28 Javascript Module Design Pattern
Oct 04, 2012 - Write powerful, clean and maintainable JavaScript. RRP $11.95 · Get the book free! In this article, I’ll be describing the structure and benefits of an extended modular design patterns, that includes four principal member types: For the full set, see JavaScript Design Patterns. JavaScript modules are the most prevalently used design patterns for keeping particular pieces of code independent of other components. This provides loose coupling to support well-structured code. For those that are familiar with object-oriented languages, modules are JavaScript "classes".
Module Pattern In Javascript Dzone Web Dev
Module Design Pattern. The module design pattern is the most relevant an widely used design pattern in JavaScript development and it provides code modularization and encapsulation. Mostly the module objects will be a singleton and it does allow us to write well-structured code and using this pattern we can achieve public and private access ...
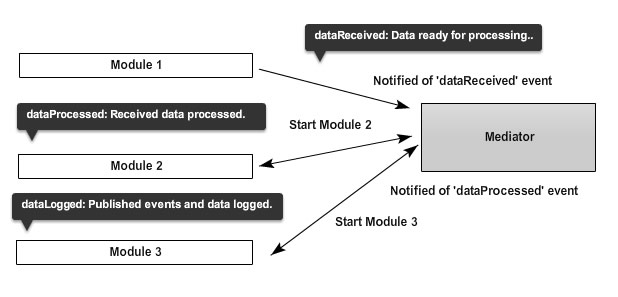
Javascript module design pattern. Jan 29, 2014 - I’m a massive fan of JavaScript’s Module Pattern and I’d like to share some use cases and differences in the pattern, and why they’re important. The Module Pattern is what we’d call a “design pattern,”and it’s extremely useful for a vast amount of reasons. Jul 22, 2016 - In JavaScript, the word “modules” refers to small units of independent, reusable code. They are the foundation of many JavaScript design patterns and are critically necessary when building any… 28/9/2020 · Concepts: The JavaScript Module Design Pattern In this topic, you will learn how to use the JavaScript Module Design Pattern to reduce the chance that your code will conflict with other scripts on your web page. Scope conflicts. In JavaScript, when you define a variable using the var element
Jun 04, 2015 - Note: which seem much better than the one from "Learning JavaScript Design Patterns" – Adrien Be Sep 15 '14 at 15:01 ... I thought i'd expand on the above answer by talking about how you'd fit modules together into an application. I'd read about this in the doug crockford book but being new ... The Revealing Module Pattern - Learning JavaScript Design Patterns [Book] The Revealing Module Pattern Now that we're a little more familiar with the Module pattern, let's take a look at a slightly improved version—Christian Heilmann's Revealing Module pattern. There is also a type of export called the default export — this is designed to make it easy to have a default function provided by a module, and also helps JavaScript modules to interoperate with existing CommonJS and AMD module systems (as explained nicely in ES6 In Depth: Modules by Jason Orendorff; search for "Default exports").
Design pattern is a broad topic, but by understanding the module pattern and the factory method you should get to grips with it. The Module Pattern. JavaScript modules were introduced in 2009, with the ES5 version of the programming language. To explore the module design pattern further, let's write a quick JavaScript module called fruitBasket. To begin, let's start with an immediately invoked function expression (IIFE), which is a ... Jul 07, 2021 - Now that we know what design patterns are, and why they are important, let’s dive into various design patterns used in JavaScript. ... A Module is a piece of self-contained code so we can update the Module without affecting the other parts of the code. Modules also allow us to avoid namespace ...
JavaScript Module Design Pattern by Aditya Jain · January 2, 2014 JavaScript Module pattern provides a way to wrap public, private methods (and variable) into a single entity and exposing only the public members to the world outside of module. Any JavaScript developer has either seen the keyword prototype, confused by the prototypical inheritance, or implemented prototypes in their code. The Prototype design pattern relies on the JavaScript prototypical inheritance. The prototype model is used mainly for creating objects in performance-intensive situations. 28/12/2019 · It doesn’t actually create a module pattern, but it can help you create a proper module pattern. Conclusion. A module pattern in JavaScript is absolutely useful and powerful. It conceals the variables and functions within the scope in which they are declared. And Closure is the root of this concept. Resources. JavaScript module pattern basis
Aug 19, 2018 - An expert web developer gives an overview of the concepts behind the module pattern in programming, and how to implement it in the JavaScript language. Module design pattern Modules in javascript provide loose coupling and independent working of certain code. One of the most important features of object-oriented programming is classes that provide encapsulation or data hiding and eventually make our data in code more secure. The Module Pattern is one of the most common design patterns used in JavaScript and for good reason. The module pattern is easy to use and creates encapsulation of our code. Modules are commonly used as singleton style objects where only one instance exists. The Module Pattern is great for services and testing/TDD.
Module The module pattern simply allows you to keep units of code cleanly separated and organized. Modules promote encapsulation, which means the variables and functions are kept private inside the module body and can't be overwritten. Creating a module in ES6 is quite simple. JavaScript modules are the most prevalently used design patterns for keeping particular pieces of code independent of other components. This provides loose coupling to support well-structured code. For those that are familiar with object-oriented languages, modules are JavaScript “classes”. Design patterns are intelligent, reusable strategies for solving common development problems faced by developers. For Web developers working with JavaScript, design patterns provide a tested, methodical plan of attack for tackling challenges that arise in real-world application development.
The Module Pattern Modules are an integral piece of any robust application’s architecture and typically help in keeping the units of code for a project both cleanly separated and organized. … - Selection from Learning JavaScript Design Patterns [Book] You may not know it, but you've used a JavaScript design pattern. Design patterns are reusable solutions to commonly occurring problems in software design. During any language's lifespan, many such reusable solutions are made and tested by a large number of developers from that language's community. The module design pattern in JavaScript is one of the most used designed pattern for keeping particular pieces of code independent from other parts. Learn ho...
JavaScript Module Pattern: In-Depth. The module pattern is a common JavaScript coding pattern. It's generally well understood, but there are a number of advanced uses that have not gotten a lot of attention. In this article, I'll review the basics and cover some truly remarkable advanced topics, including one which I think is original. We will be exploring the latter three of these options later on in the book in the section Modern Modular JavaScript Design Patterns. The Module pattern is based in part on object literals and so it makes sense to refresh our knowledge of them first. Object Literals. Nov 01, 2019 - Having the power to declare private and public variables this way is what makes the module pattern arguably the most powerful design pattern in JavaScript apps. The same pattern is essentially what's being constantly used today when we import or require from nodejs modules as well as including
Aug 10, 2016 - The design patterns in question include the following: ... JavaScript modules are the most prevalently used design patterns for keeping particular pieces of code independent of other components. This provides loose coupling to support well-structured code. The Module pattern (see our Dofactory JS product) is JavaScript's manifestation of the Singleton pattern. Several other patterns, such as, Factory, Prototype, and Façade are frequently implemented as Singletons when only one instance is needed. JavaScript Design Pattern - Module Pattern. Module pattern là một loại pattern khá mạnh và được sử dụng rất phổ biến, với một số các đặc điểm sau: Cung cấp khả năng đóng gói dữ liệu với cả thuộc tính và phương thức dạng public/private, giúp tránh xung đột về tên đối với ...
Another typical pattern found in JavaScript applications is the module design pattern. Separating the code of our app into modules plays a significant role in keeping our codebase neatly organized. Some time ago, a popular approach would be to enclose a piece of code in an Immediately Invoked Function Expression ( IIFE ). Jul 11, 2018 · 3 min read Revealing module pattern is a design pattern, which let you organise your javascript code in modules, and gives better code structure. It gives you power to create... 18/3/2018 · Modular design pattern in making. The basic way to create different modules is to have separate name-space for a set of functions to avoid name collisions in the application. In JavaScript, we can create a separate object with a set of functions to achieve this.
A design pattern used to implement the concept of software modules, defined by modular programming, in a programming language with incomplete direct support for the concept. JavaScript Closures and the Module Pattern. 2012/04/30. One of the most widely used design patterns in JavaScript is the module pattern. The module pattern makes use of one of the nicer features of JavaScript - closures - in order to give you some control of the privacy of your methods so that third party applications cannot access private ... Jul 01, 2019 - The Module Pattern is one of the important patterns in JavaScript. It is a commonly used Design Pattern which is used to wrap a set of variables and functions together in a single scope. It is used…
Dec 28, 2019 - There are many patterns of JavaScript that make your code more beautiful and stable. Programming design patterns have existed for many years with a desire for a better architecture. Today, I’m going… Browse other questions tagged javascript design-patterns module-pattern revealing-module-pattern or ask your own question. The Overflow Blog Diagnose engineering process failures with data visualization. Podcast 370: Changing of the guards: one co-host departs, and a new one enters ...
Javascript Module Design Pattern
Workshop 2 Javascript Design Patterns
Javascript Design Patterns What They Are Amp How To Use Them
Creating A Loosely Coupled Amp Multi Page Js Application Using
Popular Design Patterns Revisited In Javascript
The Power Of The Module Pattern In Javascript Jsmanifest
Comprehensive Guide To Javascript Design Patterns Toptal
Javascript Design Patterns Part 1 By Anubhuti Verma Medium
Devtools Architecture Refresh Migrating To Javascript
Learning Javascript Design Patterns
Javascript Design Patterns Module Pattern Steemit
Patterns For Large Scale Javascript Application Architecture
Revealing Module Design Pattern In Javascript By Tarun
Watch Javascript Design Patterns 20 Patterns For Advancing
Space Stem Learning Centre Learn Latest Technology With
The Module Pattern In Javascript Will Roe S Blog
Javascript Design Patterns Digitalocean
Learn About Module Design Pattern In Javascript
Harnessing The Power And Convenience Of Javascript For Each
Best Design Patterns For Writing Javascript Web Applications
Module Design Pattern In Javascript Sydlabz
Exploring The Strategy Behavioral Design Pattern In Node Js
Patterns For Large Scale Javascript Application Architecture
Javascript Design Patterns Different Types Of Design Patterns
What Is This Javascript Pattern Called And Why Is It Used
Learning Javascript Design Patterns A Javascript And Jquery
0 Response to "28 Javascript Module Design Pattern"
Post a Comment