26 Add Function To Element Javascript
6/1/2015 · You could also add the function directly to the element using $('.dynamicHtmlForm')[0].validate = function { return true; } // and later... if (!$(this).closest('.dynamicHtmlForm')[0].validate()) Or you could look at extending jQuery properly by … The JavaScript addEventListener() method allows you to set up functions to be called when a specified event happens, such as when a user clicks a button. This tutorial shows you how you can implement addEventListener() in your code. Understanding Events and Event Handlers Events are actions that happen when the
Call A Function With Parameters In Javascript Append Stack
Sometimes, you may want to load a JavaScript file dynamically. To do this, you can use the document.createElement() to create the script element and add it to the document. The following example illustrates how to create a new script element and loads the /lib.js file to the document:
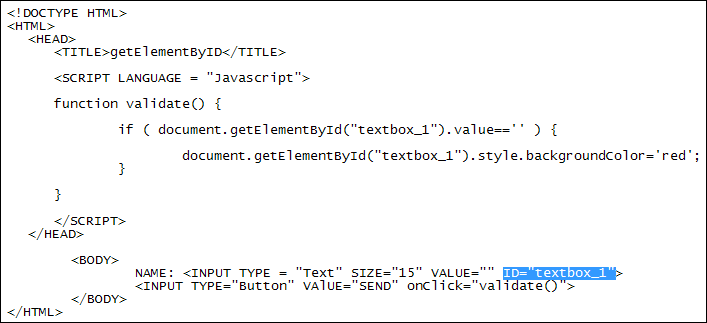
Add function to element javascript. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Oct 03, 2017 - Previously, we looked at how to insert an element before another one with vanilla JavaScript. Today, we’ll learn how to insert an element to the beginning of a set elements inside a shared parent with vanilla JS. We’re going to look at two ways to do this: The traditional way. May 03, 2013 - Simply appending the new list item ... allow me to click on it and run a function, which is often a problem when creating new elements. The link will do nothing, unless we create it and attach an event handler as well. AJAX also has this problem, pulling new information off the server will have no JavaScript readiness ...
push () ¶. The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. The new item (s) will be added only at the end of the Array. You can also add multiple elements to ... There are two ways to append HTML code to a div through JavaScript. Using the innerHTML attribute. Using the insertAdjacentHTML () method. Using the innerHTML attribute: To append using the innerHTML attribute, first select the element (div) where you want to append the code. Then, add the code enclosed as strings using the += operator on ... Set.prototype.add () The add () method appends a new element with a specified value to the end of a Set object.
26/9/2019 · We can use the inbuilt setAttribute() function of JavaScript. Syntax: var elementVar = document.getElementById("element_id"); elementVar.setAttribute("attribute", "value"); So what basically we are doing is initializing the element in JavaScript by getting its id and then using setAttribute() to modify its attribute. 10/5/2007 · posts:1147. votes: 0. I want to add a function onto an element. I know you can add any property by using setAttribute, but not sure about functions... For example.... var div = document.getElementById ( "test"); div.myFunction = function { alert ( "This is my div!"); }; //. Jun 01, 2020 - A quick guide on how selectors affect your codeWhile working on a project, I ran into an issue in my code. I was attempting to define multiple HTML elements into a collection and then change those elements based on some preset conditions. I struggled for roughly four hours of coding
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. How can I use JavaScript to add an HTML element where that blank line is? javascript html dom. Share. Improve this question. Follow edited Aug 6 '10 at 15:36. ... insertBefore is not a global function, it's a method on the HTMLElement prototype. The correct method is parentElement.insertBefore(newElement, childElement). - Andy E. We then use yet another DOM API function called Element.setAttribute() to set a class attribute on our panel with a value of msgBox. This is to make it easier to style the element — if you look at the CSS on the page, you'll see that we are using a .msgBox class selector to style the message box and its contents.
As you can see above, we first defined an event handler function and then use the addEventListener () method to attach it with the element. The addEventListener () accepts up to three parameters. The first parameter is the name of the event you want to listen for, like click, change, mouseover, and so on. The class name attribute can be used by CSS and JavaScript to perform certain tasks for elements with the specified class name. Adding the class name by using JavaScript can be done in many ways. Using .className property: This property is used to add a class name to the selected element. Syntax: element.className += "newClass"; Objects in JavaScript have other characteristics besides properties. They can also have methods. A method is simply a function attached to an object. To see what this means, take a look at this example: //create the critter //from addingMethods.html var critter = new Object(); //add some properties ...
A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2,...) This code creates a new <p> element: const para = document. createElement ( "p" ); To add text to the <p> element, you must create a text node first. This code creates a text node: const node = document. createTextNode ( "This is a new paragraph." ); Then you must append the text node to the <p> element: para. appendChild (node);
How to add JavaScript to html How ... write a function in JavaScript Is JavaScript case sensitive How does JavaScript Work How to debug JavaScript How to Enable JavaScript on Android What is a promise in JavaScript What is hoisting in JavaScript What is Vanilla JavaScript How to add a class to an element using JavaScript ... I have been trying to add onclick event to new elements I added with JavaScript. The problem is when I check document.body.innerHTML I can actually see the onclick=alert('blah') is added to the new element. But when I click that element I don't see the alert box is working. In fact anything related to JavaScript is not working.. JavaScript Learn JavaScript ... Add an HTML element: document.replaceChild(new, old) Replace an HTML element: document.write(text) Write into the HTML output stream: Adding Events Handlers. Method Description; document.getElementById(id).onclick = function(){code} Adding event handler code to an onclick event: Finding HTML Objects. The first ...
tagName A string that specifies the type of element to be created. The nodeName of the created element is initialized with the value of tagName.Don't use qualified names (like "html:a") with this method. When called on an HTML document, createElement() converts tagName to lower case before creating the element. In Firefox, Opera, and Chrome, createElement(null) works like createElement("null"). So one can add directly required code at the end of element by using JavaScript Append () jQuery functions helps to add append functions or contents those are in terms of an HTML string, DOM element, jQuery object or text node etc. 29/9/2012 · We can modify a DOM element and add to its prototype. For example, if we want to add something only to the canvas, we'd do something like this: HTMLCanvasElement.prototype.doSomething = function(arg) { ... }; We can then perform this action on a canvas element: var canvas = document.getElementById('canvasId'); canvas.doSomething(...);
In our previous example, the method called appendChild () was used to append the new element as the last child of the parent element. JavaScript create element action can also be performed with the insertBefore () method, which is used to insert a new element above some selected one. Take a look at the last line of code in the example below ... allow attaching a handler for an event that is defined in an element other than the one adding the handler. ... Which of the following is the correct way to execute a JavaScript when a button element is clicked ? Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
One or more additional DOM elements, text nodes, arrays of elements and text nodes, HTML strings, or jQuery objects to insert at the end of each element in the set of matched elements. ... A function that returns an HTML string, DOM element(s), text node(s), or jQuery object to insert at the ... Need to add some content, or update a certain part of the page using Javascript? There are 2 common ways to create and insert HTML elements in Javascript. By directly changing the inner HTML of an element - document.getElementById ("ID").innerHTML = "<p>Text</p>"; By creating and appending a new element. var para = document.createElement ("p"); The key to ensuring the above code works is to reference the clicked button by means of the this keyword. If you try to use the i variable inside the loop, you'll only have a reference to the last button in the loop, rather than the currently clicked button. It should also be noted that for better memory consumption, you should use a named function instead of the anonymous function, then ...
1a-quick-eg.html. First, we give the HTML element a unique id. Then select it with var element = document.getElementById (ID) in Javascript. Finally, take note that innerHTML can be used in two directions. When we do var contents = element.innerHTML, it will get the current contents of element. May 19, 2020 - Third, for each language, create a new li element with the textContent is assigned to the language. Finally, append li elements to the ul element by using the append() method. 1. Using jQuery - .css () method. In jQuery, you can use the .css () method for setting one or more CSS properties on an element. It works by modifying the value of the style property of the element. The above version of the .css () method takes the property name and value as separate parameters. To add multiple CSS attributes in a single ...
Because of browsers that don't ... instance, the example above works in Firefox and Opera, but add Element.extend(my_div) after creating the element to make the script really solid. You can use the dollar function as a shortcut like in the following example:... The example of using the .className property is given as follows.. Example - Adding the class name. In this example, we are using the .className property for adding the "para" class to the paragraph element having id "p1".We are applying the CSS to the corresponding paragraph using the class name "para".. We have to click the given HTML button "Add Class" to see the effect. Summary: in this tutorial, you will learn how to dynamically add options to and remove options from a select box in JavaScript. The HTMLSelectElement type represents the <select> element. It has the add() method that dynamically adds an option to the <select> element and the remove() method that removes an option from the <select> element:
May 04, 2010 - During extension, methods/properties can be added to objects directly, or to their prototypes (but only in environments that have proper support for it). The most commonly extended objects are probably DOM elements (those that implement Element interface), popularized by Javascript libraries ... There are a couple of different ways to add elements to an array in Javascript: ARRAY.push ("ELEMENT") will append to the end of the array. ARRAY.unshift ("ELEMENT") will append to the start of the array. ARRAY [ARRAY.length] = "ELEMENT" acts just like push, and will append to the end. ARRAYA.concat (ARRAYB) will join two arrays together. The createElement () method creates an Element Node with the specified name. Tip: After the element is created, use the element.appendChild () or element.insertBefore () method to insert it to the document.
function: Required. Specifies the function to run when the event occurs. When the event occurs, an event object is passed to the function as the first parameter. The type of the event object depends on the specified event. For example, the "click" event belongs to the MouseEvent object. useCapture: Optional. Jun 03, 2019 - There are several methods for adding new elements to a JavaScript array. push(): The push() method will add an element to the end of an array, while its twin function, the pop() method, will remove an element from the end of the array. If you need to add an element or multiple elements to the ...
How To Find Even Numbers In An Array Using Javascript
Jquery Append Element And Replace Selected Row Stack Overflow
Basic Javascript Removing Elements From An Array Dev Community
Powerful Js Reduce Function You Can Use It To Solve Any Data
How To Add Content To Html Body Using Js Stack Overflow
Define And Run Javascript Code Outsystems
Run Javascript In The Console Chrome Developers
Inserting Html Elements With Javascript Code Example
Append Multiple Child Elements To A Parent Element Javascript Dom Tutorial
The 10 Most Common Mistakes Javascript Developers Make Toptal
Insert A New Key In Array Javascript Code Example
Javascript Array Find How To Find Element In Javascript
Javascript Basic Compute The Sum Of Two Parts And Store Into
How To Add An Object To An Array In Javascript Geeksforgeeks
How To Append Data To Lt Div Gt Element Using Javascript
Js Reduce Arrow Function Code Example
Js Includes Vs Some Javascript Array Is A Powerful
Gtmtips Add Html Elements To The Page Programmatically
Using Getelementbyid In Javascript
How To Append Html Code To A Div Using Javascript
Run Javascript In The Console Chrome Developers
Method To Determine Javascript Functions Calls Made In A
0 Response to "26 Add Function To Element Javascript"
Post a Comment