23 Create Rest Api Javascript
When the Create Example API popup appears, choose OK . If this is not your first time using API Gateway, choose Create API. Under REST API, choose Build . Under Create new API, choose Example API and then choose Import to create the example API. For your first API, the API Gateway console starts with this option as default. The login part cannot be done in the REST API because logins with OAuth are interactive, you'll have to build a small server-side web application that perfoms the login. Once the authentication process succeeded the server can pass a username (or email address) and an access token to the JS client app that identify this user.
Creating A Rest Api Happy Coding
Express.js, Go, and Flask are probably your best bets out of the 37 options considered. "Can make use of a great number of plugins" is the primary reason people pick Express.js over the competition. This page is powered by a knowledgeable community that helps you make an informed decision.
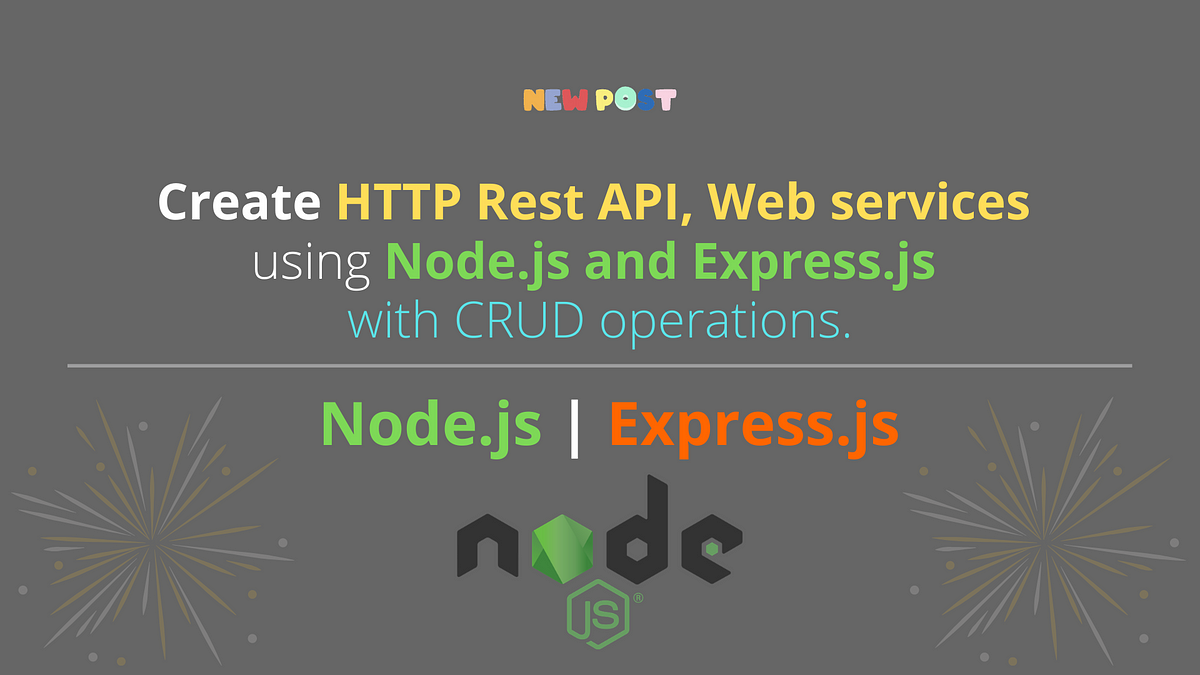
Create rest api javascript. On confirmation, the REST API should save the new training details into the database. Let's start with Visual Studio to create a new web service (REST) application using .NET Core. 1. Open VS, go to File > New and select Project > ASP .NET Core Web Application. Select the solution name (e.g. Training service) and the location of your local ... Web services based on REST Architecture are known as RESTful web services. These webservices uses HTTP methods to implement the concept of REST architecture. A RESTful web service usually defines a URI, Uniform Resource Identifier a service, which provides resource representation such as JSON and set of HTTP Methods. Creating RESTful for A Library Introducing the JavaScript Fetch Library The easiest way to call a REST API in JavaScript is to use the fetch library. It is built into the standard JavaScript library and can be used to make all sorts of HTTP calls.
Now, when consuming the REST API as a client (e.g. cURL, browser, or also a React.js application), you could retrieve all users from the database with a HTTP GET method on the /users URI or, on the same resource, create a new user with a HTTP POST method. The whole process of content rendering from frontend content to JavaScript or other programming languages that are relatable to frontend is provided by the WordPress REST API, which provides us ... Creating your own RESTful API can be a great way to build a business around data you've collected or a service you've created, or it can just be a fun personal project that allows you to learn a new skill.. Here's a list of 20 tutorials on how to design your own REST API!. This list has been sorted by the programming language used in the tutorial, which makes it easy to find the perfect ...
A RESTFula API usually provides a means to do CRUD operations on an object. What is CRUD: CRUD is an acronym and stands for Create, Read, Update, and Delete. It is a way to say "I want to be able to create, read, update, or delete something somewhere" compressed into a single word. Before there was REST there was JSON-RPC: To use the REST capabilities that are built into SharePoint, you construct a RESTful HTTP request, using the OData standard, which corresponds to the client object model API you want to use. Each SharePoint entity is exposed at an endpoint on the SharePoint site that you are targeting, and its metadata is represented in either XML or JSON format. In order to create a simple REST API that might actually be used in practice, we are going to use WordPress API namely the username_exists () function that allow us to check if the username exist in a WordPress database and return true if the username exists or false otherwise.
8/7/2017 · This tutorial introduces the idea of creating a REST API, which is a way of organizing our code so we can access our data from multiple applications. Your REST API is server code whose job it is to provide access to your data and to enforce rules like who can see what. Then other programs use your REST API to interact with your data. JavaScript/AJAX REST API POST Example. To send data to the REST API server using JavaScript/AJAX, you must send an HTTP POST request and include the POST data in the request's body. You also need to provide the Content-Type: application/json and Content-Length request headers. Below is an example of a REST API POST request to a ReqBin REST API ... As you have seen, by creating only db.json file inside the project folder and providing JSON object structure in that file, you can create your own REST API server available live on the internet without even the need for hosting it. You can find the complete source code for this application here.
26/9/2019 · Usually you'll be creating a REST API to standardise and share access to resources, such as a database. For example you might want to allow both a mobile app and a web app to access your database via a REST API. In order to do that of course, you need to know how to use your REST API from within your mobile app or web app! REST Service: the server. There are many popular libraries that make creation of these servers a breeze, like ExpressJS for NodeJS and Django for Python. REST API: this defines the endpoint and methods allowed to access/submit data to the server. We will talk about this in great detail below. https://www.seobility /en/wiki/REST_API. Firstly, create a folder. mkdir cat-api cd cat-api. Create a node.js application using yarn. Add express, cors and mongodb modules into the application. yarn init yarn add express cors mongodb. Create server.js to create an initial API.
In this tutorial, we walk you through seven steps for developing a RESTFul API using popular JavaScript frameworks such as Node.JS and Express.JS. In doing so, we learn how to integrate our API with the MongoDB database. Here are the main steps: 1. Project Initialization. 2. Install Application Dependencies. SharePoint Rest API. We worked on various client object model techniques like CSOM, JSOM, etc. Microsoft also introduced REST (Representational State Transfer) service in SharePoint 2013 which is comparable to existing SharePoint client object models.. By using Rest API, we can interact with SharePoint remotely. We can interact to with SharePoint data by using any technology that supports REST ... Select the ASP.NET Core Web API template and click Next. Name the project TodoApi and click Create. In the Create a new ASP.NET Core Web Application dialog, confirm that.NET Core and ASP.NET Core 5.0 are selected. Select the API template and click Create.
Now, this is it for this tutorial where we create or define our first Rest API endpoint in Node Js. In the next tutorial, we will integrate Mongo Database with our Node Application. Source code from this tutorial is available on GitHub. Hope you guys like the tutorial, feel free to drop any comments in the comment section below. CRUD is a programming concept denoting four basic actions (create, read, update, and delete) that can be performed on a data source. In a REST API, these actions correspond to Types of Requests or Request Methods: POST: Create action. Adds new data to the server. Using this type of request, you can, for example, add a new ticket to your inventory. Jira versions earlier than 8.4. Creating an issue using the Jira REST API is as simple as making a POST with a JSON document. To create an issue, you will need to know certain key metadata, like the ID of the project that the issue will be created in, or the ID of the issue type.
We can create a SharePoint site using Rest API in SharePoint Online Office 365. This will also work fine with SharePoint 2013 and SharePoint 2016. Here we have taken two textboxes where the user can put the SharePoint site name and the site description and a submit button. On click of that, the SharePoint site will get created. Calling Google News RESTful Web Service with JavaScript / jQuery. This guide walks you through writing a simple javascript/jquery to calling rest web service. When we call Google News API and web service with the help of javascript and jquery then we will get the JSON response given below. "description": "The number of people killed in China by ... 16/6/2021 · Now we will start with making api endpoints. 1. Firstly we have to make schema of the type of data that we want in our API, we will make the schema for products in the shop. Make a folder in the main directory names models and make a file named Products.js and the following code.
1/5/2016 · Then the arguments can be inserted into input type=hiddenvalue fields using JavaScript and the form can be submitted from the button click event listener or onclick event using one line of JavaScript. Here is an example that assumes the REST API is in file REST.php: <body><h2>REST-test</h2><input type=button onclick="document.getElementById('a'). Creating a REST API Backend using Node.js, Express and Postgres Last Updated : 28 Jul, 2020 JavaScript Backend can be developed using Node.js, Express, and Postgres. This backend can do Query operations on the PostgreSQL database and provide the status or data on the REST API. It is a new, improved programming model with Dependency Injection and new concepts such as Components, Mixins, Repositories, etc., which make this the most extensible. LoopBack allows us to create a GraphQL interface for any REST API using OpenAPI-to-GraphQL.
This is not meant to be an extensive resource on APIs or REST - just the simplest possible example to get up and running that you can build from in the future. We'll learn: What a Web API is. Learn how to use the HTTP request GET with JavaScript; How create and display HTML elements with JavaScript. It will look like this: Let's get started ...
Woocommerce Rest Api Woocommerce Docs
How To Use An Api With Javascript Beginner S Guide
Best Practices For Rest Api Design Stack Overflow Blog
Create A Crud Rest Api With Node And Express Js By Ankit
Create A Graphql Api Wrapper For Your Rest Api By John Fay
Creating A Basic Rest Api With Node Js From Scratch By
8 Create Rest Api Endpoint In Node Js Test With Postman
All Possible Ways Of Making An Api Call In Javascript By
Node Js Express Amp Mongodb Build A Crud Rest Api Example
Create And Consume Simple Rest Api In Php All Php Tricks
Rest Api Using Node Js Mongodb And Express
Rest Api Creating A Cube With Initial Data Javascript
Node Js Rest Api To Add Edit And Delete Record From Mysql
Create Rest Api On Node Js Using Visual Studio Dhananjay Kumar
Github Nguy2819 Rest Api Practice Ninjadb Mongodb
Building A Rest Api With Node And Express
Login App Create Rest Api For Authentication In Node Js
How To Create A Secure Rest Api In Node Js
Python Rest Apis With Flask Connexion And Sqlalchemy Real
How To Create Rest Api Archives Tutorialswebsite Learn
Building A Simple Rest Api With Nodejs And Express By
Node Js Express Js And Postgresql Crud Rest Api Example
0 Response to "23 Create Rest Api Javascript"
Post a Comment