31 How To Build A Javascript Library
Jul 24, 2018 - Before start, I recommend you to read my previous article about creating extra small JavaScript libraries. ... I will start from one moment that isn’t quite relevant to the topic of the article but is important —why do we need to build libraries? May 31, 2016 - Tim Severien discusses a wide variety of tips and tricks to build your own JavaScript library, ranging from API design to testing and documentation.
How To Build Your Own Javascript Library
Creating libraries properly is a vital topic, but the sources are scarce. This article will show how to squeeze the most out of the modern build toolchain: how to configure the tools, how to create an isomorphic library, and how to prevent the unused parts of the application from landing in clients' applications.
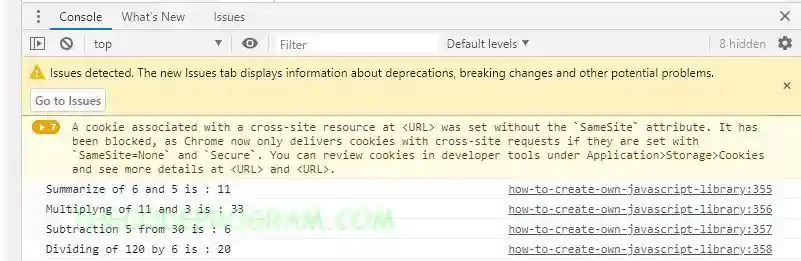
How to build a javascript library. JavaScript community is amazing. We have so many things at our disposal to make our lives easy. Take an example of npm. Before npm was a thing, distributing JavaScript libraries was such a pain. Create a new file called winner.js under app folder. This will be a new ES6 module. We calculate the winner here in this module. We will export this module to use it inside index.js, where we can create instance of this module and use it to determine the winner. We will make use of another excellent feature of ES6 i.e class. 1/4/2018 · If you are taking preexisting code to turn into a library, you just need to divide the various functions into two groups: those necessary at instantiation, and those no necessary at instantiation.
Diese Domain steht zum Verkauf Algolia Places is a JavaScript library that will help you autocomplete forms, and it specializes in addresses. You can even add a map to the search and display the location, which is incredibly useful. It's wonderfully accurate and super fast, which will definitely increase the user experience on your site. Check it out. The big 4: most popular JavaScript libraries for frontend React. React is one of the most popular JavaScript libraries that let you create complex and interactive user interfaces (UI). This library has multiple strengths and advantages when it comes to building one of the main elements of the frontend.
The build command: "build": "esbuild --bundle src/index.js --outfile=dist/main.js --minify" Build output. Both bundlers build the application code as they should - importing the one method we used and ignoring the other one. Interestingly, we didn't need to set "sideEffects": false to make it happen - just using ES modules seems to be enough. Aside from applications, webpack can also be used to bundle JavaScript libraries. The following guide is meant for library authors looking to streamline their bundling strategy. Create a directory for your package on the command line, where $package_name is your actual package name. $ mkdir $package_name && cd $package_name After determining the name of your package, you'll need to know whether it's going to be scoped (i.e., package vs. @username/package).
23/6/2015 · But this approach it's not recommended. Instead of it, you can create a new JS file to run your library code. <script type="text/javascript" src="resources/Utilities.js"></script> <script type="text/javascript" src="resources/main.js"></script> In main.js. Utilities.show("Hello"); Hope it helps. Oct 17, 2016 - Now that I have explained why you need a personal custom library as a developer, let’s get started on creating one. ... We are going to build a JavaScript library that has similar functionalities as jQuery. It manipulates a selected DOM element when creating HTML elements or editing pre-existing ... how to create javascript library of self-written functions [closed] Ask Question Asked 8 years, 3 months ago. Active 4 years, 9 months ago. Viewed 26k times 10 5. It's difficult to tell what is being asked here. This question is ambiguous, vague, incomplete, overly broad, or rhetorical and cannot be reasonably answered in its current form.
Feb 20, 2018 - As a frontend engineer, I have ... topic of libraries building. I’ll try to explain how to squeeze the most out of the modern build toolchain, that is: ... CJS (a.k.a CommonJS) is a module format popularized by node.js ecosystem, where ESM (ECMAScript Modules) is a module format already standardized as part of the JavaScript language (since ... 5/10/2012 · Step 1: Creating the Library Boilerplate. We’ll start with some wrapper code, which will contain our whole library. It’s your typical immediately invoked function expression (IIFE). window.dome = (function () { function Dome (els) { } var dome = { get: function (selector) { } }; return dome; } ()); As you can see, we’re calling ... We need our own JavaScript utility library that we can distribute via NPM. TL;DR. Here's an example GitHub repo with my personal util functions after which you can pattern your architecture: @paravano/utils. A set of JavaScript utils. Contribute to @paravano/utils development by creating an account on GitHub.
Javascript create own library like jquery. Ask Question Asked 2 years, 6 months ago. Active 2 years, 6 months ago. Viewed 523 times 1 I'm trying to create a library and i want to achieve something like JQuery, when we type $(selector) . then the IDE will show a ... Step 1: Identify the purpose of your library. This might just be one of the toughest parts of the whole process! If you are to the point in your JavaScript knowledge that you would like to build your own library, than you've likely used jQuery, mooTools, Prototype, or any number of other popular libraries. Jun 13, 2020 - It's time to use the template that we developed in the previous article 🚀 I want to develop my own s...
Again, this is similar to how jQuery extends itself (also see the "Build Your Own Library" reference). Examples See attached files - I apologize that these are mostly out-of-context, and probably have too much extra junk to be very simple to follow, but should give you an idea of what you can do with a library. For the UI Library, we need to transpile the source with Babel with the es module system as a target, and place it in lib. We can even host the lib on a CDN. We should bundle and minify the source using rollup for cjs/umd module system and es module system as a target. Modify the package.json to point to the proper target systems. 💖 My Website ️ CodeHawke 🦅 - https://www.codehawke /all_access.htmlJoin thousands of satisfied students by choosing the All Access option today. All ...
Let's create the source code for our library. For that we will be create two utility functions into two separate files in our source folder. Step 1 : Create a directory demo and run following command in it. $ npm init -y. The above command will create a package.json in your project root. I am using a --y to initialize it with default options ... So generally, libraries are .js files along with .css files (if you need to add a lot of custom styling). All the functions used in the library, along with variables, even HTML templates to be appended, are wrapped up into a class in the .js file. I previously wrote an article on JavaScript classes which could probably help you out there. Dec 30, 2015 - Start your own JavaScript library using webpack and ES6 / Two months ago I published a starter pack for React based on webpack. Today I found out that I need almost the same thing but without the React bit. This simplifies the setup but there are still some tricky parts.
If you prefer to create your library in the simplest way possible, you can simply type your code into a JavaScript file and place this file in every project you want to use. However, this adds the problem of versioning. Once you update your library, for example to fix a bug, you will need to manually update the file in every project. JavaScript is one of the 3 languages all web developers must learn: 1. HTML to define the content of web pages. 2. CSS to specify the layout of web pages. 3. JavaScript to program the behavior of web pages. This tutorial covers every version of JavaScript: The Original JavaScript ES1 ES2 ES3 (1997-1999) Mar 28, 2018 - I’m oversimplifying things, of ... in our build tools wasn’t exactly trivial. But once we figured out how to configure it, using DEGJS within JSPM really has become an incredibly powerful, yet easy-to-use, part of our day-to-day JS workflow. ... Some of the benefits of using a self-curated JavaScript library might seem ...
Build your Own JavaScript Library - Part 1. Over the course of the past few years, the JavaScript community has exploded exponentially. It it's safe to assume that libraries are the primary reason we've seen such astonishing growth. Thanks to these libraries, the differences between the popular browsers are minimized, making cross-browser ... Jun 29, 2020 - If you’re a developer it’s almost certain that you’ve used Code Libraries. In simple terms, a Code Library is a collection of reusable code that you can use in your program. Like most of us, I know… 9/2/2016 · In this tutorial, we are going to create a simple library with pure javascript without prototyping, we'll use native objects instead. If you want to know what's the difference between the use of prototype and manage manually objects, this post should help to clear your doubts. 1) Create the basic structure
When you create a library only for Node.js. Just use require instead of ES6 modules. If you want to reduce the size, you can split your library to several files, moving optional parts, utils and something else out of library core. When you create a library for modern frontend only. Use ES6 modules and don't care about. Don't build it. DHTMLX Suite is a JavaScript UI widget library for developing feature-rich web apps. Each of the 20+ widgets supports a wide configuration via a comprehensive API. Thus, users will be able to work with large data sets, enjoy inline editing and convenient content filtering, use drag-n-drop, as well as export data to Excel or CSV. Publishing a JavaScript library for public use requires some extra steps. You need to think about how people will use the library. From end users, to contributors your library now has a variety of people outside of yourself potentially making use of the code that you've released into the wild.
Many web developers create a special directory for external JavaScript files in the site's root folder: common names are js (meaning JavaScript) or libs (meaning libraries). UP TO SPEED: URL Types When attaching an external JavaScript file to a web page, you need to specify a URL for the src attribute of the <script> tag. In this tutorial I go over a super simple way to make your own library that is really similar to jQuery. I hope you like it! Please subscribe if you found th...
How To Build A Pdf Library With React Foxit Pdf Libraries
How To Create A Javascript Library 7 Tips To Create A
Build Your First Javascript Library Like Jquery
Create A Javascript Library And Fully Automate The Releases
A Full Comparison Of 6 Js Libraries For Generating Pdfs Dev
Add External Js Libraries To Eclipse Jsdt Driven Projects
Essential Javascript Libraries And Frameworks You Should Use
10 Javascript Libraries To Draw Your Own Diagrams 2020 Edition
Lineman Js Streamlines Building Javascript Client
Eclipse How To Extend The Build In Javascript Libraries
10 Most Popular Javascript Libraries To Build Your Next Project
20 Useful Javascript Data Table Libraries Bashooka
How To Write And Build Js Libraries In 2018 By Anton Kosykh
How To Create A Javascript Library 7 Tips To Create A
Writing Your First Javascript Library Hacker Noon
Create Your Own Javascript Library
Tensorflow Js Machine Learning For Javascript Developers
Top 20 Javascript Libraries You Should Know In 2021
A List Of Foundational Javascript Tools
Eclipse How To Extend The Build In Javascript Libraries
Scrollerjs Javascript Library To Build Performant Ui
How To Write A Javascript Library
How To Create A Javascript Library 7 Tips To Create A
Build Your Javascript Library And Event Listeners
How To Write And Build Js Libraries In 2018 By Anton Kosykh
D3 Js Tutorial Build Your First Bar Chart By The Educative
20 Javascript Ui Frameworks Amp Libraries For Better Frontend
The 12 Things You Need To Consider When Evaluating Any New
2020 The Year Of Learning Javascript Learning Solutions
0 Response to "31 How To Build A Javascript Library"
Post a Comment