22 Find Duplicates In Two Arrays Javascript
Get code examples like "how to compare two arrays and remove duplicates in javascript" instantly right from your google search results with the Grepper Chrome Extension. The quickest way is to use two "for" loops. Another approach would be to concatentate the two arrays, sort the new array, then delete all items that have duplicates. There are a couple other ways to go at this problem, but Dan's would probably be what I tried first, though it will be slow if the arrays are real large.
Find Common Items From Two Arrays In Javascript Clue Mediator
In the above program, two arrays are merged together and Set is used to remove duplicate items from an array. The Set is a collection of unique values. Here, Two array elements are merged together using the spread syntax ... The array is converted to Set and all the duplicate elements are automatically removed.
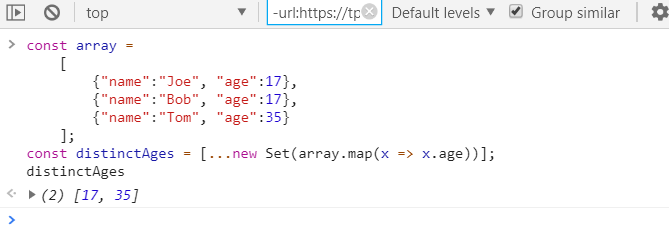
Find duplicates in two arrays javascript. There are a couple of ways to count duplicate elements in a javascript array. by using the forEach or for loop. Given an array containing integers, strings, or a mixture of data types, find the first duplicate element in the array for which the second occurrence has the minimal index. If there are more than one duplicated elements, return the element for which the second occurrence has a smaller index than the second occurrence of the other element. I have two arrays like this: var x = ['1','2','6']; var y = ['4', '5','6']; How do I find duplicates in two arrays in pure JavaScript and I would like to avoid using a loop? Output - duplicates: 6
How to find duplicates in a given array on O (n^2) In the first solution, we compare each element of the array to every other element. If it matches then its duplicate and if it doesn't, then there are no duplicates. This is also known as a brute force algorithm to find duplicate objects from Java array. In vanilla JavaScript, there are multiple ways available to remove duplicate elements from an array. You can either use the filter() method or the Set object to remove all repeated items from an array. How to compare two arrays in ... and fastest way to compare two arrays is to convert them to strings by using the JSON.stringify() method and then use the comparison operator to · Remove duplicates from array of objects javascript...
See the Pen JavaScript - Find duplicate values in a array - array-ex- 20 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus Previous: write a JavaScript program to compute the sum of each individual index value from the given arrays. toFindDuplicates(); function toFindDuplicates(element, index) { let arry = [1, 2, 1, 3, 4, 3, 5]; let resultToReturn = false; for (let i = 0; i arry.length; i++) { // nested for loop for (let j = 0; j arry.length; j++) { // prevents the element from comparing with itself if (i !== j) { // check if elements' values are equal if (arry[i] === arry[j]) { // duplicate element present resultToReturn = true; // terminate inner loop break; } } } // terminate outer loop if … Mar 11, 2020 - Get code examples like "compare two arrays and remove duplicates javascript" instantly right from your google search results with the Grepper Chrome Extension.
EDIT As stated in the comments this function does return an array with uniques, the question however asks to find the duplicates. in that case a simple modification to this function allows to push the duplicates into an array, then using the previous function toUnique removes the duplicates of the duplicates. Read this tutorial and find useful information about the simplest methods that are used for merging two arrays and removing duplicate items in JavaScript. Removing duplicates. To remove duplicates in an array we have many logical methods, but advanced javascript has provided some methods so that the task of removing duplicates has become very simple.Some of those methods are set() and filter().For better understanding lets' discuss each method individually. Set() The important use of the set() method is that it only allows unique values.
Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Checking two arrays for matches. Ask Question Asked 6 years, 9 months ago. ... If we were looking for duplicates in the same array, it works. sorry. \$\endgroup\$ - apnorton Nov 12 '14 at 17:20 ... Browse other questions tagged javascript array or ask your own question. 5/12/2016 · Add a comment. |. 1. var array1 = [1, 2, 3, 4, 5]; var array2 = [3, 4, 5]; var duplicates = array1.filter (function (val) { return array2.indexOf (val) != -1; }); console.log (duplicates); Here what you can do to find duplicates in two different arrays. Reference for the filter function.
See the Pen JavaScript - Merge two arrays and removes all duplicates elements - array-ex- 30 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to fill an array with values (numeric, string with one character) on supplied bounds. The root cause is that you directly splice items from array b while you are in the for loop and pre condition is a and b have same number of items. Compare two Javascript Arrays and remove Duplicates. Ask Question How to compare two objects and remove the duplicate objects by value in javascript-2. Another alternate method to find duplicate values in an array using JavaScript is using reduce method. Using reduce method you can set the accumulator parameter as an empty array. On each iteration check if it already exists in the actual array and the accumulator array and return the accumulator. Here is how the code looks:
21/7/2021 · Method 2: Create an empty object and loop through first array. Check if the elements from the first array exist in the object or not. If it doesn’t exist then assign properties === elements in the array. Loop through second array and check if elements in the second array exists on created object. If element exist then return true else return false. If the arrays can contain duplicates, removing those duplicates before concatenation might be a good idea too. E.g. [8, 8, 1], [5, 4, 8], [1, 2, 9] would still have three 8s in the final count, even though 3 is present in only two arrays. \$\endgroup\$ - user27318 Jul 7 '15 at 22:40 Dec 31, 2019 - Comparison of arrays by using forEach method. This question is most commonly asked in interviews. There are different ways to achieve these, But I’m showing here one of them. ... Now we are writing the compare function it helps us to compare above two arrays.
Summary: in this tutorial, you will learn how to remove duplicates from an array in JavaScript. 1) Remove duplicates from an array using a Set. A Set is a collection of unique values. To remove duplicates from an array: First, convert an array of duplicates to a Set. The new Set will implicitly remove duplicate elements. Nov 15, 2017 - I need to check a JavaScript array to see if there are any duplicate values. What's the easiest way to do this? I just need to find what the duplicated values are - I don't actually need their inde... We can simply transform the array into a Set and create a new collection without duplicates. Then we can compare the size of the Set collection with the size of the original array and detect if it ...
Find duplicates in a given array when elements are not limited to a range. Given an array of n integers. The task is to print the duplicates in the given array. If there are no duplicates then print -1. Note: The duplicate elements can be printed in any order. Recommended: Please try your approach on {IDE} first, before moving on to the ... There are various different methods for finding the duplicates in the array. We will discuss two ways for finding the duplicates in the array. Using Set: The Set object lets you store unique values of any type, whether primitive values or object references. This is the easiest method to remove the duplicate element and get unique elements from ... Solution 1: Approach: The elements in the array is from 0 to n-1 and all of them are positive. So to find out the duplicate elements, a HashMap is required, but the question is to solve the problem in constant space. There is a catch, the array is of length n and the elements are from 0 to n-1 (n elements). The array can be used as a HashMap.
If the length of the Set and the array are not the same this function will return true, indicating that the array did contain duplicates.Otherwise, if the array and the Set are the same length the function will return false and we can be certain that the original array contained no duplicate values!. I really like this second approach for how concise and expressive it is, but you might run ... 16/11/2020 · const yourArray = [1, 1, 2, 3, 4, 5, 5] const yourArrayWithoutDuplicates = [... new Set (yourArray)] let duplicates = [... yourArray] yourArrayWithoutDuplicates. forEach ((item) => { const i = duplicates. indexOf (item) duplicates = duplicates. slice (0, i) . concat (duplicates. slice (i + 1, duplicates. length)) }) console. log (duplicates) //[ 1, 5 ] Mar 21, 2019 - Recently in one of my projects I had to remove duplicates from an array. Instead of looking up a npm package that could do it for me I chose to implement it myself. Here’s what I learned about this…
8 Ways to Remove Duplicate Array Values in JavaScript. 1. De-Dupe Arrays with Set Objects. Set objects objects are my go-to when de-duplicating arrays. Try out this example in your browser console, spoiler - Set objects work great for primitive types, but may not support use cases requiring the de-duplication of objects in an array. Oct 29, 2014 - How may I retrieve an element that exists in two different arrays of the same document. For example. In Posts collection, document has the fields 'interestbycreator' and 'interestbyreader.' Each f... If a match is found, print the duplicate element. In the above array, the first duplicate will be found at the index 4 which is the duplicate of the element (2) present at index 1. So, duplicate elements in the above array are 2, 3 and 8. Algorithm. Declare and initialize an array. Duplicate elements can be found using two loops.
3/7/2021 · How to find duplicates in an array using JavaScript. There are multiple methods available to check if an array contains duplicate values in JavaScript. You can use the indexOf () method, the Set object, or iteration to identify repeated items in an array. Problem : Given an array of positive integers find all the duplicate elements. Algorithm : Iterate over the array using forEach. Find if there is a duplicate for the element using indexOf; indexOf takes two arguments first the element and the second one is the starting index; We provide the starting index as the index + 1 where index is the index of the current element Start with two arrays of strings, a and b, each in alphabetical order, possibly with duplicates. Return the count of the number of strings which appear in both arrays. The best "linear" solution makes a single pass over both arrays, taking advantage of the fact that they are in alphabetical order. Here is my code:
How To Find Duplicate Objects In An Array You'll be keeping two empty arrays, one for unique items and another for duplicate items. You'll iterate over the given objects array and check if the unique items array contains the iterated object. If found you'll push that to the duplicate array else push it to unique array list.
C Program To Delete Duplicate Elements From An Array
Find Duplicate Values In Two Arrays Javascript
Find Intersection Of Two Arrays Java Program Explained With
Javascript Array Merge Two Arrays And Removes All Duplicates
Javascript Array Distinct Ever Wanted To Get Distinct
How To Remove Array Duplicates In Es6 By Samantha Ming
Find Duplicate Elements In An Array Java Program
Removing Duplicates In An Array Of Objects In Js With Sets
How Do I Compare Two Arrays In Javascript 30 Seconds Of Code
Compare Two Arrays To Show Difference In Excel
How To Remove Duplicate Objects From A Javascript Array
Java Exercises Find The Duplicate Values Of An Array Of
Java67 How To Compare Two Arrays In Java To Check If They
How To Check Duplicate Elements In An Array More Than Once
Removing Duplicates In An Array Of Objects In Js With Sets
Find Common Elements In Two Arrays In C Kodlogs
How To Remove Duplicates From An Array In Javascript Hello
Java67 How To Compare Two Arrays In Java To Check If They
Find The Pair With The Smallest Difference In Two Unsorted
How To Remove Array Duplicates In Es6 By Samantha Ming
How To Compare Two Arrays In Javascript
0 Response to "22 Find Duplicates In Two Arrays Javascript"
Post a Comment