35 Define Class Property Javascript
To define a class in JavaScript, you can use directly the clss declaration with the name of the class. class className { constructor (params) { //properties } //methods } - Another way, assign the class to a variable: let varClass = class { constructor (params) { //properties } //methods } The diference is that "varClass" is a variable, so its ... Classes (MDN) TypeScript offers full support for the class keyword introduced in ES2015. As with other JavaScript language features, TypeScript adds type annotations and other syntax to allow you to express relationships between classes and other types.
Private Properties In Javascript Curiosity Driven
36 Define Class Property Javascript. Written By Roger B Welker Friday, May 14, 2021 Add Comment. Edit.
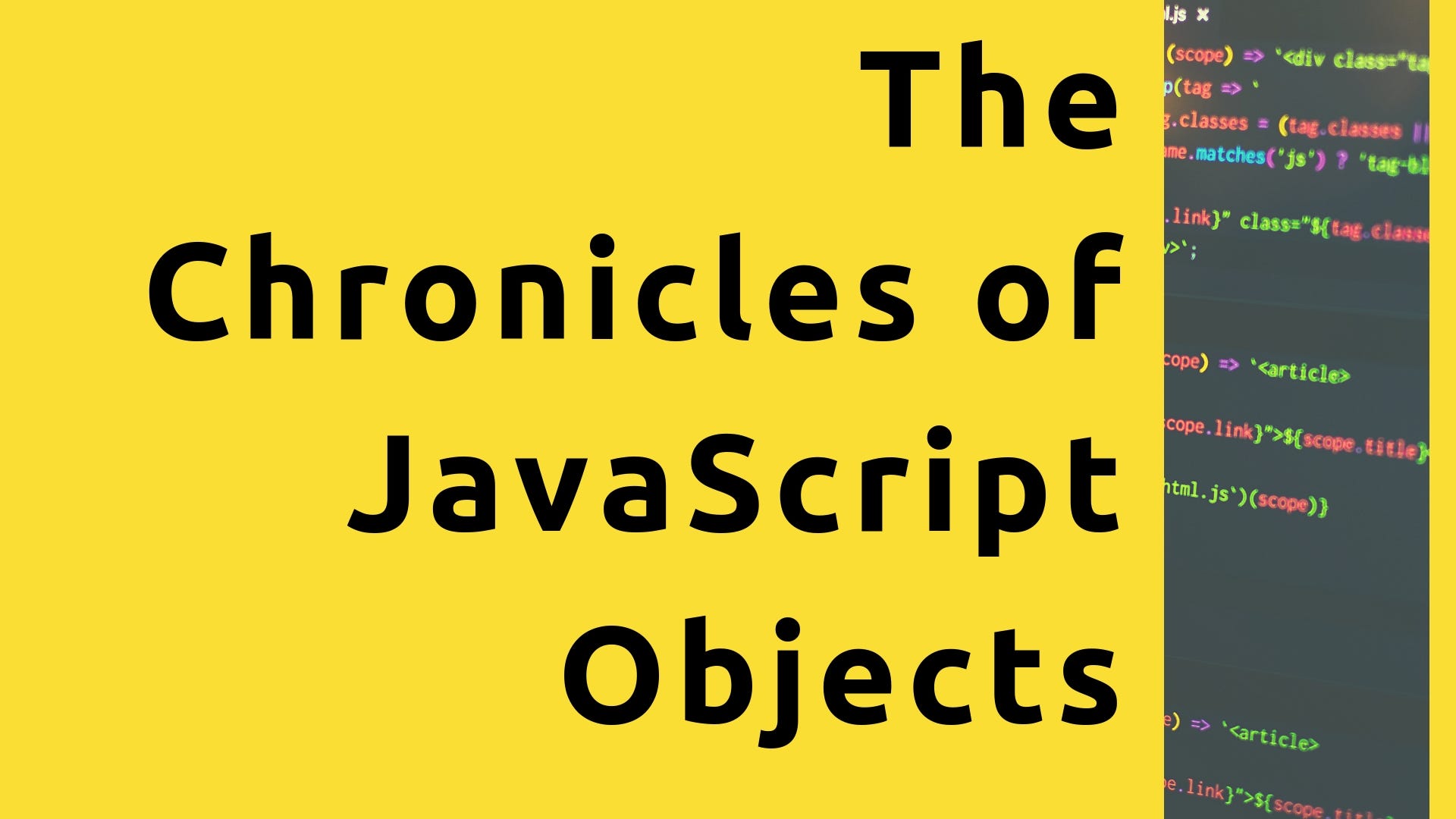
Define class property javascript. Second, you can't call these methods, and access these properties, on instances of the class in which they are defined. JavaScript developers usually use static methods and properties something like utility functions and utility properties. For example, you can use static method to create a method that will help you compare two instances of ... For a class definition, this object will be the prototype object of the class, i.e. Foo.prototype. When you access a property via super.foo or super["foo"] , it is equivalent to [[HomeObject ... Nov 06, 2019 - For example, if we have an Animal ... the Bird class can just inherit the common properties in the Animal class. They don’t have to be defined in the Bird class again. We can write the following to do inheritance in JavaScript:...
May 04, 2018 - Understanding prototypical inheritance is paramount to being an effective JavaScript developer. Being familiar with classes is extremely helpful, as popular JavaScript libraries such as React make frequent use of the class syntax. When you want to add some data to JavaScript class you can do so through class properties. These properties are by default always public. This also means that they are publicly accessible and modifiable. The same also applies to class methods. Dec 13, 2019 - JavaScript uses prototypal inheritance: every object inherits properties and methods from its prototype object. The traditional class as the blueprint to create objects, used in languages like Java or Swift, does not exist in JavaScript. The prototypal inheritance deals only with objects.
The class has two initial properties: "name" and "year". A JavaScript class is not an object. It is a template for JavaScript objects. ... The example above uses the Car class to create two Car objects. The constructor method is called automatically when a new object is created. ... If you do not define ... A JavaScript class is a blueprint for creating objects. A class encapsulates data and functions that manipulate data. Unlike other programming languages such as Java and C#, JavaScript classes are syntactic sugar over the prototypal inheritance. In other words, ES6 classes are just special functions. A class body can only contain methods, ... data properties is generally considered an anti-pattern, so this just enforces a best practice. 15.2.2.1 constructor, static methods, prototype methods # Let’s examine three kinds of methods that you often find in class definitions...
Nov 07, 2018 - Let’s see how the new Class Fields proposal improves the code above First, we can take our state variable out of the constructor and define it directly as a property (or “field”) on the class. Nov 25, 2020 - Object.defineProperty provides a handy way to add properties to JavaScript objects. It’s been around for a while, but with the introduction of class syntax in ES6, it’s not immediately obvious how to use it to add properties to a class, as opposed to an instantiated object. Class basic syntax In object-oriented programming, a class is an extensible program-code-template for creating objects, providing initial values for state (member variables) and implementations of behavior (member functions or methods). In practice, we often need to create many objects of the same kind, like users, or goods or whatever.
JavaScript Properties. Properties are the values associated with a JavaScript object. A JavaScript object is a collection of unordered properties. Properties can usually be changed, added, and deleted, but some are read only. Dec 11, 2020 - The static keyword defines a static method or property for a class. Static members (properties and methods) are called without instantiating their class and cannot be called through a class instance. Static methods are often used to create utility functions for an application, whereas static ... May 28, 2020 - The constructor property returns the constructor function for all JavaScript variables. ... how to Create a class called Person which defines the generic data and functionality of a human with multiple interests in javascript
Sep 01, 2020 - This would have thrown a runtime error on the last line, but that’s a severe consequence for simply attempting to set a property. JavaScript is purposely forgiving and ES5 permitted property modification on any object. Although clunky, the # notation is invalid outside a class definition. ... Definition and Usage The className property sets or returns the class name of an element (the value of an element's class attribute). Tip: A similar property to className is the classList property. Object.defineProperty () The static method Object.defineProperty () defines a new property directly on an object, or modifies an existing property on an object, and returns the object.
In the ancient time, there was a limitation in JavaScript: a reserved word like "class" could not be an object property. That limitation does not exist now, but at that time it was impossible to have a "class" property, like elem.class. The HTML class attribute specifies one or more class names for an element. Classes are used by CSS and JavaScript to select and access specific elements. The class attribute can be used on any HTML element. The class name is case sensitive. Different HTML elements can point to the same class name. Sep 05, 2018 - 2. Class methods are non-enumerable. In javascript each property of an object has enumerable flag, which defines its availability for some operations to be performed on that property. A class sets this flag to false for all the methods defined on its prototype.
JavaScript ES6: Classes. Objects in programming languages provide us with an easy way to model data. Let's say we have an object called user. The user object has properties: values that contain ... Apr 02, 2021 - They are labeled by the word static in class declaration. Static properties are used when we’d like to store class-level data, also not bound to an instance. Define Class in JavaScript. JavaScript ECMAScript 5, does not have class type. So it does not support full object oriented programming concept as other languages like Java or C#. However, you can create a function in such a way so that it will act as a class. The following example demonstrates how a function can be used like a class in JavaScript.
The class has two initial properties: "name" and "year". A JavaScript class is not an object. It is a template for JavaScript objects. @ Dream_Cap if you define data in the constructor, then each instance has its own data property. I want to 'store' it in the prototype so each instance has only the reference to the same data property stored in the class prototype - Paweł Feb 12 '18 at 1:10 In JavaScript, a setter can be used to execute a function whenever a specified property is attempted to be changed. Setters are most often used in conjunction with getters to create a type of pseudo-property. It is not possible to simultaneously have a setter on a property that holds an actual value. Note the following when working with the set ...
This is a pretty basic car class with a single private member and two accessor methods. As you can see, the drive method takes a number and increments the mileage property of the car instance. And like any good method, it checks to make sure the input is valid before applying it, otherwise you could end up with bad data. While using the get keyword and Object.defineProperty () have similar results, there is a subtle difference between the two when used on classes. When using get the property will be defined on the instance's prototype, while using Object.defineProperty () the property will be defined on the instance it is applied to. The class syntax does not introduce the new object-oriented inheritance model to JavaScript. Define a class in Javascript. One way to define the class is by using the class declaration. If we want to declare a class, you use the class keyword with the name of the class ("Employee" here). See the below code.
JavaScript supports what is called the shorthand property names. It allows us to create an object using just the name of the variable. It will create a property with the same name. The next object literal is equivalent to the previous one. 1 week ago - Please note that all keys in the square bracket notation are converted to string unless they're Symbols, since JavaScript object property names (keys) can only be strings or Symbols (at some point, private names will also be added as the class fields proposal progresses, but you won't use them ... The JavaScript className property accepts one value. It is called class and it is used to define a class of an element. However, when you want to JavaScript change class, remember that multiple classes have to be separated by spaces to be assigned (for example, class1 class2 ).
Feb 10, 2015 - ES Class Property Declarations. GitHub Gist: instantly share code, notes, and snippets. May 28, 2020 - Both static and instance public fields are writable, enumerable, and configurable properties. As such, unlike their private counterparts, they participate in prototype inheritance. Jul 20, 2021 - JavaScript follows a similar model, but does not have a class definition separate from the constructor. Instead, you define a constructor function to create objects with a particular initial set of properties and values. Any JavaScript function can be used as a constructor.
JavaScript. Const. The const keyword was introduced in ES6 (2015). Variables defined with const cannot be Redeclared. Variables defined with const cannot be Reassigned. Variables defined with const have Block Scope. get - a function without arguments, that works when a property is read, set - a function with one argument, that is called when the property is set, enumerable - same as for data properties, configurable - same as for data properties. For instance, to create an accessor fullName with defineProperty, we can pass a descriptor with get and ... Nov 07, 2018 - Let’s see how the new Class Fields proposal improves the code above First, we can take our state variable out of the constructor and define it directly as a property (or “field”) on the class.
Jul 07, 2021 - Gain a deeper understanding of how TypeScript's type system enhances classes via interfaces, access modifiers, decorators and other features to unlock advanced features and encourage cleaner code. Class fields are public by default, but private class members can be created by using a hash # prefix. The privacy encapsulation of these class features is enforced by JavaScript itself. When you define a class property and assign an arrow function as its value, Babel does a little trick to bind that function to the correct instance of the class and give you the right this value. It does this by setting descriptor.initializer to a function that returns the function you wrote, with the correct this value in its scope.
Seven Ways Of Creating Objects In Javascript For C
Making Sense Of Es6 Class Confusion Toptal
How To Modify Attributes Classes And Styles In The Dom
What Is Super In Javascript Css Tricks
Github 3dbeb41841bfbfcc24d55143816cf7f1 Js Objects And Json
Tools Qa How To Use Javascript Classes Class Constructor
Javascript Classes Under The Hood By Majid Tajawal Medium
Typescript Documentation Overview
Can T Access Object Property Even Though It Shows Up In A
Details Of The Object Model Javascript Mdn
Object In Javascript Top Properties Methods
Define Props On A Vue Class With Vue Property Decorator
Javascript Classes Under The Hood By Majid Tajawal Medium
Javascript Es2015 Classes And Prototype Inheritance Part 1
Abstract Classes In Javascript What Are Abstract Classes
Understanding Classes And Objects
How To Modify Attributes Classes And Styles In The Dom
Javascript Classes Under The Hood By Majid Tajawal Medium
How To Add A Class To An Element Using Javascript Javatpoint
Javascript S New Private Class Fields And How To Use Them
The Chronicles Of Javascript Objects By Arfat Salman Bits
C Class Object Examples C Objects Properties And Methods
The Ultimate Guide To Javascript Prototypal Inheritance
0 Response to "35 Define Class Property Javascript"
Post a Comment