33 If Object Is Empty Javascript
There are multiple ways we can check if an object is empty in javascript which depends on the requirement and ease of use. But in my opinion Object.keys ({}).length is the best approach to check the object is empty. How to check if object is empty in javascript Table of content : Object.keys and the constructor Property. We can combine the Object.keys method and the constructor property to check if an object is an empty object. obj makes sure that obj isn't null or undefined . Object.keys (obj).length === 0 checks that the number of keys in obj is 0. Object.keys returns array of non-inherited keys of an object.
Is Object Empty Js Es7 Code Example
24/3/2009 · var myObject = {}; // Object is empty var isEmpty = isObjectEmpty(myObject); // will return true; // populating the object myObject = {"name":"John Smith","Address":"Kochi, Kerala"}; // check if the object is empty isEmpty = isObjectEmpty(myObject); // will return false;
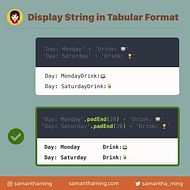
If object is empty javascript. To check whether a JavaScript Object is empty or not, you can check whether the Object variable has enumerable key names or not by using the Object.keys () method. When you have no defined property names, the Object.keys () method will return an empty Array as follows: Here's a Code Recipe to check if an object is empty or not. For newer browsers, you can use plain vanilla JS and use the new "Object.keys" 🍦 But for older browser support, you can install the Lodash library and use their "isEmpty" method 🤖 const empty = {}; Object.keys(empty).length === 0 && empty.constructor === Object _.isEmpty(empty) The JSON.stringify method is used to convert a JavaScript object to a JSON string. So we can use it to convert an object to a string, and we can compare the result with {} to check if the given object is empty. Let's go through the following example. 1
If you need to check specifically for an empty string over null, I would think checking against ""is your best bet, using the ===operator(so that you know that it is, in fact, a string you're comparing against). if (strValue === "") { javascript - check if object is empty . I am trying to create to javascript/jquery test to check if my object is empty and cannot figure it out. ... If you are using lodash library, you have an elegant way to check an empty object, array, map or a set. I presume you are aware of ES6 Import statement. By combining the use of the length property and the logical "not" operator in JavaScript, the "!" symbol, we can check if an array is empty or not. The ! operator negates an expression. That is, we can use it to return true if an array is empty.
With JavaScript, it can be difficult to check whether an object is empty. With Arrays, you can easily check with myArray.length, but on the other hand, objects do not work that way. The best way to check if an object is empty is by using a utility function like the one below. In javascript, we can check if an object is empty or not by using. JSON.stringify. Object.keys (ECMA 5+) Object.entries (ECMA 7+) And if you are using any third party libraries like jquery, lodash, Underscore etc you can use their existing methods for checking javascript empty object. A more proper ES5 solution would be Object.getOwnPropertyNames(obj).length == 0 because Object.keys only gets the enumerable properties. In ES5 we have the power of defining property attributes, we could have an object with non-enumerable properties and Object.keys will just return an empty array...Object.getOwnPropertyNames returns an array containing all the own properties of an object, even ...
Object.getOwnPropertyNames method returns all properties found inside an object. We can use this method to get the list of properties and check if any property exists or not. If not, it is an empty object. To check if an object is empty, we can use the keys() method available in the global Object object and then use the length property in the array returned from the method in JavaScript. TL;DR // empty object const emptyObj = {}; // get the number of keys // present in the object // using the Object.keys() method // then use the length property ... Here are 4 methods to check whether a JavaScript object is an empty object, meaning it is empty of object keys (object properties). Check the.length of an Array of Object.keys () Check the.length of Object.getOwnPropertyNames () Use a for...in loop with.hasOwnProperty ()
Checks whether a string is empty. JavaScript and XPages reference. This reference describes the JavaScript™ language elements, Application Programming Interfaces (APIs), and other artifacts that you need to create scripts, plus the XPages simple actions. Another use case for establishing if an object is empty is using objects as a dictionary (or map). We sometimes need to check if the object is empty to know if there are any items in our dictionary. We can use Object.keys (myDict).length to get the size of the dictionary and check if it’s empty (=== 0). In this quick tip, you'll learn how to check if an Object is empty in JavaScript. Now, if an Object is empty it won't be having any keys. So, the simplest method to check if an object is empty is to check if it has any keys or not.
23/7/2021 · JavaScript check if object is empty. To check if the Object is empty in JavaScript, use the Object.keys() method with an additional constructor. The Object.keys() is a built-in JavaScript function that returns an array of a given object’s own enumerable property names. With JavaScript, it can be difficult to check whether an object is empty. With Arrays, you can easily check with myArray.length, but on the other hand, objects do not work that way. The best way to check if an object is empty is by using a utility function like the one below. 25/2/2020 · If we pass an object to the Object.getOwnPropertyNames () method it returns an array of object properties.so that by using the length of that array we can easily check if the given object is empty or not. Similarly, we can use the Object.keys () or Object.values () method. The Object.keys () method returns an array with object keys.
Use hasOwnProperty () to Check if an Object Is Empty in JavaScript We can check if the specified property is present in the object or not. In the example below, we are checking two objects for the presence of the prop property. The isObjEmpty () function returns the boolean command if the object is empty. JavaScript has no built-in.length or.isEmpty methods for objects to check if they are empty. So we have to create our own utility method or use 3rd-party libraries like jQuery or Lodash to check if an object has own properties. Here are some of the methods you can use to make sure that a given object does not have its own properties: If it returns an empty array, it means the object does not have any enumerable property, which in turn means it is empty. Object. entries ( objectToCheck ). length === 0 You should also make sure the object is actually an object, by checking its constructor is the Object object:
javascript check empty object; how to check if object is empty javascript; js check for class in classList; javascript hass class; check if object is empty javascript; javascript check if number; NaN; jquery check if empty object; javascript isset; express check if object is empty; javascript typeof undfined; javascript check if array is empty A plain and simple JavaScript object, initialized without any keys or values. Empty. There are multiple ways to check if the person object is empty, in JavaScript, depending on which version you are using. ES6 is the most common version of JavaScript today, so let's start there. ES6 provides us with the handy Object.keys function: Object.keys will return an Array, which contains the property names of the object. If the length of the array is 0, then we know that the object is empty. function isEmpty (obj) { return Object.keys (obj).length === 0; } we can also check this using Object.values and Object.entries. This is the simplest way to check if an object is empty.
By default, JavaScript provides a property called length which could let you know if there are values inside the array, but when it comes to objects JavaScript doesn't provide any feature to determine if an object is empty. 27/1/2021 · In Javascript, all arrays are objects (try console.log(typeof [])), so they also return true, regardless of whether or not they are empty. To check if any array is empty: if (MYARRAY.length === 0) { // console.log(true); } To check if an object is empty: if (Object.keys(MYOBJECT).length === 0) { // console.log(true); } *If of an empty list will give true (if (array1)). * x.prop does not really add any elements to the array, it just assigns value "hello" to property x of the array but the array is still empty. So you should still use.length to check is array is empty.
Method 1: Using the Object.keys(object) method: The required object could be passed to the Object.keys(object) method which will return the keys in the object. The length property is used to the result to check the number of keys. If the length property returns 0 keys, it means that the object is empty.
How To Check If A Javascript Object Property Is Undefined
10 Ways To Check If An Object Is Empty In Javascript By
How To Check If Object Is Empty In Javascript Samanthaming Com
8 Ways To Check If An Object Is Empty Or Not In Javascript
React Js Check If Array Or Object Is Empty Tutorial Tuts Make
Different Ways To Check If An Object Is Empty In Javascript
Nested Object Equals Check Js Code Example
How To Check An Object Is Empty Using Javascript Geeksforgeeks
Check If Object Is Empty In Javascript First Class Js
How To Check If Object Is Empty In Javascript Samanthaming Com
How To Access Object S Keys Values And Entries In Javascript
How To Check If Object Is Empty In Javascript Samanthaming Com
Different Ways To Check If An Object Is Empty In Javascript
How To Test For An Empty Object In Javascript
Different Ways To Check If An Object Is Empty In Javascript
How To Check If An Object Is Empty Or Not In Javascript
The Toolbar Variable In Toolbar Js Is Set To An Empty Jquery
How To Remove A Property From A Javascript Object
Different Ways To Check If Object Is Empty Or Not Dev Community
How To Check If An Object Is Empty In Javascript
How Can I Check For An Empty Undefined Null String In
Lodash Check For Empty Array In An Array Of Objects Using
Typescript Empty Object For A Typed Variable Stack Overflow
Different Ways To Check If Object Is Empty Or Not Dev Community
10 Ways To Check If An Object Is Empty In Javascript By
How To Check If A Javascript Object Is Empty Of Properties
Javarevisited Jsp How To Check If Arraylist Is Empty Using
How To Check If A Javascript Array Is Empty Or Not With Length
How To Check If Array Includes A Value In Javascript
How To Check If An Object Has A Property Properly In
Javascript Check If Object Is Empty Pakainfo
0 Response to "33 If Object Is Empty Javascript"
Post a Comment