27 Remove Array Javascript By Key
Syntax -. array-name.splice (removing index, number of values [, value1,value2,... ]); The first parameter is the index of the removing item. The number of items is being removed. For example - you have specified 2 in this case this removes the index value and the next one also. It is an optional parameter from here you can set new values. When you work with arrays, it is easy to remove elements and add new elements. This is what popping and pushing is: Popping items out of an array, or pushing items into an array. ... Since JavaScript arrays are objects, elements can be deleted by using the JavaScript operator delete: Example.
Removing Duplicates In An Array Of Objects In Js With Sets
To remove a particular element from an array in JavaScript we'll want to first find the location of the element and then remove it. Finding the location by value can be done with the indexOf() method, which returns the index for the first occurrence of the given value, or -1 if it is not in the array.
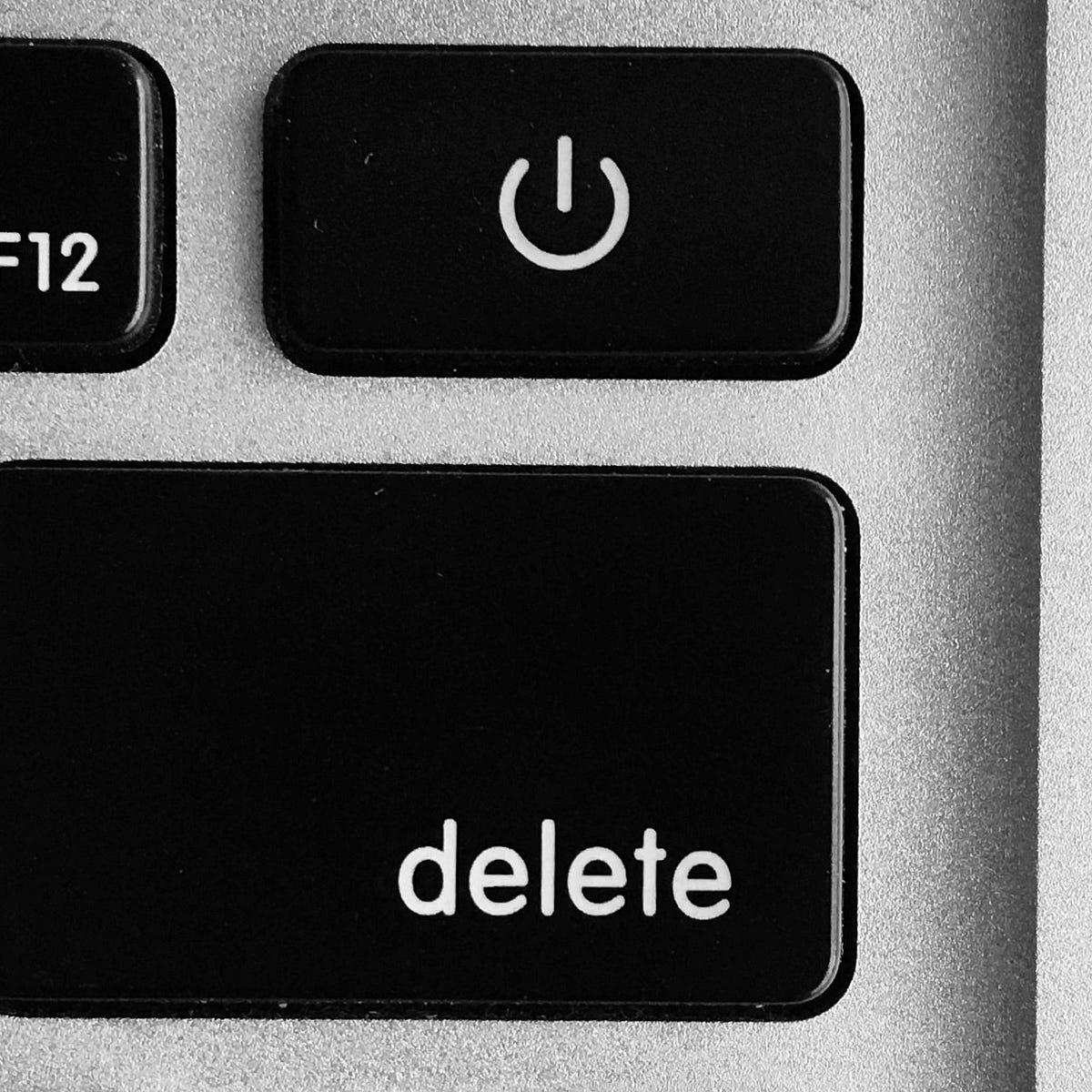
Remove array javascript by key. In the above program, an item is removed from an array using a for loop. Here, The for loop is used to loop through all the elements of an array. While iterating through the elements of the array, if the item to remove does not match with the array element, that element is pushed to newArray. The push() method adds the element to newArray. Our goal is to remove all the falsy values from the array then return the array. The good people at freeCodeCamp have told us that falsy values in JavaScript are false, null, 0, "", undefined, and NaN. They have also dropped a major hint for us! They suggest converting each value of the array into a boolean in order to accomplish this challenge. Definition and Usage. The keys () method returns an Array Iterator object with the keys of an array. keys () does not change the original array.
How do I remove an element from an array? Is the delete operator of any use? There also exists funny named splice() and slice(), what about those? I want to modify the array in-place. Use splice() to remove arbitrary item. The correct way to remove an item from an array is to use splice(). It takes an index and amount of items to delete ... javascript find and remove object in array based on key value (14 answers) Closed 2 days ago . I have an array of objects and want to remove all the objects that have a specific key value. Setting a property to undefined. If you need to perform this operation in a very optimized way, for example when you're operating on a large number of objects in loops, another option is to set the property to undefined.. Due to its nature, the performance of delete is a lot slower than a simple reassignment to undefined, more than 50x times slower. ...
Summary: in this tutorial, you will learn how to remove duplicates from an array in JavaScript. 1) Remove duplicates from an array using a Set. A Set is a collection of unique values. To remove duplicates from an array: First, convert an array of duplicates to a Set. The new Set will implicitly remove duplicate elements. Find the indexof the array element you want to remove using indexOf, and then remove that index with splice. The splice() method changes the contents of an array by removing existing elements and/or adding new elements. const array = [2, 5, 9]; If, for whatever reason, the delete key is not working (like it wasn't working for me), you can splice it out and then filter the undefined values: // To cut out one element via arr.splice(indexToRemove, numberToRemove); array.splice(key, 1) array.filter(function(n){return n}); Don't try and chain them since splice returns removed elements;
JavaScript array splice() Javascript array splice() is an inbuilt method that changes the items of an array by removing or replacing the existing elements and/or adding new items. To remove the first object from the array or last object from the array, then use the splice() method. The JavaScript array has a variety of ways you can delete array values. There are different methods and techniques you can use to remove elements from JavaScript arrays: pop — Removes from the ... 23/10/2020 · How to Remove an Object from an Array by Key in JavaScript. Published Oct 23, 2020. Suppose we have an array of objects and want to remove an object from the array by key. let arr = [ { id: 0, dog: "corgi" }, { id: 1, dog: "shih tzu" }, { id: 2, dog: "pug" }, ]; We can remove an object with a specific key using the built-in filter method.
The Map.delete () method in JavaScript is used to delete the specified element among all the elements which are present in the map. The Map.delete () method takes the key which needs to be removed from the map, thus removes the element associated with that key and returns true. If the key is not present then it returns false. Remove object from array of objects in Javascript. GitHub Gist: instantly share code, notes, and snippets. 10/6/2012 · To remove a property from an object (including an array), use delete: delete theArray['0315778255cca8d2f773642ad1d3678f']; Despite its similarity to “delete” in other languages, delete in JavaScript has nothing (directly) to do with memory management; all it does is remove a property from an object (entirely; it doesn’t just set the property’s value to undefined or something).
One of the things that _isn't so easy is removing duplicate objects from a JavaScript array of objects. In this quick tip, I'll provide you with a simple function that will return an array of JavaScript objects with duplicates removed. JavaScript function. First, let's take a look at the function which accepts two arguments: The JavaScript delete operator removes a property from an object; ... or simulate this structure with two separate arrays (one for the keys and the other for the values), or build an array of single-property objects, etc. Examples ... When you delete an array element, ... Splice array javascript cung cấp một method array.splice() để giải quyết việc nà y. và chúng ta cũng có thể hiểu index là key hoặc javascript remove element from array by key. Giả sỠbạn có một array và bạn biết xoá positioni = 2
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: Key means id, name, code, etc. Remove duplicate objects from an array using js array methods like, new Set (), forEach (), for loop, reduce (), filter () with findIndex () methods. Or you should also read this javascript array posts: Convert Array to Comma Separated String javaScript Removing the "FIRST" Elements from JavaScript Array Use the shift () method to remove the very first element from the Javascript array. No parameters are required, this method only removes the first element and the remaining elements are shifted down.
9/1/2021 · JavaScript Array elements can be removed from the end of an array by setting the length property to a value less than the current value. Any element whose index is greater than or equal to the new length will be removed. var ar = [1, 2, 3, 4, 5, 6]; ar.length = 4; console.log( ar ); How to delete an property or key from an object array. Suppose you have object array and wants to return new array with removing an key from an array Array has forEach function which contains callback called for every object, use delete syntax to delete and return an new array of boject The result is that all objects in the old array are mapped to a new object with no name key. You could also, with object destructuring, filter put unwanted properties like so: let newPetList = petList.map(({ name, ...rest }) => rest); This binds the name property to name and keeps the rest in rest, which you can return to remove the name key.
Using unset () Function: The unset () function is used to remove element from the array. The unset function is used to destroy any other variable and same way use to delete any element of an array. This unset command takes the array key as input and removed that element from the array. After removal the associated key and value does not change. JavaScript suggests several methods to remove elements from existing Array. You can delete items from the end of an array using pop (), from the beginning using shift (), or from the middle using splice () functions. 4/1/2020 · Calling the delete operator on one key array like the example will result that the space selected is gone. Adding method to Array.prototype. JavaScript is very flexible, I’ve seen somewhere when they add directly a new method to the Array.prototype with something like this: Array.prototype.remove = function(value){ /* ...
5/4/2017 · function removeKeys(array,keys){ for(var i=0; i<array.length; i++){ for(var key in array[i]){ for(var j=0; j<keys.length; j++){ if(keys[j] == key){ array[i].splice(j, 1); } } } } } removeKeys(myArray ,["id"]); The result array should look like:
How To Delete An Item From An Array In React Vegibit
Java67 How To Remove A Number From An Integer Array In Java
How To Remove Duplicates From A Javascript Array Devimal Planet
Javascript Array A Complete Guide For Beginners Dataflair
Javascript Array Remove Element From Array Tuts Make
Javascript Hash Table Associative Array Hashing In Js
7 Ways To Remove Duplicates From An Array In Javascript By
Javascript Array Remove Index Key
Remove Element From Array Javascript First Last Value
Javascript Array Methods How To Use Map And Reduce
How Can I Remove A Specific Item From An Array Stack Overflow
How To Remove And Add Elements To A Javascript Array
How Can I Remove Elements From Javascript Arrays O Reilly
How To Remove Array Duplicates In Es6 Samanthaming Com
How To Remove Duplicate Entries In Array In Javascript
Javascript How To Remove An Item From Array Tutorial
Hacks For Creating Javascript Arrays
Remove Element From Array Javascript First Last Value
Javascript Array Remove An Element Javascript Remove Element
Remove Key From Array Javascript Code Example
Remove A Specific Element From Array
How To Remove A Key From An Object In Javascript By Dr
6 Use Case Of Spread With Array In Javascript Samanthaming Com
How To Move An Array Element From One Array Position To
Removing Empty Or Undefined Elements From An Array Stack
0 Response to "27 Remove Array Javascript By Key"
Post a Comment