33 Javascript Check If String Is Palindrome
Write a JavaScript function that checks whether a passed string is palindrome or not? Note: A palindrome is word, phrase, or sequence that reads the same backward as forward, e.g., madam or nurses run. If the flag is true at the end of the function, it signifies that the given string is a palindrome. If the flag is false at the end of the function, it signifies that the given string is not a palindrome. C++ Program to Check Whether a Given String Is a Palindrome or Not
Javascript Function The Longest Palindrome In A Specified
In this example, you will learn to write a JavaScript program that checks if the string is palindrome or not.
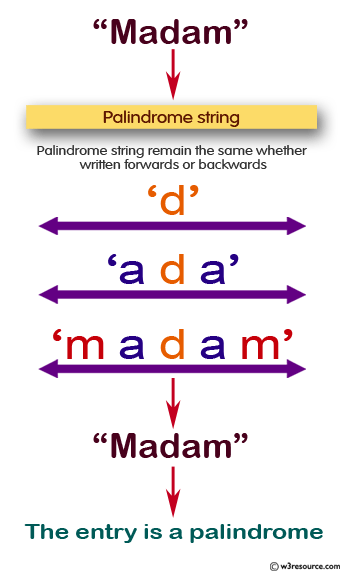
Javascript check if string is palindrome. In the above images, we entered a string MADAM and then click the OK button to check the given string is a Palindrome or not. After that, it shows the below image: Following are the built-in function to check the palindrome in JavaScript. The split (' ') function is used to convert the string into the individual array characters. 21/10/2019 · We have now looked at 8 different ways to check whether a given string is a palindrome and 3 different ways to approximate how close a given string is to be a palindrome. In terms of performance, the best way to check for a palindrome is to use either a classic for loop or a for…of loop. In terms of readability, it’s down to preference. Given a string, return true if the string is a palindrome or false if it is not. Palindromes are strings that form the same word if it is reversed. *Do* include spaces and punctuation in determining if the string is a palindrome.
for-loop reverses the string and stores the reversed string in reversed variable. At line (a), the comparison is taking place between the actual string and reversed string to check whether a string is a palindrome or not. This is how this variant works. Let's move forward to the fourth variant. 10/12/2020 · JavaScript - Find if string is a palindrome (Check for punctuation) Javascript Web Development Front End Technology Object Oriented Programming. We are required to write a JavaScript function that returns true if a given string is a palindrome. Otherwise, returns false. These are the conditions we have to keep in mind while validating the string −. The task of our function is to check whether we can make that string a palindrome string by deleting at most one character from the string. If we can do so, the function should return true, false otherwise. For example −. If the input string is −. const str = 'kjlk'; Then the output should be −. const output = true; because by deleting 'l ...
The easiest way to check for a palindrome string is by using the built-in Array methods called split (), reverse (), and join (). Here's an example of how you can do so: Check If String is Palindrome or Not using for Loop A string is a palindrome if it is read the same from forwarding or backward. For example, dad reads the same either from forwarding or backward. So the word dad is a palindrome. A palindrome is a word or sentence that's spelled the same way both forward and backward, ignoring punctuation, case, and spacing. Note: You'll need to remove all non-alphanumeric characters...
// program to check if the string is palindrome or not function checkPalindrome(str) { // convert string to an array const arrayValues = string.split(''); // reverse the array values const reverseArrayValues = arrayValues.reverse(); // convert array to string const reverseString = reverseArrayValues.join(''); if(string == reverseString) { console.log('It is a palindrome'); } else { console.log('It is not a palindrome'); } } //take input const string … In this example, you will learn how to check if the string is palindrome or not in JavaScript. Basically, we are going to learn different ways to checks if the string is palindrome or not in JavaScipt. A string is a palindrome if it is read the same from forward or backward. For example, dad reads the same either from forward or backward. A hashmap is an object in Javascript that has a key and value pair that can be used to keep a running count of items. The first thing you should be doing if you come across this problem is asking what a palindrome is defined in terms of letters. We all know that a palindrome can be read forward and backwards — popular examples being ...
The requirements for this task are that the code is returns a 'true' or 'false' for an input string. The string can be a simply word or a phrase. The other question does not address these needs. Please reopen and answer here. I am working on a function to check if a given string is a palindrome. In this tutorial you'll learn how to check if a string is a palindrome using JavaScript. Get my free 32 page eBook of JavaScript HowTos 👉 https://bit.ly/2T... Here, isPalindrome inline function is used to check for a palindrome string. It takes one string str as its argument and returns one boolean value. If str is null, return false. Find the midPoint i.e. the index of the middle character.
JavaScript Algorithms: Palindrome Checker. A palindrome is a word or sentence that reads the same from left to right as it does in reverse. A popular example of this would be "a man, a plan, a canal, Panama". If you were to write that phrase backwards, the spelling would be the same as if you had written it properly. How to check whether a passed string is palindrome or not in… First we iterate over a string in forward and backward direction. Check if all forward and backward character matches, return true. If all forward and backward character does not matches, return false. 11/2/2013 · Palindrome check in Javascript. function checkPalindrom (palindrom) { for ( var i = palindrom.length; i > 0; i-- ) { if ( palindrom [i] = palindrom.charAt (palindrom.length)-1 ) { document.write ('the word is palindrome.'); }else { document.write ('the word is not palindrome!'); } } } checkPalindrom ('wordthatwillbechecked');
The function PTest () is defined in which we will send the string which needs to be tested for palindrome functionality. In case the string is palindrome then we should receive a text in output confirming the same otherwise vice versa. The function is called at the end after the function definition. Palindrome Permutation — Cracking the Coding Interview ( Javascript, Java) Palindrome Permutation: Given a string, write a function to check if it is a permutation of a palindrome. A palindrome is a word or phrase that is the same forwards and backwards. A permutation is a rearrangement of letters.The palindrome does not need to be limited to ... Check if a string is Palindrome or not using Javascript. javascript Share on : The below function written in Javascript can be helpful to check whether a string is Palindrome or not. function palindrome_checker(str) { const converted_str = str.split('').reverse().join(''); if (str === converted_str) { console.log(str + " : String …
Write the most efficient function you can that determines whether a given string is a palindrome. Your function should accept a string as a parameter and return a boolean (true if the string is a palindrome, false if it is not). Assume that this code will be put into a real production system and write accordingly. Given a string, our task is to find string is palindrome or not. Example: Input : "race" Output : passed string is not a palindrome Explanation : if we write "race" in reverse that is "ecer" it not matches with first string so it is not a pallindrome. I created a function to test whether a single word string can be a rearranged into a palindrome (doesn't have to be a real word). My logic was if the string has an even number of letters, then each letter must occur twice. If the string has an odd number of letters, each letter must occur twice except for one.
This article is based on Free Code Camp Basic Algorithm Scripting "Check for Palindromes". A palindrome is a word, phrase, number, or other sequence of characters which reads the same backward or forward. The word "palindrome" was first coined by the English playwright Ben Jonson in the 17th century, from the Greek roots palin ("again") and dromos ("way, direction"). — src.
How To Find The Longest Palindrome In A String Using Python
C Program To Check The Given String Is Palindrome Or Not
A Few Succinct Ways To Solve The Palindrome Problem In
How To Check If A String Is A Palindrome
Skillpundit To Check Given String Is Palindrome Or Not In
Java Palindrome Program In Java String Amp Number Example
Write A Palindrome Check Function In Javascript Using String
Palindrome In Javascript Logical Explanation With Examples
Java67 How To Check If Strings Are Rotations Of Each Other
Check Whether A String Is Palindrome Or Not Face Prep
Javascript Function Check Whether A Passed String Is
Check If A String Is Palindrome In Javascript Code Example
How To Check Whether A String Is A Palindrome Or Not Through
Java Program To Check Whether A Number Is Palindrome Or Not
Check For Palindrome In Javascript 3 Different Ways
Palindrome Program In Java Tracedynamics
Algorithms 101 How To Check If A String Is A Palindrome
Palindrome In Java How To Check A Number Or String Edureka
How To Check If A String Is A Palindrome In Javascript By
Write A Palindrome Check Function In Javascript Using String And Array Methods
Js Coding Interview Question Build A Function That Tests To
C Exercises Check If A Given String Is A Palindrome Or Not
Ctci 1 4 Palindrome Permutation Javascript Es6 Solution Video
Palindrome In Php How To Check Palindrome Number Or String
Check Number Is Palindrome Or Not In Javascript
Check If A String Is A Palindrome In Javascript
Check Palindrome Javascript Code Example
Javascript Palindrome Code Example
Lex Program To Check Whether Given String Is Palindrome Or
Check If String Is Palindrome Or Not In Javascript Coding
11 Ways To Check For Palindromes In Javascript By Simon
0 Response to "33 Javascript Check If String Is Palindrome"
Post a Comment