32 Enter Javascript In Html
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... Trigger a button click on keyboard "enter" with JavaScript. Trigger a Button Click on Enter. Press the "Enter" key inside the input field to trigger the button: Button. What you'd want to do is check whether the event's key is the enter key: In your html, add the event argument <input type="text" onkeypress="clickPress(event)"> And in your handler, add an event parameter. function clickPress(event) { if (event.keyCode == 13) { // do something } }
Building A Vanilla Javascript Todo App From Start To Finish
To include our JavaScript file in the HTML document, we have to use the script tag <script type = "text/javascript" src = "function.js"> and in the "src" attribute we have to provide the path to our JavaScript file where it is stored.
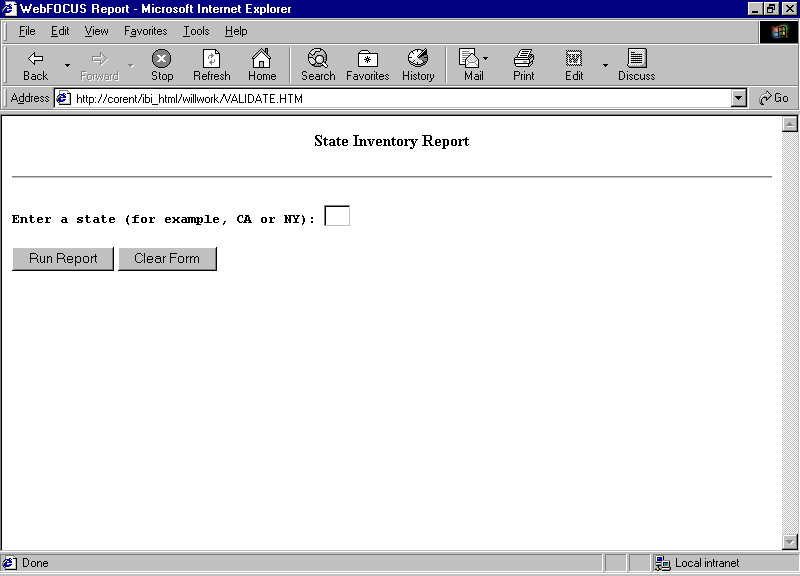
Enter javascript in html. Trigger Button Click on Enter Key Press using JavaScript. HTML example code. Get the element of the input field. Execute the keyup function with addEventListener when the user releases a key. If keyCode is 13, trigger button element with click () event and popup alert box. Lastly, we can directly code Javascript into the HTML elements themselves. This is usually done to handle certain events and user actions, for example: onclick - When the user clicks on the element. onhover - As the mouse cursor hovers over the element. JavaScript Submit textbox on pressing ENTER? The ENTER has the key code 13. We will use the event.keycode to check whether ENTER is pressed or not. Following is the code −.
In my HTML page, I had a textbox for user to input keyword for searching. When they click the search button, the JavaScript function will generate a URL and run in new window. The JavaScript function work properly when the user clicks the search button by mouse, but there is no response when the user presses the ENTER key. 10/4/2021 · Another way to insert JavaScript into your HTML files is by using an external file. This is the most commonly used method to insert JavaScript files into HTML. Let’s have a look at an example. Assuming this is how files are organized in my project, where I have the index.html file and the main.js file, all in the same project folder. The prompt () method lets you open a client-side window and take input from a user. For instance, maybe you want the user to enter a first and last name. Normally, you can use an HTML form for user input, but you might need to get the information before sending the form back to your server for processing.
How to use focus () and blur () method in Javascript with Example. Examples of Good Javascript Examples. How to validate checkbox using Javascript and HTML with Example. Examples of Good Javascript Examples. Base64 encode and decode using btoa () and atob () methods in javascript with example. Possible duplicate of Enter key press event in JavaScript - Bae Mar 23 '16 at 21:17 All the solutions are valid, but keep in mind you should use e.key or e.code, as e.which and e.keyCode are both deprecated. The HTML <script> Tag The HTML <script> tag is used to define a client-side script (JavaScript). The <script> element either contains script statements, or it points to an external script file through the src attribute. Common uses for JavaScript are image manipulation, form validation, and dynamic changes of content.
The HTML DOM allows JavaScript to change the content of HTML elements. Changing HTML Content. The easiest way to modify the content of an HTML element is by using the innerHTML property. To change the content of an HTML element, use this syntax: document.getElementById(id).innerHTML = new HTML. How to trigger click event on pressing enter key using jQuery? JavaScript Trigger a button on ENTER key. How to press ENTER inside an edit box in Selenium? Key press in (Ctrl+A) Selenium WebDriver. Typing Enter/Return key in Selenium. Detect the ENTER key in a text input field with JavaScript. Input Type Hidden. The <input type="hidden"> defines a hidden input field (not visible to a user).. A hidden field let web developers include data that cannot be seen or modified by users when a form is submitted. A hidden field often stores what database record that needs to be updated when the form is submitted.
The first way to add JavaScript to HTML is a direct one. You can do so by using the <script></script> tag that should encompass all the JS code you write. JS code can be added: between the <head> tags Detect the ENTER key in a text input field with JavaScript. You can use the keyCode 13 for ENTER key. Let's first create the input −. Now, let's use the on () with keyCode to detect the ENTER key. Following is the complete code −. To trigger a button click on keyboard enter with JavaScript, the code is as follows −Example Live Demo<!DOCTYPE html> Trigger Button Clic ...
Example press enter key programmatically in JavaScript HTML example code. Trigger an enter keypress event on my input without actually pressing the enter key, more just click on button . JavaScript is the default scripting language in HTML. JavaScript Functions and Events A JavaScript function is a block of JavaScript code, that can be executed when "called" for. For example, a function can be called when an event occurs, like when the user clicks a button. Examples of using JavaScript to access and manipulate HTML input objects. Button Object. Disable a button Find the name of a button Find the type of a button Find the value of a button Find the text displayed on a button Find the id of the form a button belongs to. Form Object.
How to trigger HTML button after hitting enter button in textbox using JavaScript ? Last Updated : 23 Apr, 2020 Given an HTML document containing text area and the task is to trigger the button when the user hit Enter button. We can do it by using "keyup", "keydown", or "keypress" event listener on textbox depending on the need. Browse other questions tagged javascript html dom-events keyboard-events enter or ask your own question. The Overflow Blog Podcast 369: Passwords are dead! To add the JavaScript code into the HTML pages, we can use the <script>.....</script> tag of the HTML that wrap around JavaScript code inside the HTML program. Users can also define JavaScript code in the <body> tag (or we can say body section) or <head> tag because it completely depends on the structure of the web page that the users use.
Enter key press event in JavaScript : Sometimes we need to trigger some action based upon the key events such as key pess, key down etc and some specific case like trigger action on enter press. For this type of events we use key code to identify the key press, using key code you can perform the action as per your requirement. You should not place Javascript code in your HTML, since you're giving those input a class ("search"), there is no reason to do this. A better solution would be to do something like this : $('.search').on('keydown', function (evt) { if(evt.keyCode == 13) The idea is to bind an event handler to the keyup JavaScript event using jQuery's.keyup (handler) method and use that handler to check for the Enter key. To determine the specific key which was pressed, you can use the event.which property. Now trigger the click event of the submit button when the Enter key is detected.
Disable enter key on an input field in JavaScript | Example code Posted June 21, 2021 June 22, 2021 by Rohit Use the key event to textarea where it should not be possible to input line breaks or submit by pressing the enter key. onblur - When a user leaves an input field onchange - When a user changes the content of an input field onchange - When a user selects a dropdown value onfocus - When an input field gets focus onselect - When input text is selected onsubmit - When a user clicks the submit button onreset - When a user clicks the reset button onkeydown - When a user is pressing/holding down a key onkeypress ... 24/4/2010 · To do so, insert a <script language="javascript"> tag in the head. This tells the text editor that you'd like to use JavaScript language to write your HTML JavaScript "program." In this example, we will greet the user using an alert. Add the script tag the HTML head of your own website to add JavaScript.
3/2/2016 · For a comprehensive review of Javascript, explore our series, How To Code in Javascript. Inserting Javascript into HTML using the <script> tag Here’s how to insert an external JavaScript file or some inline JavaScript in your HTML documents. By default, HTML 5 input field has attribute type="number" that is used to get input in numeric format. Now forcing input field type="text" to accept numeric values only by using Javascript or jQuery. You can also set type="tel" attribute in the input field that will popup numeric keyboard on mobile devices.
Prompt Method Getting Input From User In Javascript Hindi
How To Build International Phone Number Input In Html And
Print Star Pattren Program In Javascript T4tutorials Com
Form Validation Lt Javascript The Art Of Web
Working With Javascript Across Web Files Digitalocean
Add Edit And Delete Data In An Html Table Using Javascript
How To Insert Javascript Html Code Into Multi Line Sharepoint
Using An External Javascript File
How To Merge Html Css And Javascript Into Your Rails App
Hide And Show Element On Enter Key Press
Inserting Html Into A Div Stack Overflow
Default Text Label In Textbox Using Javascript Jquery
Write An Html Document And Javascript Code To Create Chegg Com
The Best Position To Load Javascript File In Html Document
Javascript Regular Expression How To Create Amp Write Them In
Html Form And Javascript Onclick Event
How To Insert Html Element At Certain Point Using Js Jquery
Run Snippets Of Javascript Chrome Developers
Get Html Tag Values With Javascript
Input Type Number Gt Html Hypertext Markup Language Mdn
Javascript Interview Questions
How To Get Values From Html Input Array Using Javascript
Making Decisions With Javascript Web Design Amp Development
How To Build International Phone Number Input In Html And
How To Create Login Form With Javascript Validation In Html
Events In Javascript Part Three
Workarounds Manipulating A Survey At Runtime Using
Validating A Form With Javascript
Tags Input Field Made With Html Css And Javascript Web Code
1 Writing Your First Javascript Program Javascript
0 Response to "32 Enter Javascript In Html"
Post a Comment