31 Javascript Array Map Get Index
The .map() method creates a new array with the result of executing a function on individual items in an array. Dec 16, 2020 - Idx simply receives the index of the current item in the iterated array that is being processed. The final argument, array, is the array we are looping through, and is rarely used. Note: manipulating this.Arg allows the this context to be changed. ... A common usage of the map() method in JavaScript ...
Javascript Array Map Method Geeksforgeeks
Sep 21, 2018 - Explore a greet collection of fancy tutorials for mobile and web development using the most evolved technologies
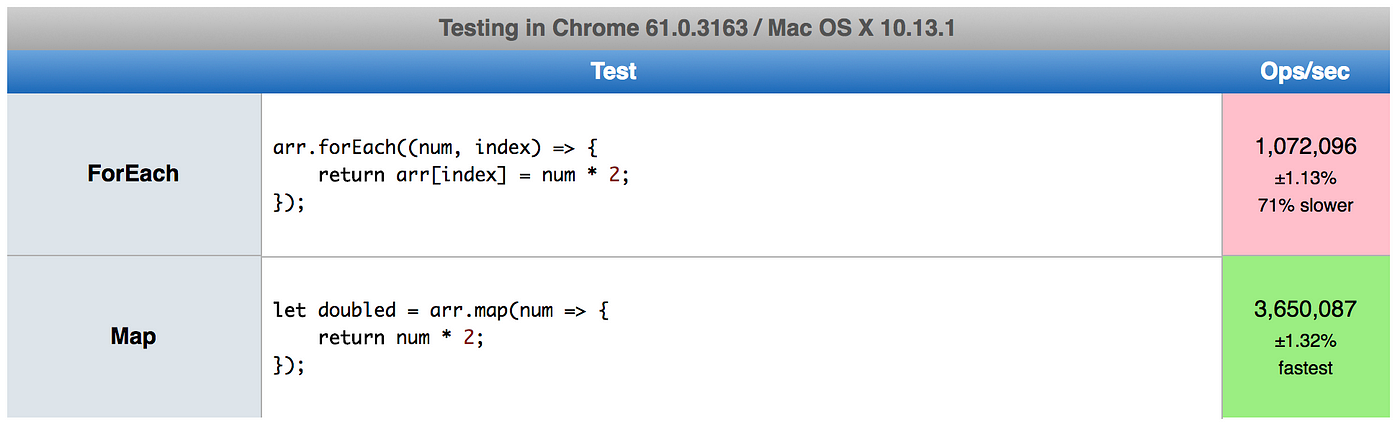
Javascript array map get index. Since JavaScript returns undefined from our spreading array at each of its indices (remember, it does this by default because it doesn't have the index key for that value), we end up with a new array that's actually filled with index keys, and therefore map-able (and reduce-able, filter-able, and forEach-able). index will be -1 which means the item was not found. Because objects are compared by reference, not by their values (differently for primitive types). The object passed to indexOf is a completely different object than the second item in the array.. You can use the findIndex value like this, which runs a function for each item in the array, which is passed the element, and its index. Code language: JSON / JSON with Comments (json) How it works. First, define a function that calculates the area of a circle. Then, pass the circleArea function to the map() method. The map() method will call the circleArea function on each element of the circles array and return a new array with the elements that have been transformed.; To make it shorter, you can pass in the map() method an ...
The array map was called upon. The map function takes a callback function as argument (and not an index as argument, as originally stated in the question before it was edited). The callback function has an index as a parameter - the callback is called automatically, so you don't provide the index yourself. Aug 10, 2020 - One of the most popular methods of iterating through datasets in JavaScript is the .map() method. .map() creates an array from calling a specific function on each item in the parent array. .map() is a non-mutating method that creates a new array inste 22/11/2019 · In JavaScript, map () method handles array elements, which creates a new array with the help of results obtained from calling function for each and every array element in an array. The index is used inside map () method to state the position of each element in an array, but it doesn’t change the original array.
Returns a new Array Iterator that contains the keys for each index in the array. Array.prototype.lastIndexOf() Returns the last (greatest) index of an element within the array equal to an element, or -1 if none is found. Array.prototype.map() Returns a new array containing the results of calling a function on every element in this array. 30/7/2020 · JavaScript Map Index To find index of the current iterable inside the JavaScript map () function, you have to use the callback function’s second parameter. An index used inside the JavaScript map () method is to state the position of each item in an array, but it doesn’t modify the original array. 13/7/2016 · Other arguments of Array.prototype.map():. The third argument of the callback function exposes the array on which map was called upon; The second argument of Array.map() is a object which will be the this value for the callback function. Keep in mind that you have to use the regular function keyword in order to declare the callback since an arrow function doesn't have its own binding …
The first is an expression and the second is the radix to the callback function, Array.prototype.map passes 3 arguments: the element; the index; the array; The third argument is ignored by parseInt—but not the second one! This is the source of possible confusion. Here is a concise example of the iteration steps: javascript create variable containing an object that will contain three properties that store the length of each side of the box · convert the following 2 d array into 1 d array in javascript Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready Click on the map to add markers Continue this until left side element is greater than or equal to the pivot element. Javascript array map index. If we had used index as key instead, then we'd have got :0, :1, :2 as ...
The map () method in JavaScript creates an array by calling a specific function on each element present in the parent array. It is a non-mutating method. Generally map () method is used to iterate over an array and calling function on every element of array. Syntax: array.map (function (currentValue, index, arr), thisValue) The indexOf () method searches an array for a specified item and returns its position. The search will start at the specified position (at 0 if no start position is specified), and end the search at the end of the array. indexOf () returns -1 if the item is not found. Map.prototype.get () The get () method returns a specified element from a Map object. If the value that is associated to the provided key is an object, then you will get a reference to that object and any change made to that object will effectively modify it inside the Map object.
It runs each element through an iteratee function and returns an array with the results. The synchronous version that adds one to each element: const arr = [1, 2, 3]; const syncRes = arr.map( (i) => { return i + 1; }); console.log(syncRes); // 2,3,4. An async version needs to do two things. First, it needs to map every item to a Promise with ... Definition and Usage. The map () method creates a new array with the results of calling a function for every array element. The map () method calls the provided function once for each element in an array, in order. map () does not execute the function for empty elements. map () does not change the original array. Hashmaps offer the same key/value functionality and come native in JavaScript (ES6) in the form of the Map() object (not to be confused with Array.prototype.map()).
Given a string S and a character ... the character C in the string. javascript ... Write a recursive function flattenRecursively that flattens a nested array. Your function should be able to handle varying levels of nesting. ... GetAll(Expression<Func<T, bool>> filter = null, ... 28/5/2020 · The Array.prototype.findIndex () method returns an index in the array if an element in the array satisfies the provided testing function; otherwise, it will return -1, which indicates that no element passed the test. It executes the callback function once for every index in the array until it finds the one where callback returns true. The syntax of JavaScript map method is as below : let finalArray = arr.map(callback( currentValue[, index[, array]]), arg) Following are the meanings of these parameters : callback: This is the callback method. It is called for each of the array arr elements. The returned value of this method is added to the final array finalArray.
This method returns the index of first element in array which satisfies the condition. If this method finds an array element for which the function returns a true value, this method returns the index of that array element and stops, Otherwise it returns -1. array: The array object to which the current element belongs to. map () method: This method is used to apply a function on every element in an array and returns a new array of. same size as the input array. Syntax: let newArray = oldArray.map ( (currentValue, index, array) { // Returns element to new Array }); Used Parameters and variables: Array.prototype.findIndex () The findIndex () method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1, indicating that no element passed the test. See also the find () method, which returns the value of an array element, instead of its index.
Get The Current Array Index in JavaScript forEach () JavaScript's forEach () function takes a callback as a parameter, and calls that callback for each element of the array: The first parameter to the callback is the array value. The 2nd parameter is the array index. That's the current position in the array the forEach () loop is at. Sep 23, 2020 - The fact that we don’t have to ... can operate directly on the item instead of having to index into an array makes things more readable. Using a forEach function solves both of these problems for us. But Javascript map() function still has at least two distinct advant... Cú pháp var new_array = arr.map (function callback (currentValue [, index [, array]]) { // Trả về element mới cho new_array } [, thisArg ])
To get the index in a for-of loop, we need to call the array with a entries() method and use destructuring syntax. The findIndex () method returns the index of the first array element that passes a test (provided by a function). The method executes the function once for each element present in the array: Array .lastIndexOf (searchElement [, fromIndex = Array .length - 1 ]) Code language: JavaScript (javascript) The lastIndexOf () method returns the index of the last occurrence of the searchElement in the array. It returns -1 if it cannot find the element. Different from the indexOf () method, the lastIndexOf () method searches for the element ...
Mar 31, 2021 - The callback function() is called on each array element, and the map() method always passes the current element, the index of the current element, and the whole array object to it. May 29, 2020 - Given a string S and a character ... the character C in the string. javascript ... Write a recursive function flattenRecursively that flattens a nested array. Your function should be able to handle varying levels of nesting. ... GetAll(Expression<Func<T, bool>> filter = null, ... Map () is one of the utilities present in the JavaScript libraries to build an array by calling out a given operation to be performed on every element on the existing array. The method is called for every element of the array and the result of the operation is mapped to the corresponding index in the new array.
Dec 06, 2019 - The function map() in Javascript ES6. This useful array method creates a new array with the results of calling a provided function on every element in the calling array.. Nov 23, 2020 - The map and reduce methods offer a modified version of an array or reduced value using callback functions. Read on as we explore the map and reduce methods in JavaScript. The Array.prototype.map() method was introduced in ES6 (ECMAScript 2015) for iterating and manipulating elements of an array in one go. This method creates a new array by executing the given function for each element in the array. The Array.map() method accepts a callback function as a parameter that you want to invoke for each item in the array. This function must return a value after ...
The Map.get () method in JavaScript is used for returning a specific element among all the elements which are present in a map. The Map.get () method takes the key of the element to be returned as an argument and returns the element which is associated with the specified key passed as an argument. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Taking our example array of [1, 2, 3], if we run [1, 2, 3].map((value, index) => index) we'll see our callback get run with 0 the first time, 1 the second time, and 2 on the final time. This second argument is extremely useful if we're trying to use map to generate data or if we need to use the index to access a corresponding item in a ...
Mar 24, 2020 - Follow along with the Exploring JavaScript Array Methods series! Exploring Array ForEach Exploring Array Map (you’re here) Exploring Array Filter Exploring Array Reduce Exploring Array...
Javascript Array Methods How To Use Map And Reduce
How To Implement A Hash Map In Javascript By Jake Zhang
Javascript Array Map Iterate Array Element Tuts Make
Javascript Array Find A Pair Of Elements From An Specified
Working With Iteration Methods In Javascript Arrays
All About Javascript Arrays In 1 Article By Rajesh Pillai
Javascript Map Amp Filter Dev Community
How To Use The Javascript Findindex Array Method
Index Inside Map Function Geeksforgeeks
Hacks For Creating Javascript Arrays
Javascript Array Map Index Example
Javascript Map Method Exercise Learnjavascript
How To Get The Index Of An Item In A Javascript Array
Why Using An Index As Key In React Is Probably A Bad Idea
Replace An Item In An Array By Number Without Mutation In
Hacks For Creating Javascript Arrays
How To Get The Index Of An Iteration In A For Of Loop In
Javascript Map Vs Foreach What S The Difference Between
Javascript Array And Its Functions Map Reduce And
How To Use The Ruby Map Method With Examples Rubyguides
Remove Object From An Array Of Objects In Javascript
Should You Use Includes Or Filter To Check If An Array
How To Check If Array Includes A Value In Javascript
What Is Javascript Map A 5 Minute Guide To Javascript Maps
Javascript Fundamental Es6 Syntax Get The Index Of The
Javascript Map Vs Foreach What S The Difference Between
How To Get The Index Of The Clicked Array Element Using
0 Response to "31 Javascript Array Map Get Index"
Post a Comment