25 How To Check Undefined Variable In Javascript
It is safe to use the undefined type in your JavaScript code to check the status of a variable. All new browsers support this type. Remember, a variable however, with a blank value is not undefined or null. var myName = ''; The variable myName has a value (blank, I would say), but it is neither null nor undefined. Menu How to check for undefined in JavaScript 16 September 2015. When a variable is declared without being assigned a value its initial value is undefined.. How do you check if a value is undefined in JavaScript?. The short answer
Undefined is also a primitive value in JavaScript. A variable or an object has an undefined value when no value is assigned before using it. So you can say that undefined means lack of value or unknown value.
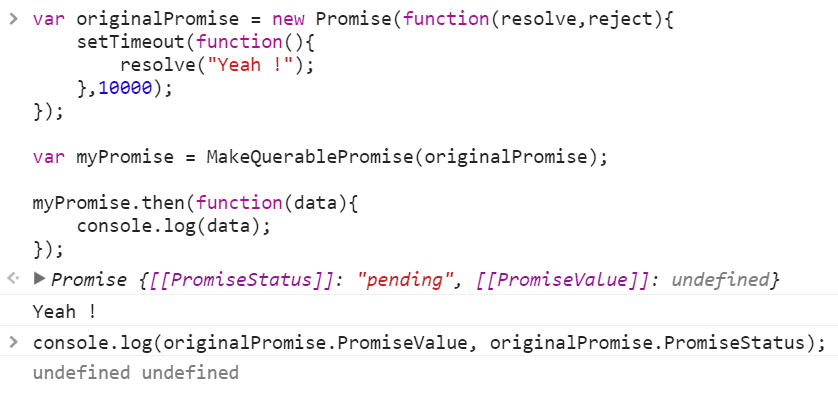
How to check undefined variable in javascript. Description. undefined is a property of the global object. That is, it is a variable in global scope. The initial value of undefined is the primitive value undefined. In modern browsers (JavaScript 1.8.5 / Firefox 4+), undefined is a non-configurable, non-writable property, per the ECMAScript 5 specification. To check if a variable is undefined, you can use comparison operators — the equality operator == or strict equality operator ===. If you declare a variable but not assign a value, it will return undefined automatically. Thus, if you try to display the value of such variable, the word "undefined" will be displayed. JQuery library was developed specifically to simplify and to unify certain JavaScript functionality. However if you need to check a variable against undefined value, there is no need to invent any special method, since JavaScript has a typeof operator, which is simple, fast and cross-platform: if (typeof value === "undefined") { //...
To check undefined in JavaScript, use (===) triple equals operator. In modern browsers, you can compare the variable directly to undefined using the triple equals operator. There are also two more ways to check if the variable is undefined or not in JavaScript, but those ways are not recommended. Those two are the following. If you just want the "right" way to check if a variable is undefined, scroll right to the bottom, where I show you the proper method to do this sort of check. Comparing against undefined. Naturally, the first method you'd think of to test if a variable is undefined would be to compare it against the undefined keyword, like this: https ... In JavaScript, a variable can be either defined or not defined, as well as initialized or uninitialized. typeof myVar === 'undefined' evaluates to true if myVar is not defined, but also defined and uninitialized. That's a quick way to determine if a variable is defined.
The above example demonstrates that accessing: an uninitialized variable number; a non-existing object property movie.year; or a non-existing array element movies[3]; are evaluated to undefined.. The ECMAScript specification defines the type of undefined value:. Undefined type is a type whose sole value is the undefined value.. In this sense, typeof operator returns 'undefined' string for an ... In simple words you can say a null value means no value or absence of a value, and undefined means a variable that has been declared but no yet assigned a value. To check if a variable is undefined or null you can use the equality operator == or strict equality operator === (also called identity operator). If you are interested in knowing whether the variable hasn't been declared or has the value undefined, then use the typeof operator, which is guaranteed to return a string: if (typeof myVar !== 'undefined') Direct comparisons against undefined are troublesome as undefined can be overwritten. window.undefined = "foo"; "foo" == undefined // true
In this post, we will learn how to check for undefined or null variable in JavaScript. If we create one variable and don’t assign any value to it, JavaScript assigns undefined automatically. null is an assignment value, we need to assign a variable null to make it null. If you don’t know the content of a variable in JavaScript, it is always a good idea to check if it is undefined or null. The variable is neither undefined nor null The variable is neither undefined nor null The variable is undefined or null The variable is undefined or null. The typeof operator for undefined value returns undefined. Hence, you can check the undefined value using typeof operator. Also, null values are checked using the === operator. Conclusion. An undefined value in JavaScript is a common occurrence— it means a variable name has been reserved, but currently no value has been assigned to that reference. It is not defined, so ...
The undefined property indicates that a variable has not been assigned a value, or not declared at all. In a JavaScript program, the correct way to check if an object property is undefined is to use the typeof operator. If playback doesn't begin shortly, try restarting your device. Videos you watch may be added to the TV's watch history and influence TV recommendations. To avoid this, cancel and sign in to YouTube on your computer. Check undefined variable. In this post, I will share a basic code in Javascript/jQuery that checks the empty, null, and undefined variables. If you using javascript/jquery usually you need to check if the variable given is not empty, nulled, or undefined before using it to your function. Because if you don't validate your variables it could ...
Variable of Type Undefined or Null in JavaScript. We can declare a variable of the above types according to the requirements. Sometimes in code, we require to check the type of a variable, and based on the result, some further actions are taken. How to check for null, undefined, empty variables in JavaScript where the variable could be anything like array or object, string, number, Boolean? Hi, coder. So, this is one of the most asked questions that how to check null, undefined, empty variables in JavaScript? If my_var is undefined then the condition will execute. If my_var is 0 then the condition will execute. If my_var is " (empty string) then the condition will execute. … This condition will check the exact value of the variable whether it is null or not. Example 2:
The explicit approach, when we are checking if a variable is undefined or null separately, should be applied in this case, and my contribution to the topic (27 non-negative answers for now!) is to use void 0 as both short and safe way to perform check for undefined. In JavaScript, checking if a variable is undefined can be a bit tricky since a null variable can pass a check for undefined if not written properly. As a result, this allows for undefined values to slip through and vice versa. Make sure you use strict equality === to check if a value is equal to undefined. How to check a not-defined variable in JavaScript? Javascript Web Development Front End Technology. To check a variable is 'undefined', you need to check using the following. If the result is "false", it means the variable is not defined. Here, the variable results in "True" −.
There are a few simple methods in JavaScript to check or determine if a variable is undefined or null. Use "null" with the operator "==" You can use the equality operator (==) in JavaScript to check whether a variable is undefined or null. Code explanation: In the code above there are two variables var and var2. First I have used a loose comparison operator to identify if the type of var is equal to undefined and in the second condition, the strict comparison is used to find if the type of var2 is equal to undefined. type of gives the data type of any variable in string form.Click to read more about it. There are 7 falsy values in JavaScript - false, 0, 0n, '', null, undefined and NaN. A nullish value consists of either null or undefined. This post will provide several alternatives to check for nullish values in JavaScript. To check for null variables, you can use a strict equality operator (===) to compare the variable with null.
18/4/2021 · To check an undefined variable in jQuery or JavaScript, we can use one of these two methods. 1. Compare variable type to undefined and 2. Compare variable to undefined. The example to check the undefined variable using typeof. Compare variable type to undefined <script> if(typeof var1 == 'undefined') { alert("var1 is undefined"); } if(typeof var2 === 'undefined') { alert("var2 is undefined"); } </script> Compare variable to undefined . Use the typeof Operator to Check Undefined in JavaScript This operator returns a string that tells about the type of the operand. If the value is not defined, it returns a string undefined. var abc; console.log(typeof abc === 'undefined')
How To Check A Variable Is Undefined In React Native React
Javascript Error Handling Typeerror Null Or Undefined Has
If Not Undefined Javascript Code Example
7 Tips To Handle Undefined In Javascript
How To Check If An Object Has A Property Properly In
Undefined Vs Null Find Out The Top 8 Most Awesome Differences
How To Check Empty Undefined Null String In Javascript
Youvcode Knowledge Shared 2 X Knowledgehow To Determine
Best Way To Check Null Undefined Empty Variables In
Javascript Typeof How To Check The Type Of A Variable Or
Is There A Standard Function To Check For Null Undefined Or
3 Ways To Check If An Object Has A Property In Javascript
How To Check For An Undefined Or Null Variable In Javascript
How To Check If A Variable Is Undefined In Js Dev Community
3 Ways To Set Default Value In Javascript Dev Community
How To Check For Undefined In Javascript By Dr Derek
Is There A Standard Function To Check For Null Undefined Or
Javascript Check If A Variable Exists Undefined Example
How To Check Undefined Variable In Jquery Javascript Devnote
Advance Javascript Understand Undefined In Javascript
Javascript Check If Empty Code Example
Javascript Data Types Grab Complete Knowledge About Data
How To Check If A Javascript Promise Has Been Fulfilled
In Javascript Is Undefined Actually Undefined Dev Community
0 Response to "25 How To Check Undefined Variable In Javascript"
Post a Comment