20 Javascript For Let Of
The JavaScript for/of statement loops through the values of an iterable objects. for/of lets you loop over data structures that are iterable such as Arrays, Strings, Maps, NodeLists, and more. The for/of loop has the following syntax: for ( variable of iterable) {. // code block to be executed. There's numerous ways to loop over arrays and objects in JavaScript, and the tradeoffs are a common cause of confusion. Some style guides go so far as to ban certain looping constructs. In this article, I'll describe the differences between iterating over an array with the 4 primary looping constructs: for (let i = 0; i < arr.length; ++i)
Understanding Var Let Amp Const In Javascript Es6 By Cem
Quick Tip - Use let with for Loops in JavaScript. The other problem using let and const will fix is with our for loop. This is something that you probably have all run into with your regular for loop, like one that will count from zero to nine: Problems that arise here are two things: First of all, if I type i into the console, it returns 10.
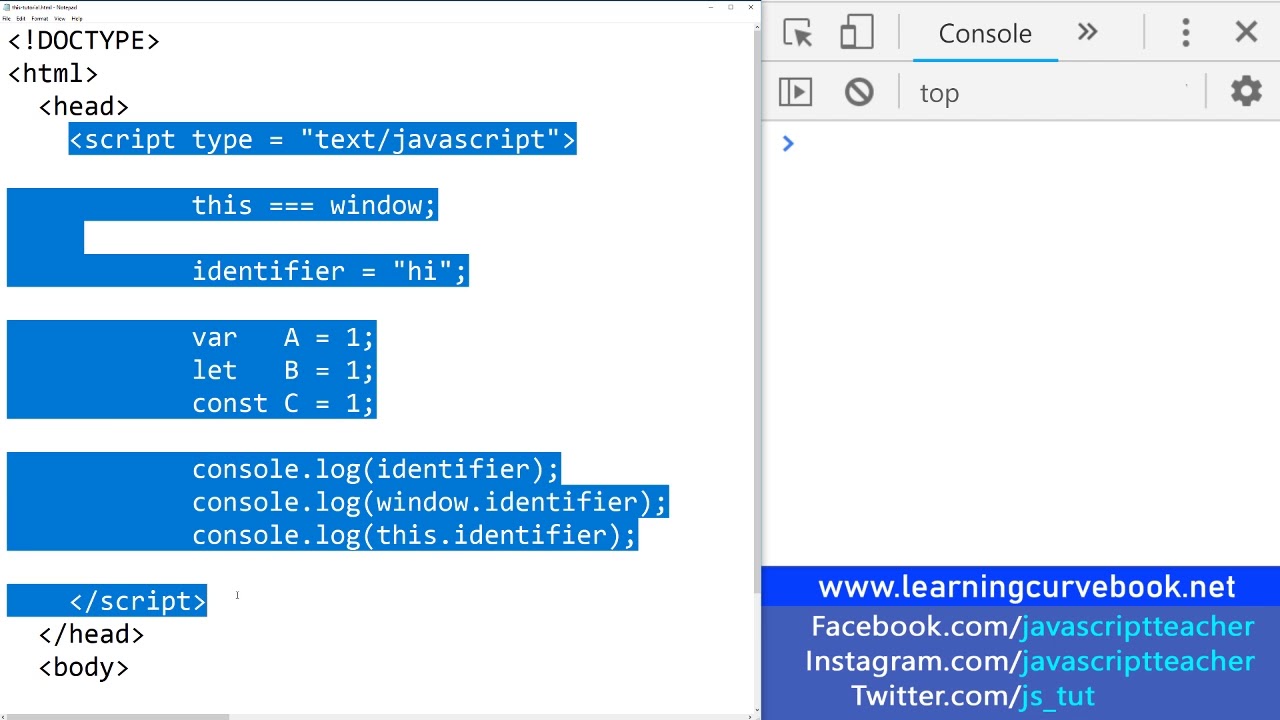
Javascript for let of. Oct 09, 2017 - ES2015 is the updated version of JavaScript (also known as ECMAScript). The more lax term for ES2015 is ES6. In ES2015, two other ways to declare variables were introduced. They are let and const. We shall discuss these types in later parts of this lesson. In this tutorial, we will learn ... are essential elements of the JavaScript programming language. ... The for statement is a type of loop that will use up to three optional expressions to implement the repeated execution of a code block. Let’s take a look at an example of ... JavaScript let Vs var. In this tutorial, you will learn about the difference between let and var in JavaScript with the help of examples. In JavaScript, both the keywords var and let are used to declare variables. The let keyword was introduced in the later version of JavaScript known as ES6(ES2015). And it's the preferred way to declare variables.
Let's see some examples of the JavaScript alert() method. Example1. In this example, there is a simple alert dialog box with a message and an OK button. Here, there is an HTML button which is used for displaying the alert box. We are using the onclick attribute and call the fun() function where the alert() is defined. Answer: A. Explanation: The "===" is known as a strict comparison operator which will result as true when the data-type, content of operand are the same. For example, In JavaScript, two strings can be considered as strict equal when the length, sequence, and same characters, are the same when compared to each other. The for await...of statement creates a loop iterating over async iterable objects as well as on sync iterables, including: built-in String, Array, Array-like objects (e.g., arguments or NodeList), TypedArray, Map, Set, and user-defined async/sync iterables. It invokes a custom iteration hook with statements to be executed for the value of each distinct property of the object.
May 28, 2021 - Since this example uses the let keyword, the variable i is blocked scope. It means that the variable i only exists and can be accessible inside the for loop block. In JavaScript, a block is delimited by a pair of curly braces {} like in the if...else and for statements: 1 week ago - var and let are both used for variable declaration in javascript but the difference between them is that var is function scoped and let is block scoped. It can be said that a variable declared with var is defined throughout the program as compared to let. An example will clarify the difference even better Example of ... The let keyword is used to declare variables in JavaScript. The var keyword can also be used to declare variables, but the key difference between them lies in their scopes. var is function scoped while let is block scoped. The var keyword allows you to re-declare a variable without any issue. However, re-declaring a variable using the let ...
In classical programming, the logical OR is meant to manipulate boolean values only. If any of its arguments are true, it returns true, otherwise it returns false. In JavaScript, the operator is a little bit trickier and more powerful. But first, let's see what happens with boolean values. Jan 18, 2019 - In javaScript, we can't loop through objects normally as we would on arrays, so, there are a few elements we can use to access either of our choices from an object. ... Step One: Convert the object to get either its key, value, or both. Step Two: loop through. // Getting the keys/property Step One: let ... JavaScript has only one type of number. Numbers can be written with or without decimals. Example. let x = 3.14; // A number with decimals. let y = 3; // A number without decimals. Try it Yourself ». Extra large or extra small numbers can be written with scientific (exponent) notation: Example. let x = 123e5; // 12300000.
The JavaScript for of statement loops through the values of an iterable object. It lets you loop over iterable data structures such as Arrays, Strings, Maps, NodeLists, and more: Syntax let allows you to declare variables that are limited to the scope of a block statement, or expression on which it is used, unlike the var keyword, which declares a variable globally, or locally to an entire function regardless of block scope. The other difference between var and let is that the latter is initialized to a value only when a parser evaluates it (see below). You also get per-iteration bindings in for loops (via let) and for-in loops (via const or let). Details are explained in the chapter on variables. 17.5 Iterating with existing variables, object properties and Array elements # So far, we have only seen for-of with a declared iteration variable.
ES6 introduced two important new JavaScript keywords: let and const. These two keywords provide Block Scope in JavaScript. Variables declared inside a { } block cannot be accessed from outside the block: Example. {. let x = 2; } // x can NOT be used here. Variables declared with the var keyword can NOT have block scope. Nov 01, 2018 - There has been a lot of confusion about the use of let and var in Javascript. So here is the difference where you would be now easily to decide what to use where and when. let gives you the privilege… Nov 02, 2017 - In this tutorial, we will learn ... are essential elements of the JavaScript programming language. ... The for statement is a type of loop that will use up to three optional expressions to implement the repeated execution of a code block. Let’s take a look at an example of ...
Let's learn about let and const variables. Before ES6, the only way you can create a variable in javascript was by using the var keyword: var x = 10; Since ES6, there are two new keywords, let and const. Below is an example to show their usage: let x = 10; const x = 10; Both of them do the same thing. they declare a variable "x" and assign the ... @Craig - You can't use const in a for loop in most cases (though you can in some), because of the "increment" part of the loop. On for (let i = 0; i < 10; ++i) for instance, after an iteration and the test, when doing the ++i part the engine creates the new i for the new iteration, assigns it the value the old i had, and then does the increment part: ++i, which modifies the new i. If you want to learn more about hoisting, study the chapter JavaScript Hoisting. Variables defined with let are also hoisted to the top of the block, but not initialized.
let and const were added as part of ES6 (a.k.a. ES2015) which has been around for three years now; browser support is good and for everything else there's Babel for transpiling ES6 into a more widely adopted JavaScript. So now is a good opportunity to look again at how we declare variables and gain some understanding of how they scope. If you don’t change variable inside the loop, you should use the const keyword instead of the let keyword as follows: let scores = [ 80, 90, 70 ]; for ( const score of scores) { console .log (score); } Code language: JavaScript (javascript) Output: 80 90 70. There are three ways to declare a variable in JavaScript: var, let, and const. Each of these variable types can be used in different circumstances, which we discuss later in this article. That said, the focus of this tutorial is the JavaScript keyword "let." "Let" allows you to declare variables that can be reassigned.
let, const and var are the three ways you can declare a variable in JavaScript. var is the older way of declaring variables while let and const were introduced in ES6 to solve the issues that arose due to the use of var. Jun 05, 2017 - TL;DR: The the first code snippet ... in the JavaScript implementation of the environment. That is, the correct behavior is to output 0, 1 and 2, instead of 3, 3 and 3. Modern browsers work correctly and output 0, 1 and 2 when you run the code. – Utku Jul 15 '17 at 12:25 · Apparently there are three different scopes here: let x = 5; for (let x = 0; ... In the following text we come to know the major difference between var and let in javascript. The main difference between let and var is that scope of a variable defined with let is limited to the block in which it is declared while variable declared with var has the global scope. So we can say that var is rather a keyword which defines a ...
Apr 28, 2021 - And now, since it's 2020, it's assumed that a lot of JavaScript developers have become familiar with and have started using these features. While this assumption might be partially true, it's still possible that some of these features remain a mystery to some devs. One of the features that came with ES6 is the addition of let ... If you're writing server-side JavaScript code , you can safely use the let statement. If you're writing client-side JavaScript code and use a browser based transpiler (like Traceur or babel-standalone ), you can safely use the let statement, however your code is likely to be anything but optimal with respect to performance. 3 weeks ago - Summary: in this tutorial, you will learn how to use the JavaScript let keyword to declare block-scoped variables. ... In ES5, when you declare a variable using the var keyword, the scope of the variable is either global or local. If you declare a variable outside of a function, the scope of ...
The for..of loop in our example iterates over the values of a data structure. The values in this specific example are 'el1', 'el2', 'el3'.The values which an iterable data structure will return using for..of is dependent on the type of iterable object. For example an array will return the values of all the array elements whereas a string returns every individual character of the string. Normally you will use statement 1 to initialize the variable used in the loop (let i = 0). This is not always the case, JavaScript doesn't care. Statement 1 is optional. You can initiate many values in statement 1 (separated by comma): ... Often statement 2 is used to evaluate the condition ... Jun 23, 2019 - In the second code tab that declares ... declared in - the highlighted lines. It is out of scope everywhere outside its block. Try uncommenting all the console.log(x) statements in the code that uses let and note how JavaScript throws an error....
The logical OR expression is evaluated left to right, it is tested for possible "short-circuit" evaluation using the following rule: (some truthy expression) || expr is short-circuit evaluated to the truthy expression.. Short circuit means that the expr part above is not evaluated, hence any side effects of doing so do not take effect (e.g., if expr is a function call, the calling never takes ... This loop logs only enumerable properties of the iterable object, in arbitrary order. It doesn't log array elements 3, 5, 7 or hello because those are not enumerable properties, in fact they are not properties at all, they are values.It logs array indexes as well as arrCustom and objCustom, which are.If you're not sure why these properties are iterated over, there's a more thorough explanation ... In JavaScript, let is a keyword which is used to declare a local variable with block scope. Unlike var keyword which declares global scope variables, with ECMAScript2016(ES6) the let keyword was introduced to define local scope variables as defining all the variables in global scope leads to a lot of problems while writing large JavaScript applications.
8/4/2020 · Normally, if we loop through an array using for-of loop we only get the values instead of index. const array = ['onion','noise','pin']; for(const value of array){ console.log(value); } To get the index in a for-of loop, we need to call the array with a entries () method and use destructuring syntax. const array … for (let i=0; i<arr.length; i++) - works fastest, old-browser-compatible. for (let item of arr) - the modern syntax for items only, for (let i in arr) - never use. To compare arrays, don't use the == operator (as well as >, < and others), as they have no special treatment for arrays. They handle them as any objects, and it's not what ... for..in is a method for iterating over "enumerable" properties of an object. It therefore applies to all objects (not only Object () s) that have these properties. An enumerable property is defined as a property of an object that has an Enumerable value of true. Essentially, a property is "enumerable", if it is enumerable.
Declaring Variables In Es6 Javascript By Yash Agrawal
Javascript The Weird Parts Chapter 1 Var Let Amp Const
Es6 Es7 Es8 Amp Writing Modern Javascript Pt1 Scope Let
Javascript Map How To Use The Js Map Function Array Method
What Is The Difference Between Var Let And Const In
Tools Qa What Is The Difference Between Javascript Let And
Answer To What S The Difference Between Using Let And Var
Understand Var Let And Const Keywords In Javascript Learn
Node Js Fixes Severe Http Bug That Could Let Attackers Crash Apps
How To Print The Numbers From 1 To 100 Without Including
Const Vs Let Vs Var Javascript W Zac Gordon
16 4 For Of Loop Topics Of Javascript Es6
Wait Until Something Something In Javascript Stack Overflow
Javascript Es6 Const Amp Let Uses And Code Examples Of The
Javascript Tutorial Part 3 Difference Between Var Let Amp Const
The Complete Guide To Loops In Javascript
Difference Between Var And Let Keyword In Javascript In
What S The Difference Between Using Let And Var In Javascript
Is Let Not A Reserved Keyword In Javascript Dev Community
0 Response to "20 Javascript For Let Of"
Post a Comment