33 Javascript Change Value Of Array Element
JavaScript Array elements can be removed from the end of an array by setting the length property to a value less than the current value. Any element whose index is greater than or equal to the new length will be removed. var input = document.getElementsByName ('array []'); The document.getElementsByName () method is used to return all the values stored under a particular name and thus making input variable an array indexed from 0 to number of inputs. The method document.getElementById ().innerHTML is used to change the inner HTML of selected Id.
Array.find is also another method we can use to check if our Array contains a certain value. This method will return the value itself or undefined if no value is found so we can use the !! operator to convert the result to boolean and quickly see if there's a match or not.
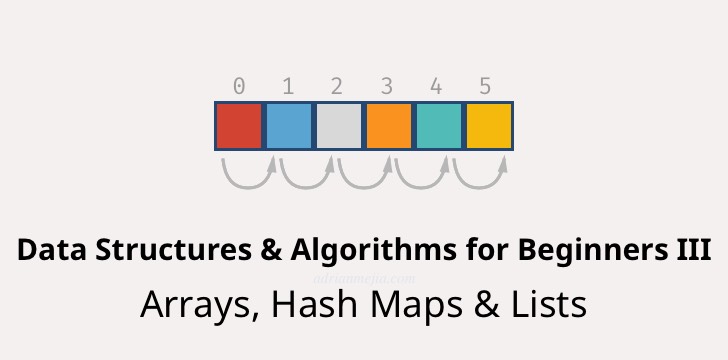
Javascript change value of array element. Top 8 Methods in Multi-Dimensional Array in JavaScript. Below are the methods used in Multi-Dimensional Array in JavaScript: 1. Pop ( ) This method is used to remove the element at the last index of the array. This will eventually result in the array length decreased by 1. While an element removed from an array then array size must be decreased and if an element added to an array then array size becomes increased. Arrays are used to store homogenous elements means the same type of elements can be stored at a time. callbackFn is invoked with three arguments: the value of the element, the index of the element, and the array object being mapped. If a thisArg parameter is provided, it will be used as callback's this value. Otherwise, the value undefined will be used as its this value.
How could we change the value of just key in just one object? Step 1: Find the element. We first want to find the index in the array of the object, or where the object is located in the array. You can find the element by any key, id or name, or any other you find useful. We will use its id that e.g. we are getting from the function . I want to make a button that changes the value of an element in an array. I am trying to do it by the following code but the element does not change. As a self learning beginner, I am probably missing something very obvious and I would appreciate if someone can point that out to me. Thank you for your answers! An array in JavaScript is a type of global object that is used to store data. ... one that uses slice() to store the seaCreatures array from the first element until whale, and a second variable to store the elements pufferfish and lobster. To join the two arrays, we'll use the concat() ... If we wanted to change the value of seahorse, ...
In JavaScript, you can use the Array.map() method to iterate over all elements and then use the string methods to change the case of the elements. Here is an example that demonstrates how to use the String.toUpperCase() method along with Array.map() to uppercase all elements in an array: 13/6/2019 · shift () removes the first item of an array and returns it unshift () adds an item (s) to the beginning of an array and changes the original array. splice () c hanges an array, by adding, removing and inserting elements. slice () copies a given part of an array and returns that copied part as a new array. We can use the spice () method on our array which is used to add and remove elements from an array. This method takes the first argument as an index which specifies the position of the element to be added or removed. The next argument it takes is the number of elements to be removed and is optional.
Java queries related to "how to replace an element in array in java" how to replace all items in an arraylist java; how to replace an item in an arraylist java; can one change an element in an array java; can you change element in array java; replace value at idx in arraylist in java; how to replace value in arraylist javascript The JavaScript function above takes in two parameters. The unique ID of our select element and the value that we want to select. Inside our function, we loop through each option in the select element. If the value of the option matches the value that we want to select, we change the selectedIndex of the element and break out of the for loop. Change the class value using classList property The classList property is a read-only property of an HTML element that returns the class attribute value as an array. The property also provides several methods for you to manipulate the class attribute value.
How to change value of object which is inside an array using JavaScript or jQuery? - Stack Overflow. The code below comes from jQuery UI Autocomplete:var projects = [ { value: "jquery", label: "jQuery", desc: "the write less, do more, JavaScript library", icon: ". Stack Overflow. 15/7/2019 · An item can be replaced in an array using two approaches: Method 1: Using splice () method. The array type in JavaScript provides us with splice () method that helps us in order to replace the items of an existing array by removing and inserting new elements at the required/desired index. Approach: JavaScript provides us an alternate array method called lastIndexOf (). As the name suggests, it returns the position of the last occurrence of the items in an array. The lastIndexOf () starts searching the array from the end and stops at the beginning of the array. You can also specify a second parameter to exclude items at the end.
Javascript change value of array element. Thank you for your answers! The score of an array is calculated by performing the following operations on the array until the size of the array is greater than 2: Select an index i such that 1 < i < N 4 of the V8 JavaScript engine Since we are creating contacts, the operation value would be create Print out the values of the array elements with this statement: console.log (people); The JavaScript Console returns the same list of elements that you put in in the previous step. Change the value of the first element by entering this statement, and then press Return or Enter: people [0] = "Georgie"; Print the values of the array's element ... update object in array by id javascript. update field in array object javascript. best way to update an object in array javascript. in array of objects update value. find an object in array and update the value obj in array node. find an object in array and update the value node.
6/7/2020 · Array.splice will modify your original array and return the removed elements so you can do the following: const arr = [1, 2, 3, 4, 5] const index = arr.indexOf(2) const splicedArr = arr.splice(index, 1) arr // [1,3,4,5]; splicedArr // [2] When there are duplicate elements, the indexOf() method returns the index of the first element it found inside the array. Knowing how the indexOf() method works, you can set a filter condition where the element's indexOf() value matches the actual index passed by the filter() method to the callback function. Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
The HTML DOM allows JavaScript to change the content of HTML elements. Changing HTML Content The easiest way to modify the content of an HTML element is by using the innerHTML property. Definition and Usage. The findIndex() method returns the index of the first array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, findIndex() returns the index of that array element (and does not check the remaining values) Converting Arrays to Strings The JavaScript method toString () converts an array to a string of (comma separated) array values.
The compare function compares all the values in the array, two values at a time (a, b). When comparing 40 and 100, the sort() method calls the compare function(40, 100). The function calculates 40 - 100 (a - b), and since the result is negative (-60), the sort function will sort 40 as a value lower than 100. See the Pen JavaScript - Move an array element from one position to another-array-ex- 38 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to create a specified number of elements and pre-filled string value array. To delete elements in an array, you pass two arguments into the splice () method as follows: Array .splice (position,num); Code language: JavaScript (javascript) The position specifies the position of the first item to delete and the num argument determines the number of elements to delete. The splice () method changes the original array and ...
22/1/2018 · The array.values() function is an inbuilt function in JavaScript which is used to returns a new array Iterator object that contains the values for each index in the array i.e, it prints all the elements of the array. Syntax: arr.values() Return values: It returns a new array iterator object i.e, elements of the given array… The Array.prototype.map() method was introduced in ES6 (ECMAScript 2015) for iterating and manipulating elements of an array in one go. This method creates a new array by executing the given function for each element in the array. The Array.map() method accepts a callback function as a parameter that you want to invoke for each item in the array. This function must return a value after ... Javascript answers related to "how to change single value in array objects in javascript". 6 ways to modify an array javascript. angularjs find and update object in array. change value in array react. change value of each property of object array javascript. change value of key in array of objects javascript.
How To Filter Out Only Numbers In An Array Using Javascript
Replace Item In Array With Javascript
How To Swap Two Array Elements In Javascript
Numpy Array Object Exercises Practice Solution W3resource
Javascript How To Update An Array Element Meshworld
Javascript Array Distinct Ever Wanted To Get Distinct
Change Array Index Position In Javascript By Up And Down
Find Minimum And Maximum Value In An Array Interview
Javascript Array Splice Delete Insert And Replace
Java Exercises Find The Index Of An Array Element W3resource
5 Working With Arrays And Loops Javascript Cookbook Book
Javascript Array Move An Array Element From One Position To
Javascript Set Input Value By Class Name Change Value
Use Push To Insert Elements Into An Array
Php Array Replace Example Array Replace Function Tutorial
4 Ways To Convert String To Character Array In Javascript
One Reduce To Rule Them All How To Use Reduce In
How To Implement Array Like Functionality In Sql Server
Data Structures In Javascript Arrays Hashmaps And Lists
How To Get Values From Html Input Array Using Javascript
Javascript Array Splice Delete Insert And Replace
6 1 Array Creation And Access Ap Csawesome
Javascript Move Item In Array To Another Index Code Example
C Exercises Update Every Array Element By Multiplication
Javascript Array A Complete Guide For Beginners Dataflair
The Fastest Way To Remove A Specific Item From An Array In
Page Items And Javascript More Than S And V
How To Find Even Numbers In An Array Using Javascript
Javascript Nested Array How Does Nested Array Work In
Hacks For Creating Javascript Arrays
Data Structures Objects And Arrays Eloquent Javascript
0 Response to "33 Javascript Change Value Of Array Element"
Post a Comment