22 Loop Through Classes Javascript
JavaScript for...of loop. The syntax of the for...of loop is: for (element of iterable) { // body of for...of } Here, iterable - an iterable object (array, set, strings, etc). element - items in the iterable. In plain English, you can read the above code as: for every element in the iterable, run the body of the loop. The problem was that I had messed up the javascript code: I had a loop and a subloop. The subloop was also using i as a counter, instead of j, so because the subloop was overriding the value of i of the main loop, ... Using foreach to loop through a class element. 0. What is a better way to handle document.getElementById throwing nullreference ...
Python Loop Tutorial Python For Loop Nested For Loop
JavaScript supports different kinds of loops: for - loops through a block of code a number of times for/in - loops through the properties of an object for/of - loops through the values of an iterable object
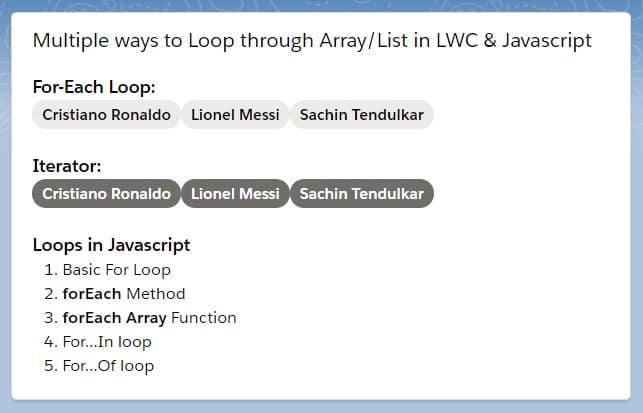
Loop through classes javascript. Note: we used obj.hasOwnProperty(key) method, to make sure that property belongs to that object because for in loop also iterates over an object prototype chain.. Object.keys. The Object.keys() method takes the object as an argument and returns the array with given object keys.. By chaining the Object.keys method with forEach method we can access the key, value pairs of the object. Loops offer a quick and easy way to do something repeatedly. This chapter of the JavaScript Guide introduces the different iteration statements available to JavaScript. You can think of a loop as a computerized version of the game where you tell someone to take X steps in one direction, then Y steps in another. querySelectorAll () returns a NodeList object, which is array-like, but not an array. It has its own forEach () method that can be used to used to loop through it (this is different from the forEach statement used to loop arrays). The NodeList returned by querySelectorAll () can be converted to a proper Javascript array using Array.from () method.
How to loop through a plain JavaScript object with the objects as members. 458. JQuery .each() backwards. 3527. Loop through an array in JavaScript. 668. jQuery to loop through elements with the same class. 257. jQuery: Check if div with certain class name exists. 2210. Iterate through object properties. 1569. Loop inside React JSX. There are many ways to loop through a NodeList object in JavaScript. Let us look at them. forEach() Method. The simplest and easiest way to loop over the results returned by querySelectorAll() is by using the forEach() method. This function executes the given function once for each node in the NodeList. Here is an example: Java Iterator. An Iterator is an object that can be used to loop through collections, like ArrayList and HashSet.It is called an "iterator" because "iterating" is the technical term for looping. To use an Iterator, you must import it from the java.util package.
The 3 methods to loop over Object Properties in JavaScript are: Object.keys (Mozilla Developer reference) Object.entries (Mozilla Developer reference) For-in loop (Mozilla Developer reference) ES6 gave JavaScript some of the new, modern and powerful methods, and one such method is.forEach (). This method is used to loop through all the elements of an array and at the same time execute a provided function for every array's element. The static keyword defines a static method or property for a class. Static members (properties and methods) are called without instantiating their class and cannot be called through a class instance. Static methods are often used to create utility functions for an application, whereas static properties are useful for caches, fixed-configuration ...
We'll use querySelectorAll () to grab all elements with demo-class applied to them, and forEach () to loop through them and apply a change. It is also possible to access a specific element with querySelectorAll () the same way you would with an array — by using bracket notation. Looping Through a String The length Property. The length property has the string length, it simply returns the number of characters in the string:. let str = "hello123"; alert(str.length); // 8 // the last character alert(str[str.length - 1]); // 3. Please note that str.length is a numeric property, not a function. There is no need to add brackets after it. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
Loop through List in Javascript. There are multiple ways to Loop through List in Javascript. Let's go through it one by one. Basic For Loop. If you are reading this post, I don't have to explain this. Just go through below for loop: for(let i=0; i<this.lstAccounts.length; i++){ console.log(this.lstAccounts[i].Name); } forEach Method Now, i want to Loop through the class name of specific div say i want to loop through #div1 which has class name '.news-member-apps' and not the other #div2. and get the value. Note: Here i am copying the previous page DIV content to current page div i.e eg: in #PrevSelectedNews and looping through it. Output: Before clicking the button: After clicking the button: Method 3: Using the basic for loop: The default for loop can be used to iterate through the array and each element can be accessed by its respective index. Syntax: for (i = 0; i . list.length; i++) { // lines of code to execute }. Example:
The Set has method checks if a value is in a Set object, using an approach that is, on average, quicker than testing most of the elements that have previously been added to the Set object. In particular, it is, on average, faster than the Array.prototype.includes method when an Array object has a length equal to a Set object's size. 1. Basic jQuery.each() Function Example. Let's see how the jQuery.each() function helps us in conjunction with a jQuery object. The first example selects all the a elements in the page and ... So, how can you loop through a ClassList in JavaScript? There are multiple approaches here. Firstly, we can split each class name by the blank space and convert the result to an Array: const wrapperElement = document.getElementById ('testWrapper') const classNames = wrapperElement.className.split (' ');
Since the objects in JavaScript can inherit properties from their prototypes, the fo...in statement will loop through those properties as well. To avoid iterating over prototype properties while looping an object, you need to explicitly check if the property belongs to the object by using the hasOwnProperty () method: The forEach () loop was introduced in ES6 (ECMAScript 2015) and it executes the given function once for each element in an array in ascending order. It doesn't execute the callback function for empty array elements. You can use this method to iterate through arrays and NodeLists in JavaScript. You can simply use the jQuery each () method to loop through elements with the same class and perform some action based on the specific condition. The jQuery code in the following example will loop through each DIV elements and highlight the background of only those elements which are empty. Let's try it out:
Generator functions are written using the function* syntax. When called, generator functions do not initially execute their code. Instead, they return a special type of iterator, called a Generator. When a value is consumed by calling the generator's next method, the Generator function executes until it encounters the yield keyword. Initialization - initializes the loop variable with a starting value which can only be executed once. Condition - specifies that the loop should stop looping; Final expression - is performed at the end of each loop execution. It is used to increment the index. for...in¶ The for...in loops through the properties of an object. It is among the ... This example loops through the page input elements and then automatically puts a check-mark on the first checkbox that the loop encounters on the page. This hopefully shows you some of the power of using pure JavaScript and also shows that using pure JavaScript is not necessarily all that much more complicated.
Looping Through Object Properties JavaScript has a built-in type of for loop that is specifically meant for iterating over the properties of an object. This is known as the for...in loop. Here is a simplified version of our main object example, gimli. How to loop through objects keys and values in Javascript? A common problem faced by programers is looping over an enumerable dataset. This data can come in the form of arrays, lists, maps or other objects. In this article we will deal with this problem and learn 4 ways to loop through objects using javascript to retrieve multiple key-value pairs.
Object Oriented Javascript For Beginners Learn Web
Tools Qa How To Use Javascript Classes Class Constructor
The Most Common Patterns To Create Objects In Javascript Es5
Object Oriented Javascript For Beginners Learn Web
Javascript Loop Through Class Elements Code Example
16 Daily Javascript Array Concat
3 Methods To Loop Over Object Properties In Javascript In
Loop Through List In Lwc And Javascript Niks Developer
Code Navigation In Visual Studio Code
Safe Ways To Inject Html Through Javascript Ta Digital Labs
Three Different Ways To Loop Through Javascript Objects By
Node Properties Type Tag And Contents
Learn Javascript Full Course For Beginners
How To Parse Outlook Emails And Show In Excel Worksheet Using Vba
How To Loop Through Input Elements In Jquery Geeksforgeeks
How To Create Modify And Loop Through Objects In Javascript
Python Loop Tutorial Python For Loop Nested For Loop
Javascript While And Do While Loop With Examples
Javascript How To Extend A Class
0 Response to "22 Loop Through Classes Javascript"
Post a Comment