20 Javascript Focus Input Element
The focus event fires when an element has received focus. The main difference between this event and focusin is that focusin bubbles while focus does not. ... JavaScript. const password = document. querySelector ('input[type="password"]'); password. addEventListener ... How to remove focus from the input HTML tag using JavaScript? Published October 23, 2020 . To remove the focus on an input tag element in HTML, you can use the blur() method on the element using JavaScript. // remove focus from the element element.blur(); Let's say we have an input tag and a button like this, <!--
Js Animation How To Assign Specific Background For Element
Definition and Usage The focus () method is used to give focus to an element (if it can be focused). Tip: Use the blur () method to remove focus from an element.
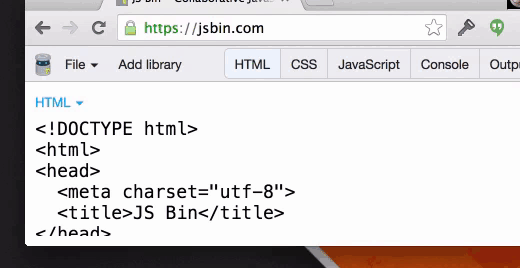
Javascript focus input element. In JavaScript, the currently active element is said to have Focus, which means it's the element being acted upon. Like a form field in which you are typing is said to have Focus. You can get the currently focused element with a simple reference on the Dom: var currentElement = document.activeElement. This will return the element which has ... 27/10/2016 · So on key up, if there is a value, focus the next. Using keyup allows you to validate the contents rather than skipping right away. They might switch to number mode on iOS for example which would trigger the focus if you simply use keypress. Codepen: http://codepen.io/anon/pen/qaGKzk changing focus to next input element. Javascript Forums on Bytes. ... changing focus to next input element Post your question to a community of 468,913 developers. It's quick & easy. changing focus to next input element. yawnmoth. Say I have two input elements and that I wanted to make it so that when the first ones input was what it should be ...
22/10/2020 · Focusing on the input tag is a common feature in modern websites to give attention to the user visiting. To set focus on an input tag element in HTML, you can use the focus() method on the element using JavaScript. Let's say we have an input tag in HTML like this, <!-- Input tag --> < input id = "myInput" type = "text" placeholder = "Enter some text here" /> 20/5/2019 · Last Updated :20 May, 2019. To set focus to an HTML form element, the focus() method of JavaScript can be used. To do so, call this method on an object of the element that is to be focused, as shown in the example. Example 1:The focus() method is set to the input tag when user clicks on Focus button. <!DOCTYPE html>. <element onfocusout="script"> In JavaScript: object.onfocusout = function(){script}; In JavaScript, with the addEventListener() method: object.addEventListener("focusout", script); Example 1: This example adds an onblur event to the <input> element and when it happens the specified code runs.
To detect if an element has the focus, you use the read-only property activeElement of the document object: const el = document .activeElement. Code language: JavaScript (javascript) To detect if an element has focus, you compare it with the document.activeElement. The following checks if the input text with the .username class has the focus: Why does this work? Simply, when the .focus() is called, the focus will be added to the beginning of the input element (which is the core problem here), ignoring the fact, that the input element already has a value in it. However, when the value of an input is changed, the cursor is automatically placed at the end of the value inside input element. 19/2/2019 · JavaScript focus method is used to give focus to a html element. It sets the element as the active element in the current document. It can be applied to one html element at a single time in a current document. The element can either be a button or a text field or a window etc. It is supported by all the browsers. Syntax: HTMLElementObject.focus()
A Boolean attribute which, if present, indicates that the input should automatically have focus when the page has finished loading (or when the <dialog> containing the element has been displayed). Note: An element with the autofocus attribute may gain focus before the DOMContentLoaded event is fired. But also JavaScript itself may cause it, for instance: An alert moves focus to itself, so it causes the focus loss at the element (blur event), and when the alert is dismissed, the focus comes back (focus event). If an element is removed from DOM, then it also causes the focus loss. If it is reinserted later, then the focus doesn't return. Introduction to JavaScript focus events. The focus events fire when an element receives or loses focus. These are the two main focus events: focus fires when an element has received focus. blur fires when an element has lost focus. The focusin and focusout fire at the same time as focus and blur, however, they bubble while the focus and blur do ...
Definition and Usage The autofocus attribute is a boolean attribute. When present, it specifies that an <input> element should automatically get focus when the page loads. Definition and Usage. The onfocus event occurs when an element gets focus. The onfocus event is most often used with <input>, <select>, and <a>. Tip: The onfocus event is the opposite of the onblur event. Tip: The onfocus event is similar to the onfocusin event. The main difference is that the onfocus event does not bubble. The JavaScript. Retrieving the currently selected element is as easy as using document.activeElement: var focusedElement = document. activeElement; This property isn't simply reserved for traditionally focusable elements, like form fields and links, but also any element with a positive tabIndex set.
The :focus CSS pseudo-class represents an element (such as a form input) that has received focus. It is generally triggered when the user clicks or taps on an element or selects it with the keyboard's Tab key. Note: This pseudo-class applies only to the focused element itself. Use :focus-within if you want to select an element that contains a ... The ComponentDidMount () method is the best place to set a focus on the input element. Let's see an example. In the above code first, we access the input element reference by using react callback refs. Next, we invoked this.searchInput.focus () method inside componentDidMount (), so that the input element is focussed. This method is a shortcut for .on( "focus", handler ) in the first and second variations, and .trigger( "focus" ) in the third.; The focus event is sent to an element when it gains focus. This event is implicitly applicable to a limited set of elements, such as form elements (<input>, <select>, etc.) and links (<a href>).In recent browser versions, the event can be extended to include all ...
If the input element is part of a form, instead of querying the element using the getElementById(), we can use the form name reference. The syntax for the usage is as follows: document.<Form name>.<Field name>.focus(); For example, assume we have a login form with an input field. We can set the focus of the input field by applying the following ... <input type="text" id="mytext"/> And If you are using JQuery, You can use this too: <script> function setFocusToTextBox(){ $("#mytext").focus(); } </script> Keep in mind that you must draw the input first $(document).ready() In this tutorial, we will learn how to get a currently focused element in JavaScript. The document.activeElement property helps us to access the element that is currently focused in the document. It also shows body or null if there is no focused element. Here is an example: <
But there are many more focusable HTML elements that might be present before input or maybe there is not input element at all inside the modal. Again, full-fledged JavaScript solutions detect and maintain a list of all focusable elements and focus the right one. If you need to support Firefox 2, you can also use this: .focus () and then .blur () something else arbitrary on your page. Since only one element can have the focus, it is transferred to that element and then removed. Works wrong on IE9 - it blurs the whole browser window if active element is document body. Calling element.select () will not necessarily focus the input, so it is often used with HTMLElement.focus. In browsers where it is not supported, it is possible to replace it with a call to HTMLInputElement.setSelectionRange () with parameters 0 and the input's value length: <input onClick="this.select();" value="Sample Text" /> <input ...
Simply, when the.focus () is called, the focus will be added to the beginning of the input element (which is the core problem here), ignoring the fact, that the input element already has a value in it. However, when the value of an input is changed, the cursor is automatically placed at the end of the value inside input element. Definition and Usage The hasFocus () method returns a Boolean value indicating whether the document (or any element inside the document) has focus. In the following, I assume the element we trap focus in is stored in a variable called element. Get focusable elements. In JavaScript we can figure out if elements are focusable, for example by checking if they either are interactive elements or have tabindex. This gives a list of common elements that are focusable:
How To Focus On The Next Field Input In Reactjs Geeksforgeeks
React Createref Vs Useref Difference With Example Codez Up
Trigger Focus Event For Input Element In Jquery
Reactjs Input Element Loses Focus After Keystroke Stack
Set Focus On The Dynamic Input Field In React Clue Mediator
Auto Focus An Input Element In A Blazor Form On Page Load
Solved Please Write Your Solution Injavascript Register T
Selecting Clearing And Focusing On Input In Javascript
How To Focus On A Input Element In React After Render Reactgo
Set The Focus To Html Form Element Using Javascript
Javascript Trap Focus Code Example
Js Animation How To Assign Specific Background For Element
Javascript Event Types 8 Essential Types To Shape Your Js
Element Focus Not Setting Document Activeelement Issue
Simple Example Of Hide Show And Toggle Element Using Jquery
Focus Input Vue 3 Code Example
Introduction To Focus Web Fundamentals Google Developers
0 Response to "20 Javascript Focus Input Element"
Post a Comment