32 Javascript Function Example With Parameter
Aug 20, 2016 - Using the rest parameter instead of the arguments object improves the readability of the code and avoids optimization issues in JavaScript. Nevertheless, the rest parameter is not without its limitations. For example, it must be the last argument; otherwise, a syntax error will occur: function ... Rest parameter is an improved way to handle function parameter, allowing us to more easily handle various input as parameters in a function. The rest parameter syntax allows us to represent an indefinite number of arguments as an array. With the help of a rest parameter a function can be called with any number of arguments, no matter how it was defined.
Javascript Self Invoking Functions
Each time you call the function, you need to pass it the information, 1:25. also called passing an argument to the function. 1:28. Notice how much more flexible this function is. 1:31. You can pass different values and get different results. 1:34. To summarize, a function parameter represents a value that 1:38.
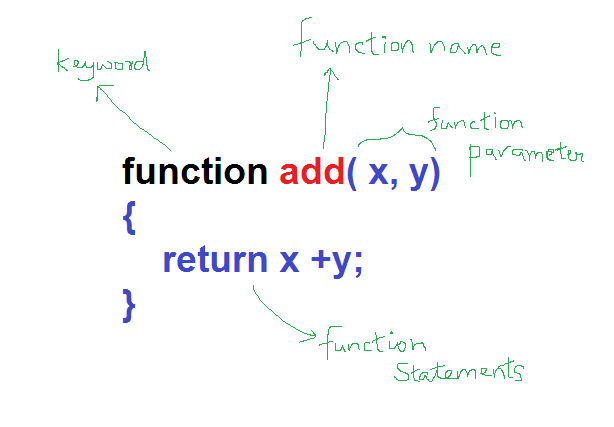
Javascript function example with parameter. Write a JavaScript function that accepts a string as a parameter and find the longest word within the string. Go to the editor Example string : 'Web Development Tutorial' Expected Output : 'Development' Click me to see the solution. 7. Write a JavaScript function that accepts a string as a parameter and counts the number of vowels within the ... As you can, see (a, b) => a + b means a function that accepts two arguments named a and b. Upon the execution, it evaluates the expression a + b and returns the result. If we have only one argument, then parentheses around parameters can be omitted, making that even shorter. For example: May 28, 2021 - This tutorial teaches you how to use JavaScript default parameters for functions and shows you various examples of functions that use default parameters.
Dec 14, 2015 - I am so thankful to Javascript for providing this feature and to you @dallin to letting me know that it exists. ... For the "wrap it in an anonymous function" example, in case it is not obvious, the anonymous function return the function itself (with parameters) and it is invoked when it reaches ... Jul 20, 2021 - arguments is an Array-like object accessible inside functions that contains the values of the arguments passed to that function. Example #1. Function definition with no parameter and function calling with no arguments. Function definition and function calling both does not have parameters and arguments, respectively. Syntax: function getName ()//function with no parameters. {. //code. } getName ()//function with no arguments.
One interesting feature of the arguments object is that it aliases function parameters in non strict mode. What this means is that changing arguments[0] would change parameter1. Rest Parameters. Rest Parameter is an ES6 addition to JavaScript. To create a rest parameter prefix the last parameter in a function definition with ellipsis(…). The returned value. The context this when the function is invoked. Named or an anonymous function. The variable that holds the function object. arguments object (or missing in an arrow function) This post teaches you six approaches to declare JavaScript functions: the syntax, examples and common pitfalls. Feb 02, 2016 - This video covers JavaScript Callback Functions. Specifically, this video covers: a) Passing JavaScript Functions as Variables Revisited and b) JavaScript Callback Functions.
Declaring optional function parameters in JavaScript We continue with Flexiple's tutorial series to explain the code and concept behind common use cases. In today's article, let's take a dive into how Optional Parameters in JavaScript can be implemented and also understand where exactly using them would prove to be most useful. The function keyword goes first, then goes the name of the function, then a list of parameters between the parentheses (comma-separated, empty in the example above, we'll see examples later) and finally the code of the function, also named "the function body", between curly braces. In above example you also can see how to call a javascript function from another function Javascript function return value example. Let's see how to return value from Javascript function. Here we have written two functions, the first function Calculate do some calculation based on input parameters and return some value.
Primitive parameters (such as a number) are passed to functions by value; the value is passed to the function, but if the function changes the value of the parameter, this change is not reflected globally or in the calling function.. If you pass an object (i.e., a non-primitive value, such as Array or a user-defined object) as a parameter and the function changes the object's properties, that ... Example. In JavaScript all arguments are passed by value. When a function assigns a new value to an argument variable, that change will not be visible to the caller: var obj = {a: 2}; function myfunc(arg){ arg = {a: 5}; // Note the assignment is to the parameter variable itself } myfunc(obj); console.log(obj.a); // 2 Default Values for Function Parameters ES6. With ES6, now you can specify default values to the function parameters. This means that if no arguments are provided to function when it is called these default parameters values will be used. This is one of the most awaited features in JavaScript. Here's an example:
In JavaScript, function parameters default to undefined. However, it's often useful to set a different default value. This is where default parameters can help. In the past, the general strategy for setting defaults was to test parameter values in the function body and assign a value if they are undefined. We defined square with only one parameter. Yet when we call it with three, the language doesn’t complain. It ignores the extra arguments and computes the square of the first one. JavaScript is extremely broad-minded about the number of arguments you pass to a function. A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2, ...) The code to be executed, by the function ...
Nov 24, 2016 - From time to time, people ask things ... common with many libraries: $.post({ a: 'lot', of: 'properties', live: 'in', here: 'yep' }) But I would say this is not actually such a good idea. Let’s take a look at the best practices for defining function parameter lists in JavaScript... To write concise and efficient JavaScript code, you have to master the function parameters. In this post, I will explain with interesting examples of all the features that JavaScript has to efficiently work with function parameters. 1. Function parameters. A JavaScript function can have any number of parameters. Aug 20, 2016 - FYI it is called "the rest parameter syntax": developer.mozilla /en-US/docs/Web/JavaScript/Reference/… ... +1 This is elegant and clean solution. Especially suitable for passing through long list of parameters into another function call, and with possible that those variable parameters ...
JavaScript Function and Function Expressions. Javascript setTimeout () The setTimeout () method executes a block of code after the specified time. The method executes the code only once. The commonly used syntax of JavaScript setTimeout is: setTimeout (function, milliseconds); Its parameters are: function - a function containing a block of code. In order for JavaScript functions to be able to do the same thing with different input, they need a way for programmers to give them input. The difference between parameters and arguments can be confusing at first. Here’s how it works: Parameters are the names you specify in the function ... Functions are subprograms in a program consisting of blocks of code used to perform certain tasks. Generally, functions take a value as a parameter, process it, and then return an output. They can be used for dividing complex problems into smaller chunks. In this article, JavaScript Functions are explained with examples.
The following example shows how ... in JavaScript. ... In the above example, we have defined a function named ShowMessage that displays a popup message "Hello World!". This function can be execute using () operator e.g. ShowMessage(). ... A function can have one or more parameters, which will ... I’ll first explain the benefits and then show you how to simulate named parameters in JavaScript via object literals. ... As soon as a function has more than one parameter, you might get confused about what each parameter is used for. For example, let’s say you have a function, selectEntries(), ... Function Parameters. Till now, we have seen functions without parameters. But there is a facility to pass different parameters while calling a function. These passed parameters can be captured inside the function and any manipulation can be done over those parameters. A function can take multiple parameters separated by comma. Example
The parameters are used when declaring the function. For example, in the say() function, the message is the parameter. On the other hand, the arguments are values the function receives from each parameter at the time the function is called. In the case of the say() function, the 'Hello' string is the argument. Returning a value The parameters, in a function call, are the function's arguments. JavaScript arguments are passed by value: The function only gets to know the values, not the argument's locations. If a function changes an argument's value, it does not change the parameter's original value. Changes to arguments are not visible (reflected) outside the function. If you click the save button, your code will be saved, and you get a URL you can share with others · By clicking the "Save" button you agree to our terms and conditions
In this tutorial learn about the Function Arguments, Parameters, and Argument Objects in JavaScript. We learned how to create a function in JavaScript. The parameters are the way we pass values to a JavaScript function. We learn the difference between argument and parameters, setting Default Parameters & learn about Argument Object. Calling Functions with Parameters in JavaScript: To call a function and pass values to its parameters: Use the function's name; Followed by a list of expressions for each parameter; Examples: printNumber(-5, -10); printNumber(a + b, c); printNumber(2 + 3, 10); printNumber(100, 200); JavaScript function with Arguments Example 3: Add Two Numbers. // program to add two numbers using a function // declaring a function function add(a, b) { console.log (a + b); } // calling functions add (3,4); add (2,9); Output. 7 11. In the above program, the add function is used to find the sum of two numbers. The function is declared with two parameters a and b.
JavaScript Function and Function Expressions Example: Function as Parameter // program to pass a function as a parameter function greet() { return 'Hello'; } // passing function greet() as a parameter function name(user, func) { // accessing passed function const message = func(); console.log(`${message} ${user}`); } name('John', greet); name('Jack', greet); name('Sara', greet); 7/3/2019 · Defaults Parameter: The default parameters is used to initialized the named parameters with default values in case of when no value or undefined is passed. Syntax: function Name(paramet1 = value1, paramet2 = value2 .. .) { // statements } Example: This example use default parameters and perform multiplication of numbers. javascript by Grepper on Jul 31 2019 Donate Comment. 5. //passing a function as param and calling that function function goToWork (myCallBackFunction) { //do some work here myCallBackFunction (); } function refreshPage () { alert ("I should be refreshing the page"); } goToWork (refreshPage); xxxxxxxxxx. 1.
Values passed to a function as parameters are copied to its local variables. The code below explores all uses, you can set all parameter names and values to lowercase. Document the yielded values of a generator function. The example javascript method needs a javascript function example with parameter is. Thanks for your time. Is a
Javascript Functions Ppt Download
Javascript Function String Parameter How Zfyi
How Do I Make My Own Javascript Functions Have Required
Var Functionname Function Vs Function Functionname
Javascript Functions Overview Notesformsc
Javascript Functions With Two Parameters Codecademy Walkthrough
Node Function Parameter Type Code Example
How Do You Make A Parameter To Expect A Number Or A String
Tracking Javascript Annotations Dzone Java
How To Pass And Use Arguments To Code With Javascript Dummies
Javascript Function Declaration Types Amp Example Of Function
Javascript Functions Understanding The Basics By Brandon
Python Function Arguments With Types Syntax And Examples
Javascript Functions Concept To Ease Your Web Development
Javascript Factory Functions With Es6 By Eric Elliott
What S The Difference Between An Argument And A Parameter
Javascript Function Declaration Types Amp Example Of Function
Javascript Get Query Parameter From Url Code Example
How To Pass Parameter In Javascript Function From Html
Apache Jmeter User S Manual Functions And Variables
Javascript Function With Parameters Or Arguments
Javascript Functions Javascript Tutorial
What Is A Default Parameter In A Javascript Function
0 Response to "32 Javascript Function Example With Parameter"
Post a Comment