29 Each Loop In Javascript
An alternative to for and for/in loops isArray.prototype.forEach(). The forEach() runs a function on each indexed element in an array. Starting at index[0] a function will get called on index[0], index[1], index[2], etc… forEach() will let you loop through an array nearly the same way as a for loop: forEach accepts a callback function and, optionally, a value to use as this when calling that callback (not used above). The callback is called for each entry in the array, in order, skipping non-existent entries in sparse arrays. Although I only used one parameter above, the callback is called with three arguments: The value of each entry, the index of that entry, and a reference to the array ...
How To Loop Through An Array Of Objects In Javascript Pakainfo
JavaScript supports the nested loop feature, where a loop is present inside another loop. A loop can have one or number and/or n nested level of loops defined inside another loop. For each outer loop, the inner loop gets to execute.
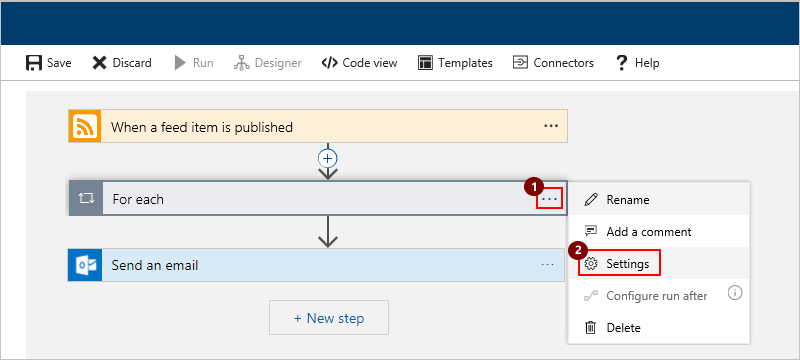
Each loop in javascript. Loop through List in LWC. To loop through List in LWC, we can make use of for:each and iterator:it. To use for:each, assign the Array to for:each and iterator variable to for:item. Then, we can use this iterator variable to access the elements in the Array. To use iterator:it, we just need to assign the Array to iterator:it. Then, we can access ... The following example logs one, two, four.. When the entry containing the value two is reached, the first entry of the whole array is shifted off—resulting in all remaining entries moving up one position. Because element four is now at an earlier position in the array, three will be skipped.. forEach() does not make a copy of the array before iterating. 14/10/2020 · javascript array for-each loop. October 14, 2020 April 11, 2021 AskAvy . javascript forEach() method calls a function once for each element in an array , callback function is not executed for array elements without values. for each code example. we have a array like.
This article describes the difference between a forEach and for loop in detail. The basic differences between the two are given below. For Loop: The JavaScript for loop is used to iterate through the array or the elements for a specified number of times. If a certain amount of iteration is known, it should be used. Update the value of each element to 10 times the original value: const numbers = [65, 44, 12, 4]; numbers.forEach(myFunction) function myFunction (item, index, arr) {. arr [index] = item * 10; } Code language: CSS (css) How it works. First, declare a variable counter and initialize it to 1.; Second, display the value of counter in the Console window if counter is less than 5.; Third, increase the value of counter by one in each iteration of the loop.; Since the for loop uses the var keyword to declare counter, the scope of counter is global. Therefore, we can access the counter ...
Since the objects in JavaScript can inherit properties from their prototypes, the fo...in statement will loop through those properties as well. To avoid iterating over prototype properties while looping an object, you need to explicitly check if the property belongs to the object by using the hasOwnProperty() method: 9/6/2021 · How to Use forEach() to Iterate Through a JavaScript Map. Jun 9, 2021 JavaScript's Map object has a handy function, forEach(), which operates similarly to arrays' forEach() function. JavaScript calls the forEach() callback with 3 parameters: the value, the key, and the map itself. 9/3/2018 · Exit Controlled Loops: In this type of loops the test condition is tested or evaluated at the end of loop body. Therefore, the loop body will execute atleast once, irrespective of whether the test condition is true or false. do – while loop is exit controlled loop. JavaScript mainly provides three ways for executing the loops.
Here's a very common task: iterating over an object properties, in JavaScript. 🏠 Go back to the homepage How to iterate over object properties in JavaScript Here's a very common task: iterating over an object properties, in JavaScript ... to generate an array with all its enumerable properties, and loop through that, using any of the above ... In the above example, we initialized the for loop with let i = 0, which begins the loop at 0. We set the condition to be i < 4, meaning that as long as i evaluates as less than 4, the loop will continue to run. Our final expression of i++ increments the count for each iteration through the loop. JavaScript forEach Loops Made Easy. James Gallagher. Jun 24, 2020. 0. The JavaScript forEach loop is an Array method that executes a custom callback function on each item in an array. The forEach loop can only be used on Arrays, Sets, and Maps. If you've spent any time around a programming language, you should have seen a "for loop.".
JavaScript Loops. Loops are handy, if you want to run the same code over and over again, each time with a different value. ... Statement 3 increases a value (i++) each time the code block in the loop has been executed. Statement 1. Normally you will use statement 1 to initialize the variable used in the loop (let i = 0). jQuery's each() function is used to loop through each element of the target jQuery object — an object that contains one or more DOM elements, and exposes all jQuery functions. It's very ... JavaScript forEach Loop. August 13, 2021. August 15, 2021. by admin. The forEach loop is a distinctive form of loop existing in most programming languages made use of to loop as a result of the things of an array. It is generally utilised to change the loop to stay away from possible off-by-one bugs/faults as it does not have a counter.
The forEach loop is a control flow statement that is used to loop through items in a collection. It proves useful when we need to perform different actions on each element of the loop individually. In this post, we took up the forEach loop. We learned what it is and how to use it. Moreover, we also compared it with the more common for a loop. In a for loop, you can easily skip the current item by using the continue keyword or use break to stop the loop altogether. But that is not the case with the forEach() method. Since it executes a callback function for each element, there is no way to stop or break it other than throwing an exception. The forEach loop is a special type of loop present in most programming languages used to loop through the elements of an array. It is mostly used to replace the loop to avoid potential off-by-one bugs/errors as it does not have a counter. It proves useful when we need to perform different actions on each element of the loop individually.
The JavaScript forEach method is one of the several ways to loop through arrays. Each method has different features, and it is up to you, depending on what you're doing, to decide which one to use. In this post, we are going to take a closer look at the JavaScript forEach method. Considering that we have the following array below: It resolves the shortcomings of the forEach loop. For Each JavaScript example files and code download. The below image shows the file structure of the JavaScript For Each example code. It has the JavaScript asset iteration.js to define forEach callbacks to parse types of array collections. In the code given above you have to do 2000 * i at line 8 because setTimeout method inside the loop doesn't makes the loop pause but actually adds a delay to each iteration. Remember that all the iteration start their time together. Thus if we only do 2000 there, that will make all the iterations execute together and it will just give 2000 ms delay in the first iteration and all other ...
The forEach method is generally used to loop through the array elements in JavaScript / jQuery and other programming languages. You may use other loops like for loop to iterate through array elements by using length property of the array, however, for each makes it quite easier to iterate and perform some desired actions on array elements. 1/1/2014 · The JavaScript Array forEach Method. The traditional for loop is easy to understand, but sometimes the syntax can be tedious. For example, the nested loop requires new variable declarations with a nested scope. Modern JavaScript has added a forEach method to the native array object. Array.prototype.forEach(callback([value, index, array]), thisArg) How to Break Out of a JavaScript forEach() Loop. Oct 5, 2020 JavaScript's forEach() function executes a function on every element in an array. However, since forEach() is a function rather than a loop, using the break statement is a syntax error:
The .each () method is designed to make DOM looping constructs concise and less error-prone. When called it iterates over the DOM elements that are part of the jQuery object. Each time the callback runs, it is passed the current loop iteration, beginning from 0. More importantly, the callback is fired in the context of the current DOM element ... The continue statement can be used to restart a while, do-while, for, or label statement.. When you use continue without a label, it terminates the current iteration of the innermost enclosing while, do-while, or for statement and continues execution of the loop with the next iteration. In contrast to the break statement, continue does not terminate the execution of the loop entirely. In programming, loops are used to repeat a block of code. For example, if you want to show a message 100 times, then you can use a loop. It's just a simple example; you can achieve much more with loops. This tutorial focuses on JavaScript for loop. You will learn about the other type of loops in the upcoming tutorials.
The Object.keys () method was introduced in ES6 to make it easier to loop over objects. It takes the object that you want to loop over as an argument and returns an array containing all properties names (or keys). After which you can use any of the array looping methods, such as forEach (), to iterate through the array and retrieve the value of ... 3. forEach is easier to read. Again, this is down to the discretion of the developer, but here's why I feel that the forEach method is a little cleaner than the for loop. In a forEach method, we pass each food type within that iteration into the callback. A for loop needs you to access the array using a temporary i variable. Categories of Loops in JavaScript The 'For' Loop. The For Loop comes first because of its simplicity and ease of use. It is a very user-friendly kind of loop that runs with a method of using a counter. ... Here, I have used all the numbers in the form of an array, then printed each of them in a console window. In the same way, you can make ...
Add Loops To Repeat Actions Azure Logic Apps Microsoft Docs
Front End Psa Are You Using For Loops In Your Javascript
How To Execute Foreach In Javascript
Javascript Loops How To Handle Async Await
Php Loop For Foreach While Do While Example
Convert A Foreach Loop To Linq Visual Studio Windows
How To Break For Each Loop In Javascript Facebook
How To Iterate Through Java List Seven 7 Ways To Iterate
Javascript Foreach How To Iterate Array In Javascript
For Each Example Enhanced For Loop To Iterate Java Array
Javascript Async Each Loop Code Example
How To Iterate Array In Javascript Code Example
Javascript Object For Each Loop Stack Overflow
Loops In Javascript Dev Community
Functions Of Javascript End Of The For Loop In Javascript
Functions Of Javascript End Of The For Loop In Javascript
How To Exit For Each Loop Help Uipath Community Forum
Ssis Foreach Loop Vs For Loop Container
For Loop In Javascript The Engineering Projects
Javascript Under The Hood Building Our Own Foreach And Map
Java For Each Loop With Examples 100 Learn All Important
Foreach Is For Side Effects The Array Method Foreach Is
Use For Each Loop To Change The Array Element Value In Javascript
Why Should Foreach Be Preferred Over Regular Iterators
For Each Loop In Java Geeksforgeeks
Best Of Jsperf 2000 2013 Part 2 3 Sitepoint
0 Response to "29 Each Loop In Javascript"
Post a Comment