25 Call An Api From Javascript
Dec 01, 2020 - How do I call a Rest API service that I've defined in the Logic tab from Javascript? and Can I pass in a dynamic request object to the API without a fixed request structure · From Javascript you can call a client action which calls a server action which has the call for the API Start with the new Fetch API, supported by all browsers except IE11 at the time of writing. It simplifies the XMLHttpRequest syntax you see in many of the other examples. The API includes a lot more, but start with the fetch() method. It takes two arguments: A URL or an object representing the request.
How To Make An Api Call In React App By Ragunath
To summarise, you can use AJAX when you want to call a PHP function from JavaScript or run PHP code on some data generated inside browsers. You can use echo in PHP to output JavaScript code which will run later in the client's browser.
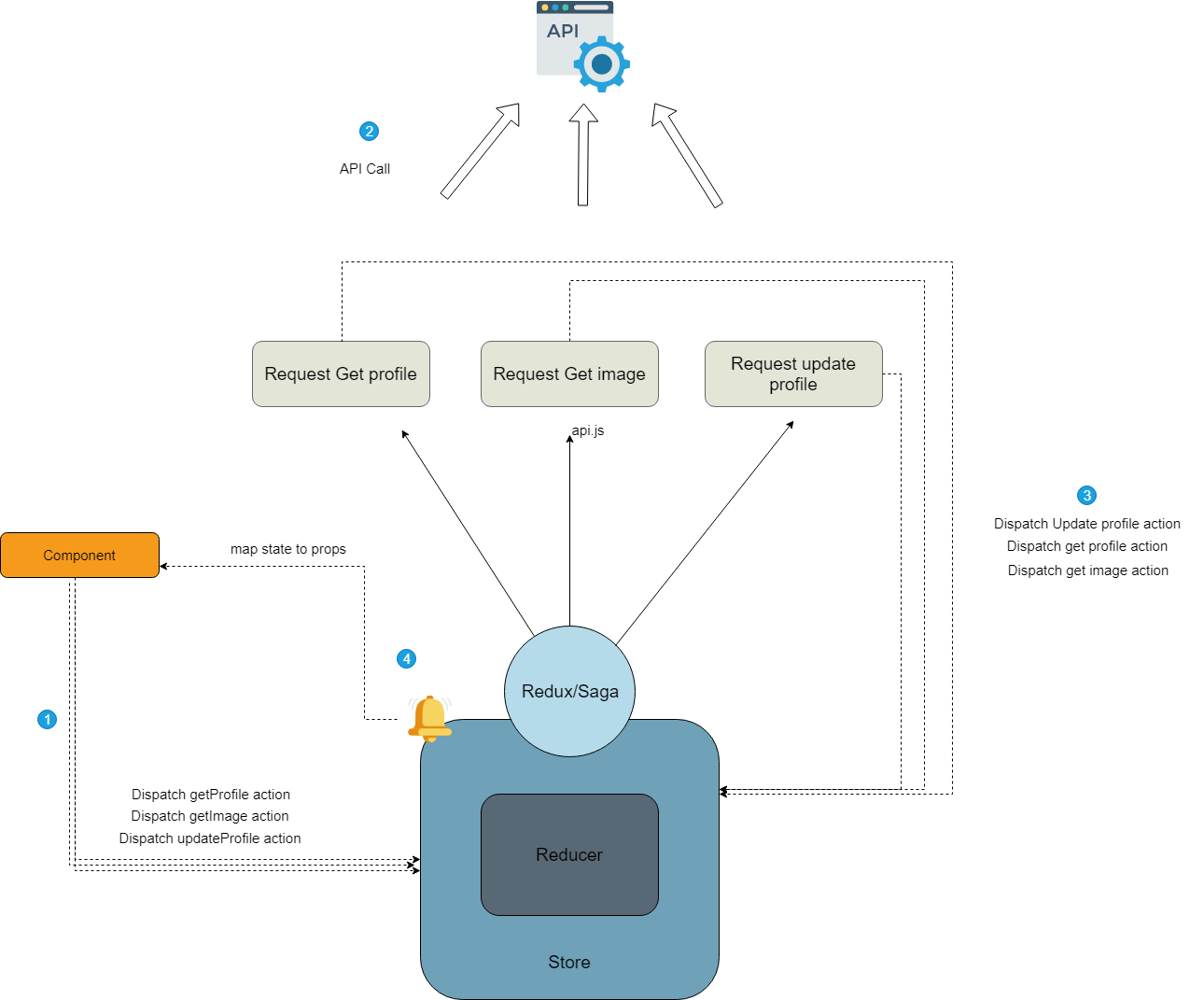
Call an api from javascript. Sep 21, 2020 - Read the Promises section of this ... loop, callbacks, promises, and async/await in JavaScript. ... The fetch() method returns a promise. If the promise returned is resolve, the function within the then() method is executed. That function contains the code for handling the data received from the API... The most Popular way to call a REST API from JavaScript is with the XMLHttpRequest (XHR) object. You can perform data communication from a URL of the Web API without having to do a full page refresh. Other methods for calling APIS in JavaScript are Fetch API and Promise. Call Web API GET method from JavaScript For each of these actions, JAAS API provides a corresponding endpoint. Browse APIs In order to demonstrate the entire CRUD functionality in JavaScript, we will complete the following steps: Make a POST request for the API used to create the object. We will save object id which was received in the answer.
Approach: First make the necessary JavaScript file, HTML file and CSS file. Then store the API URL in a variable (here api_url). Define a async function (here getapi ()) and pass api_url in that function. Define a constant response and store the fetched data by await fetch () method. May 21, 2018 - AJAX is the primary method you use to get and send data to APIs in JavaScript. Making AJAX requests with the XMLHttpRequest() method, often referred to as XHR, is a three step process: Set up our request by creating a new XMLHttpRequest(). Create an onload callback to run when the request completes. Apart from directly making an Ajax call with JavaScript, there are other more powerful methods of making an HTTP call such as $.Ajax which is a jQuery method. I'll discuss those now. jQuery methods. jQuery has many methods to easily handle HTTP requests.
Mar 03, 2021 - Free source code and tutorials for Software developers and Architects.; Updated: 5 Mar 2021 Aug 04, 2020 - The same POST API call in various JavaScript libraries ... I was testing an API using Insomnia, a very cool application that lets you perform HTTP requests to REST API or GraphQL API services. They have a nice button that generates code to replica an API request from the app, where you design ... Today's post will go through the process of calling -both- the Microsoft Graph API and your own API from the same code base. Starting Knowledge Assumption My assumption is that you are already familiar with the basics of Oauth, where you're aware that a Single Page Application (SPA) is using an " Implicit Grant Flow ".
Check it out the Fetch API demo.. Summary. The Fetch API allows you to asynchronously request for a resource. Use the fetch() method to return a promise that resolves into a Response object. To get the actual data, you call one of the methods of the Response object e.g., text() or json().These methods resolve into the actual data. One of the best features of jQuery AJAX Method is to load data from external website by calling APIs, and get the response in JSON or XML formats. In this example I will show you how easy it is to make such API calls in jQuery AJAX. OpenWeatherMap API. The OpenWeatherMap API provides the complete weather information for any location on Earth including over 200,000 cities. 6 Different ways to do Ajax calls in JavaScript. There are so many options in different ways to call Ajax in JavaScript that can improve user experiences drastically like submitting data to the ...
Jul 18, 2019 - The modern web development world is impossible to imagine without JavaScript. Over the years of its growth, this language has gone from a small add-on to a multifunctional and powerful tool. Today… In this article, we will use jQuery and JavaScript for calling the Web API. We know that the ASP. Net Web API is a framework for creating Web APIs of the .NET Framework. In this article, we will use the ASP. Net Web API to create a Web API that returns a list of items. 1 week ago - The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It also provides a global fetch() method that provides an easy, logical way to fetch resources asynchronously across the network.
Mar 28, 2021 - Hello developers!! In this post, we'll discuss various ways to make an API call for your next project. 🔎 XML HTTP Request All modern browsers support the XMLHttpRequest object to request data from a server. It works on the oldest browsers as well a... Aug 10, 2020 - As much as developers enjoy building things from scratch, some times we have to work with already existing data. Asides from the fact that using already existing data saves a lot of time (so that we… Aug 05, 2020 - To make API calls using JavaScript, a reference can be made under the <script> tag to the JavaScript library which contains functions and other configuration parameters pertaining to the API should be made. A good API always maintains appropriate documentation to its functions and parameters.
Aug 15, 2018 - Then the arguments can be inserted into input type=hidden value fields using JavaScript and the form can be submitted from the button click event listener or onclick event using one line of JavaScript. Here is an example that assumes the REST API is in file REST.php: Feb 21, 2019 - I take this approach as to not conflate Node.js with the power of Express and APIs since most tutorials do. Rather, I’ll provide one method (of many) by which to call and receive data from an external API which utilizes a third-party JavaScript library. The API we’ll be calling is a Weather ... According to the developer dashboard, your access to the Forecast API will be "cut off" after the one thousandth call. This is a hint that you'll have to handle this edge case in a later step! 3. Hit the API endpoint(s) in JavaScript. In the previous step, you used your browser or a REST client to check the API response.
February 4th, 2021. Bryan Soltis demonstrates how to call an API in Power Apps Portals using JavaScript. As a developer, Power Apps are a very interesting, yet nostalgic, concept. The platform reminds me of a lot of "game changing and exciting" systems of the past that allowed users to create complex, functional applications quickly, with ... Jul 07, 2020 - All possible ways of making an API call in JavaScript. ... In JavaScript, it was really important to know how to make HTTP requests and retrieve the dynamic data from the server/database. Web API, introduced in Dynamics CRM 2016, can be used from within CRM and also Outside CRM. To call Web API from JavaScript outside of CRM we have to implement authentication. In previous versions of Dynamics CRM, CORS was not implemented, so we cannot authenticate or can get Access Token from browsers.
May 07, 2020 - Apart from directly making an Ajax call with JavaScript, there are other more powerful methods of making an HTTP call such as $.Ajax which is a jQuery method. I’ll discuss those now. Nov 26, 2019 - The fetch function returns a Promise ... contains an HTTP response represented as a Response object. A common pattern is to extract the JSON response body by invoking the json function on the Response object. JavaScript updates the page with the details from the web API's response. The simplest fetch call accepts a ... As you may know, there are a lot of options to call API from JavaScript. Some of them are XMLHttpRequest, jQuery, fetch and so one. I am going to talk about the fetch method here because it looks cleaner in our code. Using fetch is very simple.
You can safely remove the third parameter from your code. Synchronous XMLHttpRequest (async = false) is not recommended because the JavaScript will stop executing until the server response is ready. If the server is busy or slow, the application will hang or stop. ... GET is simpler and faster ... app.run (debug = True) Note: You can host this API by simply running the above python code. JS Script: Include axios.js and the corresponding JS file in the HTML file. In the JS file, write the following code which makes a GET request using Axios to the API. A GET request to the API requires the path to the API method . May 10, 2020 - Usually, in software development, ... is called an Application Programming Interface. So, in simple terms, APIs are a way to communicate with an application. In fact, in programming, we are continuously using APIs without even realizing that we are. Remember the first JavaScript "console" statement that we wrote, it was an API from the ...
The SPA generated by this guide calls acquireTokenSilent and/or acquireTokenPopup to acquire an access token used to query the Microsoft Graph API for user profile info. If you need a sample that validates the ID token, take a look at this sample application in GitHub. The sample uses an ASP.NET web API for token validation. Get a user token ... Apr 26, 2021 - Angular and Ember) tend to be packages of HTML, CSS, JavaScript, and other technologies that you install and then use to write an entire web application from scratch. The key difference between a library and a framework is “Inversion of Control”. When calling a method from a library, the ... The easiest way to call a REST API in JavaScript is to use the fetch library. It is built into the standard JavaScript library and can be used to make all sorts of HTTP calls. Documentation for the fetch library can be found at Moz but we will cover everything you need to interact with a REST API in this tutorial.
Since an API can be accessed by many different methods - JavaScript, PHP, Ruby, Python and so on - the documentation for most APIs doesn't tend to give specific instructions for how to connect. We can see from this documentation that it tells us we can make requests with curl or regular REST calls, but we might not have a clue how to do that yet. Call APIs from JavaScript By default, you can't make WebSocket connections or calls to third-party APIs from JavaScript code. To do so, add a remote site as a CSP Trusted Site. The Lightning Component framework uses Content Security Policy (CSP), which is a W3C standard, to control the source of content that can be loaded on a page. Calling Google News RESTful Web Service with JavaScript / jQuery. This guide walks you through writing a simple javascript/jquery to calling rest web service. When we call Google News API and web service with the help of javascript and jquery then we will get the JSON response given below. "description": "The number of people killed in China by ...
This is just a fun exercise for me because I was curious to see how I can use JS to make JIra API calls. My recommendation - however - is not to use this simple approach. User OAuth to make API calls. It is much more secure than the approach described in the link above. Here is the link on how to implement OAuth ( in Java) and make calls to ... The Web API Model can be serialized automatically in JavaScript Object Notation (JSON) and Extensible Markup Language (XML) format. We can use the model to represent the data in our application. To add a new model in the application, go to the Solution Explorer and right-click the "Model" folder. The last thing to do on the server side is to add an API endpoint that requires an access token to be provided for the call to succeed. This endpoint will use the middleware that you created earlier in the tutorial to provide that protection in a scalable way.
How To Use An Api With Javascript Beginner S Guide
Api Call In Next Js Clue Mediator
How To Connect To An Api In Javascript Geeksforgeeks
How To Handle Inbound Phone Calls With Node Js Developer
Calling Web Api From Javascript Or Jquery Asp Net Core Web Api
Explore Office Javascript Api Using Script Lab Office Add
All Possible Ways Of Making An Api Call In Javascript By
Beginners Guide To Fetching Data With Ajax Fetch Api
All Possible Ways Of Making An Api Call In Javascript By
Calling Rest Api From Javascript Example Call Rest Google
Learn To Use Fetch In Api Call Easily
Bug Vue Composition Api Esm Js A6f4 37 Uncaught Error Vue
Calling The Web Api With Javascript And Jquery
Browser Console With Js Agent Apiary Help
Calling Photon Functions From Javascript
Display Database Data In Frontend Via Api Call Questions
Four Ways To Make An Api Calls In Javascript
How To Use The Results From One Api Call In Another Api Call
Another Way To Call Restful Api In Redux Hacker Noon
Excel Javascript Api Stock Data By Peter James
Here Are The Most Popular Ways To Make An Http Request In
What Is The Best Way To Call A Rest Api From Javascript
0 Response to "25 Call An Api From Javascript"
Post a Comment