32 Create List From Array Javascript
30/7/2021 · Here we will populate the dropdown list with an array. Below is the description of popular approaches used in JavaScript. Example 1: In this example, the length property is used to traverse the elements of the array and on each element create an option element and append this new element to the select element by appendChild() method. Program: Array of states for each friend. Getting The Unique List of States. The next step is to use a JavaScript Set.. JavaScript Set. The Set object lets you store unique values of any type, whether primitive values or object references.. The key word to make note of in the description is unique.. The syntax to create a new Set is,
Javascript Array Distinct Ever Wanted To Get Distinct
JavaScript has many ways to do anything. I've written on 10 Ways to Write pipe/compose in JavaScript, and now we're doing arrays. 1. Spread Operator (Shallow copy)Ever since ES6 dropped, this has been the most popular method. It's a brief syntax and you'll find
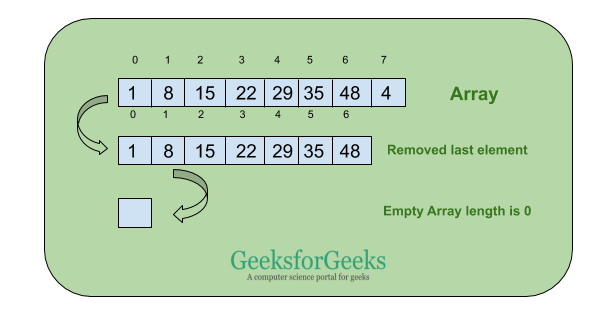
Create list from array javascript. Array.from() lets you create Arrays from: array-like objects (objects with a length property and indexed elements); or ; iterable objects (objects such as Map and Set).; Array.from() has an optional parameter mapFn, which allows you to execute a map() function on each element of the array being created. More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map ... JavaScript has a buit in array constructor new Array (). But you can safely use [] instead. These two different statements both create a new empty array named points: const points = new Array (); const points = []; These two different statements both create a new array containing 6 numbers: generate a sequence numbers between a range javascript; create array javascript numbers; es6 compare two arrays; js subtract arrays; find non common elements from 2 arrays; javascript array value dom; javascript create variable containing an object that will contain three properties that store the length of each side of the box
We will begin by looking at different ways to create an array in JavaScript. The first two of these examples create arrays where only the length is set and there are no numbered entries at all ... In this tutorial, you'll learn how to create a list or an array of years in JavaScript. You can copy this snippet and use it in your projects e.g. to create form fields. I use it quite a lot in my projects. For example, I once needed a drop-down field that would have a list of 10 most recent years (present - 10). In the above code, we have an empty array inputArray. Next we have a size variable which will be used to limit the iterations for defining a finite array size, because by nature arrays are dynamic in JavaScript. In this loop, we take the inputArray[i] and store the return value from the prompt function. As the iterations increases, the size of ...
10/3/2021 · Javascript. let list = document.getElementById ("myList"); Step 3: Now iterate all the array items using JavaScript forEach and at each iteration, create a li element and put the innerText value the same as the current item, and append the li at the list. var arr = [1, [1.1,1.2,1.3], 2, ,3]; How do i create a list using only javascript and DOM : 1 1.1 1.2 1.3 2 3 Thanks!!! To create an HTML list from an array in Javascript: Loop through the array and append list items for (let i of ARRAY) { let li = document.createElement ("li"); li.innerHTML = i; ol.appendChild (li); } Append the list to where you want document.getElementById ("TARGET").appendChild (ol); That covers the basics, read on for more examples!
Create an object from an array of key/value pairs in JavaScript. How to Create a JavaScript Object From Array of Key/Value Pairs? ... To create an object from an array of key/value pairs, you can simply use a for loop in the following way: const obj = {}; for (let i = 0; ... The JavaScript Array filter method to create a new array with desired items, a more advanced way to remove unwanted elements. Removing Elements from End of a JavaScript Array Removing Elements from Beginning of a JavaScript Array Generate an HTML ul/li list based on the contents of a JavaScript array or how to create a JavaScript list. By modifying the makeList () function to accept parameters and target elements, you can pass different arrays and generate different lists inside different containers. Solution 1
Using Array.map () to create markup from an array with vanilla JS. One thing you often need to do when building some UI elements with JavaScript is take some data from an array and create markup from it. For example, let's say I have an array of awesome wizards, like this. var wizards = ['Hermione', 'Neville', 'Gandalf']; And I want to create ... The target object is the first argument and is also used as the return value. The following example demonstrates how you can use the Object.assign () method to convert an array to an object: const names = ['Alex', 'Bob', 'Johny', 'Atta']; const obj = Object.assign({}, names); console.log( obj); Take a look at this guide to learn more about the ... 15/8/2021 · Creating a table from an array is as easy as using a loop to run through the array, and generate the HTML: var mytable = "<table><tr>"; for (var CELL of ARRAY) { mytable += "<td>" + CELL + "</td>"; } mytable += "</tr></table>"; document.getElementById("ID").innerHTML = mytable;
An array in javascript can hold many any types of data that is available in the language. We will see different ways to form a string from different types of array. Using toString() method to create a string from array. Using toString() method on an array returns the string representation of the array. Java ArrayList. The ArrayList class is a resizable array, which can be found in the java.util package.. The difference between a built-in array and an ArrayList in Java, is that the size of an array cannot be modified (if you want to add or remove elements to/from an array, you have to create a new one). While elements can be added and removed from an ArrayList whenever you want. The literal notation array makes it simple to create arrays in JavaScript. It comprises of two square brackets that wrap optional, comma-separated array elements. Number, string, boolean, null, undefined, object, function, regular expression, and other structures can be any type of array element.
In Javascript, Dynamic Array can be declared in 3 ways: Start Your Free Software Development Course. Web development, programming languages, Software testing & others. 1. By using literal. var array= ["Hi", "Hello", "How"]; 2. By using the default constructor. var array= new Array (); The array's length property is set to the number of arguments. The bracket syntax is called an "array literal" or "array initializer." It's shorter than other forms of array creation, and so is generally preferred. See Array literals for details. To create an array with non-zero length, but without any items, either of the following can be used: The Difference Between Array() and []¶ Using Array literal notation if you put a number in the square brackets it will return the number while using new Array() if you pass a number to the constructor, you will get an array of that length.. you call the Array() constructor with two or more arguments, the arguments will create the array elements. If you only invoke one argument, the argument ...
Let's try 5 array operations. Create an array styles with items "Jazz" and "Blues". Append "Rock-n-Roll" to the end. Replace the value in the middle by "Classics". Your code for finding the middle value should work for any arrays with odd length. Strip off the first value of the array and show it. Prepend Rap and Reggae to the ... 17/8/2013 · 1 Answer1. Active Oldest Votes. 1. Multidimensional Arrays is what you want. Try this. var list = []; function checkwarranty () { var x = []; x [0] = document.getElementById ('clientname').value; x [1] = document.getElementById ('select').value; x [2] = document.getElementById ('expirationdate').value; x [3] = document.getElementById ... function arrToUl(arr) { var div = document.getElementById('myList'); var ul = document.createElement('ul'); for (var i = 0; i < arr.length; i++) { if (arr[i] instanceof Array) { var list = arrToUl(arr[i]); } else { var li = document.createElement('li'); li.appendChild(document.createTextNode(arr[i])); console.log(ul.appendChild(li)); } …
A List of JavaScript Array Methods. In JavaScript, an array is a data structure that contains list of elements which store multiple values in a single variable. The strength of JavaScript arrays ... Array.from () Method. In modern JavaScript, the simplest and easiest way to convert a NodeList object to an array is by using the Array.from () method: // create a `NodeList` object const divs = document.querySelectorAll('div'); // convert `NodeList` to an array const divsArr = Array.from( divs); The output of the above code is a normal Array ... Arrays in JavaScript are high-level list-like objects with a length property and integer properties as indexes. In this article, I share a couple of hacks for creating new JavaScript arrays or cloning already existing ones. Creating Arrays: The Array Constructor
In this tutorial, we will learn how to create arrays; how they are indexed; how to add, modify, remove, or access items in an array; and how to loop through arrays. Creating an Array. There are two ways to create an array in JavaScript: The array literal, which uses square brackets. The array constructor, which uses the new keyword.
C Arraylist Tutorial With Examples
Convert List String To Array Javascript
The Beginner S Guide To Javascript Array With Examples
Javascript Take A Flat Array And Create A New Array With
How To Create An Arraylist In Java Dzone Java
How To Create A Drop Down List With Array Values Using Javascript
Jquery Add Insert Items Into Array List With Example
How To Insert An Item Into Array At Specific Index In
Javascript Arrays How To Count Poperty Values Including
How To Add An Object To An Array In Javascript Geeksforgeeks
Javascript Array Add Items In A Blank Array And Display The
Create A Comma Separated List From An Array In Javascript
Indexed Collections Javascript Mdn
5 Examples Of How To Get Python List Length Array Tuple
Hacks For Creating Javascript Arrays
Javascript Lesson 18 Arrays In Javascript Geeksread
Javascript Array A Complete Guide For Beginners Dataflair
Create Html List From Javascript Array Object Simple Examples
How To Add An Item At The Beginning Of An Array In Javascript
Hacks For Creating Javascript Arrays
How To Insert An Item Into An Array At A Specific Index
In Python What Is Difference Between Array And List Edureka
Creating A List Of Objects Inside A Javascript Object Stack
C Convert List To Array Dot Net Perls
How To Create A Dropdown List With Array Values Using
Dynamic Array In Javascript Using An Array Literal And
Creating A List Of Objects Inside A Javascript Object Stack
Remove Elements From A Javascript Array Geeksforgeeks
Javascript Array Methods How To Use Map And Reduce
How To Create An Array Containing 1 N Stack Overflow
0 Response to "32 Create List From Array Javascript"
Post a Comment