29 Javascript Regex String Match
Aug 27, 2020 - So as you can see, replace combined with regex is a very powerful tool in JavaScript. The replace method can be useful if you want to replace a specific search pattern with some other string after some processing of the original string. It can also be used to correct an invalid match – for ... To learn more about the includes() method, check out JavaScript String includes() Method guide. 3. String search() Method. The search() method searches the position of the substring in a string and returns the position of the match. The search value can be a string or a regular expression. It returns -1 if no match is found.
Don T Fear The Regex Getting Started On Regular Expressions
find a regex match in string javascript; string match regular expression javascript; javascript object match; javascript matches; react native string.match; match regex in string; string match javascript; javascript regex return matched string; match a name in a string javascript.match property; javascript regular expression extract; string ...
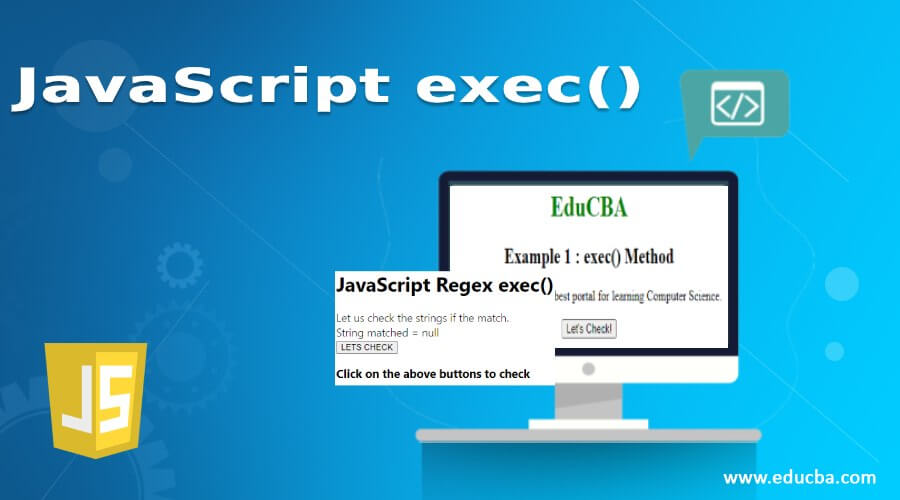
Javascript regex string match. 4 weeks ago - If it is a positive number with a positive sign, RegExp() will ignore the positive sign. const str1 = "NaN means not a number. Infinity contains -Infinity and +Infinity in JavaScript.", str2 = "My grandfather is 65 years old and My grandmother is 63 years old.", str3 = "The contract was declared null and void."; str1.match... For that we will use JavaScript strings dot match method. The match method accepts a regular expression object and retrieves the result of matching a string against the regular expression object. Let's see that with a working example. Currently our data contains the name, phone number and the credit card information. 8/12/2017 · Generally, and with default settings, ^ and $ anchors are a good way of ensuring that a regex matches an entire string. A few caveats, though: If you have alternation in your regex, be sure to enclose your regex in a non-capturing group before surrounding it with ^ and $: ^foo|bar$ is of course different from ^(?:foo|bar)$
Aug 02, 2019 - This regex matches a string containing meters only if it is immediately preceded by any two digits other than 35. The positive lookbehind ensures that the pattern is preceded by two digits, and then the negative lookbehind ensures that the digits are not 35. Read and learn two methods used to check whether a string matches RegEx in JavaScript. Also, see which method is the fastest and differences between them. In this tutorial you will learn how regular expressions work, as well as how to use them to perform pattern matching in an efficient way in JavaScript. ... Regular Expressions, commonly known as "regex" or "RegExp", are a specially formatted text strings used to find patterns in text.
The regexp.exec (str) method returns a match for regexp in the string str. Unlike previous methods, it's called on a regexp, not on a string. It behaves differently depending on whether the regexp has flag g. If there's no g, then regexp.exec (str) returns the first match exactly as str.match (regexp). First off, we'll want to use the JavaScript String match method, which takes a string or regular expression as an argument. const str = 'Hi there, my name is [name], I am [age] years old, and I work in the field of [profession].' ; const matches = str . match ( / some regex here / ) ; A sequence of characters that forms a search pattern, mainly for use in pattern matching with strings, or string matching. RegEx is nice because you can accomplish a whole lot with very little. In this case we are going to check various aspects of a string and see if it meets our requirements, being a strong or medium strength password.
In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a String Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec () and test () methods of RegExp, and with the match () , matchAll (), replace () , replaceAll (), search (), and split () methods of String. String.prototype.match () is a method in JavaScript that returns an array of results matching a string against a regular expression. Returned results depend a lot on using the global (g) flag. If a g flag is used we will get all results but without capturing groups.
RegExp.prototype.test () This method is used to test whether a match has been found or not. It accepts a string which we have to test against regular expression and returns true or false depending upon if the match is found or not. 18/8/2020 · JavaScript String match() method is used to search a string for a match against any regular expression and will return the match as an array if any match found. Javascript regex match. JavaScript match() method searches a string for a match versus a regular expression, and returns the matches, as the array. To use a javascript regex match, use a string match() method. Syntax string.match(regexp) … RegExr was created by gskinner , and is proudly hosted by Media Temple. Edit the Expression & Text to see matches. Roll over matches or the expression for details. PCRE & JavaScript flavors of RegEx are supported. Validate your expression with Tests mode. The side bar includes a Cheatsheet, ...
By default, a Group is a Capturing Group. Now, instead of using RegExp.test(String), which just returns a boolean if the pattern is satisfied, we use one of. String.match(RegExp) RegExp.exec(String) They are exactly the same, and return an Array with the whole matched string in the first item, then each matched group content. The string was split at 64a because that substring matches the regex specified. Note that the global flag - g - in split is irrelevant, unlike the i flag and other flags. This is because split splits the string at the several points the regex matches. Wrapping up. RegEx makes replaceing strings in JavaScript more effective, powerful, and fun. I want to use JavaScript (can be with jQuery) to do some client-side validation to check whether a string matches the regex: ^([a-z0-9]{5,})$ Ideally it would be an expression that returned true or false. I'm a JavaScript newbie, does match() do what I need? It seems to check whether part of a string matches a regex, not the whole thing.
Javascript String matchAll () The JavaScript String matchAll () method returns an iterator of results of matching a string against a regular expression. The syntax of the matchAll () method is: str.matchAll (regexp) Here, str is a string. Jun 13, 2021 - The method str.match(regexp) finds matches for regexp in the string str. ... If the regexp doesn’t have flag g, then it returns the first match as an array with capturing groups and properties index (position of the match), input (input string, equals str): The match () method searches a string for a match against a regular expression, and returns the matches, as an Array object. Read more about regular expressions in our RegExp Tutorial and our RegExp Object Reference. Note: If the regular expression does not include the g modifier (to perform a global search), the match () method will return only ...
The search() method executes a search for a match between a regular expression and this String object. ... A regular expression object. If a non-RegExp object regexp is passed, it is implicitly converted to a RegExp with new RegExp(regexp). Parentheses contents in the match Parentheses are numbered from left to right. The search engine memorizes the content matched by each of them and allows to get it in the result. The method str.match (regexp), if regexp has no flag g, looks for the first match and returns it as an array: 4/4/2020 · Javascript RegExp¶ Regex or Regular expressions are patterns used for matching the character combinations in strings. Regex are objects in JavaScript. Patterns are used with RegEx exec and test methods, and the match, replace, search, and split methods of String. The test() method executes the search for a match between a regex and a specified string.
The RegExp object has a number of top level methods, and to test whether a regular expression matches a specific string in Javascript, you can use RegExp.test (). To actually extract information from a string using a regular expression, you can instead use the RegExp.exec () call which provides more information about the match in its result. With Regex you have a piece of text called an expression which is used to match text in a string. This text contains normal characters, e.g. letters that you want to match, and special characters that control how things are matched. This is best explained with a few examples. May 02, 2020 - Summary: in this tutorial, you’ll learn about the JavaScript String match() method to match a string against a regular expression. To understand how the match() method works and how to use it effectively, you should have the basic knowledge of regular expression.
The String match () method matches a string against a regular expression: str.match (regexp); Code language: JavaScript (javascript) If the regexp is not a regular expression, the match () will convert it to a regular expression using the RegExp () constructor. The match () returns an array depending on whether the regular expression uses the ... Regular expressions (regex or regexp) are extremely useful in extracting information from any text by searching for one or more matches of a specific search pattern (i.e. a specific sequence of ... Sometimes it helps to enter your ... input strings. ... Code golf is a term used for the game of trying to express a particular program in as few characters as possible. Similarly, regexp golf is the practice of writing as tiny a regular expression as possible to match a given pattern, ...
In JavaScript, you can use regular expressions with RegExp () methods: test () and exec (). There are also some string methods that allow you to pass RegEx as its parameter. They are: match (), replace (), search (), and split (). Executes a search for a match in a string and returns an array of information. 4/1/2021 · const csLewisQuote = 'We are what we believe we are.'; const regex = /are/; csLewisQuote.match(regex); // ["are", index: 3, input: "We are what we believe we are.", groups: undefined] In this case, we .match() an array with the first match along with the index of the match in the original string, the original string itself, and any matching groups that were used. Photo by Mariana Medvedeva on Unsplash Use Regex.exec Instead of String.match if no Global Flag is Provided. Regex.exec is after than String.match and they both work the same if the /g flag isn ...
The search () method accepts a regular expression and returns the index of the first match in a string: let index = str.search (regexp); Code language: JavaScript (javascript) In this syntax, the regexp is a regular expression. If you pass a non-RegExp into the method, it will convert that value to a RegExp.
Introduction To The Use Of 10 Regular Expressions In
8 Regular Expressions You Should Know
How To Find Patterns Of Text In Javascript By Anh Dang
Javascript Fundamentals Understanding Regex By Timothy
A Guide To Javascript Regular Expressions
Javascript Regex Match Example How To Use Js Replace On A
Learn Regex A Beginner S Guide Sitepoint
Javascript Regular Expression Tester Codeproject
Basic Regex In Javascript For Beginners Dev Community
2 Using Regular Expressions Javascript Cookbook Book
Search A String By Matching Against A Regex In Javascript
Javascript Regular Expressions Cheatsheet And Examples
Javascript Regular Expression Word Boundaries
Javascript Extract Date From String Regex Extract Date
Javascript Exec How Exec Method Works In Javascript
How Do You Access The Matched Groups In A Javascript Regular
Javascript Regular Expression How To Create Amp Write Them In
8 Regular Expressions You Should Know
Check If String Matches Regex Typescript Code Example
Javascript Regular Expressions Programmer Sought
Match The Same String Twice With Backreferences In Regular Expressions
Github Jonschlinkert Regex Not Create A Javascript Regular
How To Match A String Of Words Using Regex Javascript Stack
Essential Guide To Regular Expressions Tools And Tutorials
An Introduction Guide To Javascript Regex
Regular Expression String Matching With String Inside
Understanding Regex With Notepad Dr Haider M Al Khateeb
0 Response to "29 Javascript Regex String Match"
Post a Comment