21 Import Statement In Javascript
Node.js 12 introduced support for the import statement behind a --experimental-modules flag and a package.json configuration option. Node.js 14 removes the need for the --experimental-modules flag, but you still need to configure your package.json. Here's how you can use ES6 imports in Node. Javascript is a functional programming language where we break our code into set of functions. ... Understanding how to export and import functions in javascript. ... my import statement would be ...
import x from "module" import {default as x} from "module" Import all: import * as obj from "module" Import the module (its code runs), but do not assign any of its exports to variables: import "module" We can put import/export statements at the top or at the bottom of a script, that doesn't matter. So, technically this code is fine:
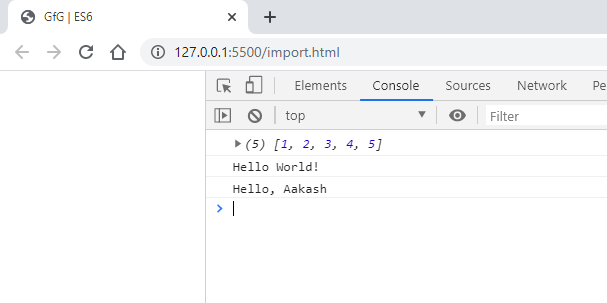
Import statement in javascript. SyntaxError: Cannot use import statement outside a module This is one of the most common issue if you are trying to use ES6 features in your JavaScript project. For example, if I use the below statement in one of my npm project : If you use import() with older browsers, remember to shim Promise using a polyfill such as es6-promise or promise-polyfill. Dynamic expressions in import() It is not possible to use a fully dynamic import statement, such as import(foo). Because foo could potentially be any path to any file in your system or project. Introducing Javascript Statements Export and Import. When writing modern web applications that's include a lot of javascript it's important to separate the code into modules. For this to work javascript provided us with two statements Export and Import. If you are familiar with languages such as C# or Java or PHP may be you see the concept ...
Java User Input. The Scanner class is used to get user input, and it is found in the java.util package.. To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation. In our example, we will use the nextLine() method, which is used to read Strings: The first thing to understand is that the purpose of the import statement is to bring something from one JavaScript file into another. This can be a function, a class, an object, or really anything... So, now they become official names for outsiders to be applied for imports. Export Default¶. In practice, there exist two types of modules.. Modules, containing a library, pack of functions ( for example, welcome.js). Modules, declaring a single entity ( for example, site.js exports only class Site). The second approach is preferred most of the time.
Export, Import, JavaScript, modules, Statements, Understanding; The author selected the COVID-19 Relief Fund to receive a donation as part of the Write for DOnations program. Introduction. In the early days of the Web, websites consisted primarily of HTML and CSS. If any JavaScript loaded into a page at all, it was usually in the form of small ... Import statements in TypeScript: which syntax to use How-To 11/2018. ... More commonly in JavaScript (CommonJS?) modules, a module author will override module.exports to a function or class instead of adding properties to the exports object like a polite module would. Use the JavaScript import () to dynamically load a module. The import () returns a Promise that will be fulfilled once the module is loaded completely. Use the async / await to handle the result of the import (). Use the Promise.all () method to load multiple modules at once.
The if/else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed. The if/else statement is a part of JavaScript's "Conditional" Statements, which are used to perform different actions based on different conditions. The import and export statements are one of the most crucial features of JavaScript ES6. It allows you to import and export JavaScript classes, functions, components, constants, any other variables between one JavaScript file and another. This feature allows you to organize your JavaScript code into small, bite-size files. Javascript Import statement is used to import bindings that are exported by another module. Using the Javascript import, the code is easier to manage when it is small and bite-size chunks. This is the thinking behind keeping functions to only one task or having files contain only a few or one component at a time.
import The static import statement is used to import read only live bindings which are exported by another module. Imported modules are in strict mode whether you declare them as such or not. The import statement cannot be used in embedded scripts unless such script has a type="module". import() in JavaScript. import() is an ES6 module. Together with export(), they are commonly referred to as ES6 import and export. This essentially means that the statements can not be used with other file types outside the ES modules. The import() statement lets the user import his modules and use them in the program. The syntax is: In JavaScript, variables can be accessed from another file using the <script> tags or the import or export statement. The script tag is mainly used when we want to access variable of a JavaScript file in an HTML file. This works well for client-side scripting as well as for server-side scripting.
JavaScript developers have been using modules with libraries' aid till the community introduced them as a built-in feature in ES6. Now let's take an example of using an import statement or modules, to illustrate the way you can use them and why they didn't work the first time I tried them. Uncaught SyntaxError: Cannot use import statement outside a module" when importing ECMAScript 6 Common Cases of "SyntaxError: Cannot use import statement outside a module" This can happen in different cases depending on whether you're working with JavaScript on the server-side with Node.js, or client-side in the browser. Node.js In the above code, we have used import statement followed by curly-braces and file path. Inside the curly braces, we have specified the name of the functionalities we need to import separated by the comma. Now in our html file, we can add this main.js module using script tag with a type=module. <!
The import statement can be used to import an entire package or sometimes import certain classes and interfaces inside the package. The import statement is written before the class definition and after the package statement (if there is any). Also, the import statement is optional. A program that demonstrates this in Java is given as follows: The import () expression The import (module) expression loads the module and returns a promise that resolves into a module object that contains all its exports. It can be called from any place in the code. We can use it dynamically in any place of the code, for instance: Today I Learned (TIL): About the usage of the @ symbol in the JavaScript import statement. I am busy working on a new project and I am using Vue to develop the front-end. I used the Vue Webpack template to bootstrap the project, and I have noticed the usage of the @ symbol in the import statement in various places.
The ECMAScript 2015 specification introduced modules to the JavaScript language, which allowed for the use of import and export statements. In this tutorial, you will learn what a JavaScript module is and how to use import and export to organize your code. Conclusion: Import and export statements in JavaScript and how to use them Import and export statements are two great features introduced ES6 (ES2015). These two statement allows you to export and import your code and use it whenever you need. This tutorial will show you what import and export statements are, how they work and how to use them. From a JavaScript language perspective, the module identifier is completely opaque. So it really depends on which module loader/bundler you are using. You most likely have something like babel-plugin-root-import in your webpack/babel config.
JS modules were not a thing when Node was first built, and so it uses a module format called CommonJS. In CommonJS, you import modules like require('my-module'). Node has a built-in package manager called NPM. You can install modules via NPM, and those modules go into the node_modulesfolder. Now, importing is an operation that requires the permission of the module. Importing is possible only if the module or named property to be imported has been exported in its declaration. In React we use the keyword export to export a particular module or a named parameter or a combination. The import statement is used to import bindings that are exported by another JavaScript file. Code manageability is the most import factor in web development. If you are building an application that has a large codebase, it isn't very easy to manage that codebase.
4 Best Practices To Write Quality Javascript Modules
Close Up Of Import Statement Of React Javascript Programming
Javascript Programming With Visual Studio Code
R 39 N 39 B On Twitter I Like Import And Export Features
Build An Api With Node Js Express And Typescript Split
Vs Code Tips Import Statement Completions In Javascript And
Customer Profile With Api Key 3 Insert The Required
Migrate Sharepoint Javascript Customizations To Sharepoint
Typescript Documentation Typescript 4 3
Es6 Import And Export Geeksforgeeks
Import Module In Python With Examples
Techniques To Bulk Copy Import And Export In Sql Server
Using Typescript With The Arcgis Api For Javascript
Reduce Javascript Payloads With Tree Shaking Web Fundamentals
Uncaught Syntaxerror Cannot Use Import Statement Outside A
Asynchronous Javascript Using Promises With Rest Apis In Node Js
Cannot Use Import Statement Outside A Module React
Import And Export Statements In Javascript And How To Use Them
0 Response to "21 Import Statement In Javascript"
Post a Comment