34 Add Class To Array Javascript
Jun 02, 2017 - Description It would be great if these examples: var class1 = 'class-one'; var class2 = 'class-two'; $('.element').addClass(class1 + ' &#... Array class in JavaScript: class Array {. constructor () {. this.length = 0; this.data = {}; } } In the above example, create a class Array which contains two properties i.e. length and data, where length will store the length of an array and data is an object which is used to store elements. Function in Array: There are many functions in array ...
Everything About Javascript Objects By Deepak Gupta
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
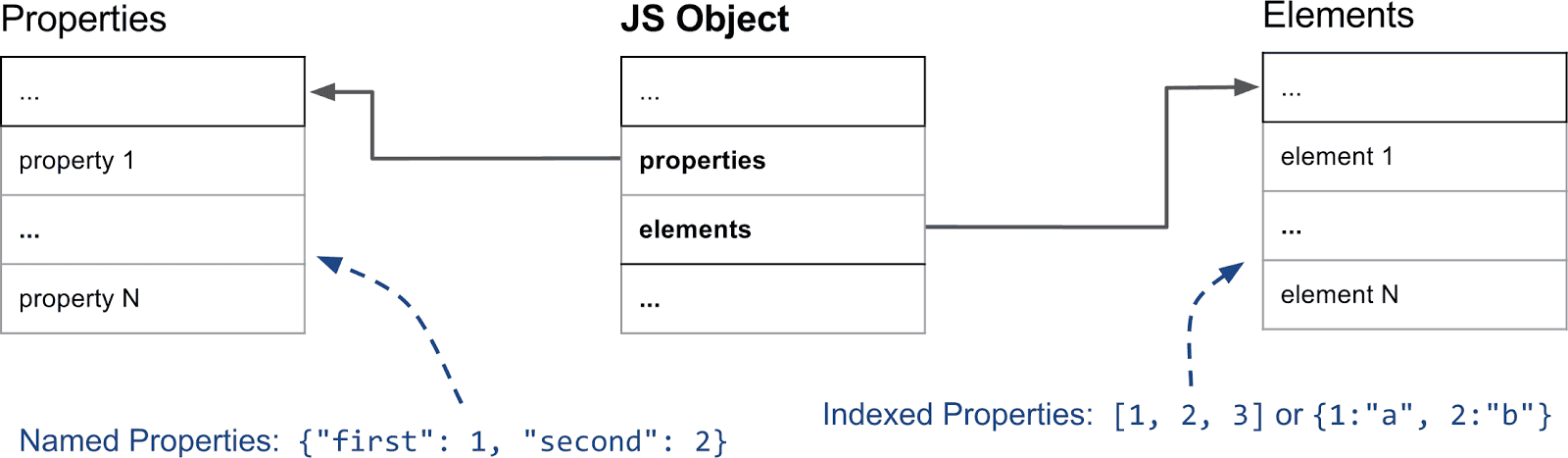
Add class to array javascript. A JavaScript array is initialized with the given elements, except in the case where a single argument is passed to the Array constructor and that argument is a number (see the arrayLength parameter below). So multidimensional arrays in JavaScript is known as arrays inside another array. We need to put some arrays inside an array, then the total thing is working like a multidimensional array. The array, in which the other arrays are going to insert, that array is use as the multidimensional array in our code. JavaScript is more than just strings and numbers. JavaScript lets you create objects and arrays. Objects are similar to classes. The objects are given a name, and then you define the object's properties and property values. An array is an object also, except arrays work with a specific number of values that you can iterate through.
5 Way to Append Item to Array in JavaScript. Here are 5 ways to add an item to the end of an array. push, splice, and length will mutate the original array. Whereas concat and spread will not and will instead return a new array. Which is the best depends on your use case 👍. Also classes are usually title-cased. Plus as a side note, while it's real that you can change consts properties while maintaining the reference, so you can push objects in an array that's declared as const, it really doesn't add up as self-documenting code, so, if you are going to modify this array, just declare it with "let" from the start. The javaScript push() method is used to add new elements to the end of an array. Note: javaScript push() array method changes the length of the given array. Here you will learn the following: How to add the single item of the array? How to add the multiple items of the array? How to push array into array in javaScript?
Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array #2 unshift - Insert an element at the beginning of the array #3 spread operator - Adding elements to an array using the new ES6 spread operator Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The getElementsByClassName method of Document interface returns an array-like object of all child elements which have all of the given class name(s).
3/4/2015 · // Select the element var div = document.querySelector('.select_me'); // Create an array with the classes to add var classArray = ['give_me', 'more_classes']; // Apply the new classes. var cl = div.classList; cl.add('just_a_test', 'okay'); cl.add.apply(cl, classArray); Aug 26, 2009 - A function returning one or more space-separated class names or an array of class names to be added to the existing class name(s). Receives the index position of the element in the set and the existing class name(s) as arguments. Within the function, this refers to the current element in the set. I am new one in object oriented javascript and trying to define a class, that have an array as a data member. this data member of class storing objects of an other class as an array. it will be more clear by this example
Arrays were an add-on afterthought to javascript; for that reason you can find various ways of using them listed on programming websites, including references to an Array object. The 'Array' object is however basically obsolete now; in this tutorial we'll look at current best practices. We can add a special static getter Symbol.species to the class. If it exists, it should return the constructor that JavaScript will use internally to create new entities in map, filter and so on. If we'd like built-in methods like map or filter to return regular arrays, we can return Array in Symbol.species, like here: The Push Method The first and probably the most common JavaScript array method you will encounter is push (). The push () method is used for adding an element to the end of an array. Let's say you have an array of elements, each element being a string representing a task you need to accomplish.
11/6/2019 · There are 3 popular methods which can be used to insert or add an object to an array. push() splice() unshift() Method 1: push() method of Array. The push() method is used to add one or multiple elements to the end of an array. It returns the new length of the array formed. An object can be inserted by passing the object as a parameter to this method. Jan 13, 2019 - Browse other questions tagged javascript arrays oop or ask your own question. ... Congratulations greg-449, on reaching 100,000 close vote reviews! ... If I have the fighter's Extra Attack feature and the warlock's Thirsting Blade eldritch invocation, how many attacks can I make? ... To subscribe ... The Array() constructor is used to create Array objects.
"js add class to array of elements" Code Answer javascript add class to element javascript by Grepper on Jul 24 2019 Donate Comment To add a class to an element, you use the classList property of the element. Suppose you have an element as follows: < div > Item </ div > Code language: HTML, XML (xml) ... JavaScript Array. JavaScript Arrays; JavaScript Array Length; Stack; Queue; Manipulate Elements: splice() Sort Elements: sort() Last Updated : 06 Mar, 2019. There are several methods for adding new elements to a JavaScript array. push (): The push () method will add an element to the end of an array, while its twin function, the pop () method, will remove an element from the end of the array. If you need to add an element or multiple elements to the end of an array, the ...
Jun 05, 2014 - I would like to add classes to an element in order from an array. I have three different classes, and I am not sure how to loop back to the beginning of the array to start over when I get to the en... Notice that this method will not mutate your original array, but will return a new one. Describing Arrays¶ JavaScript arrays are a super-handy means of storing multiple values in a single variable. In other words, an array is a unique variable that can hold more than a value at the same time. Arrays are considered similar to objects. The main ... Apr 03, 2015 - So far, I've figured out how to select elements and add a single class to them. What I'm having trouble with, is using an array to add multiple classes to an element.
Remember, JavaScript Array indexes are 0-based: they start at 0, not 1. This means that the length property will be one more than the highest index stored in the array: let cats = [] cats [30] = ['Dusty'] console.log( cats. length) Copy to Clipboard. You can also assign to the length property. Please turn on JavaScript in your browser and refresh the page to view its content. In this article, we are discussing how to add a class to an element using JavaScript. In JavaScript, there are some approaches to add a class to an element. We can use the.className property or the.add () method to add a class name to the particular element. Now, let's discuss the approaches to add a class to an element.
38 Add Class To Array Javascript Written By Roger B Welker. Friday, August 13, 2021 Add Comment Edit. Add class to array javascript. Creating Dynamic Object Array From Multiple Arrays. Js Delete An Element Of The Array. Zepto Js A Jquery Compatible Mobile Javascript Framework In 2k. 1 week ago - The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. ... Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. Neither the length of a JavaScript array nor the types ... The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. The new item (s) will be added only at the end of the Array.
divtst specifies the element where you want to add a new class name. This is followed by the classList property. Then add method is used and the desired class name is provided in the parenthesis. The examples below show how to specify element and add CSS class in different elements. Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. JavaScript is more than just strings and numbers. JavaScript lets you create objects and arrays. Objects are similar to classes. The objects are given a name, and then you define the object's properties and property values. An array is an object also, except arrays work with a specific number ...
In the above program, the splice () method is used to add an object to an array. The splice () method adds and/or removes an item. The first argument represents the index where you want to insert an item. The second argument represents the number of items to be removed (here, 0). The third argument represents the element that you want to add to ... I would like to add classes to an element in order from an array. I have three different classes, and I am not sure how to loop back to the beginning of the array to start over when I get to the end of the array of classes. Here is what I have so far: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Arrays In Javascript How To Create Arrays In Javascript
How To Add Remove And Toggle Css Classes In Javascript
Dynamically Add And Remove Html Elements Using Jquery
Ios Native Modules React Native
How To Modify Attributes Classes And Styles In The Dom
A Vue Js 2 Grid Tables Components Codespots Com
Arraylist In Java With Example Programs Collections Framework
C Arraylist Tutorial With Examples
How To Create Array In Javascript Code Example
5 Way To Append Item To Array In Javascript Samanthaming Com
Keeping Your Es6 Javascript Code Simple Dev Community
How To Manage React State With Arrays
Jquery Addclass Not Working On Elements In An Array Stack
How To Modify Attributes Classes And Styles In The Dom
Python Array Module How To Create And Import Array In
Addclass Jquery Api Documentation
Array Of Values From An Existing Array Using Map Function In
Get The Closest Element By Selector
Hacks For Creating Javascript Arrays
Javascript Typed Arrays Javascript Mdn
Making Sense Of Es6 Class Confusion Toptal
Extending Multiple Classes In Javascript By Michael P Smith
Hacks For Creating Javascript Arrays
Python Array Module How To Create And Import Array In
Numpy Array Object Exercises Practice Solution W3resource
Challenge 7 5 Custom Push Method For Arrays Solution
Add Class To Tr Element That Wraps Around A Paragraph Bundle
Javascript Array Push How To Add Element In Array
How To Add An Object To An Array In Javascript Geeksforgeeks
Javascript Array Of Objects Tutorial How To Create Update
How To Dynamically Add A Class Name In Vue By Michael
0 Response to "34 Add Class To Array Javascript"
Post a Comment