33 For I In Range Javascript
Syntax. The syntax of 'for..in' loop is −. for (variablename in object) { statement or block to execute } In each iteration, one property from object is assigned to variablename and this loop continues till all the properties of the object are exhausted. Underscore.js | _.range () It is used to print the list of elements from the start given as a parameter to the end also a parameter. The start and step parameters are optional. The default value of start is 0 and that of step is 1. In the list formed the start is inclusive and the stop is exclusive. The step parameter can be either positive or ...
Javascript Fundamental Es6 Syntax Initialize An Array
May 17, 2020 - You'll notice that the array produced ... number. range(10, 20) includes 10, but does not include 20. This is done intentionally, to match the behaviour of JavaScript methods like slice. ... Iterating from a start value to an end value, with a given step, is exactly the problem that for loops were ...
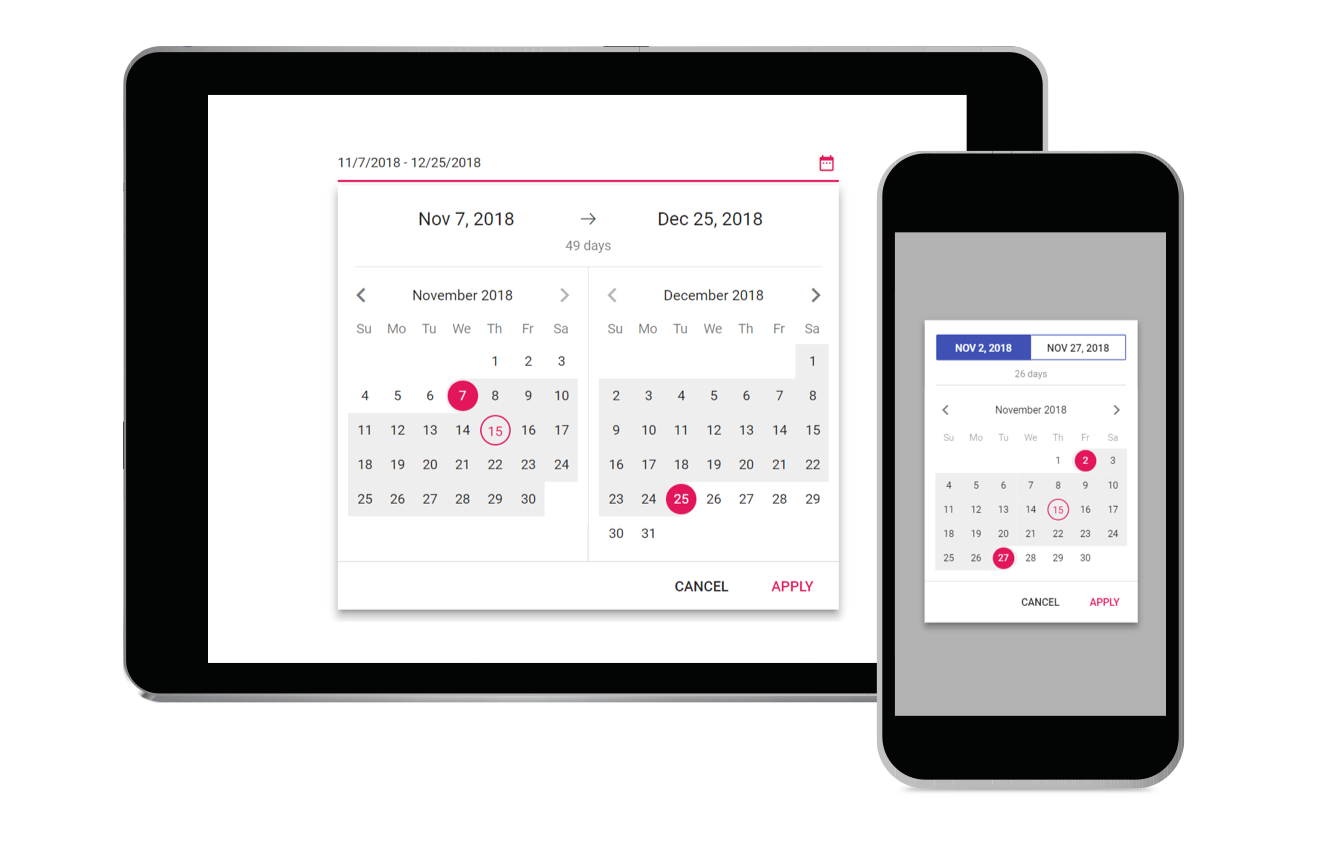
For i in range javascript. For loop with range. In the previous lessons we dealt with sequential programs and conditions. Often the program needs to repeat some block several times. That's where the loops come in handy. There are for and while loop operators in Python, in this lesson we cover for. for loop iterates over any sequence. May 20, 2020 - Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. ... Write a javascript program to find roots of quadratic equation. ... Use parseInt() in the convertToInteger function so it converts the input string ... Iterables in JavaScript. Before looking at the loops, you should know what an iterable in JavaScript is. An iterable is a JavaScript object returning a function that creates an iterator for its Symbol.iterator property.. Common iterables are arrays, typed arrays, maps, sets, and array-like objects (e.g., NodeLists).Strings are iterables as well, you can loop over each character.
Square brackets may also contain character ranges. For instance, [a-z] is a character in range from a to z, and [0-5] is a digit from 0 to 5. In the example below we're searching for "x" followed by two digits or letters from A to F: alert( "Exception 0xAF".match(/x [0-9A-F] [0-9A-F]/g) ); Here [0-9A-F] has two ranges: it searches for a ... Oct 03, 2018 - That is, there is a function that lets you get a range of numbers or characters by passing the upper and lower bounds. Is there anything built-in to JavaScript natively for this? If not, how would I implement it? The For Loop. The for loop has the following syntax: for ( statement 1; statement 2; statement 3) {. // code block to be executed. } Statement 1 is executed (one time) before the execution of the code block. Statement 2 defines the condition for executing the code block. Statement 3 is executed (every time) after the code block has been executed.
Get dates in between two dates with JavaScript. Raw. getDates.js. // Returns an array of dates between the two dates. function getDates (startDate, endDate) {. const dates = [] let currentDate = startDate. const addDays = function (days) {. A function to create flexibly-numbered lists of integers, handy for each and map loops. start, if omitted, defaults to 0; step defaults to 1. Returns a list of integers from start to stop, incremented (or decremented) by step, exclusive. ... Iterates over a list of elements, yielding each in ... Sep 03, 2020 - Recently, I found myself in just this predicament and thought that JavaScript would have a nice and easy built in function to make the process easier, like Ruby does. I was surprised to find that there is nothing similar to the simple built in Ruby Range method, and quite a bit of different…
Feb 26, 2020 - Previous: Write a JavaScript program to find a value which is nearest to 100 from two different given integer values. Next: Write a JavaScript program to find the larger number from the two given positive integers, the two numbers are in the range 40..60 inclusive. If we want to create a range of numbers and we want them as fast as possible we can use the Lodash _.range () util method. This method returns an array and takes three arguments: The first for the start of the range, second for end of range (up to) and third for step, or value incremented/decremented by. The for/in statement loops through the properties of an object. The block of code inside the loop will be executed once for each property. JavaScript supports different kinds of loops: for - loops through a block of code a number of times. for/in - loops through the properties of an object. for/of - loops through the values of an iterable object.
Here in this post, I am sharing few simple JavaScript example showing how to extract or read values from Input type Range and submit the values. I am not using any code behind procedure or an API for data submission ; the example here focuses mainly on how to extract the values from the Range slider (the element), show the values in a <label> and update the browser URL with the values, using ... For fun, I implemented a version of Python's range function in JavaScript. I found that underscore.js implements it as well, but with notable differences. I'm hoping others can comment on the differences. I assume that underscore's implementation is, if not more optimal, at least more idiomatic. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Math.random () in JavaScript generates a floating-point (decimal) random number between 0 and 1 (inclusive of 0, but not 1). Let's check this out by calling: console .log ( Math .random ()) This will output a floating-point number similar to: 0.9261766792243478. This is useful if you're dealing with percentages, as any value between 0 and 1 ... This proposal describes adding a Number.range and a BigInt.range to JavaScript. See the rendered spec at here. ... A polyfill is available in the core-js library. You can find it in the ECMAScript proposals section. May 01, 2018 - However, according to .../docs/Web/JavaScript/Reference/Global_Objects/Array/map#Description, map will not call for unassigned element. This mean we need to initialise the new Array(n) before mapping. One standard technique is to use fill. The final result is the following. function range(start, end) ...
JavaScript Range is a function that is supported by JavaScript in order to return all the integer and its value from starting to the ending while traversing from start index to the end index. The concept of the range is basically derived from the concept of selection which includes a pair of boundary points with the start range and end range. In the example below, the function contains a for statement that counts the number of selected options in a scrolling list (a <select> element that allows multiple selections). The for statement declares the variable i and initializes it to 0.It checks that i is less than the number of options in the <select> element, performs the succeeding if statement, and increments i by after each pass ... Code language: CSS (css) How it works. First, declare a variable counter and initialize it to 1.; Second, display the value of counter in the Console window if counter is less than 5.; Third, increase the value of counter by one in each iteration of the loop.; Since the for loop uses the var keyword to declare counter, the scope of counter is global. Therefore, we can access the counter ...
Math.js is an extensive math library for JavaScript and Node.js. It features big numbers, complex numbers, matrices, units, and a flexible expression parser. The following code sample gets the range B2:E6, loads its text property, and writes it to the console. The text property of a range specifies the display values for cells in the range. Even if some cells in a range contain formulas, the text property of the range specifies the display values for those cells, not any of the formulas. Mar 27, 2021 - You can find some recipes for common tasks at the end of the chapter, in “Summary” section. Maybe that covers your current needs, but you’ll get much more if you read the whole text. The underlying Range and Selection objects are easy to grasp, and then you’ll need no recipes to make ...
Mar 17, 2020 - If you found this algorithm helpful, check out my other recent JavaScript algorithm solutions: ... We are going to write a function that outputs the only number in an array that doesn’t have duplicates. ... For today’s algorithm, we are going to write a function called pangrams that will ... JavaScript exercises, practice and solution: Write a JavaScript program to get the integers in range (x, y). Favorite JavaScript utilities in single line of code. Extract year, month, day, hour, minute, second and millisecond from a date
Because we pass in the range object, inside the isInRange() function, the this keyword references to the range object. Finally, show the result array in the web console. In this tutorial, you have learned how to use the JavaScript Array filter() method to filter elements in an array based on a test provided by a callback function. Array Number Ranges in JavaScript ES6 Tue, Dec 9, 2014. Warning: Since this post was published, the ECMAScript Technical Committee has removed the comprehensions feature from ES6. This post shows usage of other (supported) features of the language, and has been left unedited. I've spent the last few days really digging into ECMAScript 6. The ... Feb 27, 2020 - What are the looping structures are available in javascripts? ... how to create a function that takes html parameter `data` and uses a for..in loop to format and console.log data ... Install and run react js project... ... Error: Node Sass version 5.0.0 is incompatible with ^4.0.0.
In this chapter, we will show you how to use sets and ranges in JavaScript. Putting several characters or character classes inside square brackets allows searching for any character among the given. To be precise, let's consider an example. Here, [lam] means any of the given three characters 'l', 'a', or 'm'. It is known as a "set". Jan 20, 2019 - JavaScript doesn’t have a built-in range() function. This can be useful to perform an iteration a certain number of times. As an alternative to this, you can use a JavaScript array. Aug 04, 2020 - JavaScript ES6 introduced us to several powerful features, such as the spread and rest syntax. Learn everything you need to know in this quick guide. Can I use an arrow function as the callback for an event listener in JavaScript?
Description. A for...in loop only iterates over enumerable, non-Symbol properties. Objects created from built-in constructors like Array and Object have inherited non-enumerable properties from Object.prototype and String.prototype, such as String 's indexOf () method or Object 's toString () method. The loop will iterate over all ... Range represents a set of one or more contiguous cells such as a cell, a row, a column, block of cells, etc. To learn more about how ranges are used throughout the API, start with Ranges in the Excel JavaScript API. [ API set: ExcelApi 1.1 ] JavaScript is a high-level, object-based, dynamic scripting language popular as a tool for making webpages interactive. Next in series: How To Define Functions in JavaScript Still looking for an answer?
22/4/2019 · This article describes how to generate a random number using JavaScript. Method 1: Using Math.random() function: The Math.random() function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). This random number can then be scaled according to the desired range. Syntax: Math.random(); Range is something I discovered recently, which again showed me that the possibilities with the Javascript and the DOM are truly endless. As stated on Mozilla's developer site, range ...
Custom Animated Range Slider Using Html Css Amp Javascript
Top 7 Best Range Input Replacement Javascript And Jquery
Creating A Range In Javascript Have You Ever Worked On A
How To Limit A Number Between A Min Max Value In Javascript
What I Learned From Reading Random Number In Range Or How I
Creating A Range Loop Directive In Angularjs
10 Javascript Range Sliders Csshint A Designer Hub
For Loop Js Range Code Example
Really Should Blog This A Pure Javascript Numbers Range
Awesome Vanilla Javascript Range Slider Example Rangeslider
Javascript Recursion Function Get The Integers In A Range
Javascript Sum All Numbers In A Range And Diff Two Arrays
Javascript Daterangepicker Syncfusion Javascript Ui
Javascript Range Slider Snippets Code Markuptag
Creating Price Range Slider Using Javascript Html And Css
Javascript Algorithm Sum All Numbers In A Range By Erica N
Javascript Basic Check Whether Two Numbers Are In Range 40
Javascript Group On An Array Of Objects With Timestamp Range
Add Range Numbers In Array Javascript Code Example
Javascript Switch Case Range Code Example
Coding Grounds Enumerable Range In Javascript
Mj Date Range Picker For Vue Js 2 Vue Script
Function Range As Range Js Code Example
Javascript Range Selector Control Html5 Slider Selector
Australia Salary Range Of Javascript Developers By Level Of
How To Create An Animated Range Slider In Html Css And
How To Do A Round Slider Html Css The Freecodecamp Forum
Smooth Custom Range Slider Control With Pure Javascript
How To Get Values From Html5 Input Type Range Using Javascript
0 Response to "33 For I In Range Javascript"
Post a Comment