26 Check Opening And Closing Brackets Javascript
Aug 03, 2014 - Given an expression string exp, write a program to examine whether the pairs and the orders of "{","}","(",")","[","]" are correct in exp. For example, the program should print true f... If the current character is an opening bracket ( or { or [ then push it to stack. If the current character is a closing bracket ) or } or ] then pop from stack and if the popped character is the matching starting bracket then fine. At the end of the traversal, if there is some opening bracket left in stack then the string is "not balanced".
1 Writing Your First Javascript Program Javascript
Or if you insist on using regexp, then use two regexp to check for balanced parens like this: function balanced(str) { var opened = str.match(/\ (/g); // match open parens var closed = str.match(/\)/g); // match close parens opened = opened? opened.length: 0; // get the count of opened parens, if nothing is matched then 0 closed = closed ...
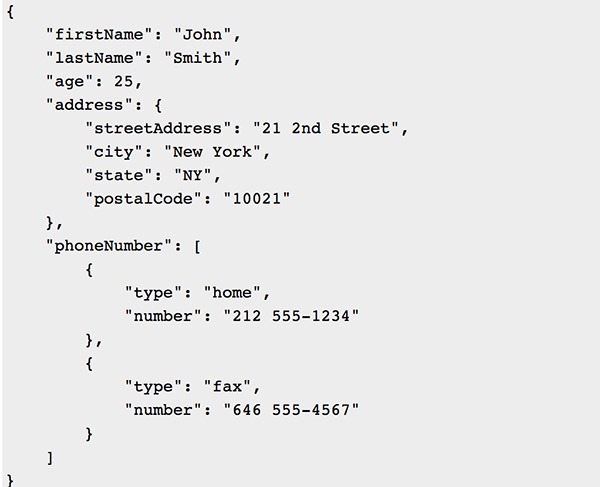
Check opening and closing brackets javascript. For every closing bracket that is encountered pop an opening bracket from the stack. If the stack becomes empty i.e. the size of the stack is 0 at some index, print the index. Else print -1. Algorithm. Initialize a string s of length/size n and an integer value representing the index of an opening square bracket. A bracket is considered to be any one of the following characters: (, ), {, }, [, or ]. Two brackets are considered to be a matched pair if the an opening bracket (i.e., (, [, or {) occurs to the left of a closing bracket (i.e., ), ], or }) of the exact same type.There are three types of matched pairs of brackets: [], {}, and (). A matching pair of brackets is not balanced if the set of ... Given an incomplete bracket sequence S. The task is to find the number of closing brackets ')' needed to make it a regular bracket sequence and print the complete bracket sequence. You are allowed to add the brackets only at the end of the given bracket sequence. If it is not possible to complete the bracket sequence, print "IMPOSSIBLE".
If there is a closing bracket, check the top of the stack. If the top of the stack contains the opening bracket match of the current closing bracket, then pop and move ahead in the string. If the top of the stack is not the opening bracket match of the current closing bracket, the parentheses are not balanced. In that case, break from the loop. we will create a map of parentheses with opening parentheses as key and corresponding closing ones as value. will declare an empty array to use as a stack. will check the string character by character. if the character is an opening bracket will push it to stack. if it is a closing bracket, will check the last entry in the stack. Parenthesis Matching Problem in JavaScript @ The Hacking School , A classic problem — Check for balanced parentheses in an expression. if the an opening bracket (i.e., (, [, or { ) occurs to the left of a closing… Need to find open and closed bracket, if the sequence of opening and closing ...
Regular expressions are the wrong tool for the job because you are dealing with nested structures, i.e. recursion. But there is a simple algorithm to do this, which I described in more detail in this answer to a previous question.The gist is to write code which scans through the string keeping a counter of the open parentheses which have not yet been matched by a closing parenthesis. Declare a map matchingParenMap and initialize it with closing and opening bracket of each type as the key-value pair respectively. Declare a set openingParenSet and initialize it with the values of matchingParenMap. Declare a stack parenStack which will store the opening brackets '{', '(', and '['. Now traverse the string expression input. If the current character is a starting bracket (' (' or ' {' or ' [') then push it to stack. If the current character is a closing bracket (')' or '}' or ']') then pop from stack and if the popped character is the matching starting bracket then fine else brackets are not balanced.
Dec 31, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. I feel that my approach with it isn't too practical as it involves a lot of conditionals. When a closing bracket is found, there has to be some way to determine if they match properly. Would an std::map be beneficial in serving as a look-up table for associating the opening and closing brackets with each other? I feel that it may help with DRY ... May 25, 2018 - If a ‘closed’ char is found, check if the matching closed parenthesis of the last element that is popped off the stack (i.e. for the last open parenthesis symbol found in the passed-in string) is not equal to the current chr. And if the chr isn’t the matching closed parenthesis for the ...
Nov 03, 2017 - Problem: "Sometimes (when I nest them (my parentheticals) too much (like this (and this))) they get confusing." Write a function that, given a sentence like the one above, along with the posi... A classic problem — Check for balanced parentheses in an expression. Two brackets are considered to be a matched pair if the an opening bracket (i.e., (, [, or {) occurs to the left of a closing... \$\begingroup\$ When you check for balanced brackets you must ensure that an opening bracket comes before a closing bracket. Your code doesn't take that into account and fails if say a function is declared with a closing brace before an opening brace (which is invalid) but your code passes it. \$\endgroup\$ - Aadit M Shah Sep 6 '12 at 14:32
Generate a string with N opening brackets [ and with N closing brackets ], in some arbitrary order. Determine whether the generated string is balanced; that is, whether it consists entirely of pairs of opening/closing brackets (in that order), none of which mis-nest. Examples The idea is to add all opening brackets to a stack and pop them off the stack when closing brackets are found. If the closing bracket doesn't match the top element (last pushed element) of the stack, then the string is invalid. The string is also invalid if it has been iterated through and the stack is not empty in the end. This utility allows you to visually check that your code's braces (a.k.a., curly braces), parentheses, brackets, and tags are balanced. It also makes it easy to see what braces open and close a given section of code.
Need to find open and closed bracket, if the sequence of opening and closing brackets is violated, then return false. But if don't revert right array to compare with left array, i don't make check brackets here { [ (3+1)+2]+}. And if reverse like now, then i fail to check here [1+1]+ (2*2)- {3/3} Dec 06, 2016 - This would be more so if the set of parentheses were to be extended and function seen as of n characters check if n types of open/close delimiters are balanced. Personally the c++ answer is by far the best here but sadly javascript is not a safe language for recursion (until tail call optimization ... Given a string of brackets, the task is to find an index k which decides the number of opening brackets is equal to the number of closing brackets. String must be consists of only opening and closing brackets i.e. ' (' and ')'.
Jul 13, 2018 - If these two (the open sign just ... in the same index the closing and the opening tokens If the index of a token from the "open" array is equal to the index of a token from the "close" array, then, these two tokens match */ var bracket = function (input) { var open = ['{', ... Jun 05, 2017 - Given a string of parenthesis such as ‘{}()[]’ return true or false if every opening parenthesis matches its closing partner. What’s the use of this? Imagine a situation if you were writing a… Sep 15, 2017 - Hi, I have been trying to solve - https://www.freecodecamp /challenges/validate-us-telephone-numbers Now i have read and searched but could not find any regex where i can check if there is any opening bracket then only there can be a closing bracket, i have been stuck on this point for some ...
For every closing bracket we will search for the opening bracket from last opening bracket to the index of this closing bracket. Once there is a match of opening and closing bracket, we will replace the pair with '*'. At last we will check the value of counter, if it is zero our expression is balanced. Pseudo-Code Approach: Declare a Flag variable which denotes expression is balanced or not.; Initialise Flag variable with true and Count variable with 0.; Traverse through the given expression. If we encounter an opening parentheses (, increase count by 1; If we encounter a closing parentheses ), decrease count by 1; If Count becomes negative at any point, then expression is said to be not balanced, ValidateJavaScript is an online validating (or linting) tool that will automatically find basic errors and help prevent potentially destructive bugs in JavaScript and JSX (React.js) code. Copy and paste or directly input your code into the editor above, click the 'Find & Fix Errors' button, and the tool will parse your code and list all errors ...
First we read the equation, ignooring all other characters and push the opening brackets into the stack using push operation and perform the pop operation for a closing bracket, if there are equal brackets i.e one closing bracket for every opening bracket then in the end we have an empty stack or else the brackets are not balanced. Trying viewing this Pen in Debug Mode, which is the preview area without any iframe and does not require JavaScript. Although what the preview is of might! Need to know how to enable JavaScript? Go here. Close this, use anyway. Find index of closing bracket for a given opening bracket in an expression. Given a string with brackets. If the start index of the open bracket is given, find the index of the closing bracket. Input : string = [ABC [23]] [89] index = 0 Output : 8 The opening bracket at index 0 corresponds to closing bracket at index 8.
As Doug suggested, you could open the Replace dialog and replace the character you want to count with itself (replace { with { or } with }). Word's search and replace function could automatically report the number of replacements made. In other way, if you want to use macro to implement, you could check below sample. Mar 03, 2020 - Given a string containing brackets, determine if all brackets form balanced pairs. Check opening and closing brackets javascript. Php Parse Syntax Errors And How To Solve Them Stack Overflow. Auto Closing Brackets Not Working On Vscode 1 57 Issue. Solving Balanced Brackets In Javascript With Stacks By Noam. Check For Balanced Brackets In An Expression Well Formedness.
Opening & Closing Brackets Count. In order to validate whether an expression is valid or not, we could count the number of opening brackets and the number of closing brackets and see if both numbers are the same.Though this would detect a lot of invalid expressions, not all invalid expressions would be detected. How to validate brackets, To check the balance, you will need only a counter which will be incremented in case of ( and I don't know javascript, this is some sort of pseudocode: Edit 2: Ah. veryBadBrakets now has more closing brackets than opening brackets. If the current character is an opening ... Closing Tag Checker for HTML5. By Alicia Ramirez. In HTML5, under certain circumstances, some closing tags are optional.If you leave those closing tags out, the W3C ...
Jul 18, 2021 - A classic problem — Check for balanced parentheses in an expression. Two brackets are considered to be a matched pair if the an opening bracket (i.e., (, [, or { ) occurs to the left of a closing… It just checks , whether the expression has equal number of opening and closing parentheses or brackets or braces and does not check the order. For example, consider the following expression. ([{)}] The above expression has the equal number of opening and closing parentheses / brackets / braces . But it is not in the right order.
Javascript For Journalists Tutorial Berkeley Advanced
Your Guide To Acing Javascript Interviews Better
Can You Answer This Question With Python The First Chegg Com
Sublime Text Identation On Square Brackets Javascript
Review Of Brackets Code Editor Webnots
Cannot Disable Auto Insert Pair Brackets Rider Support
How To Make Vscode Stop Overriding Closing Parentheses On
Java Balanced Expressions Check Stack Overflow
Javascript Check Valid Parentheses With A Stack By Bahay
Python Read Amp Write To Files Learn How To Open Load Read
Top 10 Visual Studio Code Extensions For Node Js Okta Developer
Length Of The Longest Valid Substring Geeksforgeeks
Javascript Check Valid Parentheses With A Stack By Bahay
Everything You Need To Know About Regular Expressions By
How To Check If Object Is Empty In Javascript Samanthaming Com
Top 25 Useful And Free Brackets Extensions For 2021
Xss Filter Evasion Cheat Sheet Owasp
Vs Code Tips The Auto Closing Delete Setting
Solving Balanced Brackets In Javascript With Stacks By Noam
Show Opening Bracket Line When Closing Bracket Is Selected
React For Beginners A React Js Handbook For Front End
Solving Balanced Brackets In Javascript With Stacks By Noam
Check For Balanced Brackets In An Expression Well Formedness
0 Response to "26 Check Opening And Closing Brackets Javascript"
Post a Comment