22 How To Retrieve Data From Json Object In Javascript
When fetching data from a remote server, the server's response will often be in JSON format. In this quick tip, I'll demonstrate how you can use JavaScript to parse the server's response, so ... JavaScript objects can only exist within the JavaScript language, so when you're working with data that needs to be accessed by various languages, it is best to opt for JSON. Accessing JSON Data. JSON data is normally accessed in Javascript through dot notation. To understand how this works, let's consider the JSON object sammy:
Never Confuse Json And Javascript Object Ever Again By
To get the JSON data from the response, we execute the json() function. The json() function also returns a promise. This is why we just return it and chain another then function. In the second then function we get the actual JSON data as a parameter. This data looks just like the data in our JSON file. Now we can take this data and display it on our HTML page. Notice that we are calling a function called appendData.
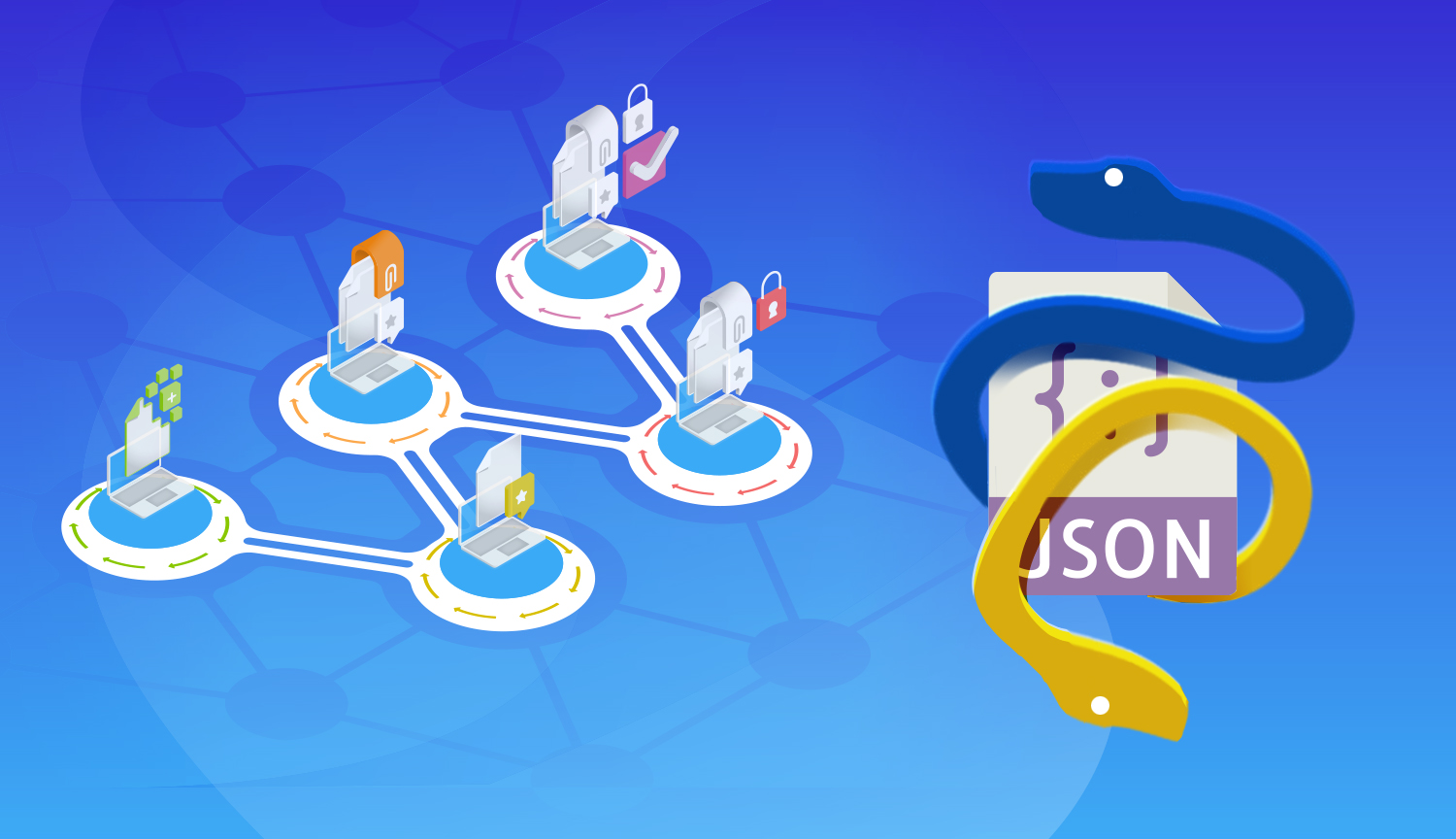
How to retrieve data from json object in javascript. Yes Luopan, I got it I have changed the json object as falloing: var obj = jQuery.parseJSON(json); alert( obj.rows[0].cell[0] ); my problem solved Thank you very much. - vissu Jul 15 '11 at 11:59 Add a comment | Let us see how to retrieve JSON values. First, we use object name and then put dot (.) followed by the key name. Now, it's time to work with complicated JSON objects. Retrieving the data from JSON Object. Retrieving all the values using Each loop in jQuery. Output - "Washington". Just to be clear, it doesn't need to address an actual JSON file directly. It could be that or it could be an API route on a web server that serves up JSON files/data. In other words, if you just start using $.getJSON to random web addresses, they aren't going to be sending back json data. To make it clearer, here are an example of each:
24/8/2020 · Now, let’s write the code for this, we will use the require module to fetch the json data and then return a filtered array like this − Example const path = "./data.json"; const parseData = (path) => { const data = require(path); //no need to parse the data as it is already parsed return data.names.filter(el => el.readable); } const results = parseData(path); console.log(results); A common use of JSON is to exchange data to/from a web server. When sending data to a web server, the data has to be a string. Convert a JavaScript object into a string with JSON.stringify(). Stringify a JavaScript Object. ... In JSON, date objects are not allowed. The JSON.stringify() ... .map((response: Response) => response.json()).do(data => console.log("All: " + JSON.stringify(data))).catch(this.handleError);} I need to parse JSON response in key value pair. Please note channel list- contain hashmap of channel name and its description so key value will differ on calls from one client to another client.
JSON stands for J ava S cript O bject N otation. JSON is a lightweight data interchange format. JSON is language independent *. JSON is "self-describing" and easy to understand. * The JSON syntax is derived from JavaScript object notation syntax, but the JSON format is text only. Code for reading and generating JSON data can be written in any ... The jQuery code uses getJSON () method to fetch the data from the file's location using an AJAX HTTP GET request. It takes two arguments. One is the location of the JSON file and the other is the function containing the JSON data. The each () function is used to iterate through all the objects in the array. It also takes two arguments. GET APIs or endpoints that are used only to retrieve data from a server using a popular data format called JSON (JavaScript Object Notation). They often send back a complex JSON object with deeply nested objects and arrays.
The JSON-encoded data will be an object with two keys: path and data The path points to a location relative to the request URL For each key in the data, the client should replace the corresponding key in its cache with the data for that key in the message: keep-alive: The data for this event is null, no action is required: cancel Encoding Data as JSON in JavaScript. Sometimes JavaScript object or value from your code need to be transferred to the server during an Ajax communication. JavaScript provides JSON.stringify() method for this purpose which converts a JavaScript value to a JSON string, as shown below: Stringify a JavaScript Object To retrieve the JavaScript object from localStorage, use the getItem () method. You still need to use the JSON.parse () method to parse the JSON string back to an object: const userStr = localStorage.getItem('user'); const userObj = JSON.parse( userStr); console.log( userObj. name); console.log( userObj. job); To learn more about localStorage ...
in JavaScript it's the most easy, just because JSON is native valid JavaScript syntax, can copy a JSON object into a JavaScript source code and assign to a variable, it just works, even no need ... JSON Object Literals. JSON object literals are surrounded by curly braces {}. JSON object literals contains key/value pairs. Keys and values are separated by a colon. Each key/value pair is separated by a comma. It is a common mistake to call a JSON object literal "a JSON object". JSON cannot be an object. Node.js is a JavaScript runtime environment that includes everything you need to execute a program written in JavaScript. MongoDB, on the other hand, is a document-based, distributed database built…
18/7/2020 · Example. Live Demo. <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Document</title> <style> body { font-family: "Segoe UI", Tahoma, Geneva, Verdana, sans-serif; } .sample,.result { font-size: 18px; font-weight: 500; color: red; } </style> </head> ... How to access nested json objects in JavaScript? Accessing nested json objects is just like accessing nested arrays. Nested objects are the objects that are inside an another object. In the following example 'vehicles' is a object which is inside a main object called 'person'. Using dot notation the nested objects' property (car) is accessed. JavaScript Object Notation (JSON) is a standard text-based format for representing structured data based on JavaScript object syntax. It is commonly used for transmitting data in web applications (e.g., sending some data from the server to the client, so it can be displayed on a web page, or vice versa).
Hello, Now, let's see post of how to get data from json file in node js. you will learn how to fetch data from json in node js. This article goes in detailed on how to get value from json object in node js. you will learn node js read json file example. For each record in the MyPLayers table, create a JSON (again) object insert the contents of the record (obtained from the ResultSet object) into it using the put() method. Finally, add the JSON object to the array created in the previous step. The retrieve-resources id will trigger the click event in our JavaScript code, which will in turn replace the placeholder content inside our display-resources div with content from our JSON file. See the Pen jQuery AJAX JSON for a demo using the above code.
JSON is a file format widely used for static storage and app config management with any of the frameworks and data servers. Any JSON file contains the key-value pair separated by the comma operator. JavaScript objects are an integral part of the React app, so they need to get accessed from JSON files/data to be uses in components. The code above uses Bootstrap and includes the retrieve-resources and display-resources ids needed for the click event and placeholder for the data retrieved from JSON. Once a user clicks the retrieve-resources link, the JS code above will begin. See the Pen JavaScript Fetch JSON for a demo using the above code. (Note in this codepen demo I am ... Let's inspect the above JSON data to understand how it works: The whole JSON data is surrounded by curly braces. This makes it a JSON object.; The JSON object consists of comma-separated key-value pairs, such as "Name": "Charlie".; Each of the JSON object's keys has to be wrapped inside double-quotes. (The value only needs to be double-quoted if it's a string).
JSON is short for JavaScript Object Notation. JSON data is represented in a logical, organized, and easy-to-access manner. JSON can contain multiple levels of objects, arrays, and various field data that can be parsed by GoAnywhere MFT. Create a New Project. To begin, create a new Project following the Getting Started with Projects tutorial. JSON or JavaScript Object Notation, as you know is a simple easy to understand data format. JSON is lightweight and language independent and that is why its commonly used with jQuery Ajax for transferring data. Here, in this article I’ll show you how to convert JSON data to an HTML table dynamically using JavaScript. In addition, you will ... So that you can use it to extract the data from the JSON array. So many of us facing problems to extract the data from the long nested JSON in postman. Here is the solution for that, please follow these steps as per your requirements. Now extract the data from the response of the nested JSON object array schema.
10/8/2016 · The problem here is that you have here an array of objects, and you are trying to access it without indexing. You should first parse it using and then access the object by indexing. let objects = JSON.parse(data) console.log(objects[0].id)
How To Read Local Json File In React Js By Rajdeep Singh
Import Json To Database Tutorial
Get Json Data From Url Javascript Code Example
Filtering Json Array In Javascript Code Example
How To Set Multiple Json Data To A One Time Request Using
Javascript How To Retrieve Json Data With Ajax
How To Extract Data From Json In Javascript Geeksread
How To Import Export Json Data Using Sql Server 2016
Access Json Object Values Code Example
How To Parse Json Data With Python Pandas By Ankit Goel
How To Read And Write A Json Object To A File In Node Js
How Do I Extract Nested Data In Python By Boris Towards
In Java How To Convert Map Hashmap To Jsonobject 4
Extract Nested Data From Complex Json
Chrome Developer Tools Inspect Json Path And Extract Data
Fetch Data Request To Nested Json Object Error React Next
Working With Json Learn Web Development Mdn
Json Handling With Php How To Encode Write Parse Decode
Load And Render Json Data Into React Components Pluralsight
How To Extract Data From Json In Javascript Geeksread
How Can I Get Asp Net C To Load Ajax Json Data Returned From
0 Response to "22 How To Retrieve Data From Json Object In Javascript"
Post a Comment