25 Javascript Prototype Inheritance Vs Class Inheritance
JavaScript is a prototypal object-oriented language, which means objects can inherit directly other objects. This is in contrast with the classical languages such as Java, where a subclass inherits from a superclass, and objects are instances of classes. However, the expressive power of JavaScript do allow both classical and prototypal inheritance. JavaScript is dynamic and before ES6 JavaScript did not provide a class implementation. The inheritance was mainly prototype-based inheritance. prototype-based inheritance In prototype-based inheritance, the inheritance is done in multiple steps. The following code block shows how the ScienceStudent inherits properties from the Student using the prototypal inheritance: function Student ...
Prototypes As Classes An Introduction To Javascript Inheritance
Objects are mutable in JavaScript, so we can augment the new instances, giving them new fields and methods. These can then act as prototypes for even newer objects. We don't need classes to make lots of similar objects… Objects inherit from objects. What could be more object oriented than that?
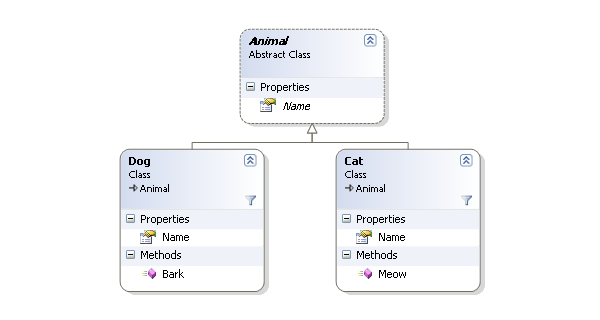
Javascript prototype inheritance vs class inheritance. 2 weeks ago - Prototype inheritance and prototype chain explained in detail. Learn the secret behind classes in JavaScript. 12/12/2010 · In JavaScript, every object is at the same time an instance and a class. To do inheritance, you can use any object instance as a prototype. In Python, C++, etc.. there are classes, and instances, as separate concepts. In order to do inheritance, you have to use the base class to create a new class, which can then be used to produce derived instances. This post is designed to be read after you read JavaScript Inheritance and the Prototype Chain. Previously we looked at how to accomplish inheritance in JavaScript using both ES5 and ES6. In our example, we abstracted the common features amongst every animal (name, energy, eat, sleep, and play) to an Animal base class. Then, whenever we wanted ...
Prototypal Inheritance As opposed to Classical Inheritance, Prototypal Inheritance does not deal with increasing layers of abstraction. An Object is either an abstraction of a real-world thing, same as before, or is a direct copy of another Object (in other words, a Prototype). Aug 04, 2020 - Continue to learn about inheritance with Educative’s course Learn Object-Oriented Programming in JavaScript. This course has in-depth explanations of advanced OOP topics like prototyping, chaining, restricted classes. By the end, you’ll have hands-on project experience with OOP techniques ... This tutorial discusses the differences in syntax between creating objects in javascript with the class keyword or with the prototype methods.Examples are gi...
Feb 10, 2013 - From time to time people mention ... class-based inheritance and prototype-based inheritance? Although each “object-oriented” programming language has its own particular set of semantics, the majority in popular use have “classes.” A class is an entity responsible ... 27/10/2013 · As you can see prototypal inheritance and classical inheritance are two different paradigms of inheritance. Some languages like Self, Lua and JavaScript support prototypal inheritance. However most languages like C++, Java and C# support classical inheritance. A Quick Overview of Object-Oriented Programming Mar 26, 2019 - This post is designed to be read ... and the Prototype Chain. Previously we looked at how to accomplish inheritance in JavaScript using both ES5 and ES6. In our example, we abstracted the common features amongst every animal (name, energy, eat, sleep, and play) to an Animal base class...
JavaScript does not have classes unlike other languages. It uses the concept of prototypes and prototype chaining for inheritance. Prototypal inheritance is all about objects. Objects inherit properties from other objects. If you want a new class to reuse the functionality of an existing class, you can create a new class that extends the existing class. This is called classical inheritance. JavaScript doesn't use classical inheritance. Instead, it uses prototypal inheritance. In prototypal inheritance, an object "inherits" properties from another object via ... Prototypal inheritance in JavaScript. Another and more modern approach to inheritance in Javascript is the prototypal inheritance. There are few subtle differences with the former approach: there is no constructor function, rather there is an explicit instance of the prototype
JavaScript Prototype Inheritance 5 years ago July 31st, 2016 6 min read Javascript Prototype inheritance vs Class inheritance. As a developer comes from .NET/Java background, class inheritance is quite familiar concept and a very neutral way to understand inheritance. We usually define a base class, and create another class to inherit from it. Jul 06, 2018 - Since I last wrote about inheritance in JavaScript (JS), ECMAScript 2015, a.k.a. ES6, introduced class syntax in an effort to bridge the gap between JS's Prototypal Inheritance and the traditional Classical Inheritance of Object-Oriented (OO) programming languages like Java and C++. Having ... Javascript, and its prototype chain, make an inheritance-based architecture possible. I will be doing a deep dive into an alternative to inheritance called composition. This approach makes use of the fact that in Javascript, functions are first-class objects. Composition is a highly flexible and modular architecture that many experts believe ...
The "Classical vs Prototypal Inheritance" Lesson is part of the full, Deep JavaScript Foundations, v3 course featured in this preview video. Here's what you'd learn in this lesson: Kyle clarifies the difference between classical and prototypal inheritance in terms of copying or linking ... While prototypal inheritance is unique to JavaScript, it is not so special that it can't be compared to inheritance in other programming languages. In Ruby, for example, there are classes;... Now if we extend this Media() and create a new class then that new class will have the same prototype chain as we saw above. Class inheritance. Though they seem to be similar, there is a minor difference, between the Class-based approach and the prototype-based approach.
Mar 28, 2019 - A really great aspect of inheritance is that JavaScript lets you modify or expand on the features of a class even after you have defined it. JavaScript will look up the prototype when trying to access properties on an object. So, you can alter classes at runtime! Nov 28, 2018 - Nevertheless, before ES6 there were no classes in JS. So how we can manage inheritance when we only have objects? In the same way that we had classes, in prototype inheritance we define objects like apple and fruit and link them together to allowing the use of common methods and properties. Jul 20, 2021 - JavaScript is an object-based language based on prototypes, rather than being class-based. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values.
Inheritance and the prototype chain JavaScript is a bit confusing for developers experienced in class-based languages (like Java or C++), as it is dynamic and does not provide a class implementation per se (the class keyword is introduced in ES2015, but is syntactical sugar, JavaScript remains prototype-based). Prototypal inheritance is a language feature that helps in that. [[Prototype]] In JavaScript, objects have a special hidden property [[Prototype]] (as named in the specification), that is either null or references another object. That object is called "a prototype": Hence, a prototype is a generalization. The difference between classical inheritance and prototypal inheritance is that classical inheritance is limited to classes inheriting from other classes while prototypal inheritance supports the cloning of any object using an object linking mechanism.
May 28, 2020 - Learn more about prototypes and how to use them for inheritance in JavaScript. See how the prototypical approach is different from class-based inheritance. Prototypes and inheritance represent some of the most complex aspects of JavaScript, but a lot of JavaScript's power and flexibility comes from its object structure and inheritance, and it is worth understanding how it works. In a way, you use inheritance all the time. Whenever you use various features of a Web API , or methods/properties ... 10/12/2019 · Today we are going do some code to implement inheritance using both prototype(ES5) and class(ES6). The prototype is an object that is associated with every functions and objects by default in JavaScript, where function's prototype property is accessible and modifiable and object's prototype property (aka attribute) is not visible.
In JavaScript, every object is at the same time an instance and a class. To do inheritance, you can use any object instance as a prototype. In Python, C++, etc.. there are classes, and instances, as separate concepts. 1 week ago - JavaScript’s class inheritance uses the prototype chain to wire the child `Constructor.prototype` to the parent `Constructor.prototype` for delegation. Usually, the `super()` constructor is also called. Those steps form single-ancestor parent/child hierarchies and create the tightest coupling ... Now since ES6, we have the class keyword. According to MDN: JavaScript classes, introduced in ECMAScript 2015, are primarily syntactical sugar over JavaScript's existing prototype-based inheritance. The class syntax does not introduce a new object-oriented inheritance model to JavaScript. Lets revisit the Person example, using this new syntax
We want to make this open-source project available for people all around the world. Help to translate the content of this tutorial to your language! Prototype-based Object Inheritance. JavaScript supports object inheritance via something known as prototype. There is an object property called prototype attached to each object. Working with class and extends keywords is easy - but actually understanding how prototype-based inheritance works is not trivial. JavaScript Prototypal Inheritance and What ES6 Classes Have to Say About It 2012/10/31 Many people come to JavaScript from other object-oriented programming languages such as Java or C++ and are confused as heck.
It also demonstrates one of the key differences in the prototype inheritance model: Prototypes are object instances, not types. Prototypes vs. Classes. The most important difference between class- and prototype-based inheritance is that a class defines a type which can be instantiated at runtime, whereas a prototype is itself an object instance. Class is just sugar for using the prototype. Under the skin, it used the prototype to achieve the concept of inheritance. Let's try the same class Media and see what it has internally. As you could see internally Media has a proto for its method and within that, it references the main Object properties. More often than not, when JavaScript folks say classical inheritance, they mean Java or C++ style inheritance, where a class is something that exists only at compile-time, and inheritance happens statically at compile-time. And when they say prototypal inheritance, they mean JavaScript's implementation where one object can delegate property ...
Nov 27, 2018 - Before ES6 classes, in JavaScript, inheritance was quite the task as you can see above. You need to understand now only when to use inheritance, but also a nice mix of .call, Object.create, this, and FN.prototype - all pretty advanced JS topics. Let’s see how we’d accomplish the same thing ... Class inheritance may or may not use the class keyword from ES6. Prototypal Inheritance: Instances inherit directly from other objects. Instances are typically instantiated via factory functions or Object.create(). Instances may be composed from many different objects, allowing for easy selective inheritance. JavaScript objects use prototype ... Jul 15, 2021 - This article has covered the remainder of the core OOJS theory and syntax that we think you should know now. At this point you should understand JavaScript object and OOP basics, prototypes and prototypal inheritance, how to create classes (constructors) and object instances, add features to ...
JavaScript is a prototype-based language, meaning object properties and methods can be shared through generalized objects that have the ability to be cloned and extended. This is known as prototypical inheritance and differs from class inheritance. This is a basic but accurate simulation of the prototypal inheritance in JavaScript using the prototype chain. Summing Up. In this article we have gone through the basics of ES6 classes and prototypal inheritance,. Hopefully you have learnt a thing or two from reading the article. If you have any questions, leave them below in the comments section. Nov 27, 2018 - Before ES6 classes, in JavaScript, inheritance was quite the task as you can see above. You need to understand now only when to use inheritance, but also a nice mix of .call, Object.create, this, and FN.prototype - all pretty advanced JS topics. Let’s see how we’d accomplish the same thing ...
What is Inheritance. Inheritance in most class-based object-oriented languages is a mechanism in which one object acquires all the properties and behaviors of another object. JavaScript is not a class-based language although class keyword is introduced in ES2015, it is just syntactical layer. JavaScript still works on prototype chain.
The Prototype Pattern Learning Javascript Design Patterns
Javascript Inheritance Prototype Vs Class Dev Community
What Is Prototypal Inheritance In Javascript We Love Js
Javascript Es2015 Classes And Prototype Inheritance Part 1
The Ultimate Guide To Javascript Prototypal Inheritance
Javascript Inheritance Delegation Patterns And Object
Javascript Prototypal Inheritance In 2018 Html Goodies
What Makes Javascript Javascript Prototypal Inheritance
Prototypes As Classes An Introduction To Javascript Inheritance
Inheritance In Javascript Coldfusion Angularjs
Object Oriented Javascript Inheritance Desalasworks
Classes In Javascript Dev Community
Making Sense Of Es6 Class Confusion Toptal
Making Sense Of Es6 Class Confusion Toptal
Class Inheritance Diagram In Javascript Mindfusion Company Blog
Stephen Walther On Asp Net Mvc Two Methods Of Creating
Inheritance In Javascript Programmer Sought
Understanding Javascript S Prototypal Inheritance Egghead Io
How Does Javascript Prototype Work Stack Overflow
Classical Vs Prototypal Inheritance Dev Community
Advanced Javascript Objects And Functions D Z Notes Beta
Understanding Javascript S Prototypal Inheritance Egghead Io
0 Response to "25 Javascript Prototype Inheritance Vs Class Inheritance"
Post a Comment