26 Sort Array By Value Javascript
I am using this code to read an xlxs file and pushing the values I need to a simpler array. I need to sort this array by the value of the top2box key so the highest value is key 0. Here is my current code // data is an array of arrays, lets loop through it to sort. The array contains each row of my xlxs file. Jan 04, 2021 - We then create a function that sorts our students variable based on the “age” value in each JSON object by comparing ages. Finally, our program prints out our sorted list of students. ... There is also a built-in JavaScript function that allows you to sort an array in reverse order: reverse().
Javascript Sort The Items Of An Array W3resource
Sort array based on another array in JavaScript. We are required to write a sorting function that sort an array based on the contents of another array. For example − We have to sort the original array such that the elements present in the below sortOrder array appear right at the start of original array and all other should keep their order −.
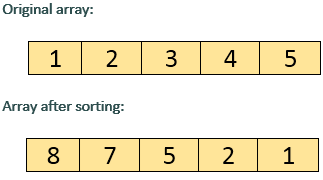
Sort array by value javascript. Nov 19, 2020 - How to sort an array numerically in JavaScript. The default ECMAScript sort is alphabetical, so a little magic is needed to sort an array in numerical order.. Home JavaScript Tutorials Sorting an array using sort() Here ... Taking things one step further, lets say your array doesn't contain just simple numeric or string values, but objects with properties instead: For getting more than one array sorted by one signature array, you could take sorting with map, where you sort an array of indices, indicating the final sorting and then reassign all arrays with this sorting. The getDfunction returns a formatted string by taking an index of array0for sorting.
Array.sort () : The sort method takes the callback function as its argument and returns the sorted array. The return condition inside the callback function. if our return condition is a - b then it sorts the array in ascending order. if our return condition is b - a then it sorts the array in descending order. Sep 26, 2019 - Arrays are a very powerful tool for a programmer. They allow one to group related data under a single data type. There are many things one can do with an array, but sometimes it is important to have… The sort() Method¶. The sort( ) method sorts the array elements in place and returns the sorted array. By default, the sort order is ascending, built upon converting the elements into strings and then comparing their sequences of UTF-16 code unit values.
Nov 26, 2019 - Let’s say that foo and bar are ... compared by the compare function, and the return value of the compare function is set up as follows: ... If you’re not familiar with arrow functions, you can read more about them here: ES6 Arrow Functions: Fat and Concise Syntax in JavaScript. ... Now let’s look at sorting an array of ... Sort object array based on another array of keys - JavaScript; Extract key value from a nested object in JavaScript? Sort Python Dictionaries by Key or Value; Java Program to remove key value pair from HashMap? Add a key value pair to dictionary in Python; Splitting an array based on its first value - JavaScript; Get value for key from nested ... Sorting an Array by Input Value in JavaScript. Zach Weber. Aug 4, 2020 · 4 min read. This is a continuation of my new series of posts on algorithms. You can check out my previous one about ...
Jul 25, 2020 - Return value: This method returns the reference of the sorted original array. Below examples illustrate the JavaScript Array sort() method: The sort () method sorts the elements of an array. The sort order can be either alphabetic or numeric, and either ascending (up) or descending (down). By default, the sort () method sorts the values as strings in alphabetical and ascending order. This works well for strings ("Apple" comes before "Banana"). The sort( ) method accepts an optional argument which is a function that compares two elements of the array.. If the compare function is omitted, then the sort( ) method will sort the element based on the elements values.. Elements values rules: 1.If compare (a,b) is less than zero, the sort( ) method sorts a to a lower index than b.In other words, a will come first.
You could sort by numbers and if a comparison sign is available, then take for the same numerical value the delta of both offsets, which reflects the order of comparison. Sort an Array of Objects in JavaScript Summary : in this tutorial, you will learn how to sort an array of objects by the values of the object's properties. To sort an array of objects, you use the sort() method and provide a comparison function that determines the order of objects. When we return a positive value, the function communicates to sort() that the object b takes precedence in sorting over the object a.Returning a negative value will do the opposite. The sort() method returns a new sorted array, but it also sorts the original array in place. Thus, both the sortedActivities and activities arrays are now sorted. One option to protect the original array from being ...
May 18, 2020 - In Javascript, Array.sort() is a built-in method of the Array Object. Using Array.sort(), we can sort arrays in descending or ascending order Jan 31, 2020 - In this tutorial, you have learned how to sort an array of objects by the values of the object’s properties. ... Primitive vs. Reference Values ... The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively. You want to sort it in Javascript, right? What you want is the sort () function. In this case you need to write a comparator function and pass it to sort (), so something like this:
When the sort () function compares two values, it sends the values to the compare function, and sorts the values according to the returned (negative, zero, positive) value. If the result is negative a is sorted before b. If the result is positive b is sorted before a. If the result is 0 no changes are done with the sort order of the two values. Jan 04, 2021 - Learn how to quickly write code to sort JavaScript arrays with this handy one-liner. Sorting an array of objects by an array JavaScript; JavaScript array sorting by level; Sorting an array of objects by property values - JavaScript; Sorting an array object by property having falsy value - JavaScript; Sorting an array objects by property having null value in JavaScript; Alternative sorting of an array in JavaScript; Sorting ...
In the above program, the sort () method is used to sort an array by the name property of its object elements. The sort () method sorts its elements according to the values returned by a custom sort function ( compareName in this case). Here, The property names are changed to uppercase using the toUpperCase () method. The function should sort the array such that the elements that are repeated for the least number of times appears first followed by the elements with increasing frequency. For example −. If the input array is −. const arr = [1,1,2,2,2,3]; Then the sorted array should be −. Jul 08, 2019 - Arrays in JavaScript come with a built-in function that is used to sort elements in alphabetical order. However, this function does not directly work on arrays of numbers or objects. Instead a custom function, that can be passed to the built-in sort() method to sort objects based on the ...
The sort () method sorts the elements of an array in place and returns the sorted array. The default sort order is ascending, built upon converting the elements into strings, then comparing their sequences of UTF-16 code units values. The time and space complexity of the sort cannot be guaranteed as it depends on the implementation. The solution is an array! An array can hold many values under a single name, and you can access the values by referring to an index number. ... But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". ... cars.sort() // Sorts the array Array methods are covered in the next chapters. Mar 28, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Sep 05, 2020 - However keep in mind - this can ... returns numeric values (such as the Date object). ... sort is one of many Array Mutator Methods along with shift, splice, reverse and others. For more info on all methods see How To Use Array Methods in JavaScript: Mutator Meth... How Bubble-sort works We have an unsorted array arr = [ 1, 4, 2, 5, -2, 3 ] the task is to sort the array using bubble sort. Bubble sort compares the element from index 0 and if the 0th index is less than 1st index then the values get swapped and if the 0th index is less than the 1st index then nothing happens. Sort array of objects by string property value - JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. Suppose, we have an array of Objects like this −. const arr = [ { first_name: 'Lazslo', last_name: 'Jamf' }, { first_name: 'Pig', last_name: 'Bodine' }, { first_name: 'Pirate', last_name: 'Prentice' } ]; We ...
Oct 02, 2020 - JavaScript arrays are an ordered collection that can hold data of any type. Arrays are created with square brackets [...] and allow duplicate elements. In JavaScript, we can sort the elements of an array with the built-in method called, sort(). In th... The JavaScript Array.sort () method is used to sort the array elements in-place and returns the sorted array. This function sorts the elements in string format. It will work good for string array but not for numbers. For example: if numbers are sorted as string, than "75" is bigger than "200". Example: The sort () method allows you to sort elements of an array in place. Besides returning the sorted array, the sort () method changes the positions of the elements in the original array. By default, the sort () method sorts the array elements in ascending order with the smallest value first and largest value last.
You could use a two pass approach by checking null values first and then order by string. To change the sort order, you could swap the parameters or use a negated result of the one of the function. You want to render this list, but first you want to order it by the value of one of the properties. For example you want to order it by the color name, in alphabetical order: black, red, white. You can use the sort() method of Array, which takes a callback function, which takes as parameters 2 objects contained in the array (which we call a and b): The JavaScript Array sort () method sorts the items of an array. The sort () method sorts the elements of a given array in a specific ascending or descending order. The syntax of the sort () method is:
Morioh is the place to create a Great Personal Brand, connect with Developers around the World and Grow your Career! Sorting an array objects by property having null value in JavaScript Javascript Web Development Front End Technology Object Oriented Programming We are required to write a JavaScript function that takes in an array of objects. The objects may have some of their keys that are mapped to null. We are required to write a JavaScript function that takes in one such array of objects. The function should sort this array based on two different properties − sort by the higher "resFlow" value, but with the lowest "resHP" value.
Top 10 Javascript Array Methods You Should Know
Indexed Collections Javascript Mdn
Javascript Es5 Sorting An Array Of Objects By Multiple
How To Group An Array Of Associative Arrays By Key In Php
The Javascript Array Handbook Js Array Methods Explained
Java Program To Sort The Elements Of An Array In Ascending
Search Insert And Delete In A Sorted Array Geeksforgeeks
How To Sort An Array Of Numbers In Javascript How To Sort An Array Of Strings In Javascript
Java Program To Sort The Elements Of An Array In Descending
Sort Array Of Objects By String Property Value In Javascript
How To Merge Two Unsorted Arrays In Sorted Order In Java
Use A Custom Sort Function On An Array In Javascript
How To Sort Array Least To Greatest Javascript Stack Code Example
How To Sort Array Objects In Javascript By Value Property
Counting Sort Algorithm In Javascript Learnersbucket
Sort Array Of Objects By Hash Javascript Code Example
How To Sort Arrays In Javascript By Object Key Value The
Sorting Arrays With Lodash S Sortby Function Mastering Js
Sort In Descending Order With Custom Method In Javascript
How To Sort Array Of List Of Objects In Javascript Stack
How To Sort Array By Groups With Javascript Stack Overflow
Sorting Object Property By Values Stack Overflow
Solved Multiple Choice 1 The Javascript Array Object Su
The Python Sort List Array Method Ascending And Descending
The Sort Functions In Excel Sort And Sortby The Excel Club
0 Response to "26 Sort Array By Value Javascript"
Post a Comment