33 Javascript For Loop Over Array
As you can see above, the for loop has three statements: init is executed just once before the execution of the code block starts. This is where you define whether to loop the entire array or start midway. condition defines the condition until the loop continues executing the code block. There are multiple ways one can iterate over an array in Javascript. The most useful ones are mentioned below. Using for loop. Using while loop. This is again similar to other languages. using forEach method. The forEach method calls the provided function once for every array element in the order.
How To Loop Through An Array In JavaScript; Loop Through An Array Using For Loop. One of the methods to loop through an array is using a simple for loop. JavaScript arrays being zero indexed arrays, you can iterate over the array starting from zero until the length of the array using for loop. Here is an example to loop through the array_list ...
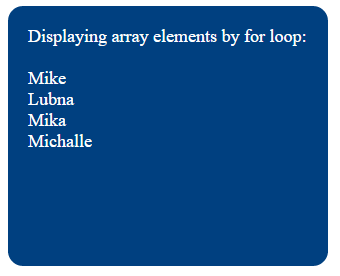
Javascript for loop over array. The JavaScript forEach method is one of the several ways to loop through arrays. Each method has different features, and it is up to you, depending on what you're doing, to decide which one to use. In this post, we are going to take a closer look at the JavaScript forEach method. Considering that we have the following array below: Dec 14, 2019 - The basic for loop is actually the fastest in the test cases. In addition to the above methods, we can also use map, filter, and reduce which are more advanced JavaScript functions. These are used for specific cases that you can learn about in the article below: A Quick Overview of map(), filter(), ... The loop will execute the code in the body first and then check the condition. It will continue that process until the bottom condition is false.. Similar to the previous methods, an i counter variable is used to measure how many loops have already occurred. And it stops the loop when each item in the array has been iterated over.
7/1/2021 · The for-of loop was added to JavaScript in ECMAScript 6: const arr = ['a', 'b', 'c']; arr.prop = 'property value'; for (const elem of arr) { console.log(elem); } // Output: // 'a' // 'b' // 'c' for-of works really well for looping over Arrays: It iterates over Array elements. We can use await. And it’s easy to migrate to for-await-of, should you need to. A for loop is a common way looping through arrays in JavaScript, but it is no considered as the fastest solutions for large arrays: for (var i=0, l=arr.length; i<l; i++) { console.log(arr[i]); } 2) While loop. A while loop is considered as the fastest way to loop through long arrays, but it is usually less used in the JavaScript code: 1 week ago - Therefore, it is better to use a traditional for loop with a numeric index when iterating over arrays, because the for...in statement iterates over user-defined properties in addition to the array elements, if you modify the Array object (such as adding custom properties or methods).
14 hours ago - There are different ways to loop over arrays in JavaScript, but it can be difficult choosing the right one. Plus keeping each method straight can drive a developer nuts. There is a classic JavaScript for loop, JavaScript forEach method and a collection of libraries with forEach and each helper ... Do not use for in over an Array if the index order is important. The index order is implementation-dependent, and array values may not be accessed in the order you expect. It is better to use a for loop, a for of loop, or Array.forEach () when the order is important. Array.forEach () The forEach () method calls a function (a callback function) once for each array element. Example. const numbers = [45, 4, 9, 16, 25]; let txt = ""; numbers.forEach(myFunction); function myFunction (value, index, array) {. txt += value + "<br>"; }
Mar 15, 2021 - JavaScript array loop tutorial shows how to loop over arrays in JavaScript. We can loop over elements with forEach method and for and while statements. JavaScript supports different kinds of loops: for - loops through a block of code a number of times. for/in - loops through the properties of an object. for/of - loops through the values of an iterable object. while - loops through a block of code while a specified condition is true. The algorithmic complexity of this approach is O (n^2) as splice function and the for loop both iterate over the array (splice function shifts all elements of array in the worst case). Instead you can just push the required elements to the new array and then just assign that array to the desired variable (which was just iterated upon).
for loop in javascript javasript javasript javasript javasript javascript javascript javascript javascript javascript javascript ... Which array function is used to loop through a array. ... Use a for loop to iterate over an array. Looping Using JSON JSON stands for JavaScript Object Notation. It's a light format for storing and transferring data from one place to another. So in looping, it is one of the most commonly used techniques for transporting data that is the array format or in attribute values. As you can see, there are quite a few ways to perform a for-each loop over an array in JavaScript, and the construct you choose largely depends on what you want to accomplish and your specific use-case. In this article we saw how to iterate over arrays using Array.forEach, built-in for loops, for-in loops, and for-of loops.
Javascript Array For Loop : Javascript Array is basically a variable which is capable of storing the multiple values inside it. Javascript array plays important role when dealing with to store multiple values. You can create array simply as - var arrayName = []. 22/7/2021 · How to loop through an array of objects in JavaScript July 22, 2021 Atta To iterate through an array of objects in JavaScript, you can use the forEach () method aong with the for...in loop. Here is an example that demonstrates how you can loop over an array containing objects and print each object's properties in JavaScript: Download Run Code. 2. Using Array.prototype.forEach() function. The forEach() method has a benefit over the traditional for-loop as there is no need to explicitly maintain the indexing information since the callback has an index parameter, which is the index of the current element at each iteration.
Each time the for loop runs, it has a different value - and this is the case with arrays. A for loop examines and iterates over every element the array contains in a fast, effective, and more controllable way. A basic example of looping through an array is: 6 Ways to Loop Through an Array in JavaScript. for/of Loop. The for/of loop statement has two expressions: Iterator - refers to the array who will be iterated; Variable - The value of the next iteration stored in a variable (which has to be declared with either const, let, or var to hold the value) Syntax Looping over an array and any other objects in JavaScript is a common problem lots of programmers encounter the most. There are several ways to loop over an array in JavaScript. Let's have a look and find the optimal one for you. Watch a video course JavaScript - The Complete Guide (Beginner + Advanced)
Jun 10, 2020 - Dealing with arrays is everyday work for every developer. In this article, we are going to see 6 different approaches to how you can iterate through in Javascript. ... Update - executed every time after the code block of the loop has been executed. Dec 17, 2015 - We can break the $.each() loop at a particular iteration by making the callback function return false. Returning non-false is the same as a continue statement in a for loop; it will skip immediately to the next iteration. ... Iterates over items in an array, accessing both the current item ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Feb 14, 2020 - A common problem many programmers encounter is looping over an enumerable data set. This data can come in the form of arrays, lists, maps, or other objects. Luckily, developers have at their disposal a variety of tools applicable to this exact problem. The JavaScript language, in particular, ... Loop Through An Array Using for loop. Javascript for loop is a traditional approach to iterate through an array. Javascript array starts from zero indexes so array first element at zeroth index and last index of an array is equal to array length minus one. Apr 28, 2021 - This post will discuss how to loop through an array backward in JavaScript... The standard approach is to loop backward using a for-loop starting from the end of the array towards the beginning of the array.
1 week ago - Note: for...in should not be used to iterate over an Array where the index order is important. Array indexes are just enumerable properties with integer names and are otherwise identical to general object properties. There is no guarantee that for...in will return the indexes in any particular order. The for...in loop ... The for...of statement creates a loop iterating over iterable objects, including: built-in String, Array, array-like objects (e.g., arguments or NodeList), TypedArray, Map, Set, and user-defined iterables. It invokes a custom iteration hook with statements to be executed for the value of each distinct property of the object. In every programming language it is very common that looping through the array, similarly in Javascript also to loop through the array, we will use forEach(). forEach() takes callback function as a parameter. A callback function that accepts up to three arguments. value, which is nothing but the current value
3 weeks ago - The forEach() method executes a provided function once for each array element. The JavaScript Loop is used to iterate through an array of items (which can be a number array, string array, etc) or objects. There are many ways to do it and so in this tutorial we will look on them one by one. Here I have taken an array of numbers and I will do the JavaScript Loop through array by using the different looping methods. The loop body will execute not only for array elements, but also for any other expando properties someone may have added. For example, if your array has an enumerable property myArray.name, then this loop will execute one extra time, with index == "name". Even properties on the array's prototype chain can be visited.
Apr 28, 2021 - This article will provide you with a solid understanding of exactly how to iterate over an Array data structure in JavaScript. Whether you are just beginning to learn JavaScript or are here for a refresher, there will be value for you either way. This article will walk you through one Output: Before clicking the button: After clicking the button: Method 3: Using the basic for loop: The default for loop can be used to iterate through the array and each element can be accessed by its respective index. Syntax: for (i = 0; i . list.length; i++) { // lines of code to execute }. Example:
For Loops For Of Loops And For In Loops In Javascript
Using Javascript For Loop Simply And In Js Arrays
How To Loop Through An Array In Javascript
3 Ways To Loop Over Object Properties With Vanilla Javascript
Loop Through An Array In Javascript Stack Overflow
For Loop Javascript Old School Loops In Javascript For
For Loop In Javascript Learn How For Loop Works In Javascript
Looping Javascript Arrays Using For Foreach Amp More
Javascript Foreach How To Loop Through An Array In Js
Using A For Each Loop On An Empty Array In Javascript Stack
How To Loop Through Array Of Objects In Javascript Es6 Reactgo
How To Iterate Through An Array In Javascript Mastering Js
How To Loop Through The Array Of Json Objects In Javascript
For Loop Javascript Old School Loops In Javascript For
Looping Over Javascript Array Of Arrays With Data For Loop
Javascript Loop Through Array Of Objects Stack Overflow
Loop Through Arrays Within Arrays Stack Overflow
How To Loop Through A Javascript Array
A Guide To Es6 Iterators In Javascript With Examples
Javascript Loop Through Object Array Code Example
How To Loop Through An Array In Javascript
How To Loop Through An Array In Javascript
Javascript For Loop How To Loop Through An Array In Js
Javascript Foreach Vs For Loop To Iterate Through Arrays
How To Loop Through An Array Of Objects In Javascript Pakainfo
Need Some Help Regarding Basic Data Structure Challenge
Bash Loop Through A List Of Strings
How To Iterate Through An Object Keys And Values In Javascript
Javascript Three Ways To Reverse An Array By Bahay Gulle
0 Response to "33 Javascript For Loop Over Array"
Post a Comment