32 Document Ready Plain Javascript
Vanilla JS equivalents of jQuery methods. GitHub Gist: instantly share code, notes, and snippets. Oct 01, 2017 - Now lets talk about the difference between those methods First of all ,all of them can be used to check if the DOM is fully loaded and ready to be manipulated by your JavaScript code . Secondly , $(document).ready() and $(window).load() are jQuery methods and not pure JavaScript methods so ...
Trigger A Keypress Keydown Keyup Event In Js Jquery
Example: js vanilla dom ready document.addEventListener("DOMContentLoaded", function() { // code });
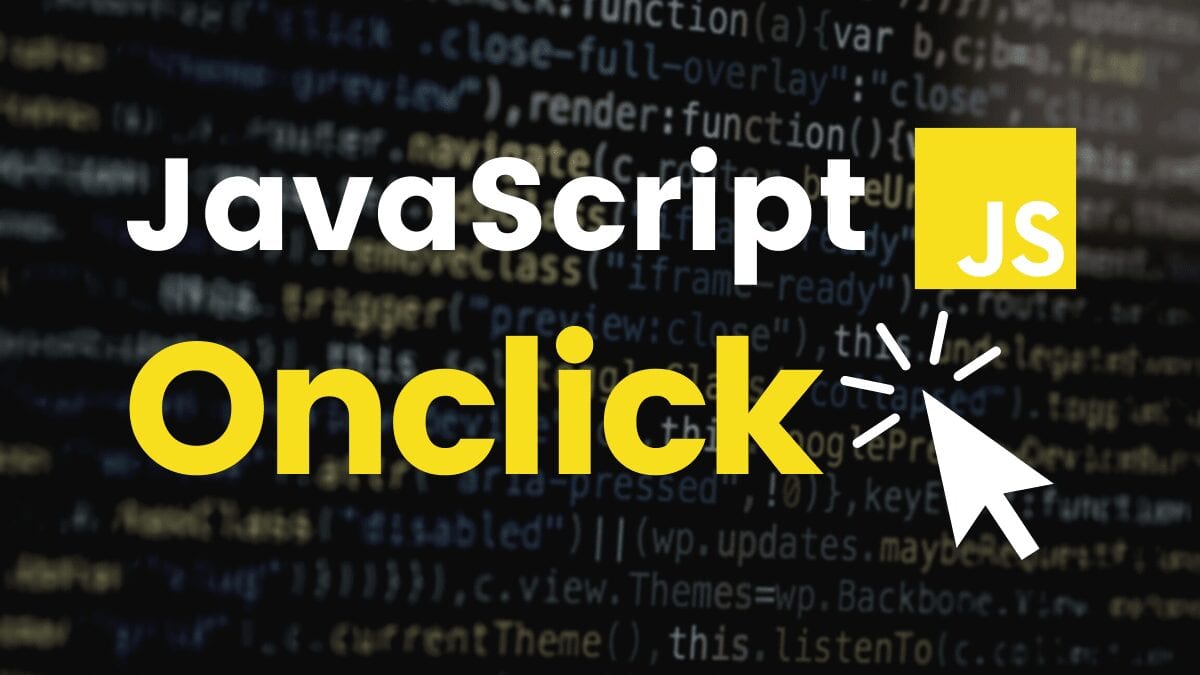
Document ready plain javascript. 4. If the ready handler is fired, then just schedule the newly added callback to fire right after this thread of JS finishes with setTimeout(fn, 1). 5. But, if the ready handler has not already fired, then add this new callback to the list of callbacks to be called later. 6. Now, check if the document is already ready. If so, execute all ready ... In this article, we'll look at the vanilla JavaScript equivalent of jQuery features. Wait for Document to be Ready With jQuery, we can use the $ (document).ready callback to run code when the DOM is loaded: $ (document).ready (() => { The readyState of a document can be one of following: The document is still loading. The document has finished loading and the document has been parsed but sub-resources such as scripts, images, stylesheets and frames are still loading. The document and all sub-resources have finished loading. The state indicates that the load event is about to ...
Apr 02, 2020 - Get code examples like "document ready plain javascript" instantly right from your google search results with the Grepper Chrome Extension. Example: js vanilla dom ready document.addEventListener("DOMContentLoaded", function() { // code }); To check if the document is ready and execute some code, you bind an event listener to the DOMContentLoaded event of the document object: document.addEventListener("DOMContentLoaded", handler); ... The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively. Recent Tutorials.
With jQuery, adding, removing and checking if an element has certain classes is really simple. It's a bit more complex with plain JavaScript, but not too much so. Giving element a class called "foo" and replacing all the current classes: var element = document.querySelector(".some-class"); Detect if document is ready in pure JavaScript While JavaScript does provide the load event for executing code when a page is rendered, this event does not get triggered until all assets such as images have been completely received. In most cases, the script can be run as soon as the DOM hierarchy has been fully constructed. There are three options: If script is the last tag of the body, the DOM would be ready before script tag executes When the DOM is ready, "readyState" will change to "complete" Put everything under 'DOMContentLoaded' event listener
Running code when the document is ready. A page can't be manipulated safely until the document is "ready." Here's how to make sure code isn't run prematurely. This function is the equivalent of jQuery's $ (document).ready () method: document. addEventListener ( 'DOMContentLoaded', function () … 11/8/2021 · To find the equivalent of jQuery’s $(document).ready in plain JavaScript, we can listen to the DOMContentLoaded event on the document object with the addEventListener method. To do this, we write: document.addEventListener("DOMContentLoaded", (event) => { console.log('document ready') }); to listen to the DOMContentLoaded event on document . Nov 20, 2016 - If you’re searching for a plain JavaScript alternative for the ready method you can proceed with the DOMContentLoaded event. If your system requirements include IE < 9 you can use the onreadystatechange event. If you’re using jQuery in your project you can safely proceed with using the jQuery document ...
Javascript has a couple of alternatives to jQuery's $(document).ready()function, which is used to detect when the DOM has loaded and is ready to be manipulated. A typical jQuery example would look something like this: // wait until DOM has loaded before running code ES6-DocReady A replacement, using plain javascript and ES6 module syntax, for jQuery's.ready () to run codes after the document is ready. Check if the document is already ready. If so, execute all ready handlers. If we haven't installed event listeners yet to know when the document becomes ready, then install them now. If document.addEventListener exists, then install event handlers using .addEventListener() for both "DOMContentLoaded" and "load" events. The "load" is a ...
Document ready. If you need to wait for the DOM to fully load before e.g. attaching events to objects in the DOM, you'd typically use $(document).ready() or the common short-hand $() in jQuery. We can easily construct a similar function to replace it with by listening to DOMContentLoaded: Now web browsers and rendering engines are much more smarter and increasingly support standard javascript API. Document ready function is widely used feature from jQuery. With growing trends in modern web development and much better browser support for vanilla JS API's, We can replace or reduce jQuery dependency easily. 11/12/2020 · Using vanilla JavaScript with no jQuery, the simplest way to check if the document is ‘ready’ to be manipulated is using the DOMContentLoaded event: document .addEventListener( 'DOMContentLoaded' , function ( ) { // do something here ... }, false );
docReady is a single plain javascript function that provides a method of scheduling one or more javascript functions to run at some later point when the DOM has finished loading. It works similarly to jQuery's $ (document).ready (), but this is a small single standalone function that does not require jQuery in any way. Apr 25, 2016 - 81 votes, 64 comments. jQuery is useful and all but I do not want to add it to my code just for the $(document).ready();, especially if I am not … So ready to get started? Note that we're not using jQuery - just plain JavaScript. Doing it this way will expose us to the inner workings of the raw script. Let's begin. Get all the links. First of all, we need to get all the links in the page. We have a simple utility called "document.links" - which returns an array of links!
16/1/2018 · Javascript Web Development Front End Technology. In jQuery, if you want an event to work on your page, you should call it inside the $ (document).ready () function. Everything inside it will load as soon as the DOM is loaded and before the page contents are loaded. $ (document).ready (function () { alert (“Document loaded successful!"); jQuery $(document).ready() Equivalent in Vanilla JavaScript May 17th, 2015 When you have JavaScript code that interacts with elements on a page, in order to avoid any error, you must wait for these elements to be added to the DOM. 38 Document Ready To Javascript. Written By Joan A Anderson Thursday, August 12, 2021 Add Comment. Edit. Document ready to javascript. Introduction To Jquery. Web Dev 7 Exploring Basic Javascript Dom Manipulations Without Jquery. Jquery Document Ready Alternatives Ppt Download. Javarevisited Difference Between Jquery Document Ready.
Check if the document is already ready. If so, execute all ready handlers. If we haven't installed event listeners yet to know when the document becomes ready, then install them now. If document.addEventListener exists, then install event handlers using .addEventListener() for both "DOMContentLoaded" and "load" events. The "load" is a backup ... Nov 17, 2018 - How to wait for the DOM ready event in plain JavaScript · How to run JavaScript as soon as we can, but not sooner ... I usually don’t use arrow functions inside for the event callback, because we cannot access this. In this case we don’t need so, because this is always document. Oct 06, 2014 - So, to replicate the $(document).ready() function in plain Javascript (i.e. not using jQuery), you can use one of the following. ... DOMContentLoaded is an event fired when the DOM has finished loading, but doesn't wait for stylesheets or images to load. In this respect, it's functionally identical to jQuery's $(document...
The reason is simple. .NET is injecting the script inline, into the DOM. So its functions are called before $(document).ready(), which fires after DOM is loaded. This means, your logical sequence of JavaScript calls has gone for a toss! The solution is even more simple. Just add $(document).ready() in your function definition: Apr 02, 2020 - Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript The cross-browser way to check if the document has loaded in pure JavaScript is using readyState. if (document.readyState === 'complete') { // The page is fully loaded } You can detect when the document is ready…
1. The JS file is executed when it is downloaded, and at this moment it blocks the DOM rendering process, so if you don't wrap your code inside `$(document).ready`, your code might get executed with an incomplete DOM tree (so you don't have all th... The nice thing about $(document).ready()is that it fires before window.onload. The load function waits until everything is loaded, including external assets and images. $(document).ready, however, fires when the DOM tree is complete and can be manipulated. If you want to acheive DOM ready, without jQuery, you might check into this library. Yes you can have 2 Document Ready Function in your jQuery code but they serve no purpose at all. You just need one document Ready Function and put all you jQuery codes there. The Document Ready Function can have 2 types of syntaxes: 1. First one [...
Traversing The Dom With Vanilla Javascript By Eli Landau
How To Create An Html Attribute Using Vanilla Javascript
Fetch Api Introduction To Promised Based Data Fetching In
Build A To Do App With Vanilla Javascript And Local Storage
Jquery To Ship With Asp Net Mvc And Visual Studio Scott
The Basic Software You Need To Build A Website
Rendering Pdf Files In The Browser With Pdf Js Pspdfkit
Typescript Support In Svelte Learn Web Development Mdn
Javascript Document Ready Example Web Code Geeks 2021
Introducing The Webassembly Backend For Tensorflow Js
How To Install An Faq Dropdown Accordion In Squarespace
Learn Vanilla Javascript Before Fancy Frameworks Snipcart
Workarounds Manipulating A Survey At Runtime Using
How To Do Javascript App Prototyping With Parceljs
Document Ready Function Don T Work Stack Overflow
Get Dataset Value Javascript Code Example
Can We Use Document Ready In Asp Net Page Stack Overflow
How To Create Better Tooltips With Plain Javascript And Css
How To Create A Download File Text Csv Button Laserfiche
How To Build End To End Custom Applications In Cloud Foundry
Web Components A Native Component Model For Ui
Javascript Best Practices Backbone Js And Mario For The Php
An Introduction To Web Components
From Jquery To Javascript How To Make The Move Dev
Engineered Code Blog Powerapps Portals Liquid And
8 Types Of Pdf Standards Each Serves A Unique Purpose
How Can I Hide Or Show Html Based On User S Role Or Group
Vanilla Js Is Plain Js Some More Info Stackoverflow Com A
Javascript Onclick A Step By Step Guide Career Karma
0 Response to "32 Document Ready Plain Javascript"
Post a Comment