21 Random Rgb Color Javascript
Question: How to generate random rgb color using javascript Answer: We will talk about two methods to create random RGB color. We have solve one similar query that is ' How To Generate a Random Color in JavaScript ', you can read that solution. Colors on the computer are made up of a red, green, blue triplet; typically called RGB. And each of the 3 pieces can be in the range from 0 to 255. Java also allows us to create a color using floats for the values in the range of 0.0 to 1.0, or from 0% to 100% of that color.
How To Convert Rgb Colors To Hexadecimal With Javascript
For a random RGB color, we would need three numbers between 0 - 255, and for a color, in the HSL format, we need one number between 0 - 360 and two numbers between 0 - 100. If we want a random HEX color, we would need a number between 0 - 16777215, which we then convert from decimal to hexadecimal. RGB
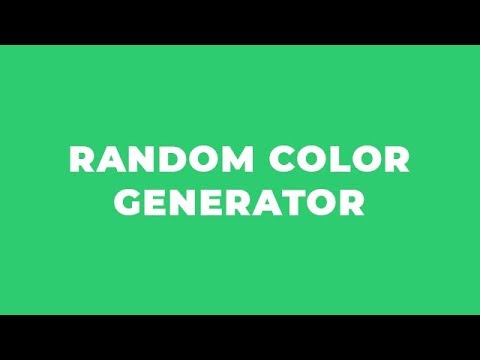
Random rgb color javascript. Options. You can pass an options object to influence the type of color it produces. The options object accepts the following properties: hue - Controls the hue of the generated color. You can pass a string representing a color name: red, orange, yellow, green, blue, purple, pink and monochrome are currently supported. If you pass a hexidecimal color string such as #00FFFF, randomColor will ... Generating random RGB colors in a javascript web app would be very easy to implement. We would just have a function with no parameters which would generate a random color from 0 - 255. Something like this: const digit = () => `$ {Math.round(Math.random() * 255)}`. and now to generate our colors, we make another function to return an array of ... Generate a random color in JavaScript. To get a random hex color in JavaScript, multiple a random value to the decimal color value 16777215 and convert it to hexadecimal value. Extract this to a function and prefix # to it.
Random RGB Color. To generate a random RGB color, first generate a random number between 0 and 255. In JavaScript, this can be done by using the Math.random() function which returns a random number between 0 (inclusive) and 1 (exclusive). We then multiply this number by 256 to get a number between 0 (inclusive) and 256 (exclusive). By default, it will give yellow color, and when you will click the button, random color will be displayed. Following is the output −. Output. Following is the snapshot of sample output after clicking the button Click the button to get random color −. Above, we successfully generated random color. The variable random_colour uses the Math object to pick three random values between 0 and 256, these values are combined as a string to form a RGB colour value. Math.random gives a random number between 0 - 1, e.g. 0.6288472721544226 - we then times it by 255 + 1 (hence the 256) which would give us 160.98490167153219 then Math.floor is used ...
The crucial line is the one here where we take our hue value from the square and subtract it by 180: var complementaryColor = getHSLAColor (color.h - 180, color.s, 25, 1); This ensures that our new randomly generated color is based on the complement of the primary square color we are starting of with. JavaScript exercises, practice and solution: Write a JavaScript function to create random background color. Random RGB Color. JavaScript. function get_random_rgb () { var rgb = [Math.floor (Math.random () * 256),Math.floor (Math.random () * 256),Math.floor (Math.random () * 256)]; return 'rgb ('+rgb.join (', ')+')'; // rgb (171, 236, 219) // return 'rgb ('+rgb.join (' ')+')'; // rgb (171 236 219) } Usage. HTML.
Javascript random color from array. Random Color From Array, I'm looking to build something with JavaScript that will pick a random value ( background-color) from an array of given hex colors and apply it to If you want to constrict it to known colors, you can create an array of the colors and randomly select it like so. var colors = ['red', 'green', 'blue', 'orange', 'yellow']; myDiv.style ... Next, we define the variables for the colors corresponding to RGB (Red, Green, Blue). In the code below, we assign a random number, ranging from 0-255 using the Math.random function. The function will throw a floating-point, pseudo-random number in the range 0-1 (inclusive of 0, but not 1) with approximately uniform distribution over that ... Quiz Program In JavaScript; Random Color Generator In JavaScript Source Code. Before sharing source code lets talk something about the program. I create just 2 divs, one for show color code one for show color. When we click on div background color also become changed. I use jQuery to create this, this is possible to create in pure javascript ...
How To Generate a Random Color in JavaScript. Chris Coyier on Dec 10, 2009 (Updated on Feb 19, 2020 ) Here's a quicky (there is a PHP version too): var randomColor = Math.floor( Math.random()*16777215).toString(16); If you'd prefer they are a bit more pleasing or need to generator colors that work together, we have an article about that. 3/12/2012 · JavaScript Code snippet to generate a random RGB color. Could be useful for supplying color values to transitions to/from with a single line of code. See Demo. var hue = 'rgb (' + (Math.floor ... Generate Single HEX Code Color Array Using Pure JavaScript: var randomColor = Math.floor(Math.random()*16777215).toString(16); Generate Single RGB Code Color Array Using Pure JavaScript: JavaScript Code snippet to generate a random RGB color. Could be useful for supplying color values to transitions to/from with a single line of code.
Generating random HSL colors in Javascript. Dec 5, 2017 ... Hue-Saturation-Lightness (HSL) is an alternative to the RGB color model. Rather than having three values that represents red, green and blue, we now have three values representing hue, saturation and lightness. string.charCodeAt(i) returns the UTF-16 code for the character at index i Bit operators work on 32 bits numbers. Any numeric operand in the operation is converted into a 32 bit number. hash << 5 is equivalent to hash * Math.pow(2, 5) (hash * 32), except the bit operator << makes sure our result is a 32 bit number.; hash & hash again, makes sure we only return a 32 bit number. This article covers the trick to generate a random color everytime in a single line of code so that you need not write down whole hexadecimal code and save time for yourselves. Tagged with beginners, webdev, javascript, 1minjs.
Tired of checking every place online to get a simple solution for generating random colors in JS? In one single post, you'll be guaranteed to get everything you need to generate random colors - with dark and light options as well! Generating a random color with JavaScript. In this tutorial, I will show you how to generate a random RGB color using JavaScript. As you probably already know, the RGB color model consists of three numbers ranging from 0 to 255. Therefore, we can easily generate a random color by generating three random numbers between 0 and 255. I have this code that uses RGB color selection and I was wondering how to make JavaScript do a random color using the RGB method and remember it throughout the code. EDIT: I tried this: var RGBColor1 = (Math.round, Math.random, 255) var RGBColor2 = (Math.round, Math.random, 255) var RGBColor3 = (Math.round, Math.random, 255) but it doesn't work.
If you ever using random color generator, perhaps you will easily copied the color hex code, by clicking the color. We can make our program do it too. If you read the CSS, you will get something interesting in line 58-61. I give the padding for .color class from pallete text. If I change the transparent into another color, you will see this. When the background becomes dark then text color becomes white, and when background color becomes any light color then the text becomes black. So, Today I am sharing Random Text Color Contrast Generator Using JavaScript and CSS. This program will help to create a good color and background combination. Also, you can learn to create this by yourself. How To Generate a Random Color in JavaScript, get rgb colour based on a string, JavaScript to generate random hex codes of color, string to color javascript, random light color javascript, random material color generator javascript example. Checkout more articles on JavaScript.
Math.random is a JavaScript function that returns a random number from 0 to 1(not including 1). Math.floor function returns the largest integer less than or equal to a number. Generating random color. This is a simple example to generate random colors in javascript. Random Color Generator. Hex Color: 7D84EA. RGB Color: rgb (125,132,234) RandomColors is a free online random HEX, RGB color code generator. You can refresh this page or click Random Color Generator button to generate various random colors. AdEx Adview. Randomly shuffling an array of literals in JavaScript; Changing the color of a single X-axis tick label in Matplotlib; Changing the color and marker of each point using Seaborn jointplot; Creating permutations by changing case in JavaScript; Hexadecimal color to RGB color JavaScript; RGB color to hexadecimal color JavaScript
Random Color Generator Expo App Dev Community
How To Sort Colors Properly Mathematica Stack Exchange
9 Useful Javascript Color Libraries Bashooka
Build A Hex Color Generator With Html Css Js Enlight
Javascript Math Create Random Background Color W3resource
Javascript Random Gradient Generator Auto Generate Gradients
Generating Random Color Values Using Javascript Sitepoint
Generative Art With P5 Js Program Your Own Art By Kelly
Random Password Generator With Javascript
React Random Color Change Javascript The Freecodecamp Forum
React Random Background Color To Each Element For Loop
How To Change Text Color Depending On Background Color Using
Random Color Generator Using Javascript
Random Color Generator In Javascript Generate Random Colours
Change Background Color Randomly Using Js Code Example
Build A Random Hex Color Generator With Vanilla Javascript
Average 2 Hex Colors Together In Javascript Stack Overflow
Laporeon Colorgenerator Githubmemory
0 Response to "21 Random Rgb Color Javascript"
Post a Comment