see how we can perform arithmetic calculations in JavaScript without button. This is completely an event driven ... In the above snippet, we're listening for a click event on the element with a class of calculator-keys.Since all the keys on the calculator are children of this element, the click event filters down to them too. This is known as event delegation. Inside the callback function of the event listener, we extract the target property of the click event using destructuring assignment which makes it ...
JavaScript engines enhance the language by providing a rich environment, offering also an event-driven platform for JavaScript. In practice, JavaScript in the browser can interact with HTML elements, which are event emitters, that is, subjects able to emit events. Consider this trivial example, an HTML document with a button: Javascript supports event-driven programming, where events are expected and processed ("handled") by the program (with an "event handler") when notified of a happening in the environment. When used within a web page — which is constructed on a "document object model" (DOM) — Javascript can react to DOM events. Some common events ...
Event Driven Programming Techformist
Sensors Free Full Text Design And Realization Of An
When You Should And Shouldn T Use Node Js For Your Project
Pros And Cons Of Node Js Web App Development Altexsoft
Node Js Vs Java Performance Best Comparison To Learn With
Event Driven Computing With Amazon Sns And Aws Compute
Node Js Event Loop Geeksforgeeks
Pros And Cons Of Javascript Full Stack Development Altexsoft
Energies Free Full Text Event Driven Coulomb Counting For
Comprehensive Guide To Javascript Design Patterns Toptal
Event Driven Computing With Amazon Sns And Aws Compute
4 1 Overview Of Java Script Originally Developed
Introduction To Event Driven Programming
Event Driven Io Server Side Javascript Environment Based On
4 1 Overview Of Javascript Ppt Download
Node Js Event Loop How Even Quick Node Js Async Functions
Memory Devices And Applications For In Memory Computing
Experimental Demonstration Of Supervised Learning In Spiking
0 Response to "21 Event Driven Computation In Javascript"
Post a Comment