20 How To Create String Array In Javascript
Mar 01, 2021 - Guide to String Array in JavaScript. Here we discuss the function and type with an example which includes traditional array, string array as an object. String.split () Method The String.split () method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as an argument. You can also optionally pass in an integer as a second parameter to specify the number of splits.
How To Replace An Element In Array In Java Code Example
Sep 08, 2017 - In this case, alert would pop-up a 0,1. When it would be an array, it would pop-up a 0, and when alert(array[1]); is called, it should pop-up the 1. Is there any chance to convert such string into a JavaScript array?
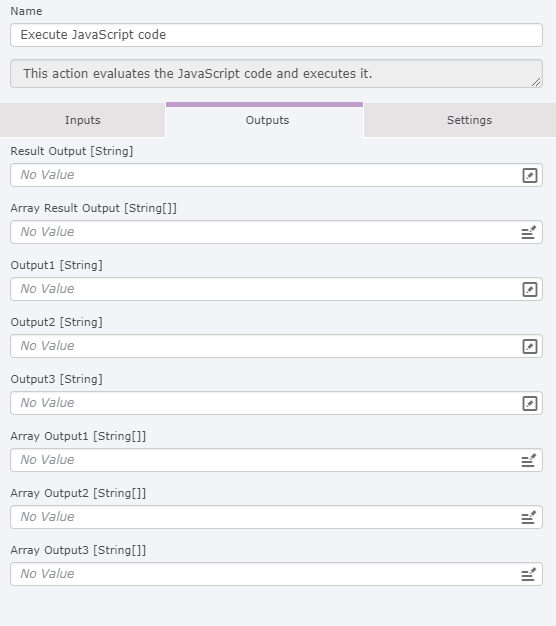
How to create string array in javascript. May 14, 2020 Atta In vanilla JavaScript, you can use the Array.join () method to convert an array into a human-readable string. This method takes an array as input and returns the array as a string. By default, the elements are joined together by using a comma (,) as a separator: The element was removed, but the array still has 3 elements, we can see that arr.length == 3.. That's natural, because delete obj.key removes a value by the key.It's all it does. Fine for objects. But for arrays we usually want the rest of elements to shift and occupy the freed place. In JavaScript, we can create a string in a couple of ways: Using the string literal as a. In general, a string represents a sequence of characters in a programming language. Let's look at an example of a string created using a sequence of characters, ... method combined with other string and array methods. Let's see one here. It could be a ...
you call the Array () constructor with two or more arguments, the arguments will create the array elements. If you only invoke one argument, the argument initializes the length of the new array; the new array's elements are not initialized. Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. This is what's called grapheme clusters - where the user perceives it as 1 single unit, but under the hood, it's in fact made up of multiple units. The newer methods spread and Array.from are better equipped to handle these and will split your string by grapheme clusters 👍 # A caveat about Object.assign ⚠️ One thing to note Object.assign is that it doesn't actually produce a pure array.
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Javascript Object Notation. In layman's terms, we use the JSON.stringfy (ARRAY) function to turn an array or object into a JSON encoded string - Then store or transfer it somewhere. We can then retrieve this JSON encoded string, do the opposite of JSON.parse (STRING) to get the "original" array or object back. USEFUL BITS & LINKS You should use objects when you want the element names to be strings (text). You should use arrays when you want the element names to be numbers. ... JavaScript has a buit in array constructor new Array(). But you can safely use [] instead. These two different statements both create a new empty ...
Jul 20, 2021 - The Array.from() static method creates a new, shallow-copied Array instance from an array-like or iterable object. Javascript Object Notation. Simply put, representing arrays and objects in a flat string format. If you are looking for a way to store or transfer an array as a string - JSON is one of the de-facto industry standards and one that you should know. P.S. JSON.stringify (ARRAY) will turn an array into a string. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
We have seen how to create string using elements of an array. Now we will see how to create an array from a string by using some delimiter. For this we will use split () method which works on a string and create array out of it. This is one of the method used to create an array. Dec 07, 2018 - Occasionally in JavaScript it’s necessary to populate an array with some defaults — an object, string or numbers. What’s the best approach for the job? Lately, I’ve been working with Oleksii Trekhleb… Jan 05, 2021 - The String in JavaScript can be converted to Array in 5 different ways. We will make use of split, Array.from, spread, Object.assign and for loop. Let’s discuss all the methods in brief. ... This method is used to split a string based on a separator provided and returns an Array of substring.
An array in javascript can hold many any types of data that is available in the language. We will see different ways to form a string from different types of array. Using toString() method to create a string from array. Using toString() method on an array returns the string representation of the array. Type of Array in JavaScript with Example. There are two types of string array like integer array or float array. 1. Traditional Array. This is a normal array. In this, we declare an array in such a way the indexing will start from 0 itself. 0 will be followed by 1, 2, 3, ….n. Put a single input field on the page. Add an onfocushandler to it. Check if there is another input element after this one, and if there is, check if it's empty. If there is, don't do anything.
In this article, you will learn how to create an array from a string in Javascript. Let's say you have a string variable named 'a' with value "codesource". var a = "codesource"; In order to create an array from the string, you can use the Array.from() method. In this example, you will be creating an array of characters from the string ... The syntax of creating an array in Java using new keyword −. type [] reference = new type [10]; Where, type is the data type of the elements of the array. reference is the reference that holds the array. And, if you want to populate the array by assigning values to all the elements one by one using the index −. An element inside an array can be of any type, and different elements of the same array can be of different types : string, boolean, even objects or other arrays. This means that it's possible to create an array that has a string in the first position, a number in the second, an object in the third, and so on.
To string, or Not to String. If you were to run the following in your browser: alert([1, 2, 3]); Copy. You would receive an alert with the message 1,2,3 without any additional work on your part. If all you need to do is display the contents of an array in this format, this can get you pretty far. JavaScript's string split method returns an array of substrings obtained by splitting a string on a separator you specify. The separator can be a string or regular expression. An array is a special type of variable that stores multiple values using a special syntax. An array can be created using array literal or Array constructor syntax. Array literal syntax: var stringArray = ["one", "two", "three"]; Array constructor syntax:var numericArray = new Array(3); A single array can store values of different data types.
In this tutorial, we will discuss the string array in Java in detail along with the various operations and conversions that we can carry out on string arrays. Declaring A String Array. A String Array can be declared in two ways i.e. with a size or without specifying the size. Given below are the two ways of declaring a String Array. How to convert a string into an array of characters in JavaScript? Here are 4 ways using the built-in split and 3 new ES6 methods. Read which is best for different scenarios... Given an array containing array elements and here we will join all the array elements to make a single string. To join the array elements we use arr.join () method. This method is used to join the elements of an array into a string. The elements of the string will be separated by a specified separator and its default value is a comma (,).
One of JavaScript's most helpful built-in array methods is .join() (Array.prototype.join()), which returns a string primitive. You can use any separator you want. In fact, the separator string ... The split () method splits a string into an array of substrings, and returns the new array. If an empty string ("") is used as the separator, the string is split between each character. The split () method does not change the original string. The split () method is used to split a string on the basis of a separator. This separator could be defined as a comma to separate the string whenever a comma is encountered. This method returns an array of strings that are separated.
The two-dimensional array is an array of arrays, that is to say, to create an array of one-dimensional array objects. They are arranged as a matrix in the form of rows and columns. JavaScript suggests some methods of creating two-dimensional arrays. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The array can be created in JavaScript, as given below. var country = [ "India" , "England" , "Srilanka" ]; In the above creation, you are initializing an array, and it has been created with values “India”, “England”, and “Srilanka”. The index of “India”, “England”, and “Srilanka” is 0, 1, and 2, respectively.
1 week ago - The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Array.prototype.join () The join () method creates and returns a new string by concatenating all of the elements in an array (or an array-like object ), separated by commas or a specified separator string. If the array has only one item, then that item will be returned without using the separator. You can use various array functions like so: var input = new Array("a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s"); var output = new Array(); var length = 3; for (var i = 0; i < …
First way: Using join () method. The join () method converts the array of elements into a string separated by the commas. Example: const arr = [1,'a','b','c']; console.log(arr.join()); We can also pass our own separator as an argument to the join () method. In this below example, we passed - as a separator argument so that string is separated ... 18/2/2018 · To create an array of strings in JavaScript, simply assign values −. var animals = ["Time", "Money", "Work"]; You can also use the new keyword to create an array of strings in JavaScript −. var animals = new Array ("Time", "Money", "Work"); The Array parameter is a list of strings or integers. Creating an Array There are two ways to create an array in JavaScript: The array literal, which uses square brackets. The array constructor, which uses the new keyword.
Javascript Array Splice Delete Insert And Replace
Difference Between Array And String Difference Between
How To Declare String Array And Assign Value In Array In For
Javascript Array Distinct Ever Wanted To Get Distinct
Create An Array Of Alphabet Characters In Javascript With
Data Structures In Javascript Arrays Hashmaps And Lists
Es6 Way To Clone An Array Samanthaming Com
Javascript Array A Complete Guide For Beginners Dataflair
Angularjs Expressions Array Objects Eval Strings Examples
How To Create An Array Of Strings In C Dynamically Using
How To Remove Commas From Array In Javascript
How To Apply Regular Expression On Array Of Strings String
How To Construct Caml Query For An Array Of Strings Ishir
Gtmtips Create String From Multiple Object Properties Simo
How To Convert String To String Array In Java Javatpoint
Javascript Typed Arrays Javascript Mdn
4 Ways To Convert String To Character Array In Javascript
How To Convert An Array To A String In Javascript
Java String Array Understanding Different Aspects Of String
0 Response to "20 How To Create String Array In Javascript"
Post a Comment