23 Concat Array Of Arrays Javascript
The original Clojure version can be reimplemented in JavaScript: function reductions (xs, f, init) { if (typeof (init) === "undefined") { return reductions (_.rest (xs), f, _.first (xs)) } if (xs.length === 0) return [init] var y = f (init, _.first (xs)) return [init].concat (reductions (_.rest (xs), f, y)) } In JavaScript there are manyways to merge or combine arrays let's learn the most commonly used methods to merging arrays. Array.Concat( ) method. JavaScript concat method creates the new array by merging two or more arrays instead of mutating the existing arrays.
15 Common Operations On Arrays In Javascript Cheatsheet
To merge two or more arrays in JavaScript, the concat () function is used. The syntax for concatenating multiple arrays using the concat method is: array_name. concat ( first_array, second_array, …, N_array) The concat () function takes multiple arrays as a parameter and joins them together as one array. The concat () function returns a new ...
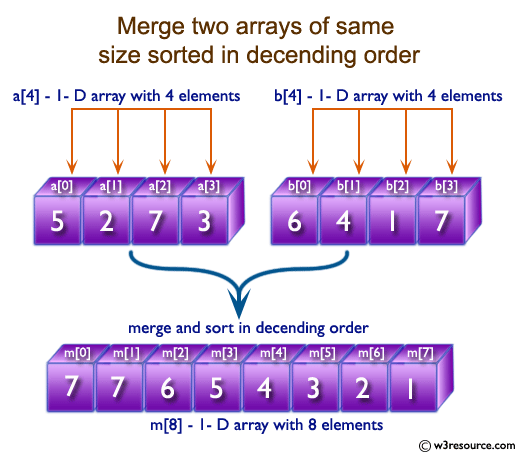
Concat array of arrays javascript. The arr.concat () method is used to merge two or more arrays together. This method does not alter the original arrays passed as arguments. filter() is used to identify if an item is present in the "merged_array" or not. Just like the traditional for loop, filter uses the indexOf() method to judge the occurrence of an item in the merged_array. Pseudo Code for the ES5 Solution. concat() the arrays together, and store in another array (A) Use the filter() to iterate through array ... Lo and behold, here's the difference on Chrome: 👉 Link to the test on JsPerf. To merge arrays of size 10 for 10,000 times, .concat performs at 0.40 ops/sec, while .push performs at 378 ops/sec. push is 945x faster than concat! This difference might not be linear, but it is already is already significant at this small scale.
JavaScript has a built-in method for merging strings or arrays together called concat (). It allows you to take an array, combine it with one or many different arrays and make a new array containing the elements from all the arrays - the same principle applies to strings. JavaScript Array Concat refers to combining of two or more arrays which in turn returns a new array. Combining different arrays does not result in the change of data in existing individual arrays. For concatenation, we use concat () method or Array.concat () function. Let us see the syntax and how it works. Summary: in this tutorial, you will learn how to use the JavaScript Array concat () method to merge two or more arrays into a single array. To merge two or more arrays, you use the concat () method of an Array object. The concat () method returns a new array and doesn’t change the original arrays.
The array concat method and some other ways of adding two arrays together So there is adding two strings or numbers together with the addition operator in javaScript, but then there is adding two or more objects together including Arrays and how such an operation should be handled. The syntax of concat () method is as follows − array.concat (value1, value2,..., valueN); valueN − Arrays and/or values to concatenate to the resulting array. First, declare an empty array and then use the push () method with the spread operator. The contents of the arrays will be copied in the desired array. Remember, if you don't use the spread...
You can use apply () method of concat and pass the given array of arrays as a second parameter to the apply method as shown in the example below. Let's say myarrays is the given array of arrays and you need to convert it to a flat array. var myarrays = [ ["10"], Provide more Array arguments to array.concat(). var arrConc = array1.concat(array2, array3, array4); Joining two arrays may sound like an easy task yet looking, for example, at this StackOverflow question and the number of answers (let's note that none was accepted up to this day!) it may cause a bit of headache.. While we can use external libraries to join arrays, we can simply rely on plain JavaScript.
Append an Array to an Array of Arrays in JavaScript admin July 22, 2015 I started working with JavaScript last week in order to create some D3 visualizations, and have become rather stuck on what can only be a very simple task. The JavaScript concat () method concatenates two or more arrays into a single array. The concat () method returns the concatenated array and does not change the existing arrays. Find the syntax of concat () method. const new_array = array1.concat(array2, array3, ...arrayN) Find a sample example. The concat () method is used to merge two or more arrays. This method does not change the existing arrays, but instead returns a new array.
The JavaScript array concat () method combines two or more arrays and returns a new string. This method doesn't make any change in the original array. Let's see two ways in which concat () method can be used while working with arrays in JavaScript. 1) Merging two or more arrays Let's now see how we can use the concat method to merge two given arrays, array 1 and array 2 Definition and Usage The concat () method concatenates (joins) two or more arrays. The concat () method does not change the existing arrays, but returns a new array, containing the values of the joined arrays.
arr = arr.concat(arr1).concat(arr2).concat(arr3) Or see @Radko Dinev answer for an even simpler (and better) way to do it. If you have an array of arrays (with a variable number of arrays), you can try: Javascript add array to array. To add an array to an array in JavaScript, use the array.concat() or array.push() method. The array concat() is a built-in method that concatenates two or more arrays. The concat() function does not change the existing arrays but returns a new array containing the values of the joined arrays. array.concat() I am trying to concat a multidimensional array so I can iterate and display all the data. There is a very good post on how to access nested data here, but I am looking for a solution that works with a specific data structure.. Here is my data:
JavaScript offers multiple ways to merge arrays. You can use either the spread operator [...array1,...array2], or a functional way [].concat (array1, array2) to merge 2 or more arrays. These approaches are immutable because the merge result is stored in a new array. Example 2: Concatenating nested arrays. The concat() method returns the shallow copy of the concatenated elements in the following way: It copies object references to the new array. (For example: passing a nested array) So if the referenced object is modified, the changes are visible in the returned new array. 2220. You can use concat to merge arrays: var arrays = [ ["$6"], ["$12"], ["$25"], ["$25"], ["$18"], ["$22"], ["$10"] ]; var merged = [].concat.apply ( [], arrays); console.log (merged); Using the apply method of concat will just take the second parameter as an array, so the last line is identical to this:
Array.prototype.flat () The flat () method creates a new array with all sub-array elements concatenated into it recursively up to the specified depth. Description The concat () method is used to join two or more arrays. Concat does not alter the original arrays, it returns a copy of the same elements combined from the original arrays. Watch JavaScript array object concat method video tutorial. 11/6/2021 · JavaScript arrays can contain values of different types. If you only want to concatenate strings, you can filter out non-string values using filter () and typeof as shown below. let array = ['The', 97, 'Dream', 'Team']; let jumble = array.join (); jumble; // 'The 97 Dream Team' let text = array.filter (v => typeof v === 'string').join (); text; // ...
Merge Arrays Javascript Concat Method Tuts Make
How To Merge Two Unsorted Arrays In Sorted Order In Java
How To Merge Arrays Together In Javascript Weekly Webtips
Merge Multiple Arrays Into One In Jquery
Javascript Lesson 22 Concat Method In Javascript Geeksread
C Exercises Merge Two Arrays Of Same Size Sorted In
Reduce Flatten An Array Of Arrays Dev Community
Merge Two Sorted Arrays With O 1 Extra Space Geeksforgeeks
How To Merge Array Of Objects In Single Array In Javascript
Concatenate Multiple Arrays Javascript Code Example
Javascript Array How To Merge Two Arrays In Javascript
Javascript Array Push Is 945x Faster Than Array Concat
How To Merge Two Arrays In Javascript And De Duplicate Items
Js Array Concat Explained Step By Step By Abdullah Medium
Javascript Array Concat Performance
Arrays In Javascript How To Create Arrays In Javascript
Javascript Array Push Is 945x Faster Than Array Concat
Javascript Array Concat Method Merge Add Two Arrays
Javascript Array Concat How To Concat Array In Javascript
How To Merge Two Arrays In Javascript By Jonathan Hsu
Hacks For Creating Javascript Arrays
Javascript Array Of Arrays Get One Row Code Example
0 Response to "23 Concat Array Of Arrays Javascript"
Post a Comment