32 Javascript Map Return Object
Write a function called mapObject that takes in an object and a callback and returns an object. Your function should invoke the callback for every property in the object and use what it returns as the new value for the return object's properties. javascript map function for objects inside an array Map.prototype.values () The values () method returns a new Iterator object that contains the values for each element in the Map object in insertion order.
Javascript Array To Object How To Convert An Array Into An
Just like.map (),.reduce () also runs a callback for each element of an array. What's different here is that reduce passes the result of this callback (the accumulator) from one array element to...
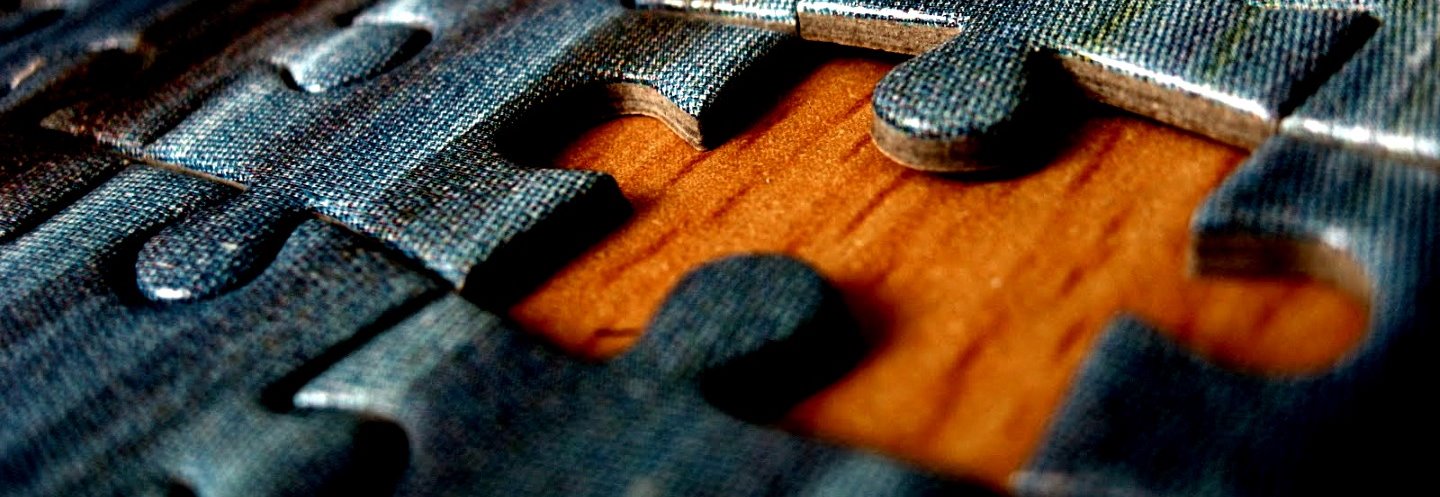
Javascript map return object. Pandas error: “IndexError: iloc ... target object” ... Symfony\Component\Debug\Exception\FatalThrowableError Type error: ReflectionFunction::__construct() expects parameter 1 to be string, array given ... ‘yield’ expression implicitly results in an ‘any’ type because its containing generator lacks a return-type ... The main thing to notice is the use of Promise.all(), which resolves when all its promises are resolved.. list.map() returns a list of promises, so in result we'll get the value when everything we ran is resolved. Remember, we must wrap any code that calls await in an async function.. See the promises article for more on promises, and the async/await guide. .map()isn't the usual way to modify properties an existing array of objects. You cando that, but it's not what.map()is normally used for. Instead, it's a way to create a newarray. A.map()callback returns a value, and those returned values get pushed into a new array, which.map()finally returns.
JavaScript exercises, practice and solution: Write a JavaScript function to calculate the sum of values in an array. JavaScript: function and object with properties. ... JS map return object, You're very close already, you just need to return the new object that you want. The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value. @KhalilRavanna I think you've misread the code here - this answer isn't using map correctly because it isn't doing a return - it's abusing map as if it were a forEach call. If he actually did return myObject[value] * 2 then the result would be an array containing the original values doubled, instead of an object containing the original keys with doubled values, the latter clearly being what ...
.map () accepts a callback function as one of its arguments, and an important parameter of that function is the current value of the item being processed by the function. This is a required parameter. With this parameter, you can modify each item in an array and create a new function. To get the keys of a Map object, you use the keys () method. The keys () returns a new iterator object that contains the keys of elements in the map. The following example displays the username of the users in the userRoles map object. for (let user of userRoles.keys ()) { console.log (user.name); } // John Doe // Lily Bush // Peter Drucker This pattern is great, as it allows us to easily add some local variables above the return statement, a common practice for us.. But what if we don't need to declare any local variables and just want to return an object?. We've heard of an arrow function's implicit return feature - just delete the return statement and the curly {} braces right?
Returning Object Literals from Arrow Functions in JavaScript June 9, 2015. Arrow functions are one of the great new features of ECMAScript 2015. They allow you to define functions using a concise syntax that doesn't require the function keyword.. Using the classical function expression syntax, you could define a function to square a given integer as follows: Let us solve a problem using map method. We have a JSON object say "orders" with lot of keys like name, description, date, status. We need an array of orders whose status is delivered. 15/12/2017 · If you want to alter the original objects, then a simple Array#forEach will do: rockets.forEach(function(rocket) { rocket.launches += 10; }); If you want to keep the original objects unaltered, then use Array#map and copy the objects using Object#assign:
Jan 29, 2020 - Join Stack Overflow to learn, share knowledge, and build your career · Find centralized, trusted content and collaborate around the technologies you use most callbackFn is invoked with three arguments: the value of the element, the index of the element, and the array object being mapped.. If a thisArg parameter is provided, it will be used as callback's this value. Otherwise, the value undefined will be used as its this value. The this value ultimately observable by callbackFn is determined according to the usual rules for determining the this seen ... Example 1: map add key to object in array javascript const newArr = [ {name: 'eve'}, {name: 'john'}, {name: 'jane'} ].map(v => ({...v, isActive: true})) Example 2: r
Sử dụng map một cách độc lập. Ví dụ sau sẽ minh họa cách dùng map lên một String một cách độc lập để trả về một mảng các mã byte trong bảng ASCII đại diện cho từng ký tự của chuỗi. var map = Array. prototype. map; var a = map.call('Hello World', function(x) { return x.charCodeAt(0); }); Dec 22, 2019 - Make a program that filters a list of strings and returns a list with only your friends name in it.javascript ... Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript Map an object's keys to an array. ... Map the original array to a new one; each element is squared. ... Map the original array to a new one, removing numbers less than 50 by returning null and subtracting 45 from the rest.
In JavaScript, most functions are both callable and instantiable: they have both a and [[Construct]] internal methods. As callable objects, you can use parentheses to call them, optionally passing some arguments. As a result of the call, the function can return a value. var player = makeGamePlayer("John Smith", 15, 3); 20/8/2020 · The syntax of JavaScript map method is as below : let finalArray = arr.map(callback( currentValue[, index[, array]]), arg) Following are the meanings of these parameters : callback: This is the callback method. It is called for each of the array arr elements. The returned value of this method is added to the final array finalArray. Mar 31, 2021 - And that's all you need to know about the Array.map() method. Most often, you will only use the element argument inside the callback function while ignoring the rest. That's what I usually do in my daily projects :) ... You may also be interested in other JavaScript tutorials that I've written, including how to sum an array of objects ...
Map.prototype.get () The get () method returns a specified element from a Map object. If the value that is associated to the provided key is an object, then you will get a reference to that object and any change made to that object will effectively modify it inside the Map object. Specifically, the Array Map Method operates on an array to run a transformation on every element of the array. It does so through use of a callback function which is called for each item of the array. After running the callback function on each item, the Map Method returns the transformed array, leaving the original array unchanged.
Mar 25, 2018 - This works, until you want to return an object. You see, we use brackets {} to create objects, and if we'll try to return an object via one-liner: const numbers = [2, 3, 4]; const squares = numbers.map(n => { result: n**2 }); // Result: // [undefined, undefined, undefined] JavaScript Map Objects. A Map holds key-value pairs where the keys can be any datatype. A Map remembers the original insertion order of the keys. A Map has a property that represents the size of the map. Using the set () with chaining. Since the set () method returns back the same Map object, you can chain the method call like below: myMap.set('bar', 'foo') .set(1, 'foobar') .set(2, 'baz'); Copy to Clipboard.
Write a function called mapObject that takes in an object and a callback and returns an object. Your function should invoke the callback for every property in the object and use what it returns as the new value for the return object's properties. For plain objects, the following methods are available: Object.keys (obj) - returns an array of keys. Object.values (obj) - returns an array of values. Object.entries (obj) - returns an array of [key, value] pairs. Please note the distinctions (compared to map for example): The first difference is that we have to call Object.keys (obj ... Example 1: javascript map array const myArray = ['Sam', 'Alice', 'Nick', 'Matt']; // Appends text to each element of the array const newArray = myArray.map(name => {
Definition and Usage. The map() method creates a new array with the results of calling a function for every array element.. The map() method calls the provided function once for each element in an array, in order.. map() does not execute the function for empty elements. map() does not change the original array. Code language: JSON / JSON with Comments (json) How it works. First, define a function that calculates the area of a circle. Then, pass the circleArea function to the map() method. The map() method will call the circleArea function on each element of the circles array and return a new array with the elements that have been transformed.; To make it shorter, you can pass in the map() method an ... Object.entries(colorsHex) returns an array of key-value pairs extracted from the object. Access of keys-values of a map is more comfortable because the map is iterable. Anywhere an iterable is accepted, like for() loop or spread operator, use the map directly. colorsHexMap keys-values are iterated directly by for() loop:
A lot of people are mentioning that the previous methods do not return a new object, but rather operate on the object itself. For that matter I wanted to add another solution that returns a new object and leaves the original object as it is: Return subset of JSON Object using Javascript map() function Tags: arrays , javascript , json My question is if there is a simple way to return a subset of JSON object that will contain all ‘columns’ rather than specifying individually which ‘columns’ to return. Nov 25, 2020 - And the standard iteration for ... as the map. ... A Set is a special type collection – “set of values” (without keys), where each value may occur only once. ... new Set(iterable) – creates the set, and if an iterable object is provided (usually an array), copies values from it into the set. set.add(value) – adds a value, returns the set ...
The forEach method executes the provided callback once for each key of the map which actually exist. It is not invoked for keys which have been deleted. However, it is executed for values which are present but have the value undefined. callback is invoked with three arguments:. the entry's value; the entry's key; the Map object being traversed; If a thisArg parameter is provided to forEach, it ...
Introduction To Javascript Source Maps Html5 Rocks
What Is The Difference Between Javascript Map And Object
Map In Javascript Geeksforgeeks
Write Javascript Loops Using Map Filter Reduce And Find
Javascript Filter Return Object Not Array
Javascript Map Object Examples Of Javascript Map Object
Javascript Map Return Undefined Object Despite Object Exist
Exploring Sets And Maps In Javascript Scotch Io
When To Use Map Instead Of Plain Javascript Object
How Can I Create A Storytelling Map With Carto Js Carto
Getting Javascript Properties For Object Maps By Index Or
Strongloop Type Hinting In Javascript
Javascript Map Data Structure With Examples Dot Net Tutorials
How To Use The Ruby Map Method With Examples Rubyguides
Ben Lesh On Twitter Kentcdodds Maxfmckay I Am Not
Javascript Tracking Key Value Pairs Using Hashmaps By
Using Javascript S Map Function The Map Function Simply
Map In Javascript Geeksforgeeks
How To Find Unique Values By Property In An Array Of Objects
Maps In Javascript Using Map Vs Using Object
How To Access Object Array Values In Javascript Stack Overflow
Convert Object Keys According To Table Map Object Code
Deep Nested Array Of Objects Not Rendering Stack Overflow
Javascript Es6 Map Return Certain Object Only Stack Overflow
Everything About Javascript Objects By Deepak Gupta
Javascript Map Array Array Map Method Mdn
Javascript Array Map How To Map Array In Javascript
0 Response to "32 Javascript Map Return Object"
Post a Comment