20 Regex Search And Replace Javascript
In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a String The replace () method returns a new string with some or all matches of a pattern replaced by a replacement. The pattern can be a string or a RegExp, and the replacement can be a string or a function to be called for each match. If pattern is a string, only the first occurrence will be replaced. The original string is left unchanged.
Regular Expression In Javascript
This online Regex Replace tool helps you to replace string using regular expression (Javascript RegExp). Coding.Tools. Regex Replace Online Tool. Regular Expression. ... Replace regular expression matches in a string using Python: import re regex_replacer = re pile("test", re.IGNORECASE) test_string = "This is a Test string." ...
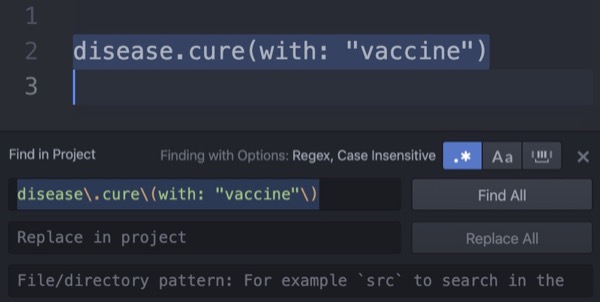
Regex search and replace javascript. let newStr = str.replace (regexp, newSubstr); Code language: JavaScript (javascript) In this syntax, the replace () method find all matches in the str, replaces them by the newSubstr, and returns a new string ( newStr ). The following example uses the global flag ( g) to replace all occurrences of the JS in the str by the JavaScript: let str ... Search and Replace for Windows is a fast, easy to use utility for search and replace in multiple files, directories and archives. Supports grep style Regular Expressions. Script language for webmasters and programmers. Using regular expressions in JavaScript Regular expressions are used with the RegExp methods test () and exec () and with the String methods match (), replace (), search (), and split (). These methods are explained in detail in the JavaScript reference.
It seems like the dot character in Javascript's regular expressions matches any character except new line and no number of modifiers could change that. Sometimes you just want to match everything and there's a couple of ways to do that. You can pick an obscure character and apply a don't match character range with it ie [^`]+. Regular expressions, abbreviated as regex, or sometimes regexp, are one of those concepts that you probably know is really powerful and useful. But they can be daunting, especially for beginning programmers. It doesn't have to be this way. JavaScript includes several helpful methods that make using regular expressions much more JavaScript RegExp Reference Previous Next RegExp Object. A regular expression is an object that describes a pattern of characters. Regular expressions are used to perform pattern-matching and "search-and-replace" functions on text. Syntax /pattern/modifiers; Example.
Old post but it worth to extend @ChaosPandion answer for other use cases with more restricted RegEx. E.g. ensure the (f) or capturing group surround with a specific format /z(f)oo/: > a="foobazfoobar" 'foobazfoobar' > a.replace(/z(f)oo/, function($0,$1) {return $0.replace($1, $1.toUpperCase());}) 'foobazFoobar' // Improve the RegEx so `(f)` will only get replaced when it begins with a dot or ... The search pattern can be used for text search and text to replace operations. A regular expression can be a single character or a more complicated pattern. Regular expressions can be used to perform all types of text search and text replace operations. Nov 24, 2019 - This will determine if you need to change the case of the first letter of after. This extra bit of logic is needed and used to create the replacement value for the replace method’s second argument. I can not think of a way to only use regex without this extra piece of logic to solve this problem.
var str = 'asd-0.testing'; var regex = /asd-(\\d)\\.\\w+/; str.replace(regex, 1); That replaces the entire string str with 1. I want it to replace the matched substring instead of the whole string... The replace () method in JavaScript searches a string for a specified value or a regular expression and returns a new string with some or all matched occurrences replaced. The replace () method accepts two parameters: const newStr = string.replace( substr | regexp, newSubstr |function); The first parameter can be a string or a regular expression. Nov 07, 2011 - Not the answer you're looking for? Browse other questions tagged javascript regex replace or ask your own question.
4 weeks ago - Learn how to use regular expressions (regex) with find and replace actions. A call to regexp.exec (str) returns the first match and saves the position immediately after it in the property regexp.lastIndex. The next such call starts the search from position regexp.lastIndex, returns the next match and saves the position after it in regexp.lastIndex. Some applications support special tokens in replacement strings that allow you to insert the subject string or the part of the subject string before or after the regex match. This can be useful when the replacement text syntax is used to collect search matches and their context instead of making ...
JavaScript regular expressions automatically use an enhanced regex engine, which provides improved performance and supports all behaviors of standard regular expressions as defined by Mozilla JavaScript. The enhanced regex engine supports using Java syntax in regular expressions. The enhanced regex Url Validation Regex | Regular Expression - Taha match whole word Match or Validate phone number nginx test Match html tag Blocking site with unblocked games Find Substring within a string that begins and ends with paranthesis Empty String Match dates (M/D/YY, M/D/YYY, MM/DD/YY, MM/DD/YYYY) Checks the length of number and not starts with 0 Aug 02, 2019 - If you have ever done any sort of sophisticated text processing and manipulation in JavaScript, you’ll appreciate the new features introduced in ES2018. In this article, we take a good look at how the ninth edition of the standard improves the text processing capability of JavaScript.
4 weeks ago - Learn how to use regular expressions (regex) with find and replace actions. EDIT following a good suggestion from @Niet the Dark Absol. As soon as there are several unique characters to look for, with the same replacement, this kind of regexp /(a|b|c)/ can be replaced by /[abc]/, which is both simpler and more efficient!. Any of the above proposed solutions can be improved this way, so the latter one becomes: Oct 20, 2018 - In a specified input string, replaces strings that match a regular expression pattern with a specified replacement string.
In JavaScript, you can use regular expressions with RegExp () methods: test () and exec (). There are also some string methods that allow you to pass RegEx as its parameter. They are: match (), replace (), search (), and split (). Executes a search for a match in a string and returns an array of information. The replaceAll () method returns a new string with all matches of a pattern replaced by a replacement. The pattern can be a string or a RegExp, and the replacement can be a string or a function to be called for each match. The original string is left unchanged. Sometimes we need to find only those matches for a pattern that are followed or preceded by another pattern. There's a special syntax for that, called "lookahead" and "lookbehind", together referred to as "lookaround".
Mostly a regular expression is used to validate a string against a pattern or extract a specific substring out of a string or check if a string contains a certain substring, and for checking this, we use the RegExp.test() function.. JavaScript String test(), replace() and match() Functions:. The test() function is used along with a regular expression pattern providing a string as an argument. Nov 07, 2011 - Connect and share knowledge within a single location that is structured and easy to search. ... How can I use javascript regexp to do a case insensitive, global search and replace on a string with the following pattern: With RegEx JavaScript, you can either search for or replace a piece of text, by using string methods like search () and replace (). RegEx are made of modifiers and patterns. Patterns are comprised of brackets, metacharacters, and quantifiers. Previous Topic Next Topic
Save Your Code. If you click the save button, your code will be saved, and you get a URL you can share with others. Both approaches make use of JavaScript's .replace () method to search out and replace the specified word in the given statement. The .replace () method is used to return a new string with some or all matches of a patternreplaced by a replacement. It accepts either a regular expression (regex) pattern or a string for replacement. Aug 02, 2018 - Find out the proper way to replace all occurrences of a string in plain JavaScript, from regex to other approaches
I need a simple workflow to copy text -> use RegEx to replace various text within the clipboard content and paste it back. My current workflow is to simply create a bunch of linked find and replace utility arguments inside Alfred. I am not sure if this is the best approach compared to a simple... Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The.replace method is used on strings in JavaScript to replace parts of string with characters. A regular expression is a special sequence of characters that help you match or find other strings or sets of strings, using a specialized syntax held in a pattern. They can be used to search, edit, or manipulate text and data. The replaceFirst () and replaceAll () methods replace the text that matches a given regular expression.
Next: Replacement and Lax Matches, Previous: Unconditional Replace, Up: Replace [Contents][Index] ... The M-x replace-string command replaces exact matches for a single string. The similar command M-x replace-regexp replaces any match for a specified regular expression pattern (see Regexps). The replace () method searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced. Note: If you are replacing a value (and not a regular expression), only the first instance of the value will be replaced. The string method string.replace (regExpSearch, replaceWith) searches and replaces the occurrences of the regular expression regExpSearch with replaceWith string. To make the method replace () replace all occurrences of the pattern you have to enable the global flag on the regular expression:
Javascript Replace All Occurrences Of String Code Example
Javascript Regex Match Example How To Use Js Replace On A
Find And Replace Text Using Regular Expressions Pycharm
Search Amp Replace Using Regular Expressions Hhllcks De
Use Regular Expressions Visual Studio Windows Microsoft
How To Replace All Occurrences Of A String In Javascript
Javascript Regex Match Example How To Use Js Replace On A
Find And Replace Using Regular Expression In Visual Studio
Introduction To The Use Of 10 Regular Expressions In
Find And Replace Regex Html Tag Or Attribute Html Tuts Com
Javascript Replace Method To Change Strings With 6 Demos
How Do I Use Grep Regex To Remove Newline Characters Like Cr
A Step By Step On How To Replace A Text In Notepad With
Editpad Pro Convenient Text Editor With Complete Support
Javascript Regular Expression How To Create Amp Write Them In
Regex Replace Using Atom Samwize
Everything You Need To Know About Regular Expressions By
How Javascript Works Regular Expressions Regexp By
Javascript Replace A Step By Step Guide Career Karma
0 Response to "20 Regex Search And Replace Javascript"
Post a Comment