28 Regular Expression For Numbers In Javascript
JavaScript regular expression In JavaScript, we build regular expressions either with slashes // or RegExp object. A pattern is a regular expression that defines the text we are searching for or manipulating. It consists of text literals and metacharacters. 1.1.1 Address Code Rules: Address code length 6 bits. Start with numbers 1-9. The last 5 digits are 0-9 digits. According to the above rules, write the regular expression of address code: /^ [1-9]\d {5}/. 1.1.2 year code rule: Year code length 4 bits. Start with numbers 18, 19 or 20. The remaining two digits are 0-9.
Playing With Regexp Named Capture Groups In Node 10
Regular expressions via Wikipedia: A sequence of characters that forms a search pattern, mainly for use in pattern matching with strings, or string matching. RegEx is nice because you can accomplish a whole lot with very little. In this case we are going to check various aspects of a string and see if it meets our requirements, being a strong ...
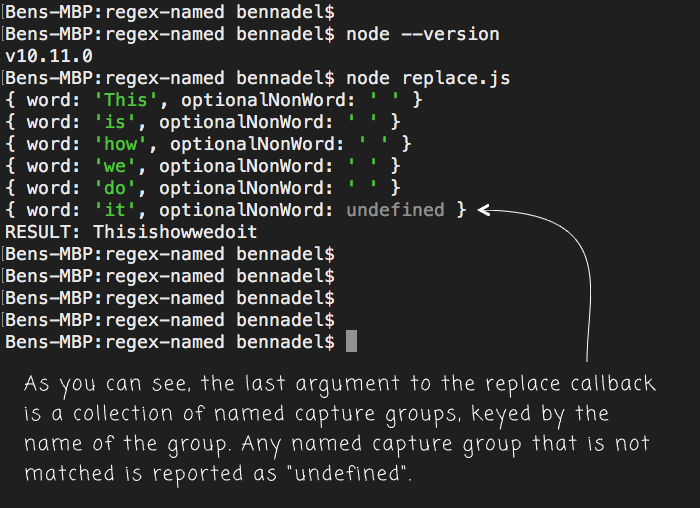
Regular expression for numbers in javascript. In this video tutorial, we will understand repeating characters, special characters & perform mobile number validation.A regular expression in JS is an objec... Give it a TRY! » Note: The regular expression removes parentheses, spaces and dashes, but numbers or letters will remain intact.. JavaScript Form Validation: Removing Nondigits. Nondigit(i.e character that is not a digit ) can be removed using the regular expression [^0-9] or \D. Eg: nondigits in zipcodes, phone numbers etc.. Example: JavaScript Form Validation: Removing Non Digits. Give it a ... Matching Numbers in JavaScript Strings using Regular Expressions. Learn how to match number patterns such as phone numbers and credit cards in the unstructured text using JavaScript Strings This video is a follow-up video for Search a String by matching against a regex in JavaScript.
Javascript RegExp¶ Regex or Regular expressions are patterns used for matching the character combinations in strings. Regex are objects in JavaScript. Patterns are used with RegEx exec and test methods, and the match, replace, search, and split methods of String. The test() method executes the search for a match between a regex and a specified ... The rule of thumb is that simple regular expressions are simple to read and write, while complex regular expressions can quickly turn into a mess if you don't deeply grasp the basics. How does a Regular Expression look like. In JavaScript, a regular expression is an object, which can be defined in two ways. Regular expressions allow you to check a string of characters like an e-mail address or password for patterns, to see so if they match the pattern defined by that regular expression and produce actionable information. Creating a Regular Expression. There are two ways to create a regular expression in Javascript.
To get a string contains only letters and numbers (i.e. a-z, A-Z or 0-9) we use a regular expression /^ [0-9a-zA-Z]+$/ which allows only letters and numbers. Next the match () method of string object is used to match the said regular expression against the input value. Here is the complete web document.+ The regular expression uses a look-ahead to find positions within the string where the only thing to the right of it is one or more groupings of three numbers, until either a decimal or the end of string is encountered. JavaScript regular expressions automatically use an enhanced regex engine, which provides improved performance and supports all behaviors of standard regular expressions as defined by Mozilla JavaScript. The enhanced regex engine supports using Java syntax in regular expressions. The enhanced regex
A regular expression can easily check whether a user entered something that looks like a valid phone number. Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec and test methods. In JavaScript, regular expressions are represented by RegExp object, which is a native JavaScript object like String, Array, and so on. There are two ways of creating a new RegExp object — one is using the literal syntax, and the other is using the RegExp () constructor. JavaScript. JavaScript Guide. Regular expressions. Regular expression syntax cheatsheet. ... A regular expression may have multiple capturing groups. In results, matches to capturing groups typically in an array whose members are in the same order as the left parentheses in the capturing group. ... Quantifiers indicate numbers of characters or ...
A JavaScript Regular Expression is an object, which specifies a pattern of characters. Moreover, a typical example of a regular expression implementation is the find and replace functionality provided by all the text editors. Apart from it, one can use regex in the below scenarios: First, we can split the string into multiple strings. There are two ways you can create a regular expression in JavaScript. Using a regular expression literal: The regular expression consists of a pattern enclosed between slashes /. For example, cost regularExp = /abc/; Here, /abc/ is a regular expression. Using the RegExp() constructor function: You can also create a regular expression by calling the RegExp() constructor function. For example, To my knowledge, this matches all the variations on numbers that Java and JavaScript will ever throw at you, including "-Infinity", "1e-24" and "NaN". It also matches numbers you might type, such as "-.5". As written, reSnippet is designed to be dropped into other regular expressions, so you can extract (or avoid) numbers.
A regular expression is a string that describes a pattern e.g., email addresses and phone numbers. In JavaScript, regular expressions are objects. JavaScript provides the built-in RegExp type that allows you to work with regular expressions effectively. Regular expressions are useful for searching and replacing strings that match a pattern. In JavaScript, regular expressions are also objects. These patterns are used with the exec () and test () methods of RegExp, and with the match (), matchAll (), replace (), replaceAll (), search (), and split () methods of String. This chapter describes JavaScript regular expressions. Creating a regular expression 12/12/2014 · Regular Expression in JS for numbers. Accept only numbers between 6 and 15 digits, 6 is the minimum. Numbers cannot contain groups of repeated digits, such as 408408 or 123123 Numbers cannot contain only two different digits, such as 121212.
To get a string contains only numbers (0-9) we use a regular expression (/^ [0-9]+$/) which allows only numbers. Next, the match () method of the string object is used to match the said regular expression against the input value. Here is the complete web document. Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The .replace method is used on strings in JavaScript to replace parts of Regular expression examples in JavaScript, regex syntax, regular expression pattern matching example, regular expression for email, numbers only, mobile number, form validation, name and password, URL, CVV, Expiry Date.
var patt = /w3schools/i. Try it Yourself ». Example explained: /w3schools/i is a regular expression. w3schools is a pattern (to be used in a search). i is a modifier (modifies the search to be case-insensitive). For a tutorial about Regular Expressions, read our JavaScript RegExp Tutorial. The easiest way to validate phone numbers using Javascript would be to use Regular expressions. However there are different formats of Phone numbers depending on the user's preference. Here are some regular expressions to validate phone numbers. Phone number validation using a simple Regular expression Javascript code to match pattern and display result: aadhar card validation. 1. " function validation () " is a function that checks the number. 2. Here " regexp" is the variable. 3. In " document.getElementById ("aadhaar").value " we are taking entered aadhaar number from user. 4." (regexp.test (x)) "in this condition, we ...
In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a String See " Regular Expression in JavaScript " for full coverage. In JavaScript (and Perl), a regex is delimited by a pair of forward slashes, in the form of /.../. There are two sets of methods, issue via a RegEx object or a String object. JavaScript Regular Expression for Phone Number Verification; JavaScript Regular Expression for Phone Number Verification. 2020-04-23 14:27:27. 100620. 0. javascript. It comes as no surprise to any business owner that communication is key. The ability to collect a phone number allows businesses to verify information from clients, expand outreach ...
Link for all dot net and sql server video tutorial playlistshttp://www.youtube /user/kudvenkat/playlistsLink for slides, code samples and text version of ... In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a Regular Expression :
Regex For Iranian Mobile Phone Numbers Github
Form Validation Using Js Regular Expressions Menyhart Media
Regular Expression Javascript To Check Filename To See If
Javascript Regular Expression How To Create Amp Write Them In
Basic Regex In Javascript For Beginners Dev Community
Javascript Validation With Regular Expression Count Number
How Javascript Works Regular Expressions Regexp By
Regex Tricks Change Strings To Formatted Numbers 231webdev
Javascript Regular Expression How To Create Amp Write Them In
Everything You Need To Know About Regular Expressions By
Javascript Regex Only Capturing First Match Stack Overflow
A Beginner S Guide To Regular Expressions In Javascript
8 Regular Expressions You Should Know
Introduction To The Use Of 10 Regular Expressions In
2 Using Regular Expressions Javascript Cookbook Book
Essential Guide To Regular Expressions Tools And Tutorials
Regular Expressions Eloquent Javascript
Useful Regular Expressions For Validating Input In Google
Javascript Phone Number Validation W3resource
Find And Replace Text Using Regular Expressions Pycharm
Everything You Need To Know About Regular Expressions By
Form Validation In Javascript Using Regular Expression Part1
Javascript Regular Expressions
Everything You Need To Know About Regular Expressions By
Javascript Regular Expression Want To Exclude Function Words
How To Replace Number With String In Javascript
0 Response to "28 Regular Expression For Numbers In Javascript"
Post a Comment