35 Javascript Array Insert First
Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array #2 unshift - Insert an element at the beginning of the array #3 spread operator - Adding elements to an array using the new ES6 spread operator The first and probably the most common JavaScript array method you will encounter is push (). The push () method is used for adding an element to the end of an array. Let's say you have an array of elements, each element being a string representing a task you need to accomplish.
Powershell Array Guide How To Use And Create
Definition and Usage The unshift () method adds new items to the beginning of an array, and returns the new length. unshift () overwrites the original array. Tip: To add new items at the end of an array, use push ().
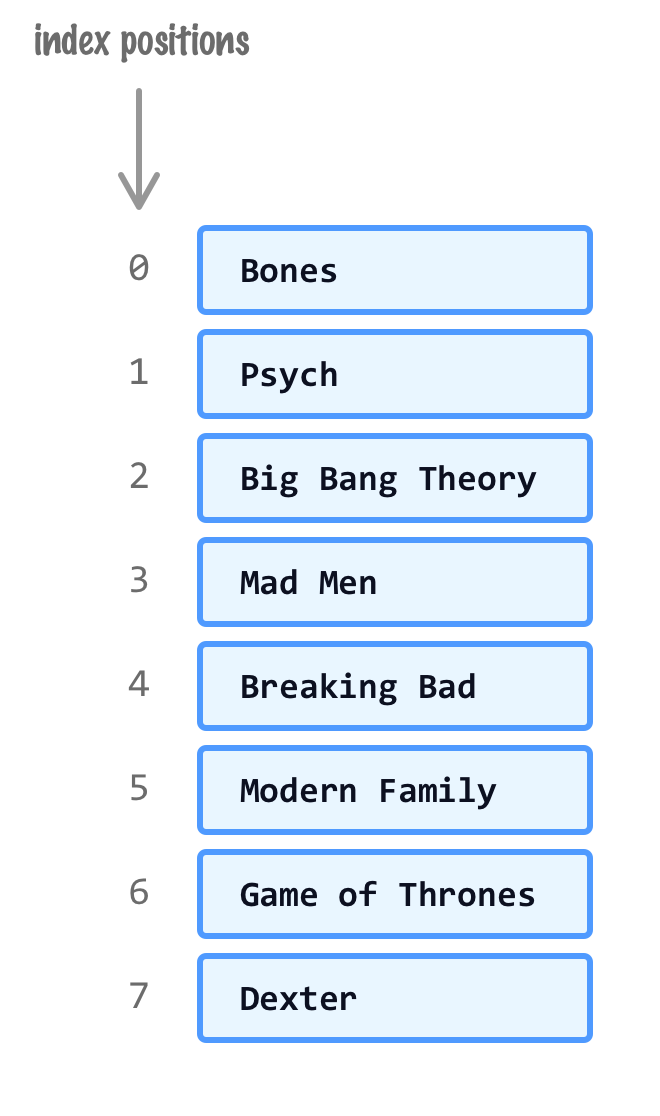
Javascript array insert first. To insert element into JavaScript array, splice method should be used. For inserting first parameter should be index before which new element will be inserted. Indexes are counted from zero. Second parameter should be 0 as we do not remove element. The splice method must be called on array ... The JavaScript Array.splice () method is used to change the contents of an array by removing existing elements and optionally adding new elements. This method accepts three parameters. The first parameter determines the index at which you want to insert the new element or elements. Does Javascript have any built-in functionality that does this? The complexity of my method is O(n) and it would be really interesting to see better implementations. ... FYI: If you need to continuously insert an element at the beginning of an array, it is faster to use push statements followed ...
Dec 18, 2020 - The unshift() method adds one or more elements to the beginning of an array and returns the new length of the array. An element inside an array can be of a different type, such as string, boolean, object, and even other arrays. So, it means that you can create an array having a string in the first position, a number in the second, and more. javascript array variable javascript objects Do you find this helpful? You can use the unshift() method to easily add new elements or values at the beginning of an array in JavaScript. This method is a counterpart of the push() method, which adds the elements at the end of an array. However, both method returns the new length of the array.
Aug 02, 2020 - Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript 3230. Use unshift. It's like push, except it adds elements to the beginning of the array instead of the end. unshift/push- add an element to the beginning/end of an array. shift/pop- remove and return the first/last element of an array. Adding Elements to the Start of an Array The unshift () method in array objects adds one or more elements to the start of an array. When executed, it also returns the new length of an array:
Method 1: push () method of Array. The push () method is used to add one or multiple elements to the end of an array. It returns the new length of the array formed. An object can be inserted by passing the object as a parameter to this method. The object is hence added to the end of the array. Pop, Push, Shift and Unshift Array Methods in JavaScript JavaScript gives us four methods to add or remove items from the beginning or end of arrays: pop() : Remove an item from the end of an array Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript
JavaScript arrays are zero-indexed. The first element of an array is at index 0, and the last element is at the index value equal to the value of the array's length property minus 1. Using an invalid index number returns undefined. On the first run, sum is the initial value (the last argument of reduce), equals 0, and current is the first array element, equals 1. So the function result is 1. On the second run, sum = 1, we add the second array element (2) to it and return. On the 3rd run, sum = 3 and we add one more element to it, and so on… The calculation flow: Get JavaScript: The Definitive Guide, 5th Edition now with O’Reilly online learning. O’Reilly members experience live online training, plus books, videos, and digital content from 200+ publishers. ... One or more values that are inserted at the start of array.
JavaScript | Add new elements at the beginning of an array Last Updated : 01 Apr, 2019 Adding new elements at the beginning of existing array can be done by using Array unshift () method. This method is similar to push () method but it adds element at the beginning of the array. JavaScript has a buit in array constructor new Array (). But you can safely use [] instead. These two different statements both create a new empty array named points: const points = new Array (); Aug 23, 2019 - var colors = ["white","blue"]; colors.unshift("red"); //add red to beginning of colors // colors = ["red","white","blue"]
push () / pop () — add/remove elements from the end of the array unshift () / shift () — add/remove elements from the beginning of the array concat () — returns a new array comprised of this array joined with other array (s) and/or value (s) Found a problem with this page? Nov 04, 2019 - The elements you want to add to the array (beginning from the start point) In our code example, we gave both the first and second parameter a 0 value. By giving those items a value of 0, we're telling the splice() method to insert our element at the beginning of the originalArray we provided. The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length.
Apr 02, 2021 - Future Studio provides on-demand learning & wants you to become a better Android (Retrofit, Gson, Glide, Picasso) and Node.js/hapi developer! Create an array styles with items "Jazz" and "Blues". Append "Rock-n-Roll" to the end. Replace the value in the middle by "Classics". Your code for finding the middle value should work for any arrays with odd length. Strip off the first value of the array and show it. Prepend Rap and Reggae to the array. The array in the process: Deleting elements using JavaScript Array's splice () method. To delete elements in an array, you pass two arguments into the splice () method as follows: Array .splice (position,num); Code language: JavaScript (javascript) The position specifies the position of the first item to delete and the num argument determines the number of elements to ...
If you aren't adverse to extending natives in JavaScript, you could add this method to the Array prototype: Array. prototype. insert = function ( index, item) { this.splice( index, 0, item); }; I've tinkered around quite a bit with arrays, as you may have noticed: Remove an Item From an Array. Clone Arrays. How to use JavaScript push, concat, unshift, and splice methods to add elements to the end, beginning, and middle of an array. HTML Javascript Programming Scripts Adding an element to an array can be done using different functions for different positions. Adding an element at the end of the array This can be accomplished using the push method.
Behind the scenes, the Map.set just insert elements into an array (take a look at DecentHashMap.set). So, similar to Array.push we have that: Insert an element in HashMap runtime is O(1). If rehash is needed, then it will take O(n) Our implementation with rehash functionality will keep collisions to the minimum. 15 Common Operations on Arrays in JavaScript (Cheatsheet) The array is a widely used data structure in JavaScript. The number of operations you can perform on arrays (iteration, inserting items, removing items, etc) is big. The array object provides a decent number of useful methods like array.forEach (), array.map () and more. Say you want to add an item at the beginning of an array. To perform this operation you will use the splice() method of an array. splice() takes 3 or more arguments. The first is the start index: the place where we'll start making the changes. The second is the delete count parameter. We're adding to the array, so the delete count is 0 in all our examples.
20/12/2019 · 1. JavaScript Add Element to Array First. You can use the javascript unshift() method to add elements or items first or beginning array in javascript. unshift() method javascript. Definition:-The javaScript unshift() method adds new items to the first or beginning of an array. and it will return the new length of array. Syntax of unshift() method is There are two ways to create an array in JavaScript: The array literal, which uses square brackets. The array constructor, which uses the new keyword. Let's demonstrate how to create an array of shark species using the array literal, which is initialized with []. An array starts from index 0,So if we want to add an element as first element of the array, then the index of the element is 0.If we want to add an element to nth position, then the index is (n-1) th index.
I notice that you wish to keep the array the same length as the original, even if the supplied item is prepended to the array. You also speak like scaling is going to be an issue, so I will add some tests that will use a reasonably large data set. For that purpose, I decided to write this article about some of the best methods we can use to add and remove elements in arrays in Javascript. In this article we'll be covering push , pop , shift , unshift , concat , splice , slice and also on how to use the ES6 spread operator for javascript arrays. Array indexes start from 0, so if you want to add the item first, you'll use index 0, in the second place the index is 1, and so on. To perform this operation you will use the splice () method of an array. This function is very powerful and in addition to the use we're going to make now, it also allows to delete items from an array.
6 days ago - Pop Push Shift and Unshift Array Methods in JavaScript. We will see pop, push, shift, and unshift method one by one in Javascript Node.js with example. The first argument is the index of an array where you want to add an element. The second argument is the number of elements that you want to remove from the index element. The third argument is the element that you want to add to the array. Example 3: Add Element to Array Using Spread Operator
Data Structures In Javascript Arrays Hashmaps And Lists
Javascript Array Methods Cheat Sheet Part 1 Therichpost
Javascript Array Remove Element From Array Tuts Make
Javascript Array A Complete Guide For Beginners Dataflair
C Program To Insert An Element In An Array
How To Use Bash Array In A Shell Script Scripting Tutorial
Insert Data At First Position In Array Javascript
How To Append Element To Array In Javascript Tecadmin
Creating Indexing Modifying Looping Through Javascript
How To Populate Html Table From Array Using Javascript With
Javascript Tutorial Adding Elements To Array In Javascript
A Quick Guide To Queues In Javascript By Kenneth Young
How To Insert An Element At A Specific Position In An Array
How To Remove Array Duplicates In Es6 By Samantha Ming
Dynamic Array Data Structure Interview Cake
Arrays In Python What Are Python Arrays Amp How To Use Them
Implementing A Javascript Stack Using Push Amp Pop Methods Of
Let S Get Those Javascript Arrays To Work Fast Gamealchemist
Removing Items From An Array In Javascript Ultimate Courses
Some Powerful Things You Can Do With The Array Splice Method
How Can I Add New Array Elements At The Beginning Of An Array
Understanding Array Splice In Javascript Mastering Js
How To Add An Item At The Beginning Of An Array In Javascript
How To Insert An Item Into Array At Specific Index In
Java67 How To Copy Array In Java Arrays Copyof And
Queue Abstract Data Type Wikipedia
Picking A Random Item From An Array Kirupa Com
How Can I Add New Array Elements At The Beginning Of An Array
Program To Insert An Element In An Array C Programs
Javascript Array Distinct Ever Wanted To Get Distinct
5 Working With Arrays And Loops Javascript Cookbook Book
0 Response to "35 Javascript Array Insert First"
Post a Comment