30 How To Clone Html Element Javascript
Watch this video tutorial to see how to create a clone of HTML Element. ... Simple Loading Screen using HTML and CSS Cloning HTML Element using HTML DOM cloneNode Method Change Mouse Cursor using CSS and JavaScript Loading Bar Using Jquery and CSS Mouse Following Eyes using CSS and JavaScript ... Clone the original DOM node recursively. Compute the style for the node and each sub-node and copy it to corresponding clone and don't forget to recreate pseudo-elements, as they are not cloned in any way.
Traversing An Html Table With Javascript And Dom Interfaces
Nov 26, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
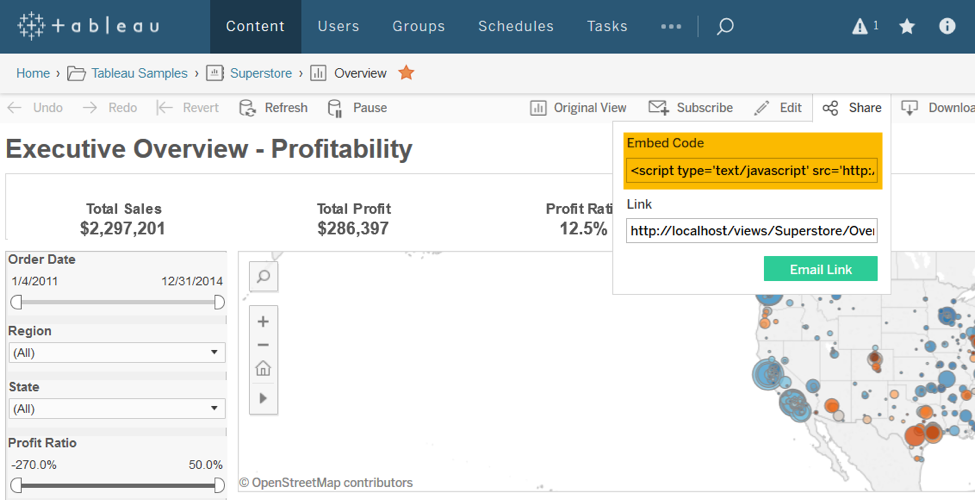
How to clone html element javascript. // Syntax: let newClone = node.cloneNode([deep]) // Example: let p = document.getElementById("para1") let p_prime = p.cloneNode(true) 4. Generate unique id. The .clone() method completely copied the element. The newly created element has the same id as of the selector element.. You will face the problem when you try to retrieve the value by id better way to avoid this use the class instead if you want.. You can modify the id attribute after creating the element using .attr().. Completed Code Copy a <li> element from one list to another: // Get the last <li> element ("Milk") of <ul> with id="myList2". var itm = document.getElementById("myList2").lastChild; // Copy the <li> element and its child nodes. var cln = itm.cloneNode(true); // Append the cloned <li> element to <ul> with id="myList1". document.getElementById("myList1").
Cloning a JavaScript object is a task that is used mostly because we do not want to create the same object if the same object already exists. There are few ways. By iterate through each property and copy them to new object. Using JSON method as the source object MUST be JSON safe. Apr 03, 2020 - Guide to explain how to clone an HTML DOM element with Vanilla Javascript. See the code examples in the Codepen! Clone an element const cloned = ele.cloneNode(true); Using the cloneNode (true) method will deep copy a given element. In the sample code above, all attributes and children of the original node ele will be cloned in cloned as well.
How to clone a dom element using native javascript I needed to clone a dom element in a project and have searched this at google and stackoverflow but i just finded a lot of big functions to do this, looking at jsref I findend the cloneNode method, a native javascript method to clone dom elements. JavaScript has many ways to do anything. I've written on 10 Ways to Write pipe/compose in JavaScript, and now we're doing arrays. 1. Spread Operator (Shallow copy)Ever since ES6 dropped, this has been the most popular method. It's a brief syntax and you'll find JavaScript offers many ways to create shallow and deep clones of objects. You can use the spread operator (...) and Object.assign () method to quickly create a shallow object duplicate. For the deep cloning of objects, you can either write your own custom function or use a 3rd-party library like Lodash.
Get the HTML of the element to clone with .innerHTML, and then just make a new object by means of createElement()... var html = document.getElementById('test').innerHTML; var clone = document.createElement('span'); clone.innerHTML = html; JavaScript HTML DOM Document lets us either to collect or change parameters that of an HTML element. And, by using them, we can either copy any HTML element we want or let a visitor perform that action. In order to create a new HTML element, we have to use the HTML DOM createElement() method. I have a html element (like select box input field) in a table. Now I want to copy the object and generate a new one out of the copy, and that with JavaScript or jQuery. I think this should work somehow but I'm a little bit clueless at the moment. Something like this (pseudo code):
The <template> HTML element is a mechanism for holding HTML that is not to be rendered immediately when a page is loaded but may be instantiated subsequently during runtime using JavaScript. Think of a template as a content fragment that is being stored for subsequent use in the document. While the parser does process the contents of the ... 16/5/2021 · Clone HTML Element Objects in JavaScript. To clone HTML element objects in JavaScript, we can call the cloneNode method with true to clone the element. For instance, if we have the following element: <div> hello world </div> Then we can clone the div by writing: const clone = document.querySelector('div').cloneNode(true); console.log(clone) Lastly, the HTML body document.body itself is also an element! We also can insert elements into the body. To add an element to the top of the body (top of page) - document.body.insertAdjacentElement("afterbegin", ELEMENT). To append an element to the bottom of the body (bottom of page) - document.body.appendChild(ELEMENT). P.S.
I need to clone the id and then add a number after it like so id1, id2, etc. Every time you hit clone you put the clone after the latest number of the id. $("button").click(function() { ... Copy a Node. The cloneNode () method creates a copy of a specified node. The cloneNode () method has a parameter (true or false). This parameter indicates if the cloned node should include all attributes and child nodes of the original node. The following code fragment copies the first <book> node and appends it to the root node of the document: Cloning. Cloning in javascript is nothing but copying an object properties to another object so as to avoid creation of an object that already exists. There are a few ways to clone a javascript object. 1) Iterating through each property and copy them to a new object. 2) Using JSON method. 3) Using object.assign () method.
However, it doesn't copy the event listeners added via addEventListener() or assignment to element's properties such as originalNode.onclick = eventHandler(). If you clone a node with an id attribute and place the cloned node in the same document, the id will be duplicate. When used in conjunction with one ... way to duplicate elements on a page. Consider the following HTML: As shown in the discussion for .append(), normally when an element is inserted somewhere in the DOM, it is moved from its old location. So, given the code: ... Note: When using the .clone() method, ... 6 days ago - Warning: cloneNode() may lead to duplicate element IDs in a document! If the original node has an id attribute, and the clone will be placed in the same document, then you should modify the clone's ID to be unique.
The object variable is a container for a newly initialized object. The copy variable points to the same object and is a reference to the object. The object { a: 2, b: 3, } shows that there exist two ways of gaining success. This method can remove any form of immutability, leading to bugs. There is a naive way to copy objects: it's looping through the original one copying every p JavaScript preprocessors can help make authoring JavaScript easier and more convenient. For instance, CoffeeScript can help prevent easy-to-make mistakes and offer a cleaner syntax and Babel can bring ECMAScript 6 features to browsers that only support ECMAScript 5. First, we give the HTML element a unique id. Then select it with var element = document.getElementById (ID) in Javascript. Finally, take note that innerHTML can be used in two directions. When we do var contents = element.innerHTML, it will get the current contents of element.
// Get the element var elem = document.querySelector('#elem1'); Now, we can use the Element.cloneNode () method to create a copy. You call the cloneNode () method on the element you want to copy. If you want to also copy elements nested inside it, pass in true as an argument. Code language: JavaScript (javascript) By default, the cloneNode() method only clones the target element, but not all descendants of the target element. To clone the element and also its descendants, you pass true into the cloneNode() method: Grab the source element - var source = document.getElementById ("source") Create an evil clone - let evilclone = source.cloneNode (true). Take note of the true parameter here, this is to specify "also clone the children enclosed within". By default, this is false and the children will be excluded from the cloning vat.
Jul 27, 2017 - A little bit old-school but it will do in some circumstances. HTML elements allow us to add an event attribute with a tiny bit of JavaScript functionality inside it. Like so: JavaScript HTML DOM Elements Previous Next This page teaches you how to find and access HTML elements in an HTML page. Finding HTML Elements. Often, with JavaScript, you want to manipulate HTML elements. To do so, you have to find the elements first. There are several ways to do this: Copy an element including event handlers How to use the clone() method to copy an element, including event handlers. ... Get certified by completing a course today! ... If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: ... Thank You For Helping Us! Your message has been sent to W3Schools. ... HTML ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Not only will you need to clone, but you'll probably want to do deep cloning as well. node.cloneNode(true); Documentation is here. If deep is set to false, none of the child nodes are cloned. Any text that the node contains is not cloned either, as it is contained in one or more child Text nodes. Copying text to clipboard can be done in 5 simple steps. Create a <textarea> element and append it to our HTML document. Fill the text or content you want to copy in this <textarea>. Use HTMLElement.select() to select the contents of this <textarea>. Copy the content of the <textarea> using Document.execCommand ('copy').
Add the following javascript code to your page: The function copies the visible text of the element to the clipboard. This works as if you had selected the text and copied it with ctrl+c. Use the parameter "id" to select the element you want to copy. August 09, 2020 Atta To clone a DOM element in JavaScript, you can use the element's cloneNode () method. This method creates a copy of the node and returns the clone. Here is an example: Returns an exact copy of the current node. The new node contains the same attributes with the same values as the original element. The child elements of the cloned node can also be copied in the original order with the cloneNode method (depending on the first parameter of the cloneNode method).
Mar 28, 2016 - In jquery, we can clone an element and all its events with clone() method. Example: $(".element").clone(true); How can we do the same thing with native Javascript? I'm updating my post. Jan 28, 2014 - We sort of talked about how everything ... and cloneNode. With everything you've learned here, there is nothing preventing you from starting off with a completely empty page and using just a few lines of JavaScript to populate everything inside it: <!DOCTYPE html> <html> <head> ... // Syntax: let newClone = node.cloneNode([deep]) // Example: let p = document.getElementById("para1") let p_prime = p.cloneNode(true)
Create a deep copy of a DOM element. In modern browsers, including IE 8, there's a native clone method for replacing jQuery's $.clone() method:
Best Practices For Using Vwo Editor Vwo
7 Ways To Copy Content From A Website With Disabled Text
Parameters In Custom Javascript Steps
Creating Removing And Cloning Dom Elements
View Webpage Source Html Css And Javascript In Google Chrome
Chapter 3 1 Finding Elements Dom Selection
How Can We Convert Html String To Object Using Javascript
Html Liquid Element Display Better Content Of Your Pages
Github Gerhobbelt Hilitor Text Highlighting Anywhere In
How To Clone Javascript Object Tecadmin
7 Tools For Building Web Components Bits And Pieces
40 Useful Tooltips Scripts With Css Javascript And Jquery
Clone Html Element Using Jquery The Techjoomla Blog
Javascript Copy Data In Dom Show In Popup Html In Google
4 Ways To Duplicate Html Elements In Javascript
Duplicate Html Elements Using Javascript Repeatjs Css Script
Jquery Remove All Inner Html Code Example
What Is The Most Efficient Way To Deep Clone An Object In
Embedding Views Amp Javascript Api Usage
Getting Width Amp Height Of An Element In Javascript
A Javascript Library For Adding Context Menus To Any Html
3 Ways To Clone Objects In Javascript Samanthaming Com
Copy All Html From Chrome S Inspect Element
Print The Content Of A Div Element Using Javascript
The Most Popular Content Manipulation Methods In Jquery Vegibit
0 Response to "30 How To Clone Html Element Javascript"
Post a Comment