23 Change Html Text With Javascript
How can I change a text with JavaScript innerHTML? This is my example: I have the following text: Most programmers live on coffee. I want to add to this text: and chocolate So the new text should look like: Most programmers live on coffee and chocolate. JavaScript - Change Text in Paragraph. To change the text in a paragraph using JavaScript, get reference to the paragraph element, and assign new text as string value to the innerHTML property of the paragraph element.
Change Innerhtml Based On Id Using Javascript Didn T Work
Now, we want to change the text or font color of the content present inside the <div> element. Changing the text color To change the text color of a given element, first we need to access it inside the JavaScript by using the document.getElementId () or document.querySelector () methods and set its style.color property to your desired color.
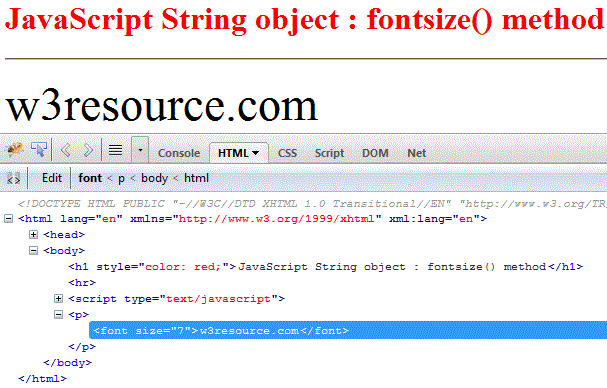
Change html text with javascript. Given an HTML document and the task is to change the text of span element. There are two property used to change the content. HTML DOM textContent Property: This property set/return the text content of the defined node, and all its descendants. By setting the textContent property, the child nodes the removed and are replaced by a single text node having the specified string. The biggest issue is that you want to save the question element, and then set its innerHtml attribute. Same with any answer element. Of course, as I made the answers in my demo option elements, you have to set their text attribute instead. May 18, 2020 - Invalid DOM property `for`. Did you mean `htmlFor`? ... Cannot inline bytecode built with JVM target 1.8 into bytecode that is being built with JVM target 1.6. Please specify proper '-jvm-target' option
How to change the text color in JavaScript: In this post, we will learn how to change the color of a text in JavaScript. We will create one small html-css-js project, that will contain only one button and clicking on that button will change the color of a text. Property to change: We need to change the color property of a component. Javascript provides us with the textContent property that we can use to change the text inside an element. How to set the font family for text with JavaScript? How to set the font size of a Tkinter Canvas text item? Set the font size with CSS; How to change the font size of a text using JavaScript? How to increase the font size of a Text widget? How to set the boldness of the font with JavaScript? How to set the font size of Matplotlib axis Legend?
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The JavaScript innerHTML property sets the HTML contents of an element on a web page. InnerHTML is a property of the HTML DOM. innerHTML is often used to set and modify the contents of a <p> element. You can use innerHTML like so: Find Your Bootcamp Match Save Your Code. If you click the save button, your code will be saved, and you get a URL you can share with others.
May 21, 2021 - The easiest way to change the text content of HTML pages is to manipulate the textContent property of the specific element using JavaScript. First, you need to select the element you want to change the text. It may be a Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. By using JavaScript, you can change any part of an HTML document in response to input from the person browsing the page. Before you get started, take a look at a couple of concepts. The first is a method called getElementById. A method is an action that's done to or by an object in a JavaScript program.
By using JavaScript, you can change any part of an HTML document in response to input from the person browsing the page. Before you get started, take a look at a couple of concepts. The first is a method called getElementById. A method is an action that’s done to or by an object in a […] The syntax to change style of a HTML element dynamically using JavaScript is. HTMLElement.style="styling_data". Try Online. In the following example, we are changing the style of an element whose id is message. document.getElementById ("message").style="color:#f00;padding:5px;" Try Online. JavaScript - how to change text on HTML pages. This guide will show you how to change text on pages using JavaScript. Posted on May 21, 2021. The easiest way to change the text content of HTML pages is to manipulate the textContent property of the specific element using JavaScript. First, you need to select the element you want to change the text.
Vanilla JavaScript provides two really easy ways to get and set content in the DOM—one to manipulate markup, and the other just for text. Manipulating HTML You can use the innerHTML to get and set HTML content in an element. var elem = document.querySelector('#some-elem'); // Get HTML content var html = elem.innerHTML; // Set HTML content elem.innerHTML = 'We can dynamically change the HTML. Any code can be removed without warning (if it is deemed offensive, damaging or for any other reason). w3schools are not responsible or liable for any loss or damage of any kind during the usage of provided code. ... The code has too many characters. ... JavaScript can change HTML content. Apr 14, 2019 - Learn how to change HTML text and CSS using JavaSCript function. Also learn, Self Invoking Anonymous Function and Arrow function in JS
Nov 17, 2017 - Vanilla JavaScript provides two really easy ways to get and set content in the DOM—one to manipulate markup, and the other just for text. ... You can use the innerHTML to get and set HTML content in an element. 1 week ago - This example fetches the document's current HTML markup and replaces the "<" characters with the HTML entity "<", thereby essentially converting the HTML into raw text. This is then wrapped in a <pre> element. Then the value of innerHTML is changed to this new string. innerText will not return the text of elements that are hidden with CSS (textContent will). Try it » Tip: Sometimes this property can be used instead of the nodeValue property, but remember that this property returns the text of all child nodes as well. Tip: To set or return the HTML content of an element, use the innerHTML property.
The text () method sets or returns the text content of the selected elements. When this method is used to return content, it returns the text content of all matched elements (HTML markup will be removed). When this method is used to set content, it overwrites the content of ALL matched elements. Tip: To set or return the innerHTML (text + HTML ... This article will illustrate how to dynamically change the Type attribute of HTML TextBox from Text to Password using JavaScript. HTML does not allow the Type attribute to be modified with JavaScript at runtime and hence the same can be achieved by creating a dynamic TextBox next to the Password TextBox and hiding the Password TextBox. We have simply created a text that identifies with the id "d1". Just open the text.html to check whether the text has begun showing on it or not. There it is! Create a Button. Let us now create a button that says "Change Text" in the body section. To create a button all you have to do is use a button tag with some input in it.
Changing HTML INPUT Type from Password to Text using JavaScript. Inside the ShowPassword JavaScript function, the reference of the CheckBox is received as parameter. Then the Password TextBox is referenced and if the CheckBox is checked, a dynamic TextBox element is created and appended next to it and the Password TextBox is hidden. The onchange event occurs when the value of an element has been changed. For radiobuttons and checkboxes, the onchange event occurs when the checked state has been changed. Tip: This event is similar to the oninput event. The difference is that the oninput event occurs immediately after the value of an element has changed, while onchange occurs ... Changing html content using JavaScript and CSS. Note: The innerHTML method has a problem when run on Netscape 6.2 and 7.02 on the PC (and possibly other versions). The problem occurs if you try to change the text of a button more than once in the same session (using innerHTML).
Change text with innerHTML. There is a simple way to change the text of any element. We have to use the innerHTML property of our selected element to change the text. Syntax. document.getElementById('id_name').innerHTML = "new_text"; Example 1 1 week ago - The textContent property of the Node interface represents the text content of the node and its descendants. Apr 04, 2018 - In JavaScript, the getElementById() function provides the capability to replace data in an element previously included in a document and identified by an id attribute. You include the idattribute within an HTML tag. For instance, to include the id attribute to identify a form as the contactForm, ...
Here we are going to see how we can change the text of an element using JavaScript function with various examples. JavaScript uses the innerHTML property to change the text of an element. Syntax document.getElementById('id_name').innerHTML = "new_text"; Explanation. Here id_name is the id of the HTML tag which you want to select. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Ever wonder how you could change the contents of an HTML element? Maybe you'd like to replace the text in a paragraph to reflect what a visitor has just selected from a drop down box. By manipulating an element's innerHtml you'll be able to change your text and HTML as much as you like · Each ...
The image and text can be changed by using javascript functions and then calling the functions by clicking a button. We will done that into 3 sections., in the first sesction we will create the structure by using only HTML in the second section we will desing minimally to make it attractive by using simple CSS and in the third section we will ... Jul 21, 2021 - Given an HTML document and the task is to change the text of a label using JavaScript. What is a label ? The <label>tag is used to provide a usability improvement for mouse users i.e, if a user clicks on the text within the <label> element, it toggles the control. HTML provides structure and meaning to our text, CSS allows us to precisely style it, and JavaScript contains a number of features for manipulating strings, creating custom welcome messages and prompts, showing the right text labels when needed, sorting terms into the desired order, and much more.
Get code examples like "change html text with javascript" instantly right from your google search results with the Grepper Chrome Extension. Depending on the kind of element being changed and the way the user interacts with the element, the change event fires at a different moment:. When the element is :checked (by clicking or using the keyboard) for <input type="radio"> and <input type="checkbox">;; When the user commits the change explicitly (e.g., by selecting a value from a <select>'s dropdown with a mouse click, by selecting a ...
Change The Html Source Code Using Javascript Or Jquery And
Html Javascript Add Javascript File To Html Dataflair
1 For The Following Html File Write Chegg Com
Javascript 25 Changing Html Content And Attribute
Javascript Fontsize Method String Object W3resource
Live Edit In Html Css And Javascript Intellij Idea
Using Spotfire Text Areas To Increase Usability Of Analytics
Javascript Replace Html Tags Replace And Regex Example
How To Change Buttons Content In Javascript Dev Community
How To Change Html Table Cell Value Ie If I Enter Quantity
Live Editing Html And Css With Chrome Devtools Lucidchart
How To Change Text Color In Html Javatpoint
Javascript Button To Change Text Color Of Paragraph Youtube
In This Video We Will Learn How To Change Attributes And
Change Attribute Value For A Html Element In Javascript
Javascript Changing Html Elements
Javascript Getelementbyid How Getelementbyid Work With
Change Html Content Using Javascript And Google Tag Manger
Live Edit In Html Css And Javascript Webstorm
How To Dynamically Change The Title Of Web Page Using
Javascript Events Explore Different Concepts And Ways Of
0 Response to "23 Change Html Text With Javascript"
Post a Comment