31 Javascript Replace String At Index
In this article we'll cover various methods that work with regexps in-depth. str.match(regexp) The method str.match(regexp) finds matches for regexp in the string str.. It has 3 modes: If the regexp doesn't have flag g, then it returns the first match as an array with capturing groups and properties index (position of the match), input (input string, equals str): Javascript queries related to "remove character at index from string javascript" javascript remove part of string by index; delete caractere js at index
Python Remove The Characters Which Have Odd Index Values Of
Nov 28, 2019 - To replace a character from a string there are popular methods available, the two most popular methods we are going to describe in this article. The first method is by using the substr() method. And in the second method, we will convert the string to an array and replace the character at the index. ...
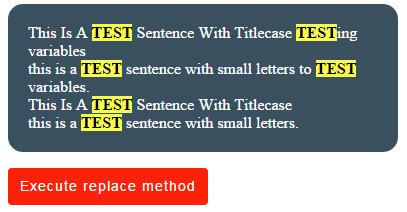
Javascript replace string at index. 3. replaceAll () method. 4. Key takeaway. 1. Splitting and joining an array. If you google how to "replace all string occurrences in JavaScript", most likely the first approach you'd find is to use an intermediate array. Here's how it works: Split the string into pieces by the search string: const pieces = string.split(search); Code language: JavaScript (javascript) The JavaScript String replace () method returns a new string with a substring (substr) replaced by a new one (newSubstr). Note that the replace () method doesn't change the original string. It returns a new string. The second parameter can be a function. This function will be invoked when the match is found (or for every match foundm if using a global regex /g), with a number of arguments:. the string that matches the pattern; an integer that specifies the position within the string where the match occurred
Definition and Usage The replace () method searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced. Note: If you are replacing a value (and not a regular expression), only the first instance of the value will be replaced. Dec 04, 2020 - String.prototype.replaceAt=function(index, char) { var a = this.split(""); a[index] = char; return a.join(""); } ... let hello:string = "Hello World"; console.log(hello.replaceAt(2, "!!")); // Should display He!!o World ... // How to create string with multiple spaces in JavaScript var a = ... Definition and Usage. The indexOf () method returns the position of the first occurrence of a specified value in a string. indexOf () returns -1 if the value is not found. indexOf () is case sensitive. Tip: Also look at the lastIndexOf () method.
Jun 24, 2020 - Get code examples like "string replace with index js" instantly right from your google search results with the Grepper Chrome Extension. Jun 24, 2020 - String.prototype.replaceAt=function(index, char) { var a = this.split(""); a[index] = char; return a.join(""); } ... // How to create string with multiple spaces in JavaScript var a = 'something' + '\xa0\xa0\xa0\xa0\xa0\xa0\xa0' + 'something'; Watch a video course JavaScript - The Complete Guide (Beginner + Advanced) ... The first method is split and join which converts the string into an array and replaces the character at the specified index. The string is converted into an array by using split() with the separator as a blank character ...
First character is at index 0. If start is positive and greater than, or equal, to the length of the string, substr() returns an empty string. If start is negative, substr() uses it as a character index from the end of the string. If start is negative or larger than the length of the string, start is set to 0: length: Optional. The arguments of substring() represent the starting and ending indexes, while the arguments of substr() represent the starting index and the number of characters to include in the returned string. Furthermore, substr() is considered a legacy feature in ECMAScript and could be removed from future versions, so it is best to avoid using it if ... Jun 24, 2020 - String.prototype.replaceAt=function(index, char) { var a = this.split(""); a[index] = char; return a.join(""); } ... // How to create string with multiple spaces in JavaScript var a = 'something' + '\xa0\xa0\xa0\xa0\xa0\xa0\xa0' + 'something';
Normally JavaScript's String replace () function only replaces the first instance it finds in a string: app.js. const myMessage = 'this is the sentence to end all sentences'; const newMessage = myMessage.replace('sentence', 'message'); console.log(newMessage); Copy. In this example, only the first instance of sentence was replaced. Extracts a part of a string and returns a new string: split() Splits a string into an array of substrings: substr() Extracts the characters from a string, beginning at a specified start position, and through the specified number of character: substring() Extracts the characters from a string, between two specified indices: toLowerCase() Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The.replace method is used on strings in JavaScript to replace parts of string with characters.
The JavaScript string replace () method is used to replace a part of a given string with a new substring. This method searches for specified regular expression in a given string and then replace it if the match occurs. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Each character in a JavaScript string can be accessed by an index number, and all strings have methods and properties available to them. In this tutorial, we will learn the difference between string primitives and the String object, ... We can search a string for a value, and replace it with a new value using the replace() method. The first ...
A higher-level approach is to use the substring () method of the String class. It will create a new String by concatenating the substring of the original String before the index with the new character and substring of the original String after the index: Jul 20, 2021 - The String object's charAt() method returns a new string consisting of the single UTF-16 code unit located at the specified offset into the string. searchValue. The string value to search for. If no string is explicitly provided, searchValue will be coerced to "undefined", and this value will be searched for in str. So, for example: 'undefined'.indexOf() will return 0, as undefined is found at position 0 in the string undefined.'undefine'.indexOf() however will return -1, as undefined is not found in the string undefine.
15/9/2009 · String.prototype.replaceAt = function(index, replacement) { return this.substr(0, index) + replacement + this.substr(index + replacement.length); } And use it like this: var hello = "Hello World"; alert(hello.replaceAt(2, "!!")); Apr 28, 2021 - This post will discuss how to replace a character at the specified index in JavaScript... The idea is to split the string into two substrings before and after the specified position, and then concat them together with the specified character in the middle. Using your idea I did this to catch and replace hidden chars in text. sampleData= '+ check if this has hidden chars'; // it does not if it did the + would be replaced by ERROR or something else. function ReplaceAt (string, index, replace) {. return string.substring (0, index) + replace + string.substring (index + 1); }
The array type in JavaScript provides us with splice() method that helps us in order to replace the items of an existing array by removing and inserting new elements at the required/desired index. Approach: There is no predefined method in String Class to replace a specific character in a String, as of now. However, this can be achieved indirectly by constructing a new String with 2 different substrings, one from beginning till the specific index - 1, the new character at the specific index and the other from the index + 1 till the end. setCharAt() is a function that can be used to change a single character in a string in JavaScript
From the "related questions" bar, this old answer seems to be applicable to your case. Replacing a single character (in my referenced question) is not very different from replacing a string. How do I replace a character at a particular index in JavaScript? slice() extracts the text from one string and returns a new string. Changes to the text in one string do not affect the other string. slice() extracts up to but not including endIndex.str.slice(1, 4) extracts the second character through the fourth character (characters indexed 1, 2, and 3). As an example, str.slice(2, -1) extracts the third character through the second to last character in ... Array.some will check if at least one value in the array matches the condition in our callback function and Array.every will check that ALL of the elements in the Array match that condition.. Replacing an element of an Array at a specific index Now that we know how to check if the Array includes a specific element, let's say we want to replace that element with something else.
String.prototype.lastIndexOf () The lastIndexOf () method returns the index within the calling String object of the last occurrence of the specified value, searching backwards from fromIndex. Returns -1 if the value is not found. 18/1/2018 · C# program to remove characters starting at a particular index in StringBuilder; Replace an element at a specified index of the Vector in Java; C# program to replace n-th character from a given index in a string; How to replace a character in a MySQL table? Place an H1 element and its text at a particular index in jQuery In JavaScript, replace () is a string method that is used to replace occurrences of a specified string or regular expression with a replacement string. Because the replace () method is a method of the String object, it must be invoked through a particular instance of the String class.
How to replace a character at a particular index in JavaScript , The second part of the string can be extracted by using the starting index parameter as 'index + 1', which denotes the part of the string after the To replace a character at a particular index in JavaScript, you can try to run ... Jun 30, 2021 - This article will discuss how to replace at a specific index in a javascript string using different methods and example illustrations. Javascript does not have an in-built method to replace a particular index. Therefore, one has to create a custom function to fulfill the purpose. May 09, 2012 - If you have a general ASP.NET question on a topic that's not covered by one of the other more specific forums - ask it here · Latest: Jun 25, 2021 05:41 AM
4/7/2020 · The string doesn’t have a direct method to replace a character at a particular index, like in array we have splice method. So we need to take help of other string methods to achieve this functionality. using substring method; using replace method; using character array; Using substring method. As we know the substring method helps to get part of the string by using the startIndex and endIndex. Let’s create functionality for replacing a character from string by given index String.prototype.replace () The replace () method returns a new string with some or all matches of a pattern replaced by a replacement. The pattern can be a string or a RegExp, and the replacement can be a string or a function to be called for each match. If pattern is a string, only the first occurrence will be replaced.
How To Remove Commas From Array In Javascript
Javascript Replace Method To Change Strings With 6 Demos
Manipulating Characters In A String The Java Tutorials
Ansible Replace Line In File Ansible Replace Examples
Strings In Powershell Replace Compare Concatenate Split
Javascript Array Indexof And Lastindexof Locating An Element
How To Replace All Occurrences Of A String In Javascript
How To Manipulate A Part Of String Split Trim Substring
Javascript Split How To Split A String Into An Array In Js
4 Ways To Remove Character From String In Javascript
How To Replace All Dots In A String Using Javascript
Sql Substring Function Overview
Python Program To Replace Single Or Multiple Character
Javascript Cut String If Too Long Code Example
Replace De String Multiplo Em Javascript Jose Junior
Overview Of The Sql Replace Function
Insert Character Into String Before End Javascript Code Example
Jquery Replace String Guide To Implementation Of Jquery
How To Replace A Character At A Particular Index In
Javascript Basic Remove A Character At The Specified
How To Use Strings In Javascript With Selenium Webdriver
Overview Of The Sql Replace Function
Essential Javascript String Methods By Trey Huffine Level
Jquery Replace String Guide To Implementation Of Jquery
Javascript Array Splice Delete Insert And Replace
14 Most Useful Javascript String Methods By Abdullah Imran
Discussion Of How To Search And Replace String Across
How To Replace An Element In Array In Java Code Example
Java String Replace Replacefirst And Replaceall Method
0 Response to "31 Javascript Replace String At Index"
Post a Comment