32 How To Make A Javascript Game
Create Conway's Game of Life in JavaScript . Learn how to create Conway's Game of Life on the HTML5 canvas with JavaScript. Implement the game rules and check which cells will live or die each generation, to create your own simulation of life! myScore = new component ("30px", "Consolas", "black", 280, 40, "text"); myGameArea.start(); } var myGameArea = {. canvas : document.createElement("canvas"), start : function() {. this.canvas.width = 480; this.canvas.height = 270; this.context = this.canvas.getContext("2d");
10 Amazing Javascript Games In Under 13kb Of Code Tutorialzine
A crucial part of learning how to make a JavaScript game is understanding that our game will exist in different states. There will be two principle states involved: selection and results. During the selection state, players will have the opportunity to select a move, and the AI's choice will be unknown, or "deciding".
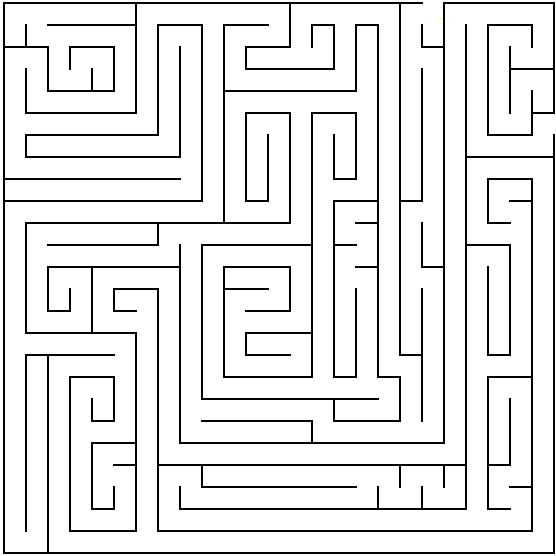
How to make a javascript game. 1 week ago - It is also recommended for those interested in software-related fields of study and development to learn JavaScript inside and out to better your skills and make learning further things a breeze. Can JavaScript be used to make games? Yes, JavaScript has been the basis for many games over the years. Jan 11, 2018 - Hello everyone, today I’ll be writing about how to make a simple multiplayer online game. By the end of this tutorial you’ll have a functional server where players can connect and drive around… Add JavaScript code blocks inside your game. GDevelop supports adding JavaScript code blocks at any point in the events of your game. It's a good way to implement a complex algorithm or reuse functions from open-source libraries. Events and code blocks can seamlessly be mixed together - so you can implement parts or even the totality of your ...
Learn how to create a platformer game using vanilla JavaScript. First, learn to organize the code using the Model, View, Controller (MVC) strategy and the pr... Create The Tetris Game Using JavaScript. In general, to create a JavaScript game, you'll need two things, the first is the HTML5 canvas, and the second is JavaScript. At anytime you can go below, and WATCH THE TUTORIAL. First things first, this is what you need to know about the Tetris game. The game board: the game is played on a board, that ... Nov 27, 2019 - Bursts of code to power through your day. Web Development articles, tutorials, and news.
Jul 20, 2021 - HTML5 is HTML in it’s latest version, ... endless possibilities combining HTML, CSS and JavaScript. Having HTML along with all these super powers that go beyond making a simple website allows us to make, among other things, games.... Jan 22, 2020 - Make amazing games with JavaScript. A must-read for JavaScript lovers ... I have just launched DavisonPro.dev. A standalone blog where I write about Javascript, Web development and software development. ... I’ve been using JavaScript for some few years to develop Web and Mobile apps, and ... How to create a slingshot shooting game with matter.js - A JavaScript 2D physics engine library.Source Code: https://redstapler.co/matter-js-tutorial-game-de...
To make the game more enjoyable for the player, we can also add a score that increases when the snake eats food. Create a new variable score and set it to 0 after the snake declaration. let score = 0;Next add a new div with an id "score" before the canvas. We can use this to display the score. 2/9/2016 · Second, write down all the things you need to program to actually make the game. For me it was: an inventory system; an item generator; a player stat system; a saving system; Third, start making your game by solving these problems one by one. Need help actually creating the game? It’s much easier to break your game down into small tasks. QICI Engine. Based on Phaser, this free JavaScript library provides a web-based suite to make HTML5 games. As its predecessor, Qici uses Pixi's WebGL and Canvas rendering for desktop and mobile web browsers. The engine contains three parts: QICI Core - a game engine library; QICI Widget - a UI library to create applications; and QICI Editor ...
Once the game engine is initialized, it will do the animation via the main () function.Create a file called engine.js and inside it create the Engine object. This object is an anonymous function. It calls itself and passes in this which is the global context. var Engine = (function (global) { }) (this); JavaScript and HTML5 Canvas game tutorial code. This page shows you the code you need to make your own game using JavaScript and the HTML5 canvas. The game involves a player (hero) catching an enemy (monster) as many times as possible in a 30 second time limit. This version of the game has been modified from the tutorial at Lost Decade Games ... As my experience grew and I began to understand the underlying concepts behind what makes a game it started to come back around again, but instead of using traditional languages I've been having a lot of fun experimenting with video game development with JavaScript.
To begin coding the game, create a new folder in your documents. Use your favorite text editor to open that folder, then create three new files and name them: index.html, style.css, and script.js. It's possible to do everything in one file with HTML5, but it's more organized to keep everything separate. 1024 JS Game. 1024 js game is powered by HTML5 and JavaScript, it is a 3D puzzle game with a colourful user interface. To play the game, you have to move the cube to the endpoint through the tile grid while also avoiding to fall into the holes in less than 1024 moves to beat the high score. Demo Download. Create a Javascript Game. Learn how to create a web game using Phaser 3, a popular javascript game engine. Draw a map using an editor, implement the player, make the player move, apply physics, collisions, and implement the enemies. Purchase includes access to 30+ courses, 100+ premium tutorials, 120+ hours of videos, source files and ...
Desktop and Mobile HTML5 game framework. A fast, free and fun open source framework for Canvas and WebGL powered browser games. In today's creative coding tutorial we will learn JavaScript by building games. I will teach you how to make a game with vanilla JS and HTML canvas element a... Tips for creating games with JavaScript.Talk given by Sean Hornsby at the JavaScriptLA meetup.🐦 Sean on twitter: https://twitter /mushiwulf🔗 Check out t...
In this step-by-step tutorial we create a simple MDN Breakout game written entirely in pure JavaScript and rendered on HTML5 <canvas>. Every step has editable, live samples available to play with so you can see what the intermediate stages should look like. You will learn the basics of using the <canvas> element to implement fundamental game ... Jul 25, 2015 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers. Simple Hangman Game In Javascript - Free Code Download. By W.S. Toh / Tips & Tutorials - Javascript / August 18, 2021 August 18, 2021. Welcome to a tutorial on how to create a Hangman game with Javascript. So you are interested in learning how to create a game with Javascript, or maybe just forced to do so in a school project. 😆 Well ...
Nov 27, 2019 - Naturally, with a programming language this versatile, questions arise about its ability to create games. After all, we’ve seen many different programming languages adjust to different industries. ... JavaScript can be used to make games using a variety of platforms and tools. The game_control.htm and acceleration.htm examples demonstrate how easy it is to get started creating your own games in JavaScript. To make your games even more realistic, consider adding gravity and friction. Gravity is what makes bodies thrown into the air slow down, stop, and then fall. To put it simply, gravity is the force of attraction. Here, I used if else statements to create a range depending on number of moves that will give some of the stars a style of visibility: collapse. 5. The Timer. When the player starts a game, a displayed timer should also start. Once the player wins the game, the timer stops. We can create a timer using JS with the code below.
In this JavaScript tutorial you will learn how to create a game using JavaScript. We will be creating a RPG/Battle Arena style game which is to help you unde... May 01, 2019 - And i will walk you through making an awesome game. I have just launched DavisonPro.dev. A standalone blog where I write about Javascript, Web development and software development. ... I’ve been using JavaScript for some few years to develop Web and Mobile apps, and recently i have been ... Beginner JavaScript Game Tutorial For Professional Use. I have seen many tutorials for creating HTML5 JavaScript games, and while most do a fine job introducing the base level technologies, most also introduce bad practices that would become big problems if you actually wanted to make a real game. Writing a game engine from scratch is a good ...
The <canvas> element offers all the functionality you need for making games. Use JavaScript to draw, write, insert images, and more, onto the <canvas>..getContext ("2d") The <canvas> element has a built-in object, called the getContext ("2d") object, with methods and properties for drawing. Create a new file, index.html, and add it to the directory you created for the game. We will set up the canvas, the two containers for the chat boxes, and the color swatch. Make sure that for your external files, you include PubNub's JavaScript SDK and ChatEngine JavaScript SDK. That's it for the html file! Yes, apart from making our websites more attractive, beautiful, we can also use JavaScript to create several kinds of games. So let's see how we can create a game using HTML and JavaScript. To create the game, we will be going to use the HTML Canvas, so before going further, we need to understand the term HTML Canvas. HTML Canvas <Canvas> is one of the many types of HTML elements.
Jan 23, 2018 - In this story, we will start making a little shoot’em up game with PixiJS, a really simple and cool Javascript library. What we are going to do exactly is to make a spaceship able to move and shoot, enemy waves coming through and a beautiful animated background with moving clouds. Jul 02, 2019 - It is an annual coding competition where super talented JavaScript developers show off their games made with only 13kB of code or less. We've chosen some of our favorite games from last year to share with you. Enjoy them as a tiny gaming break from work or as a source of coding inspiration! In general, to create a JavaScript game, you'll need two things, the first is the HTML5 canvas, and the second is JavaScript. You first go and create the canvas element, inside your HTML file : <canvas id="snake" width="200" height="200"></canvas> view raw code5.js hosted with ❤ by GitHub
In other words, this is javascript coding game. When I was learning web development, always I asked my instructor how can I create games in javascript. Now I am able to create very small programs in javascript. I am still learning, & And as much as I know, I share with you all. Because I believe sharing knowledge is the best way to increase ... Create a Reflex Game using JavaScript. 23, Apr 20. Building a Dice Game using JavaScript. 10, May 20. Design Dragon's World Game using HTML CSS and JavaScript. 14, Dec 20. Corona Fighter Game using JavaScript. 14, Dec 20. Crack-The-Code Game using JavaScript. 31, Dec 20. Pig Game Design using JavaScript. Jul 25, 2020 - The best way to learn any programming language is through hands-on projects. The Snake Game is a simple game you can make using the basics of JavaScript and HTML. Snake is a classic video game from the late 70s. The basic goal is to navigate a snake and eat as many apples as possible without ...
Gs Code The Simplest Ways To Make The Best Of Javascript
How To Make A Basic Web Game With No Plugins Using Only Html5
Butterfly Javascript Game By Bug7a
How To Make A Simple Html5 Canvas Game Lost Dec Evered
Creating A Simple Windows 8 Game With Javascript Game Basics
How To Make A Simple Html5 Game With Enchant Js
Foundation Game Design With Html5 And Javascript Springerlink
Make Your Own Game Coderdojo Scholastic Kids
Build A Maths Game Using Html Css Amp Javascript Development
Javascript Game Development Tips On How To Learn Javascript
Game Dev Tricks And Tips Creating A Checker Board In Css
How To Build A Game With Html Css And Javascript
10 Amazing Javascript Games In Under 13kb Of Code Tutorialzine
Js13kgames Tutorial How To Make A Text Game With Html5
Javascript Game Development Tips On How To Learn Javascript
Javascript Tutorial Make A Quiz Game Designers Hub
Top 5 Best Practices For Building Html5 Games In Action
Learn To Create Games With Jquery With Jquery Game
Learn How To Code Javascript By Playing A Game Ana Rute
Microbit Programming How To Make A Catching Apple Game By
Javascript Game Foundations A Web Server Code Incomplete
Html5 Games Workshop Make A Platformer Game With Javascript
Create An Html5 And Javascript Maze Game With A Timer
How To Add Keyboard Controls To Your Html5 Game
Javascript Snake Game Tutorial Build A Simple Interactive Game
Using Javascript To Make Your Games With Gdevelop
Build A Extreme 3d Car Driving Racing Game In Browser Using
Do It Yourself Tutorials Vanilla Javascript Gamedev
2d Breakout Game Using Pure Javascript Game Development Mdn
Six Ways To Level Up Your Javascript
0 Response to "32 How To Make A Javascript Game"
Post a Comment