31 Javascript Number To String
The toString () method in Javascript is used with the number and converts a number to a string. The toString () function is used to return the string representing the specified Number object. Javascript Int to String To convert Javascript int to string, use the toString () method. Nov 06, 2017 - In JavaScript, you can represent a number is an actual number (ex. 42), or as a string (ex. '42'). If you were to use a strict comparison to compare the two, it would fail because they’re two different types of objects.
C Convert Int To String Javatpoint
Sep 13, 2020 - There are two main ways to convert a string to a number in javascript. One way is to parse it and the other way is to change its type to a Number. All of the tricks in the other answers (e.g. unary plus) involve implicitly coercing the type of the string to a number.
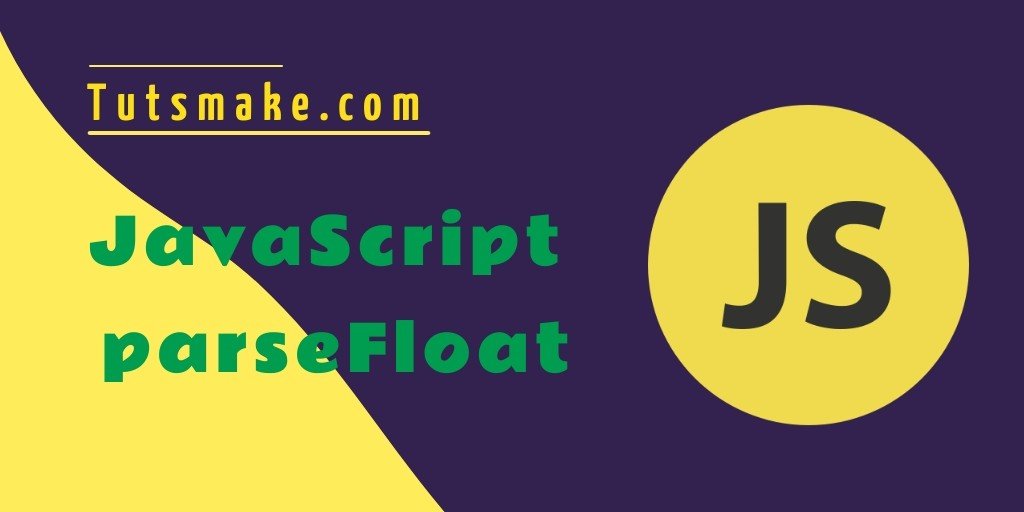
Javascript number to string. Convert a number to string with toString () method You can also call the toString () method immediately on a number value, but you need to add parentheses () to wrap the value or JavaScript will respond with an Invalid or unexpected token error. The toString () method can also convert floating and negative numbers as shown below: Save Your Code. If you click the save button, your code will be saved, and you get a URL you can share with others. May 13, 2019 - In JavaScript, you can represent a number is an actual number 30, or as a string ‘30’.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. toString(base) function converts a given a number to the string. It takes an optional parameter base which can be used to specify the base in which the number will be represented as a string. It works for floating point and exponential numbers also. 17 Jul 2014 — The global method Number() can convert strings to numbers. Strings containing numbers (like "3.14") convert to numbers (like 3.14). Empty ...
Use the Number() Function to Convert a String to a Number in JavaScript. The Number() is a function of the Number construct which can be used to convert other data types to a number format (as per the MDN Docs). It returns NaN if the input parameter is either undefined or not convertible to a number. For instance: 5 days ago - An integer between 2 and 36 that represents the radix (the base in mathematical numeral systems) of the string. If radix is undefined or 0, it is assumed to be 10 except when the number begins with the code unit pairs 0x or 0X, in which case a radix of 16 is assumed. Using the regular expression and match method you can extract phone numbers from strings in JavaScript. Let's take three different patterns of number that you want to search. 10 digits + sign followed by 10 digits
5. valueOf () Method. This method returns the actual content numerical value stored in the variable, literal or expression using which it is called. In javascript, a number can be of type number or object. Internally, javascript uses the valueOf () method to retrieve the primitive valued number. Let us see an example of the same. Jul 17, 2014 - The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript assumes the following: Aug 10, 2017 - Tutorial on JavaScript number to string. Learn to convert int to string with JavaScript. Practice JavaScript number to string here with examples.
javascript check if string is number . javascript by Grepper on Jul 25 2019 Donate Comment . 1. js check if string is integer . javascript by TC5550 on Jun 04 2020 Comment . 0. js check if string is int . javascript by Av3 on Feb ... JavaScript Number - toString(), This method returns a string representing the specified object. The toString() method parses its first argument, and attempts to return a string representation Aug 21, 2020 - A beginner's guide on how to convert a number into a string using JavaScript.
targetLength. The length of the resulting string once the current str has been padded. If the value is less than str.length, then str is returned as-is.. padString Optional. The string to pad the current str with. If padString is too long to stay within the targetLength, it will be truncated from the end.The default value is "" (U+0020 'SPACE'). The .toString () method that belongs to the Number.prototype object, takes an integer or floating point number and converts it into a String type. There are multiple ways of calling this method. If a number ( base) is passed as a parameter to the .toString () method, the number will be parsed and converted to that base number: 16/12/2019 · How to convert a number to a string in JavaScript javascript 1min read To convert a number to a string, we need to use the Number.toString () method in JavaScript. Struggling with JavaScript frameworks?
Use parseFloat () function, which purses a string and returns a floating point number. The argument of parseFloat must be a string or a string expression. The result of parseFloat is the number whose decimal representation was contained in that string (or the number found at the beginning of the string). JavaScript to Convert Numbers Into Words If you want to be able to do these conversions on your site, you will need a JavaScript code that can do the conversion for you. The simplest way to do this is to use the code below; just select the code and copy it into a file called toword.js. // Convert numbers to words JavaScript NaN In JavaScript, NaN (Not a Number) is a keyword that indicates that the value is not a number. Performing arithmetic operations (except +) to numeric value with string results in NaN.
The parseInt function converts its first argument to a string, parses it, and returns an integer or NaN. If not NaN, the returned value will be the integer that is the first argument taken as a number in the specified radix (base). For example, a radix of 10 indicates to convert from a decimal number, 8 octal, 16 hexadecimal, and so on. The difference between the String() method and the String method is that the latter does not do any base conversions. How to Convert a Number to a String in JavaScript¶ The template strings can put a number inside a String which is a valid way of parsing an Integer or Float data type: In JavaScript parseInt() function is used to convert the string to an integer. This function returns an integer of base which is specified in second argument of parseInt() function. parseInt() function returns Nan( not a number) when the string doesn't contain number. Syntax: parseInt(Value, radix)
In JavaScript, toLocaleString () is a Number method that is used to convert a number into a locale-specific numeric representation of the number ( rounding the result where necessary) and return its value as a string. Because toLocaleString () is a method of the Number object, it must be invoked through a particular instance of the Number class. Let's check out the different ways of converting a value to a string in JavaScript. The preferred way from Airbnb's style guide is… 2 weeks ago - When parsing data that has been serialized to JSON, integer values falling outside of this range can be expected to become corrupted when JSON parser coerces them to Number type. A possible workaround is to use String instead.
24/2/2015 · 2. The only valid solution for almost all possible existing and future cases (input is number, null, undefined, Symbol, anything else) is String (x). Do not use 3 ways for simple operation, basing on value type assumptions, like "here I convert definitely number to string and here definitely boolean to string". The toString() method returns a number as a string. All number methods can be used on any type of numbers (literals, variables, or expressions): ... To convert a string to an integer parseInt() function is used in javascript.parseInt() function returns Nan( not a number) when the string doesn't contain number.If a string with a number is sent then only that number will be returned as the output. This function won't accept spaces. If any particular number with spaces is sent then the part of the number that presents before space will be ...
There are 3 methods available in JavaScript to Convert String to Number. Those are, parseInt() parseFloat() Number() Using parseInt() - Convert String to Number in JavaScript. ParseInt() parses a string and returns a whole number. Spaces are allowed. Only the first number is returned. var text = '42px'; var integer = parseInt(text, 10 ... 4 weeks ago - If no signs are found, the algorithm ... number-parsing on the rest of the string. A value passed as the radix argument is coerced to a Number (if necessary), then if the value is 0, NaN or Infinity (undefined is coerced to NaN), JavaScript assumes the following:... Nov 03, 2019 - Managing data is one of the fundamental concepts of programming. Converting a Number to a String is a common and simple operation. The same goes for the other...
Dec 16, 2020 - In JavaScript, you can represent a number as type number (ex. 12), or as a type string (ex. '12').But... Jul 20, 2021 - The toString() method in Javascript is used with a number and converts the number to a string. It is used to return a string representing the specified Number object. The Number object overrides the toString() method of the Object object. (It does not inherit Object.prototype.toString()).For Number objects, the toString() method returns a string representation of the object in the specified radix.. The toString() method parses its first argument, and attempts to return a string representation in the specified radix (base).
Use the toString () Function to Convert an Integer to a String in JavaScript The method object.toString () returns the string. We can take the required number object and use it with this function to convert it into a string. Check the code below. Learn how to convert a string to a number using JavaScript. This takes care of the decimals as well. Number is a wrapper object that can perform many operations. If we use the constructor (new Number("1234")) it returns us a Number object instead of a number value, so pay attention.Watch out for separators between digits: Unary plus method. If you have integer or float values as string then you can also use Unary plus approach in JavaScript to convert string to number. In this approach we use plus sign + before the string and it gives us output as number. For instance, Lets take a look at the examples. console.log (+'123'); // output: 123.
In JavaScript, toPrecision () is a Number method that is used to convert a number to a specified precision ( rounding the result where necessary) and return its value as a string. Because toPrecision () is a method of the Number object, it must be invoked through a particular instance of the Number class. Dec 15, 2020 - Number() can be used to convert JavaScript variables to numbers. We can use it to convert the string too number. If the value cannot be converted to a number, NaN is returned.
Hour 3 Using Javascript In The Mongodb Shell
How To Convert A String To A Number In Javascript
Javascript Datatypes Primitive Vs None Primitive Kamp Blog
How To Work With Date And Time In Javascript Using Date
Extract Number From String Javascript Code Example
How To Convert A String To A Number In Javascript
Hour 3 Using Javascript In The Mongodb Shell
Ways To Convert String To Number In Js Time To Hack
Strings In Javascript Javascript Tutorial By Wideskills
Convert String To Number Array In Javascript With React Hooks
27 String To Number Conversion Javascript Bizanosa
Javascript Parsefloat Convert String To Float Number Tuts Make
How To Convert A String To An Integer In Javascript Stack
What Are The Different Data Types In Javascript Edureka
5 Ways To Convert A Value To String In Javascript By
Convert String To Real Number Knime Analytics Platform
How To Check If A Variable Is An Array In Javascript
Javascript Numbers Get Skilled In The Implementation Of Its
Javascript Convert String To Number
Modified Java Script Value Hitachi Vantara Lumada And
Javascript Add The Number To A String Explanation Amp Example
Javascript Lesson 2 Variables Appending Strings Adding Numbers
Javascript Extract A Specific Number Of Characters From A
How Do You Make A Parameter To Expect A Number Or A String
How To Compare String And Number In Javascript Code Example
How To Convert A String To A Number In Javascript
How To Get Numbers From A String In Javascript
How To Convert String To Number In Javascript Or Typescript
Convert A String To A Number In Javascript Clue Mediator
9 Ways To Convert Strings Into Numbers In Javascript By
0 Response to "31 Javascript Number To String"
Post a Comment