35 Javascript Split String By Words
JavaScript Split String: Main Tips. JavaScript string.split () method splits a data string into a substrings array and returns the new array. You can specify where the string will be split by choosing a separator. It's also possible to limit the number of substrings to return. We may come across situations where we need to convert string to an array of string in order to access individual words. In Javascript, we use the split () function to breakdown a large string to an array of small strings based on separator if required. The separator is nothing but a delimiter with which we want to split the string.
All The Ways To Extract Text Or Numbers From A String In
I'm looking for an optimal javascript function to split a camelcase string and return an array. I suppose one could loop through the string, check if character is uppercase and start building a new word to add to the array but that seems incredibly wasteful. must be some easy way to do it. This was a fun little morning brain warmer.
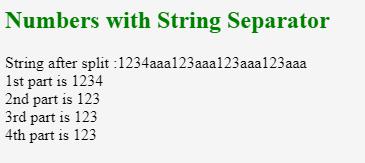
Javascript split string by words. separator: It is used to specifie the character, or the regular expression, to use for splitting the string. If the separator is unspecified then the entire string becomes one single array element. The same also happens when the separator is not present in the string. This is what's called grapheme clusters - where the user perceives it as 1 single unit, but under the hood, it's in fact made up of multiple units. The newer methods spread and Array.from are better equipped to handle these and will split your string by grapheme clusters 👍 # A caveat about Object.assign ⚠️ One thing to note Object.assign is that it doesn't actually produce a pure array. In this simple program, we will use the JavaScript to input a list of keywords from the user. The keywords will be prompted by the browser and the user will enter the keywords, separated by "," character. From the input, we will separate the keywords using the split () method of String object. The split method takes single argument, which is ...
The split method syntax. In order to use an object's method, you need to know and understand the method syntax. The split method syntax can be expressed as follows: string.split ( separator, limit) The separator criterion tells the program how to split the string. It determines on what basis the string will be split. String.prototype.split () The split () method divides a String into an ordered list of substrings, puts these substrings into an array, and returns the array. The division is done by searching for a pattern; where the pattern is provided as the first parameter in the method's call. The split() method separates an original string into an array of substrings, based on a separator string that you pass as input. The original string is not altered by split().. Syntax const splitStr = str.split(separator, limit); separator - a string indicating where each split should occur; limit - a number for the amount of splits to be found; Examples: const str = "Hello.
javascript split query string; how to split a word javascript; convert string to arr js; example of split function js; turn string into an array; slipt in js; split a text to words array javascript; splitting strings in jav; how to split a string in javascript based on delimiter; javascript split words by space; converting a string into an ... The split () method divides a String into an ordered list of substrings, puts these substrings into an array, and returns the array. The division is done by searching for a pattern; where the pattern is provided as the first parameter in the method's call. 28/4/2008 · First make actual separate words: replace( /([a-z])([A-Z])/, "$1 $2"); Then split on the space: split( /\s/);
In JavaScript, split () is a string method that is used to split a string into an array of strings using a specified delimiter. Because the split () method is a method of the String object, it must be invoked through a particular instance of the String class. Note that the result array may have fewer entries than the limit in case the split() reaches the end of the string before the limit. JavaScript split() examples. Let's take some examples of using the split() method. 1) Splitting the strings into words example. The following example uses the split() method to split the string into words: Write a JavaScript function to split a string and convert it into an array of words.
The slice () method extracts parts of a string and returns the extracted parts in a new string. Use the start and end parameters to specify the part of the string you want to extract. The first character has the position 0, the second has position 1, and so on. Tip: Use a negative number to select from the end of the string. 1. Reverse a String With Built-In Functions. For this solution, we will use three methods: the String.prototype.split () method, the Array.prototype.reverse () method and the Array.prototype.join () method. The split () method splits a String object into an array of string by separating the string into sub strings. Split Method in Javascript The Split method is used to split a string into an array of strings and breaking at a specified delimiter string or regular expression.
6/8/2013 · This will split your string based on word count (not character count, so the exact length of each half could be quite different, depending on the placement of long & short words). var s = "This is a string of filler text"; var pieces = s.split(" "), firstHalfLength = Math.round(pieces.length/2), str1 = "", str2 = ""; for (var i = 0; i < firstHalfLength; i++){ str1 += (i!=0?" The split () Method in JavaScript The split () method splits (divides) a string into two or more substrings depending on a splitter (or divider). The splitter can be a single character, another string, or a regular expression. After splitting the string into multiple substrings, the split () method puts them in an array and returns it. Given a statement which contains the string and separators, the task is to split the string into substring. String split () Method: The str.split () function is used to split the given string into array of strings by separating it into substrings using a specified separator provided in the argument.
The split method in JavaScript. The JavaScript split method is used to break a given string into pieces by a specified separator like a period (.), comma, space or a word/letter. The method returns an array of split strings. Syntax of split method. This is how you may use the split method of JS: The split () method divides a string into a list of substrings. It is used to make individual words or values in a string accessible by indexing. split () can separate out a string by any character, such as a white space or a comma. If you're looking to learn more about JavaScript, read our guide on the best tutorials for JavaScript beginners. How can I split these strings in to two parts from the common word? var str= "12344A56789"; Instead of having to write substring multiple times, is there a way to split the string from 4A and get the following results? var first = "1234"; var second = "56789"; NOTE: Each string lengths are different.
JavaScript split ExamplesSeparate parts from a string with the split function. Split on characters, strings and patterns. Split on characters, strings and patterns. dot net perls slice () extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: the start position, and the end position (end not included). This example slices out a portion of a string from position 7 to position 12 (13-1): Remember: JavaScript counts positions from zero. First position is 0. Split String into Words JavaScript. The following example uses the split() method to split the string into Words...
All Languages >> Javascript >> Next.js >> split words of string in array javascript "split words of string in array javascript" Code Answer. javascript slice string from character . javascript by Lokesh003 on Jun 28 2020 Donate Comment . 4 Add a Grepper Answer ... Some words are also delimited by more than just a single space. Let's use string.split () function and pass a single-space string as a separator string. var text = ' Splitting String Into Tokens in JavaScript ' console.log(text.split(' ')) It gives something like: ['', 'Splitting', 'String', '', '', 'Into', '', 'Tokens', 'in', 'JavaScript', ''] How To Index, Split, and Manipulate Strings in JavaScript Development JavaScript. By Tania Rascia. Last Validated on August 24, 2021 Originally Published on July 14, 2017 Introduction. A string is a sequence of one or more characters that may consist of letters, numbers, or symbols. Each character in a JavaScript string can be accessed by an ...
17/4/2020 · Use the split() method of a string instance. It accepts an argument we can use to cut the string when we have a space: const text = "Hello World! Hey, hello!" text.split(" ") The result is an array. In this case, an array with 4 items: [ 'Hello', 'World!', 'Hey,', 'hello!' Definition and Usage The split () method splits a string into an array of substrings, and returns the new array. If an empty string ("") is used as the separator, the string is split between each character. The split () method does not change the original string.
Javascript Split String How Does Split String Work In
String Methods Javascript Count Number Of Words Inside A
3 Ways To Replace All String Occurrences In Javascript
Javascript String Split How To Split String In Javascript
Javascript Split String Function
Convert String To Array Javascript Split Separator Regex
Javascript String Split How To Split String In Javascript
Javascript String Split How To Split String In Javascript
Basics Of Javascript String Split Method By Jakub
Javascript String Split Tutorial
Add A Before Each Word In A String Using Jquery Stack
Java String Split Developer Helps
Uppercase The First Letter Of A String In Javascript Dev
How To Split A String Into An Array In Javascript
Loop Through Words In A String Javascript Iterate Words In
Algodaily Find Duplicate Words Step Two
Javascript Split String How Does Split String Work In
Javascript String Split How To Split String In Javascript
Javascript Split A String Into Equal Lengths By Cliff Hall
How To Split Strings By Spaces Help Uipath Community Forum
Split String Overview Outsystems
Java67 3 Ways To Count Words In Java String Google
Github Jonschlinkert Split String Split A String On A
Regex How To Split String Into Words Questions
Strings In Powershell Replace Compare Concatenate Split
Javascript Find The Longest Word In A String Code Example
You Can Include Delimiters In The Result Of Javascript S
How To Find The Longest Word In A String In Javascript
How To Calculate The Number Of Words In A String Using Jquery
Convert Comma Separated String To Array Using Javascript
How To Split A String Help Uipath Community Forum
0 Response to "35 Javascript Split String By Words"
Post a Comment